How to Get Started with Docker and Kubernetes: A Comprehensive Guide for Beginners
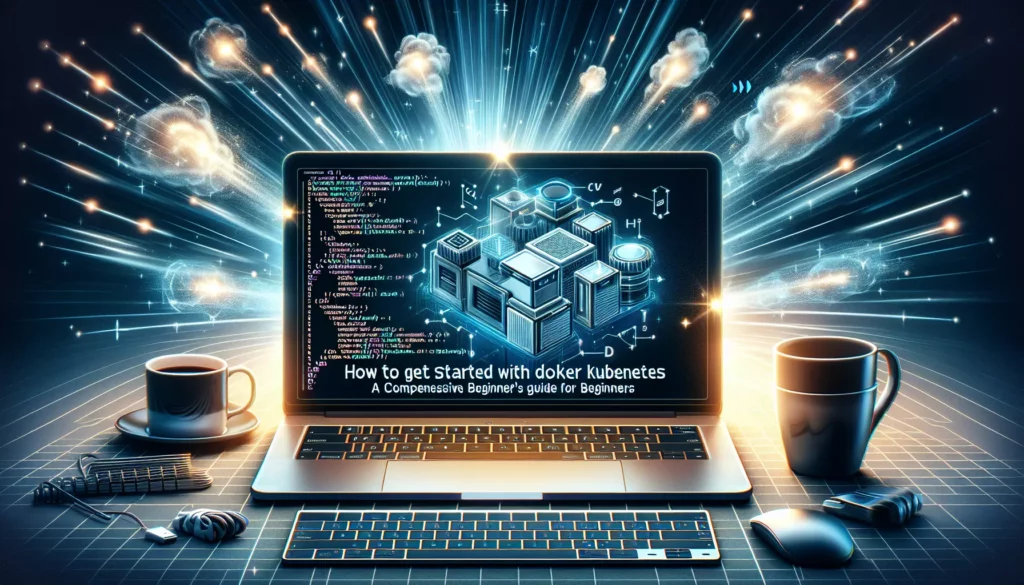
In today’s fast-paced world of software development and deployment, containerization and orchestration have become essential skills for developers and DevOps engineers alike. Docker and Kubernetes are two of the most popular technologies in this space, revolutionizing the way we build, ship, and run applications. This comprehensive guide will walk you through the basics of Docker and Kubernetes, helping you understand their core concepts and get started with practical implementations.
Table of Contents
- Introduction to Containerization and Orchestration
- Docker Basics
- Installing Docker
- Essential Docker Commands
- Creating a Dockerfile
- Introduction to Docker Compose
- Introduction to Kubernetes
- Setting Up a Kubernetes Cluster
- Understanding Kubernetes Objects
- Working with kubectl
- Deploying an Application to Kubernetes
- Best Practices and Tips
- Conclusion
1. Introduction to Containerization and Orchestration
Before diving into Docker and Kubernetes, it’s essential to understand the concepts of containerization and orchestration.
Containerization
Containerization is a lightweight alternative to full machine virtualization that involves encapsulating an application in a container with its own operating environment. This approach offers several benefits:
- Consistency across different environments
- Improved resource utilization
- Faster application deployment and scaling
- Isolation between applications
Orchestration
Container orchestration refers to the automated arrangement, coordination, and management of software containers. It addresses challenges such as:
- Deploying containers across multiple hosts
- Scaling containers up or down based on demand
- Load balancing between containers
- Managing container lifecycle and health
Now that we have a basic understanding of these concepts, let’s explore Docker and Kubernetes in detail.
2. Docker Basics
Docker is an open-source platform that automates the deployment, scaling, and management of applications using containerization. It provides a way to package applications and their dependencies into standardized units called containers.
Key Docker Concepts
- Docker Engine: The runtime that runs and manages containers.
- Docker Image: A lightweight, stand-alone, executable package that includes everything needed to run a piece of software, including the code, runtime, system tools, libraries, and settings.
- Docker Container: A runtime instance of a Docker image.
- Dockerfile: A text file that contains instructions for building a Docker image.
- Docker Registry: A repository for storing and distributing Docker images.
3. Installing Docker
To get started with Docker, you’ll need to install it on your system. The installation process varies depending on your operating system.
For Windows and macOS
- Download Docker Desktop from the official Docker website.
- Run the installer and follow the on-screen instructions.
- Once installed, launch Docker Desktop.
For Linux
For Linux, you’ll need to install Docker Engine. Here’s an example for Ubuntu:
$ sudo apt-get update
$ sudo apt-get install docker-ce docker-ce-cli containerd.io
After installation, verify that Docker is installed correctly by running:
$ docker --version
$ docker run hello-world
4. Essential Docker Commands
Now that you have Docker installed, let’s explore some essential commands to get you started:
docker pull <image>
: Download an image from a registrydocker run <image>
: Create and start a container from an imagedocker ps
: List running containersdocker ps -a
: List all containers (including stopped ones)docker stop <container>
: Stop a running containerdocker rm <container>
: Remove a containerdocker images
: List available imagesdocker rmi <image>
: Remove an imagedocker build -t <tag> .
: Build an image from a Dockerfile
Let’s try a simple example:
$ docker pull nginx
$ docker run -d -p 8080:80 --name my-nginx nginx
$ docker ps
$ curl http://localhost:8080
$ docker stop my-nginx
$ docker rm my-nginx
This sequence of commands pulls the Nginx image, runs a container, verifies it’s running, tests the web server, and then stops and removes the container.
5. Creating a Dockerfile
A Dockerfile is a text document that contains all the commands a user could call on the command line to assemble an image. Let’s create a simple Dockerfile for a Python application:
# Use an official Python runtime as a parent image
FROM python:3.9-slim
# Set the working directory in the container
WORKDIR /app
# Copy the current directory contents into the container at /app
COPY . /app
# Install any needed packages specified in requirements.txt
RUN pip install --no-cache-dir -r requirements.txt
# Make port 80 available to the world outside this container
EXPOSE 80
# Define environment variable
ENV NAME World
# Run app.py when the container launches
CMD ["python", "app.py"]
To build an image from this Dockerfile:
$ docker build -t my-python-app .
And to run a container from this image:
$ docker run -p 4000:80 my-python-app
6. Introduction to Docker Compose
Docker Compose is a tool for defining and running multi-container Docker applications. It uses YAML files to configure application services and performs the creation and start-up process of all the containers with a single command.
Here’s a simple docker-compose.yml
file:
version: '3'
services:
web:
build: .
ports:
- "5000:5000"
redis:
image: "redis:alpine"
To run this multi-container application:
$ docker-compose up
This command builds the images if they don’t exist and starts the containers.
7. Introduction to Kubernetes
Kubernetes, often abbreviated as K8s, is an open-source container orchestration platform that automates many of the manual processes involved in deploying, managing, and scaling containerized applications.
Key Kubernetes Concepts
- Cluster: A set of nodes that run containerized applications managed by Kubernetes.
- Node: A worker machine in Kubernetes, part of a cluster.
- Pod: The smallest deployable units of computing that you can create and manage in Kubernetes.
- Service: An abstract way to expose an application running on a set of Pods as a network service.
- Deployment: Describes a desired state for a set of Pods, allowing for easy updates and rollbacks.
8. Setting Up a Kubernetes Cluster
There are several ways to set up a Kubernetes cluster. For learning purposes, we’ll use Minikube, which creates a single-node Kubernetes cluster on your local machine.
Installing Minikube
- Install a hypervisor like VirtualBox or HyperKit.
- Download and install Minikube from the official Minikube website.
- Start Minikube:
$ minikube start
Installing kubectl
kubectl is the Kubernetes command-line tool. Install it following the instructions for your operating system from the official Kubernetes documentation.
9. Understanding Kubernetes Objects
Kubernetes objects are persistent entities in the Kubernetes system that represent the state of your cluster. Let’s explore some fundamental objects:
Pods
A Pod is the smallest deployable unit in Kubernetes. It can contain one or more containers. Here’s a simple Pod definition:
apiVersion: v1
kind: Pod
metadata:
name: nginx-pod
spec:
containers:
- name: nginx
image: nginx:1.14.2
ports:
- containerPort: 80
Deployments
Deployments provide declarative updates for Pods and ReplicaSets. Here’s an example:
apiVersion: apps/v1
kind: Deployment
metadata:
name: nginx-deployment
spec:
replicas: 3
selector:
matchLabels:
app: nginx
template:
metadata:
labels:
app: nginx
spec:
containers:
- name: nginx
image: nginx:1.14.2
ports:
- containerPort: 80
Services
Services define a logical set of Pods and a policy by which to access them. Here’s a simple Service definition:
apiVersion: v1
kind: Service
metadata:
name: nginx-service
spec:
selector:
app: nginx
ports:
- protocol: TCP
port: 80
targetPort: 80
10. Working with kubectl
kubectl is the command-line interface for running commands against Kubernetes clusters. Here are some essential kubectl commands:
kubectl get pods
: List all pods in the current namespacekubectl get services
: List all serviceskubectl create -f <filename>
: Create a resource from a filekubectl apply -f <filename>
: Apply changes to a resourcekubectl delete -f <filename>
: Delete a resourcekubectl logs <pod-name>
: View logs for a specific podkubectl exec -it <pod-name> -- /bin/bash
: Open a shell in a pod
11. Deploying an Application to Kubernetes
Let’s deploy a simple application to our Kubernetes cluster. We’ll use the Nginx deployment we defined earlier.
- Save the deployment YAML to a file named
nginx-deployment.yaml
- Apply the deployment:
$ kubectl apply -f nginx-deployment.yaml
- Verify the deployment:
$ kubectl get deployments
$ kubectl get pods
- Create a service to expose the deployment:
$ kubectl expose deployment nginx-deployment --type=LoadBalancer --port=80
- Check the service:
$ kubectl get services
If you’re using Minikube, you can access the service using:
$ minikube service nginx-deployment
12. Best Practices and Tips
As you continue your journey with Docker and Kubernetes, keep these best practices in mind:
- Use official base images: They are maintained and regularly updated for security.
- Minimize layers in Dockerfiles: Combine commands to reduce the number of layers and image size.
- Don’t run containers as root: Use the USER instruction in your Dockerfile to switch to a non-root user.
- Use namespaces in Kubernetes: They help organize and isolate resources within a cluster.
- Implement resource limits: Set CPU and memory limits for your containers to prevent resource exhaustion.
- Use liveness and readiness probes: They help Kubernetes understand the health of your applications.
- Keep your clusters up to date: Regularly update Kubernetes and your container images to benefit from the latest features and security patches.
- Use version control for your Kubernetes manifests: Treat your infrastructure as code.
13. Conclusion
Docker and Kubernetes have revolutionized the way we develop, deploy, and manage applications. This guide has provided you with a solid foundation to start your journey into the world of containerization and orchestration. Remember, mastering these technologies takes time and practice, so don’t be discouraged if everything doesn’t click immediately.
As you continue to learn, consider exploring more advanced topics such as:
- Kubernetes Helm for package management
- Istio for service mesh
- Prometheus and Grafana for monitoring
- CI/CD pipelines with Docker and Kubernetes
The cloud-native landscape is vast and constantly evolving, offering endless opportunities for learning and growth. Keep experimenting, stay curious, and happy containerizing!
Remember, AlgoCademy is here to support your journey in coding education and programming skills development. While this guide focuses on Docker and Kubernetes, the problem-solving and algorithmic thinking skills you develop here will be invaluable as you work with these technologies. Continue to leverage AlgoCademy’s interactive coding tutorials and AI-powered assistance to enhance your overall programming capabilities, which will undoubtedly complement your Docker and Kubernetes skills.