How to Explain Your Thought Process Effectively in Coding Interviews
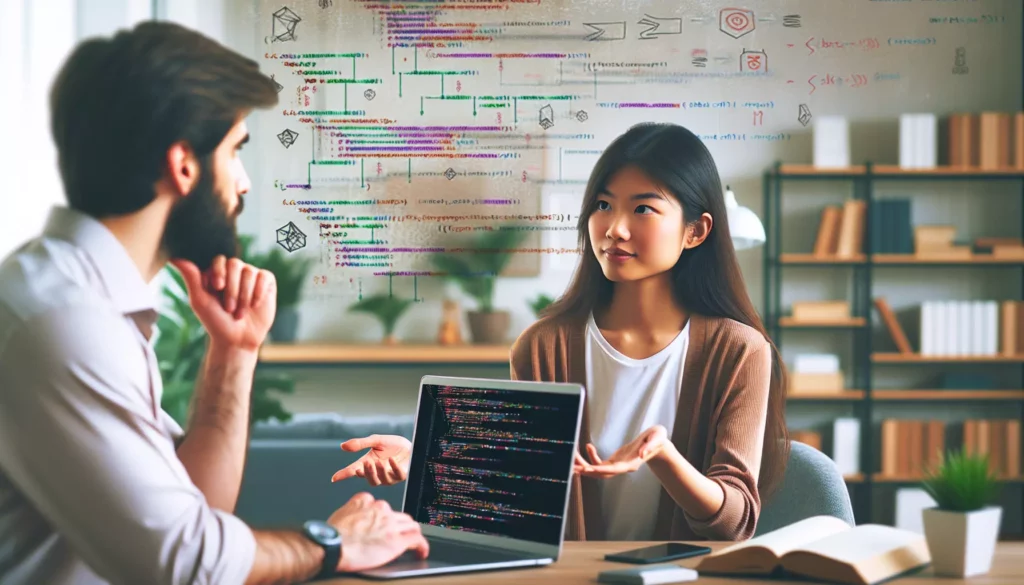
Coding interviews can be nerve-wracking experiences, especially when you’re aiming for positions at top tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google). While your coding skills are crucial, equally important is your ability to articulate your thought process clearly and effectively. This skill not only demonstrates your problem-solving abilities but also showcases your communication prowess – a vital trait in any collaborative development environment.
In this comprehensive guide, we’ll explore strategies to help you explain your thought process during coding interviews, enhancing your chances of success and leaving a lasting impression on your interviewers.
1. Understanding the Importance of Explaining Your Thought Process
Before diving into the strategies, it’s crucial to understand why explaining your thought process is so important in coding interviews:
- Demonstrates problem-solving skills: It shows how you approach and break down complex problems.
- Reveals communication abilities: Clear explanation reflects your ability to convey technical concepts effectively.
- Provides insight into your coding style: It gives interviewers a glimpse of how you would work on real projects.
- Allows interviewers to guide you: By understanding your thinking, interviewers can provide hints if you’re stuck.
- Shows your ability to work in a team: Clear communication is essential for collaborative coding environments.
2. Preparing for Effective Explanation
Preparation is key to articulating your thoughts clearly during an interview. Here are some steps to help you prepare:
2.1. Practice Problem-Solving Aloud
Get comfortable with verbalizing your thoughts by practicing coding problems aloud. This can be done alone or with a study partner. As you solve problems, explain each step of your process, from understanding the problem to implementing the solution.
2.2. Familiarize Yourself with Common Problem-Solving Techniques
Understanding and being able to explain common problem-solving techniques will make your thought process more structured and coherent. Some key techniques include:
- Divide and Conquer
- Dynamic Programming
- Greedy Algorithms
- Backtracking
- Depth-First Search (DFS) and Breadth-First Search (BFS)
2.3. Review Data Structures and Algorithms
Ensure you have a solid grasp of fundamental data structures and algorithms. Being able to explain why you chose a particular data structure or algorithm for a problem is crucial.
2.4. Prepare a Mental Checklist
Develop a mental checklist to guide your problem-solving process. This could include steps like:
- Understand the problem
- Identify the inputs and outputs
- Consider edge cases
- Develop a high-level approach
- Choose appropriate data structures and algorithms
- Implement the solution
- Test and optimize
3. Strategies for Explaining Your Thought Process During the Interview
Now that you’re prepared, let’s explore strategies to effectively explain your thought process during the actual interview:
3.1. Start with a High-Level Overview
Begin by providing a brief, high-level overview of your approach. This gives the interviewer a roadmap of your thinking and allows them to follow along more easily. For example:
"To solve this problem, I'm thinking of using a hash map to store the frequency of elements, then iterating through the array to find the first non-repeating element. This approach should give us a time complexity of O(n) and space complexity of O(n)."
3.2. Think Aloud
As you work through the problem, verbalize your thoughts. This includes explaining why you’re considering certain approaches, any trade-offs you’re weighing, and how you’re arriving at decisions. For instance:
"I'm considering using a stack for this problem because we need to process elements in a last-in-first-out order. However, I'm also thinking about the time complexity. Let me explore if there's a more efficient approach..."
3.3. Explain Your Code as You Write It
As you begin coding, explain each part of your code. This helps the interviewer understand your implementation and coding style. For example:
"I'm going to start by initializing a hash map to store the character frequencies. Then, I'll iterate through the string to populate this map."
# Initialize the hash map
char_freq = {}
# Populate the hash map
for char in string:
char_freq[char] = char_freq.get(char, 0) + 1
"Now that we have the character frequencies, we'll iterate through the string again to find the first character with a frequency of 1."
# Find the first non-repeating character
for char in string:
if char_freq[char] == 1:
return char
return None # If no non-repeating character is found
3.4. Discuss Time and Space Complexity
Always discuss the time and space complexity of your solution. This shows that you’re thinking about efficiency and scalability. Explain how you arrived at your complexity analysis:
"The time complexity of this solution is O(n), where n is the length of the string. We iterate through the string twice: once to build the hash map and once to find the first non-repeating character. The space complexity is O(k), where k is the size of the character set. In the worst case, where all characters are unique, k would be equal to n."
3.5. Consider and Discuss Edge Cases
Show that you’re thinking about potential edge cases or special scenarios. This demonstrates thoroughness and attention to detail:
"We should consider a few edge cases:
1. What if the string is empty? We should return None.
2. What if there are no non-repeating characters? We should also return None.
3. What if the string contains characters outside the ASCII range? We might need to adjust our approach depending on the expected input."
3.6. Explain Your Testing Strategy
Discuss how you would test your solution. This shows that you value code quality and reliability:
"To test this solution, I would start with some basic test cases:
1. A string with a clear first non-repeating character: 'leetcode' should return 'l'
2. A string with no non-repeating characters: 'aabbcc' should return None
3. An empty string: '' should return None
4. A string with all unique characters: 'abcdef' should return 'a'
I would also test with larger strings to ensure the solution scales well."
3.7. Discuss Potential Optimizations
If time allows, discuss potential optimizations or alternative approaches. This demonstrates your ability to think critically about your own solutions:
"While this solution is efficient, we could potentially optimize it further by using a single pass through the string. We could use a linked hash map to maintain the order of characters while storing their frequencies. This would allow us to find the first non-repeating character in a single pass, potentially improving performance for very large strings."
4. Common Pitfalls to Avoid
When explaining your thought process, be aware of these common pitfalls:
4.1. Silence
Avoid long periods of silence. If you need time to think, communicate this to the interviewer:
"I'm taking a moment to consider the best approach for this problem. I'm weighing the trade-offs between using a hash map versus a more memory-efficient solution."
4.2. Rushing
Don’t rush to start coding immediately. Take time to understand the problem and explain your approach:
"Before I start coding, I'd like to make sure I understand the problem correctly and outline my approach. Is it okay if I take a minute to do this?"
4.3. Ignoring Interviewer Hints
Pay attention to hints or questions from the interviewer. They often provide valuable guidance:
"Thank you for that hint. You're right, I hadn't considered using a two-pointer approach. Let me think about how we could apply that to this problem..."
4.4. Over-Explaining
While explanation is important, avoid getting bogged down in unnecessary details. Stay focused on the key points of your approach:
"To keep things concise, I'll focus on the main steps of my approach. Please let me know if you'd like me to elaborate on any specific part."
5. Handling Difficult Situations
Even with thorough preparation, you may encounter challenging situations. Here’s how to handle them:
5.1. When You’re Stuck
If you find yourself stuck, don’t panic. Explain your thought process and ask for a hint if needed:
"I'm considering a few approaches, but I'm not sure which would be most efficient. I've thought about using a stack or a queue, but I'm wondering if there's a more optimal solution. Could you provide a hint about what data structure might be most suitable here?"
5.2. When You Make a Mistake
If you realize you’ve made a mistake, acknowledge it and explain how you’d correct it:
"I just realized that my current approach doesn't account for negative numbers. Let me step back and adjust my solution to handle this case."
5.3. When You Need to Change Your Approach
If you need to change your approach mid-way, explain why:
"As I'm working through this, I've realized that a dynamic programming approach would be more efficient than my initial recursive solution. Would it be okay if I switched gears and explained a DP approach instead?"
6. Practice Makes Perfect
Improving your ability to explain your thought process is a skill that requires practice. Here are some ways to hone this skill:
6.1. Mock Interviews
Participate in mock interviews with peers or mentors. This allows you to practice in a realistic setting and receive feedback on your communication.
6.2. Coding Platforms
Use coding platforms like LeetCode, HackerRank, or AlgoCademy to practice solving problems. As you solve each problem, practice explaining your approach out loud.
6.3. Record Yourself
Record yourself solving problems and explaining your thought process. Review the recordings to identify areas for improvement in your communication.
6.4. Teach Others
Teaching coding concepts to others is an excellent way to improve your ability to explain complex ideas clearly.
7. Leveraging AlgoCademy for Interview Preparation
AlgoCademy is an excellent resource for preparing for coding interviews, particularly when it comes to improving your ability to explain your thought process:
7.1. Interactive Tutorials
AlgoCademy’s interactive tutorials guide you through problem-solving steps, helping you internalize a structured approach to tackling coding challenges.
7.2. AI-Powered Assistance
The platform’s AI-powered assistance can provide feedback on your solutions, helping you understand different approaches and how to explain them effectively.
7.3. Problem-Solving Framework
AlgoCademy emphasizes a problem-solving framework that aligns well with the expectations of top tech companies. Practicing with this framework helps you develop a clear and logical thought process.
7.4. FAANG-Focused Content
With content specifically tailored for FAANG interviews, AlgoCademy helps you practice explaining your thought process in the context of the types of problems you’re likely to encounter in these high-stakes interviews.
Conclusion
Explaining your thought process effectively in coding interviews is a crucial skill that can set you apart from other candidates. It demonstrates not only your technical prowess but also your ability to communicate complex ideas clearly – a valuable asset in any development team.
By following the strategies outlined in this guide and consistently practicing your explanation skills, you’ll be well-prepared to articulate your problem-solving approach in your next coding interview. Remember, the goal is not just to solve the problem, but to demonstrate your reasoning, adaptability, and communication skills throughout the process.
Leverage resources like AlgoCademy to refine your skills, and approach each interview as an opportunity to showcase your unique problem-solving style. With preparation and practice, you’ll be well-equipped to excel in coding interviews and take the next step in your programming career.