How to Explain Your Solution in a Coding Challenge Interview
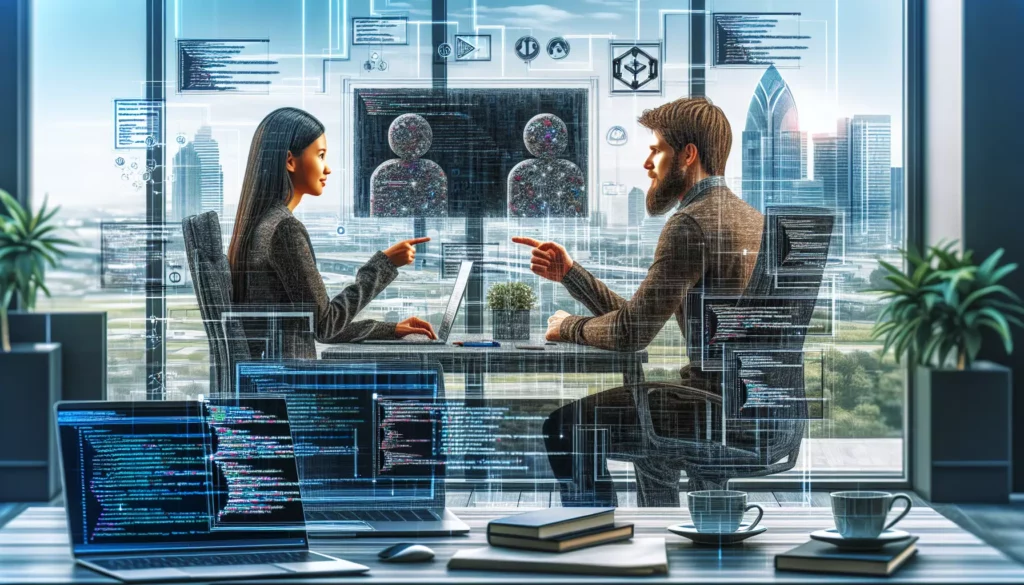
When it comes to technical interviews, particularly for positions at top tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), your ability to solve coding challenges is crucial. However, equally important is your capacity to effectively communicate and explain your solution. This skill can make the difference between a good interview and a great one. In this comprehensive guide, we’ll explore the best practices for articulating your thought process and solution during a coding challenge interview.
1. Understanding the Importance of Explanation
Before diving into the how-to, it’s essential to understand why explaining your solution is so critical:
- Demonstrates your thought process: Interviewers want to see how you approach problems, not just the final result.
- Shows communication skills: In a real work environment, you’ll need to explain your code to colleagues.
- Reveals your depth of understanding: A clear explanation shows you truly grasp the concepts, not just memorized solutions.
- Allows for collaboration: It opens up dialogue with the interviewer, potentially leading to improvements or alternative approaches.
2. Before You Start Coding
The explanation process begins even before you write a single line of code:
2.1. Clarify the Problem
Start by restating the problem to ensure you’ve understood it correctly. Ask questions to clarify any ambiguities. This shows attention to detail and prevents misunderstandings.
Interviewer: "Write a function to find the longest palindromic substring in a given string."
You: "Just to clarify, we're looking for the longest substring that reads the same forwards and backwards, correct? And if there are multiple palindromes of the same length, should I return all of them or just one?"
2.2. Discuss Input and Output
Clearly state what inputs the function will receive and what output it should produce. This sets clear expectations and demonstrates your systematic approach.
You: "So, the function will take a string as input, and it should return a string - the longest palindromic substring. If there are multiple palindromes of the same maximum length, returning any one of them would be acceptable."
2.3. Consider Edge Cases
Think about potential edge cases and discuss them with the interviewer. This shows foresight and thoroughness.
You: "I'm considering a few edge cases:
1. An empty string input
2. A string with no palindromes longer than one character
3. A string that is entirely a palindrome
Would you like me to handle these specifically?"
3. Explaining Your Approach
Once you’ve clarified the problem, it’s time to explain your approach:
3.1. Outline Your Strategy
Before coding, provide a high-level overview of your planned approach. This gives the interviewer insight into your problem-solving strategy.
You: "I'm thinking of using a dynamic programming approach for this problem. We can build a 2D table where cell [i][j] represents whether the substring from index i to j is a palindrome. We'll fill this table bottom-up, and keep track of the longest palindrome we've found."
3.2. Discuss Time and Space Complexity
Analyze the time and space complexity of your proposed solution. This demonstrates your understanding of algorithmic efficiency.
You: "This approach would have a time complexity of O(n^2) where n is the length of the string, as we're filling an n x n table. The space complexity would also be O(n^2) due to the 2D table we're using."
3.3. Consider Alternatives
If you know multiple ways to solve the problem, briefly mention them and explain why you chose your particular approach.
You: "An alternative approach could be to use the center expansion method, which would have the same time complexity but O(1) space complexity. However, I chose the DP approach as it's more straightforward to implement and easier to extend for related problems."
4. During the Coding Process
As you write your code, continue to explain your thought process:
4.1. Think Aloud
Verbalize your thoughts as you code. This gives the interviewer insight into your problem-solving process and allows them to provide hints if needed.
You: "I'll start by initializing our DP table. All substrings of length 1 are palindromes, so I'll set the diagonal to True..."
4.2. Explain Each Step
As you implement each part of your solution, explain what it does and why it’s necessary.
You: "Now I'm implementing the main loop. We'll iterate over different lengths, from 2 to the length of the string. For each length, we'll check all possible substrings of that length..."
4.3. Use Clear Variable Names
Use descriptive variable names that make your code self-explanatory. This makes your code easier to understand and demonstrates good coding practices.
// Instead of:
int s, e;
// Use:
int start_index, end_index;
5. After Completing the Code
Once you’ve finished writing your solution, there are still important steps to take:
5.1. Walk Through an Example
Use a simple example to walk through your code, explaining how it works step-by-step. This demonstrates that you understand your own solution and can explain it clearly.
You: "Let's walk through this with the string 'babad'. We start by initializing our DP table..."
5.2. Discuss Test Cases
Propose a few test cases, including edge cases, and explain how your code would handle them.
You: "I'd like to test this with a few cases:
1. 'babad' - should return 'bab' or 'aba'
2. 'cbbd' - should return 'bb'
3. 'a' - should return 'a'
4. '' (empty string) - should return ''
5. 'abcba' - should return 'abcba'"
5.3. Analyze the Actual Complexity
Confirm that the time and space complexity of your implemented solution matches your initial analysis. If there are any differences, explain why.
You: "As we discussed earlier, the time complexity is O(n^2) due to the nested loops, and the space complexity is also O(n^2) because of our DP table. This matches our initial analysis."
6. Handling Follow-up Questions
Interviewers often ask follow-up questions to probe your understanding further:
6.1. Be Open to Optimization
If the interviewer asks about potential optimizations, be open to discussing them. This shows your ability to iterate and improve on solutions.
Interviewer: "Can you think of a way to optimize the space complexity?"
You: "Yes, we could potentially reduce the space complexity to O(n) by only storing two rows of the DP table at a time, since we only need the previous row to calculate the current one. This would slightly complicate the code but significantly reduce memory usage."
6.2. Discuss Trade-offs
When discussing alternative approaches or optimizations, be prepared to talk about the trade-offs involved.
You: "While the center expansion method would reduce our space complexity to O(1), it would make the code more complex and potentially harder to maintain. It's a trade-off between space efficiency and code simplicity."
6.3. Connect to Real-World Scenarios
If possible, relate the problem or your solution to real-world scenarios. This demonstrates your ability to see the bigger picture.
You: "This type of problem could be relevant in text processing applications, like finding the longest repeating sequence in DNA strands, or in certain types of data compression algorithms."
7. Common Pitfalls to Avoid
When explaining your solution, be aware of these common mistakes:
7.1. Overcomplicating the Explanation
While it’s important to be thorough, avoid getting bogged down in unnecessary details. Keep your explanation clear and concise.
7.2. Being Defensive
If the interviewer points out an issue or suggests an improvement, don’t become defensive. Instead, show your ability to accept feedback and iterate on your solution.
7.3. Assuming Too Much Knowledge
Don’t assume the interviewer is familiar with every concept or algorithm you’re using. Be prepared to explain fundamental concepts if asked.
7.4. Rushing Through the Explanation
Take your time to explain clearly. It’s better to be thorough and correct than fast and unclear.
8. Practice Makes Perfect
Explaining your solution effectively is a skill that improves with practice. Here are some ways to hone this skill:
8.1. Mock Interviews
Conduct mock interviews with friends or use online platforms that offer this service. This will help you get comfortable explaining your solutions under pressure.
8.2. Teach Others
Try explaining coding concepts or solutions to others, even if they’re not programmers. This will force you to break down complex ideas into simpler terms.
8.3. Record Yourself
Record yourself solving and explaining coding problems. This allows you to review your performance and identify areas for improvement.
8.4. Participate in Coding Communities
Engage in online coding communities where you can discuss solutions with others. This exposure to different perspectives will enhance your ability to explain and discuss code.
9. Tailoring Your Explanation to the Company
Different companies may have different expectations when it comes to explaining solutions:
9.1. Research the Company’s Values
Some companies prioritize optimal solutions, while others may value clean, maintainable code more. Tailor your explanation accordingly.
9.2. Understand the Role
If you’re interviewing for a specific team or role, try to relate your explanation to that context. For example, if it’s a backend role, you might focus more on scalability and efficiency.
9.3. Use Relevant Terminology
If you know the company uses specific technologies or methodologies, incorporate relevant terminology into your explanation where appropriate.
10. Conclusion
Explaining your solution in a coding challenge interview is not just about showcasing your coding skills—it’s an opportunity to demonstrate your problem-solving approach, communication abilities, and depth of understanding. By following these guidelines and practicing regularly, you can significantly improve your performance in technical interviews.
Remember, the goal is not just to solve the problem, but to show how you think, how you approach challenges, and how you can contribute to a team. Clear, confident, and thoughtful explanations of your solutions will set you apart and increase your chances of success in even the most challenging coding interviews.
As you continue to develop your skills with platforms like AlgoCademy, focus not just on solving problems, but on articulating your solutions clearly and effectively. This holistic approach to coding education will serve you well in your journey towards landing your dream job in the tech industry.