How to Explain Complex Concepts Simply: A Comprehensive Guide
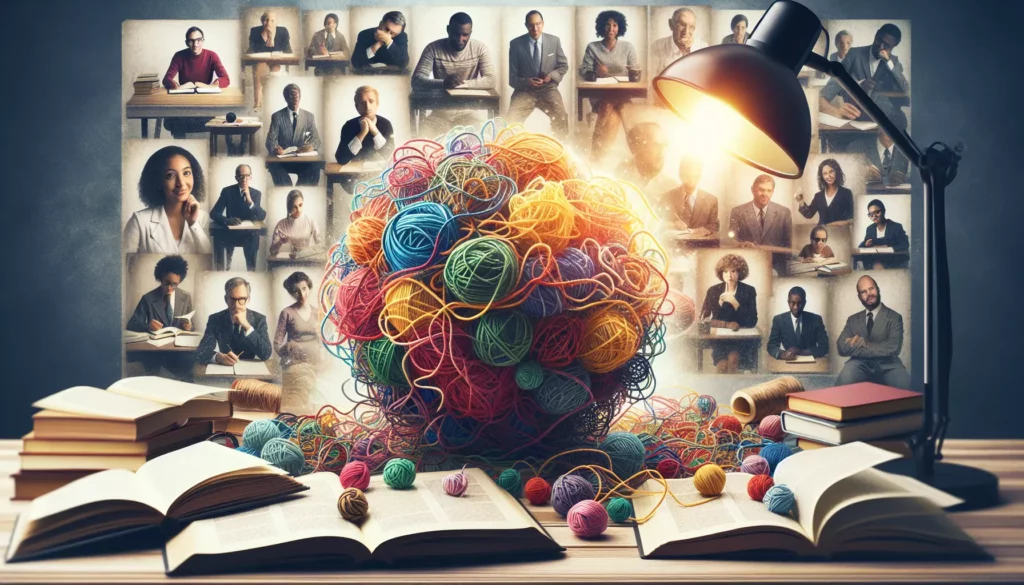
In today’s fast-paced world of technology and innovation, the ability to explain complex concepts in simple terms is an invaluable skill. Whether you’re a teacher, programmer, or business professional, being able to break down intricate ideas into digestible chunks can make a world of difference in communication and understanding. This guide will walk you through the art of simplifying complex concepts, using examples from the world of coding education and programming skills development.
1. Understand Your Audience
Before diving into explanations, it’s crucial to know who you’re talking to. Are they complete beginners or do they have some background knowledge? For instance, when explaining coding concepts on a platform like AlgoCademy, you’d approach things differently for a novice coder versus someone preparing for a technical interview at a major tech company.
Tips for Understanding Your Audience:
- Assess their current knowledge level
- Identify their goals and motivations
- Consider their learning style (visual, auditory, kinesthetic)
- Determine the context in which they’ll apply the knowledge
2. Start with the Big Picture
When tackling a complex topic, it’s often helpful to start with an overview before diving into the details. This gives your audience a framework to hang more specific information on later.
Example: Explaining Algorithms
Instead of jumping straight into the intricacies of a specific algorithm, you might start with:
“An algorithm is like a recipe for solving a problem. Just as a recipe tells you the steps to make a dish, an algorithm outlines the steps a computer needs to follow to complete a task.”
3. Use Analogies and Metaphors
Relating complex concepts to familiar ideas can make them much more accessible. Good analogies create a bridge between the known and the unknown.
Example: Explaining Variables in Programming
“Think of variables like labeled boxes. Just as you can put different items in a box and label it for easy retrieval, variables in programming store different types of data that you can access using their names.”
4. Break It Down into Smaller Parts
Complex concepts often consist of several simpler ideas. By breaking these down and explaining each part separately, you can make the overall concept more manageable.
Example: Explaining Object-Oriented Programming (OOP)
Instead of trying to explain OOP all at once, break it down into its core principles:
- Encapsulation: Bundling data and methods that work on that data within one unit
- Inheritance: Creating new classes based on existing ones
- Polymorphism: The ability of objects to take on multiple forms
- Abstraction: Hiding complex implementation details and showing only the essential features of an object
5. Use Visual Aids
Many people are visual learners, and even for those who aren’t, diagrams, flowcharts, and other visual representations can help clarify complex ideas.
Example: Explaining Sorting Algorithms
When explaining sorting algorithms like bubble sort or quicksort, using animated visualizations can make the process much clearer than words alone. Platforms like AlgoCademy often incorporate such visual aids in their interactive tutorials.
6. Provide Real-World Examples
Connecting abstract concepts to real-world applications helps make them more relatable and easier to understand.
Example: Explaining Big O Notation
When discussing time complexity in algorithms:
“Big O notation is like estimating how long it would take to find a book in libraries of different sizes. In a small library (O(1)), you might find it instantly. In a medium-sized library (O(log n)), it would take a bit longer. In a huge library (O(n)), you might need to check every book, which would take much longer.”
7. Use Interactive Elements
Engagement is key to understanding. Interactive elements can help learners grasp concepts by allowing them to experiment and see results in real-time.
Example: Teaching Coding Concepts
Platforms like AlgoCademy often provide coding environments where learners can write and run code snippets, immediately seeing the results of their work. This hands-on approach can be particularly effective for understanding programming concepts.
8. Explain the ‘Why’ Along with the ‘What’
Understanding the reasoning behind a concept can make it easier to grasp and remember. Always try to explain why something works the way it does, not just what it does.
Example: Explaining Version Control
When teaching about Git:
“Version control systems like Git are essential because they allow multiple people to work on the same project without overwriting each other’s changes. It’s like having a time machine for your code – you can always go back to a previous version if something goes wrong.”
9. Use Progressive Disclosure
Start with the basics and gradually introduce more complex ideas. This approach prevents overwhelming your audience and allows them to build on their understanding step by step.
Example: Teaching Data Structures
When explaining data structures, you might start with simple arrays, then move on to linked lists, then trees, and finally more complex structures like graphs. Each step builds on the previous one, making the learning process more manageable.
10. Encourage Questions and Provide Feedback
Creating an environment where learners feel comfortable asking questions can greatly enhance understanding. Similarly, providing timely and constructive feedback helps reinforce correct understanding and correct misconceptions.
Example: AI-Powered Assistance in Coding Education
Platforms like AlgoCademy often incorporate AI-powered assistants that can provide immediate feedback on code submissions, explain errors, and offer suggestions for improvement. This real-time interaction can significantly enhance the learning process.
11. Use Storytelling
Humans are naturally drawn to stories. Framing complex concepts within a narrative can make them more engaging and memorable.
Example: Explaining Recursion
You could tell a story about Russian nesting dolls to explain recursion:
“Imagine you have a set of Russian nesting dolls. To find the smallest doll, you open the largest doll to reveal a smaller one inside. You keep opening dolls until you can’t open any more – that’s your base case. This process of opening dolls (calling the function) until you reach the smallest one (the base case) is similar to how recursion works in programming.”
12. Relate to Prior Knowledge
Building on what your audience already knows can make new concepts feel less alien. Try to connect new ideas to familiar ones whenever possible.
Example: Explaining APIs
When introducing APIs (Application Programming Interfaces), you might relate them to familiar interfaces:
“An API is like the buttons on a vending machine. You don’t need to know how the machine works internally – you just press a button (make an API call) and get your snack (receive data or perform an action). The API provides a simple interface to interact with complex systems behind the scenes.”
13. Use Repetition and Reinforcement
Repeating key points in different ways can help reinforce understanding. This doesn’t mean simply saying the same thing over and over, but rather approaching the concept from different angles.
Example: Teaching Loops in Programming
When explaining loops, you might:
- Start with a simple definition
- Show a basic code example
- Relate it to a real-world repetitive task
- Demonstrate different types of loops (for, while, do-while)
- Have learners write their own loops to solve problems
Each step reinforces the concept in a different way.
14. Address Common Misconceptions
Every field has its share of common misunderstandings. By proactively addressing these, you can help your audience avoid pitfalls and develop a clearer understanding.
Example: Explaining the Difference Between ‘==’ and ‘===’ in JavaScript
A common source of confusion for new JavaScript programmers:
console.log(5 == "5"); // true
console.log(5 === "5"); // false
Explanation:
“The double equals (==) checks for value equality with coercion allowed, while the triple equals (===) checks for both value and type equality. In the first case, JavaScript converts the string ‘5’ to a number before comparison. In the second case, it compares both value and type, finding them different.”
15. Use Mnemonics and Memory Tricks
Mnemonics can be powerful tools for remembering complex information. Creating memorable phrases or acronyms can help learners recall important concepts.
Example: Remembering SOLID Principles in Object-Oriented Design
- Single Responsibility Principle
- Open-Closed Principle
- Liskov Substitution Principle
- Interface Segregation Principle
- Dependency Inversion Principle
The acronym “SOLID” itself serves as a mnemonic for these important principles.
16. Provide Practice Opportunities
Theory alone is often not enough to fully grasp complex concepts. Providing opportunities for practical application can significantly enhance understanding.
Example: Learning Data Structures and Algorithms
Platforms like AlgoCademy often provide coding challenges and problems that require the application of specific data structures or algorithms. This hands-on practice helps reinforce theoretical knowledge and develop problem-solving skills.
17. Use Comparisons and Contrasts
Highlighting similarities and differences between related concepts can help clarify their unique characteristics and use cases.
Example: Explaining the Difference Between Arrays and Linked Lists
Feature | Array | Linked List |
---|---|---|
Memory allocation | Contiguous | Non-contiguous |
Element access | O(1) time complexity | O(n) time complexity |
Insertion/Deletion | Costly for large arrays | Efficient |
18. Leverage Multiple Learning Modalities
People learn in different ways. By incorporating various teaching methods, you can cater to different learning styles and reinforce concepts through multiple channels.
Example: Teaching a New Programming Language
- Visual learners: Provide flowcharts and diagrams
- Auditory learners: Offer video tutorials with verbal explanations
- Reading/writing learners: Provide written documentation and examples
- Kinesthetic learners: Offer interactive coding exercises
19. Use Scaffolding
Scaffolding involves providing support to learners and gradually removing it as they become more proficient. This approach helps bridge the gap between what learners can do on their own and what they can do with guidance.
Example: Teaching Problem-Solving in Programming
- Start by providing a complete solution and explaining each step
- Next, provide a partial solution and have learners complete it
- Then, provide only the problem statement and some hints
- Finally, present problems without any additional support
20. Encourage Peer Learning
Sometimes, learners can explain concepts to each other in ways that are more relatable than a teacher’s explanation. Encouraging peer learning can reinforce understanding for both the explainer and the listener.
Example: Pair Programming
In coding education, pair programming is a technique where two programmers work together at one workstation. One writes code while the other reviews each line of code as it is typed in. This collaborative approach can enhance understanding and catch errors early.
Conclusion
Explaining complex concepts simply is both an art and a science. It requires a deep understanding of the subject matter, empathy for the learner, and a toolkit of techniques to bridge the gap between expert knowledge and novice understanding. By employing these strategies, you can make even the most intricate ideas accessible to a wide audience.
Remember, the goal is not to oversimplify to the point of inaccuracy, but to create clear pathways of understanding that learners can follow. Whether you’re teaching coding concepts on a platform like AlgoCademy or explaining any other complex topic, these techniques can help you communicate more effectively and foster deeper understanding in your audience.
As you continue to practice and refine your explanation skills, you’ll find that the ability to simplify complex ideas is not just valuable in educational settings, but in all areas of life where clear communication is key. From technical interviews to business presentations, the skill of making the complex simple is one that will serve you well in countless situations.