How to Explain Your Problem-Solving Process During an Interview
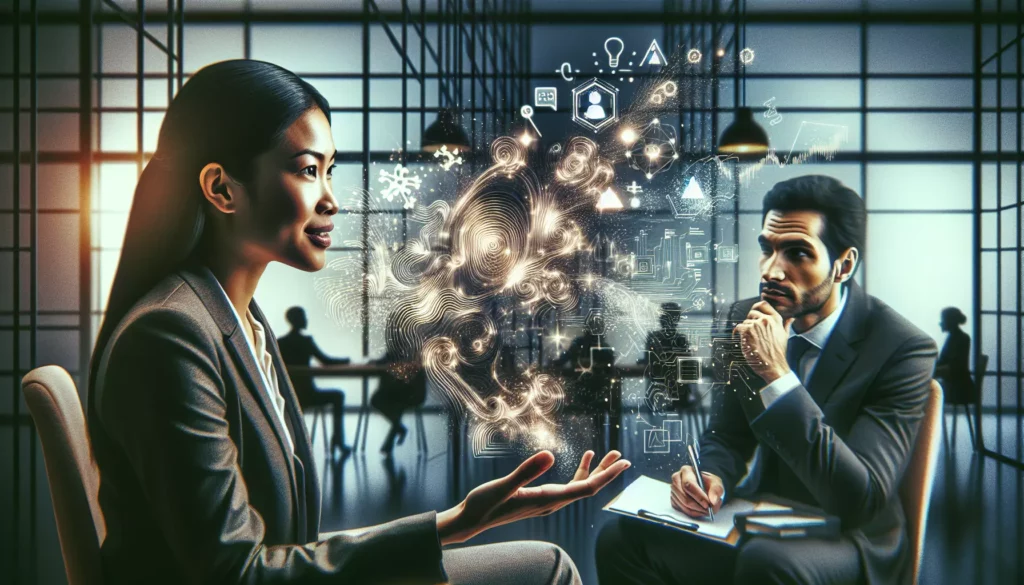
In the competitive world of tech interviews, particularly for positions at major companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), your ability to solve problems is just as important as your coding skills. However, what many candidates overlook is the crucial skill of effectively communicating their problem-solving process. This article will guide you through the art of explaining your problem-solving approach during a technical interview, helping you stand out and demonstrate your value as a potential team member.
Why Explaining Your Problem-Solving Process Matters
Before diving into the how-to, it’s essential to understand why articulating your problem-solving process is so important:
- Demonstrates Thought Process: It shows interviewers how you think and approach challenges.
- Reveals Communication Skills: Clear explanation reflects your ability to convey complex ideas to team members.
- Highlights Analytical Abilities: It showcases your capacity to break down and analyze problems systematically.
- Shows Adaptability: Your process can indicate how you might handle unfamiliar problems in the future.
- Provides Insight into Work Style: It gives interviewers a glimpse of how you might collaborate and contribute to the team.
The STAR Method: A Framework for Explanation
One effective way to structure your explanation is by using the STAR method. This acronym stands for Situation, Task, Action, and Result. Here’s how you can apply it to explain your problem-solving process:
1. Situation
Start by briefly describing the context of the problem. This could include:
- The type of project or application you were working on
- Any constraints or limitations you were facing
- The broader goals or objectives that the problem was related to
For example: “I was working on a web application that needed to handle a large volume of concurrent user requests while maintaining fast response times.”
2. Task
Clearly state the specific problem or challenge you needed to solve. Be concise but provide enough detail for the interviewer to understand the complexity. For instance:
“The task was to optimize the database queries to reduce load times from an average of 5 seconds to under 1 second, even during peak usage periods.”
3. Action
This is where you dive into your problem-solving process. Explain the steps you took to address the challenge. Be sure to:
- Break down your approach into clear, logical steps
- Highlight any tools, technologies, or methodologies you used
- Mention any collaboration with team members or stakeholders
- Discuss any alternatives you considered and why you chose your specific approach
For example:
- “First, I analyzed the existing database structure and identified inefficient queries.”
- “Then, I used database profiling tools to pinpoint the most resource-intensive operations.”
- “I redesigned the database schema to improve indexing and reduce redundant data.”
- “Next, I optimized the SQL queries, implementing caching mechanisms where appropriate.”
- “Finally, I conducted load testing to ensure the changes could handle the expected user volume.”
4. Result
Conclude by sharing the outcomes of your problem-solving efforts. Include:
- Quantifiable improvements or metrics
- Any positive feedback or recognition you received
- Lessons learned or insights gained from the experience
For instance: “As a result of these optimizations, we reduced the average load time to 0.8 seconds, even during peak hours. This led to a 15% increase in user engagement and positive feedback from both the product team and end-users.”
Tips for Effective Communication During the Interview
Now that you have a framework for explaining your problem-solving process, here are some additional tips to enhance your communication during the interview:
1. Think Aloud
Interviewers are interested in your thought process, not just the final solution. Practice verbalizing your thoughts as you work through problems. This might feel unnatural at first, but it’s a valuable skill in technical interviews. For example:
“I’m considering using a hash table here because it would give us O(1) lookup time, which is crucial for the performance requirements of this problem. However, I need to consider the space complexity trade-off…”
2. Use Visual Aids
If you’re in a whiteboard interview or using a shared document, don’t hesitate to draw diagrams or write pseudo-code to illustrate your ideas. Visual representations can make your explanations clearer and demonstrate your ability to communicate complex concepts. For instance:
// Pseudo-code for a binary search
function binarySearch(arr, target):
left = 0
right = arr.length - 1
while left <= right:
mid = (left + right) / 2
if arr[mid] == target:
return mid
else if arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1 // Target not found
3. Explain Your Reasoning
Don’t just state what you’re doing; explain why you’re making certain decisions. This shows your critical thinking skills and depth of understanding. For example:
“I’m choosing to use a min-heap for this problem because we need to efficiently keep track of the smallest element, and a min-heap provides O(log n) insertion and O(1) access to the minimum element, which aligns with our performance requirements.”
4. Discuss Trade-offs
Real-world problem-solving often involves balancing different factors. Show that you understand this by discussing the pros and cons of different approaches. For instance:
“While a recursive solution would be more elegant and potentially easier to read, I’m opting for an iterative approach in this case. The reason is that for very large inputs, a recursive solution might lead to stack overflow issues, whereas an iterative solution would be more memory-efficient and robust.”
5. Be Open to Feedback
If the interviewer provides hints or suggestions, show that you can incorporate them into your thinking. This demonstrates adaptability and teamwork. You might say:
“Thank you for that suggestion. I see how using a two-pointer technique could simplify the solution. Let me incorporate that and walk you through my updated approach.”
6. Practice Active Listening
Make sure you fully understand the problem before diving into a solution. Ask clarifying questions and restate the problem to confirm your understanding. This shows attention to detail and prevents misunderstandings. For example:
“Just to confirm, we’re looking for an in-place solution that optimizes for space complexity, even if it means a slight trade-off in time complexity. Is that correct?”
Common Pitfalls to Avoid
While explaining your problem-solving process, be aware of these common mistakes:
1. Jumping to Code Too Quickly
Resist the urge to start coding immediately. Take time to think through the problem and discuss your approach first. This shows that you value planning and don’t rush into implementation without consideration.
2. Being Too Vague
Avoid general statements like “I would optimize the algorithm.” Instead, be specific about what optimizations you would make and why. For instance:
“To optimize this algorithm, I would replace the nested loops with a hash table lookup, reducing the time complexity from O(n^2) to O(n) at the cost of O(n) additional space.”
3. Ignoring Constraints
Always consider and explicitly address any constraints mentioned in the problem statement, such as time/space complexity requirements or input limitations. This demonstrates attention to detail and practical problem-solving skills.
4. Overcomplicating the Solution
While it’s important to show your knowledge, avoid using overly complex solutions when a simpler one would suffice. If you do choose a more complex approach, be prepared to justify why it’s necessary or beneficial.
5. Not Admitting When You’re Stuck
If you encounter a roadblock, it’s better to admit it and discuss your thoughts than to pretend you know the answer. You might say:
“I’m not entirely sure how to proceed at this point. Here are a couple of ideas I’m considering… What are your thoughts on these approaches?”
Practicing Your Explanation Skills
Like any skill, effectively explaining your problem-solving process improves with practice. Here are some ways to hone this ability:
1. Mock Interviews
Engage in mock interviews with peers, mentors, or through online platforms. This allows you to practice articulating your thoughts in a realistic setting and receive feedback.
2. Solve Problems Aloud
When practicing coding problems, make a habit of explaining your thought process out loud, even when you’re alone. This helps make verbalization feel more natural during actual interviews.
3. Record Yourself
Try recording video or audio of yourself solving and explaining problems. Reviewing these recordings can help you identify areas for improvement in your communication style.
4. Teach Others
Explaining concepts and problem-solving approaches to others, whether through tutoring, writing blog posts, or creating video tutorials, can significantly improve your ability to communicate technical ideas clearly.
5. Participate in Code Reviews
If possible, engage in code reviews at work or in open-source projects. This provides practice in both explaining your own code and understanding others’ approaches.
Conclusion
Mastering the art of explaining your problem-solving process is a valuable skill that can set you apart in technical interviews, especially for competitive positions at top tech companies. By using structured approaches like the STAR method, incorporating effective communication techniques, and avoiding common pitfalls, you can showcase not just your coding abilities, but also your thought process, analytical skills, and potential as a team member.
Remember, the goal is not just to solve the problem, but to demonstrate how you approach challenges, think critically, and communicate complex ideas. With practice and mindful application of these strategies, you’ll be well-equipped to impress interviewers and highlight your problem-solving prowess.
As you continue to develop your skills, platforms like AlgoCademy can be invaluable resources. They offer interactive coding tutorials, AI-powered assistance, and step-by-step guidance that can help you not only improve your coding skills but also practice articulating your problem-solving approach. By combining technical proficiency with strong communication skills, you’ll be well-positioned to excel in even the most challenging technical interviews.