How to Find the Area of a Rectangle: A Step by Step Guide with Coding Examples
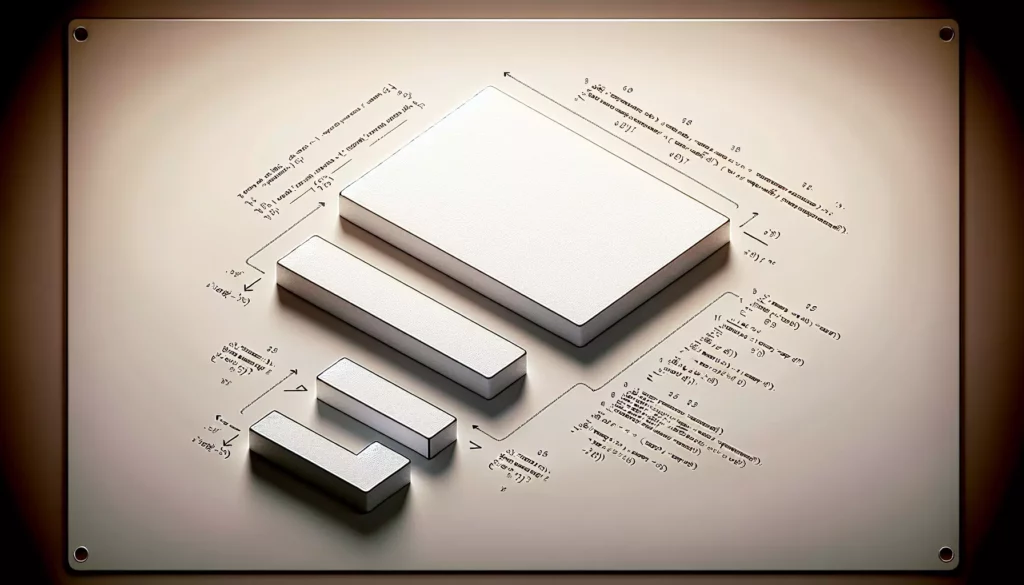
Finding the area of a rectangle is one of the fundamental mathematical concepts that everyone learns in school. It’s also a perfect example to introduce programming concepts to beginners. In this comprehensive guide, we’ll explore how to calculate the area of a rectangle both mathematically and programmatically, providing coding examples in various languages along the way.
Understanding Rectangle Area: The Basic Formula
Before diving into code, let’s review the mathematical foundation. The area of a rectangle is calculated using a simple formula:
Area = Length × Width
Where length and width are the dimensions of the rectangle. This formula works because a rectangle can be thought of as a collection of unit squares arranged in rows and columns. The number of squares equals the product of the length and width.
Calculating Rectangle Area in Different Programming Languages
Let’s see how we can implement this formula in various programming languages. This is an excellent starting point for beginners learning to code.
Python Implementation
Python’s simplicity makes it ideal for beginners to understand the concept:
def calculate_rectangle_area(length, width):
area = length * width
return area
# Example usage
length = 5
width = 3
area = calculate_rectangle_area(length, width)
print(f"The area of a rectangle with length {length} and width {width} is {area} square units.")
This Python function takes two parameters: length and width, multiplies them, and returns the area. The example usage demonstrates how to call the function with specific values.
JavaScript Implementation
For web developers, here’s how you might calculate a rectangle’s area in JavaScript:
function calculateRectangleArea(length, width) {
return length * width;
}
// Example usage
const length = 5;
const width = 3;
const area = calculateRectangleArea(length, width);
console.log(`The area of a rectangle with length ${length} and width ${width} is ${area} square units.`);
Java Implementation
Java’s object oriented approach provides another perspective:
public class RectangleArea {
public static double calculateArea(double length, double width) {
return length * width;
}
public static void main(String[] args) {
double length = 5.0;
double width = 3.0;
double area = calculateArea(length, width);
System.out.println("The area of a rectangle with length " + length +
" and width " + width + " is " + area + " square units.");
}
}
Creating a Rectangle Class
As you advance in programming, you’ll learn about object oriented programming. Let’s create a Rectangle class in Python that encapsulates the properties and behaviors of a rectangle:
class Rectangle:
def __init__(self, length, width):
self.length = length
self.width = width
def calculate_area(self):
return self.length * self.width
def calculate_perimeter(self):
return 2 * (self.length + self.width)
def is_square(self):
return self.length == self.width
# Example usage
rectangle = Rectangle(5, 3)
print(f"Area: {rectangle.calculate_area()} square units")
print(f"Perimeter: {rectangle.calculate_perimeter()} units")
print(f"Is it a square? {rectangle.is_square()}")
This class not only calculates the area but also includes methods for perimeter calculation and checking if the rectangle is a square. This demonstrates how programming can extend basic mathematical concepts.
Handling Edge Cases and Input Validation
In real world applications, input validation is crucial. Let’s enhance our Python function to handle potential issues:
def calculate_rectangle_area(length, width):
# Input validation
if not (isinstance(length, (int, float)) and isinstance(width, (int, float))):
raise TypeError("Length and width must be numbers")
if length <= 0 or width <= 0:
raise ValueError("Length and width must be positive numbers")
# Calculate area
area = length * width
return area
# Test with valid inputs
try:
print(calculate_rectangle_area(5, 3)) # Output: 15
# Test with invalid inputs
print(calculate_rectangle_area(-5, 3)) # Raises ValueError
except Exception as e:
print(f"Error: {e}")
This function now checks if the inputs are numbers and if they are positive, raising appropriate exceptions if these conditions aren't met.
Visualizing Rectangles with Programming
Programming isn't just about calculations; it can also help visualize concepts. Here's a simple Python program that uses the turtle module to draw a rectangle and display its area:
import turtle
def draw_rectangle_and_show_area(length, width):
# Set up the turtle
t = turtle.Turtle()
t.speed(1) # Slow speed to see the drawing
# Draw the rectangle
for _ in range(2):
t.forward(length * 50) # Scale for visibility
t.left(90)
t.forward(width * 50)
t.left(90)
# Calculate and display area
area = length * width
t.penup()
t.goto(length * 25, width * 25) # Move to center of rectangle
t.write(f"Area = {area} square units", align="center", font=("Arial", 12, "normal"))
# Keep the window open
turtle.done()
# Example usage
draw_rectangle_and_show_area(5, 3)
This program creates a visual representation of the rectangle and displays its area, making the concept more tangible for learners.
Interactive Rectangle Area Calculator
To make learning more engaging, we can create an interactive calculator. Here's a simple web based version using HTML, CSS, and JavaScript:
<!DOCTYPE html>
<html>
<head>
<title>Rectangle Area Calculator</title>
<style>
body {
font-family: Arial, sans-serif;
max-width: 600px;
margin: 0 auto;
padding: 20px;
}
.calculator {
border: 1px solid #ccc;
padding: 20px;
border-radius: 5px;
}
.form-group {
margin-bottom: 15px;
}
label {
display: block;
margin-bottom: 5px;
}
input {
width: 100%;
padding: 8px;
box-sizing: border-box;
}
button {
background-color: #4CAF50;
color: white;
padding: 10px 15px;
border: none;
border-radius: 4px;
cursor: pointer;
}
.result {
margin-top: 20px;
font-weight: bold;
}
#rectangle {
margin-top: 20px;
border: 2px solid black;
position: relative;
}
</style>
</head>
<body>
<h1>Rectangle Area Calculator</h1>
<div class="calculator">
<div class="form-group">
<label for="length">Length (units):</label>
<input type="number" id="length" min="1" value="5">
</div>
<div class="form-group">
<label for="width">Width (units):</label>
<input type="number" id="width" min="1" value="3">
</div>
<button onclick="calculateArea()">Calculate Area</button>
<div class="result" id="result">
Area: 15 square units
</div>
<div id="rectangle" style="width: 250px; height: 150px;">
<div style="position: absolute; top: 50%; left: 50%; transform: translate(-50%, -50%);">
Area: 15 sq units
</div>
</div>
</div>
<script>
// Initial calculation
calculateArea();
function calculateArea() {
// Get input values
const length = parseFloat(document.getElementById('length').value);
const width = parseFloat(document.getElementById('width').value);
// Validate inputs
if (isNaN(length) || isNaN(width) || length <= 0 || width <= 0) {
document.getElementById('result').textContent = 'Please enter positive numbers for length and width.';
return;
}
// Calculate area
const area = length * width;
// Display result
document.getElementById('result').textContent = `Area: ${area} square units`;
// Update visual representation
const maxWidth = 400;
const maxHeight = 300;
const scaleFactor = Math.min(maxWidth / length, maxHeight / width);
const rectangle = document.getElementById('rectangle');
rectangle.style.width = `${length * scaleFactor}px`;
rectangle.style.height = `${width * scaleFactor}px`;
rectangle.innerHTML = `<div style="position: absolute; top: 50%; left: 50%; transform: translate(-50%, -50%);">Area: ${area} sq units</div>`;
}
</script>
</body>
</html>
This interactive calculator allows users to input the length and width of a rectangle and see both the calculated area and a visual representation that scales according to the input dimensions.
Rectangle Area in Real World Programming Applications
Understanding how to calculate the area of a rectangle isn't just an academic exercise; it has practical applications in programming:
1. Graphics Programming
When developing games or graphical applications, you often need to calculate areas for collision detection, determining if one object is inside another, or allocating screen space.
2. UI/UX Design
In web and application development, understanding areas helps with layout calculations, determining how much content can fit in a given space, and responsive design.
3. Image Processing
Many image processing algorithms require area calculations for operations like cropping, scaling, or determining regions of interest.
4. Data Visualization
When creating charts and graphs, area calculations are essential for proper sizing and proportional representation of data.
Advanced Rectangle Area Concepts in Programming
Finding the Area of Overlapping Rectangles
A more advanced problem is calculating the area of the intersection of two rectangles. Here's a Python solution:
def calculate_overlap_area(rect1, rect2):
"""
Calculate the area of overlap between two rectangles.
Each rectangle is represented as [x1, y1, x2, y2] where
(x1, y1) is the bottom left corner and (x2, y2) is the top right corner.
"""
# Find the coordinates of the intersection
x_overlap = max(0, min(rect1[2], rect2[2]) - max(rect1[0], rect2[0]))
y_overlap = max(0, min(rect1[3], rect2[3]) - max(rect1[1], rect2[1]))
# Calculate overlap area
return x_overlap * y_overlap
# Example
rect1 = [0, 0, 5, 3] # A 5x3 rectangle with bottom left at origin
rect2 = [3, 1, 7, 4] # A 4x3 rectangle that partially overlaps with rect1
overlap_area = calculate_overlap_area(rect1, rect2)
print(f"The area of overlap is {overlap_area} square units")
Working with Rotated Rectangles
Calculating the area of a rotated rectangle is more complex but still follows the same basic principle. The area doesn't change when a rectangle is rotated.
Teaching Rectangle Area in Coding Education
At AlgoCademy, we believe in teaching programming concepts through practical examples that build on fundamental knowledge. The rectangle area problem serves as an excellent starting point because:
- It introduces basic arithmetic operations in code
- It demonstrates function creation and parameter passing
- It can be expanded to teach object oriented programming concepts
- It provides opportunities to discuss input validation and error handling
- It can be visualized, making abstract concepts more concrete
- It has real world applications that students can relate to
Conclusion
Finding the area of a rectangle may seem like a simple mathematical operation, but as we've seen, it provides a rich context for learning programming concepts at various levels. From basic calculations to object oriented design, input validation, visualization, and real world applications, this fundamental geometric problem offers numerous opportunities for growth in coding skills.
Whether you're just starting your programming journey or looking to reinforce your understanding of coding principles, implementing rectangle area calculations in different ways can be a valuable exercise. As you progress in your coding education, you'll find that these basic concepts form the foundation for more complex algorithms and applications.
Remember, at AlgoCademy, we emphasize not just knowing how to code, but understanding the principles behind the code. By mastering simple concepts like calculating the area of a rectangle, you're building the algorithmic thinking skills that will serve you well in technical interviews and real world programming challenges.
Ready to continue your coding journey? Explore our interactive tutorials and coding challenges to further develop your skills and prepare for technical interviews at top tech companies.