How to Embrace Trial and Error When Developing Algorithms
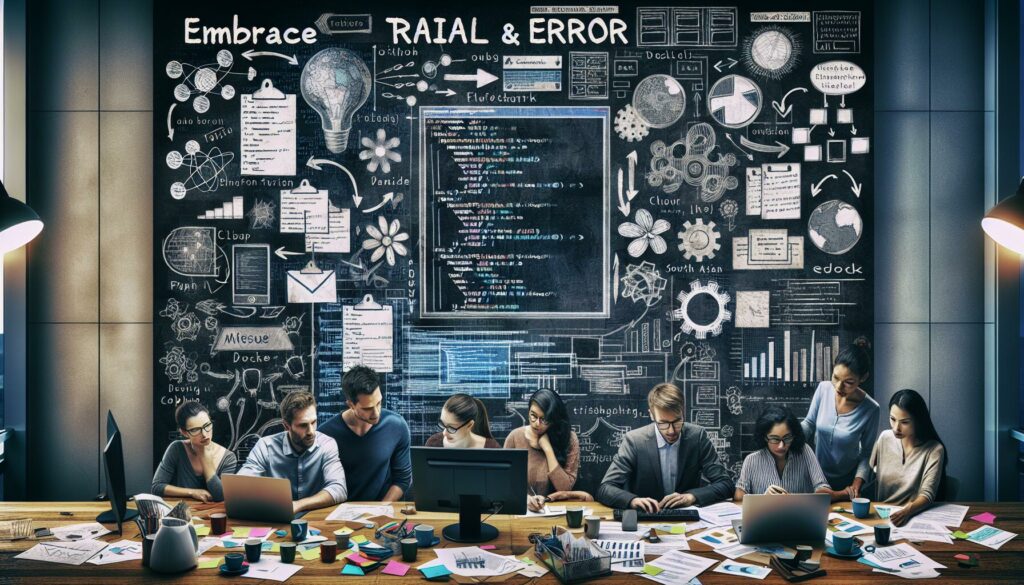
In the world of programming and algorithm development, trial and error is not just a methodology; it’s an essential mindset that can lead to breakthrough solutions and profound learning experiences. As we delve into the art of algorithm design, particularly in the context of coding education and interview preparation, understanding how to effectively utilize trial and error becomes crucial. This approach is especially relevant when tackling complex problems often encountered in technical interviews for major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, and Google).
Understanding the Importance of Trial and Error in Algorithm Development
Before we dive into the practical aspects of embracing trial and error, it’s important to recognize why this approach is so valuable in algorithm development:
- Promotes Creative Problem-Solving: Trial and error encourages you to think outside the box and explore multiple solutions.
- Enhances Learning: By making mistakes and correcting them, you gain a deeper understanding of the problem and potential solutions.
- Builds Resilience: It teaches you to persevere through challenges and not be discouraged by initial failures.
- Mimics Real-World Scenarios: In actual software development, perfect solutions rarely come on the first try.
- Improves Debugging Skills: Regular practice with trial and error sharpens your ability to identify and fix issues in your code.
Strategies for Effective Trial and Error in Algorithm Development
1. Start with a Basic Solution
When faced with a complex algorithmic problem, begin with the simplest solution that comes to mind, even if it’s not optimal. This approach, often called the “brute force” method, serves as a starting point and helps you understand the problem better.
def find_max(arr):
max_value = arr[0]
for num in arr:
if num > max_value:
max_value = num
return max_value
# Example usage
numbers = [3, 7, 2, 9, 1, 5]
print(find_max(numbers)) # Output: 9
This simple implementation may not be the most efficient for large datasets, but it’s a valid starting point.
2. Analyze and Identify Bottlenecks
Once you have a working solution, analyze its performance and identify areas for improvement. Look for bottlenecks or inefficiencies in your code.
For instance, in the previous example, if we frequently need to find the maximum value in a list that’s constantly being updated, we might consider using a data structure like a max heap for more efficient operations.
3. Implement Incremental Improvements
Make small, incremental changes to your algorithm and test each modification. This step-by-step approach allows you to see the impact of each change clearly.
import heapq
class MaxHeap:
def __init__(self):
self.heap = []
def push(self, value):
heapq.heappush(self.heap, -value)
def pop_max(self):
return -heapq.heappop(self.heap)
def peek_max(self):
return -self.heap[0] if self.heap else None
# Example usage
max_heap = MaxHeap()
numbers = [3, 7, 2, 9, 1, 5]
for num in numbers:
max_heap.push(num)
print(max_heap.peek_max()) # Output: 9
This implementation using a max heap allows for more efficient insertion and retrieval of the maximum value, especially for dynamic datasets.
4. Use Visualization Tools
Visualizing your algorithm’s behavior can provide insights that might not be apparent from looking at the code alone. Use tools like debuggers, print statements, or even graphical visualizations to understand how your algorithm is working.
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n-i-1):
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
print(f"After pass {i+1}: {arr}") # Visualization
# Example usage
numbers = [64, 34, 25, 12, 22, 11, 90]
bubble_sort(numbers)
This visualization helps you see how the array changes after each pass of the bubble sort algorithm.
5. Benchmark Different Approaches
When you have multiple potential solutions, benchmark them against each other to determine which performs best under various conditions.
import time
def time_function(func, *args):
start_time = time.time()
result = func(*args)
end_time = time.time()
return result, end_time - start_time
# Example usage
def linear_search(arr, target):
for i, num in enumerate(arr):
if num == target:
return i
return -1
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
numbers = list(range(1000000))
target = 999999
linear_result, linear_time = time_function(linear_search, numbers, target)
binary_result, binary_time = time_function(binary_search, numbers, target)
print(f"Linear Search: {linear_time:.6f} seconds")
print(f"Binary Search: {binary_time:.6f} seconds")
This benchmarking helps you make informed decisions about which algorithm to use based on performance metrics.
6. Learn from Edge Cases
Pay special attention to edge cases and boundary conditions. These often reveal weaknesses in your algorithm and provide opportunities for improvement.
def divide(a, b):
if b == 0:
raise ValueError("Cannot divide by zero")
return a / b
# Test with edge cases
try:
print(divide(10, 0))
except ValueError as e:
print(f"Error: {e}")
print(divide(0, 5)) # Edge case: dividing 0 by a number
print(divide(-10, 3)) # Edge case: negative numbers
print(divide(2.5, 1.5)) # Edge case: floating-point numbers
Testing with these edge cases helps ensure your algorithm is robust and handles various scenarios correctly.
7. Collaborate and Seek Feedback
Don’t hesitate to collaborate with peers or seek feedback from more experienced developers. Different perspectives can lead to novel solutions or improvements you might not have considered.
Applying Trial and Error in Different Algorithmic Paradigms
The trial and error approach can be applied across various algorithmic paradigms. Let’s explore how it works in different contexts:
1. Greedy Algorithms
In greedy algorithms, you make the locally optimal choice at each step. Trial and error can help you refine your greedy criteria.
def coin_change_greedy(amount, coins):
coins.sort(reverse=True)
count = 0
for coin in coins:
while amount >= coin:
amount -= coin
count += 1
return count if amount == 0 else -1
# Example usage
print(coin_change_greedy(63, [1, 5, 10, 25])) # Output: 6 (25 + 25 + 10 + 1 + 1 + 1)
print(coin_change_greedy(42, [1, 5, 10, 25])) # Output: 4 (25 + 10 + 5 + 1 + 1)
Through trial and error, you might discover that this greedy approach doesn’t always yield the optimal solution for all coin denominations, leading you to explore dynamic programming solutions.
2. Dynamic Programming
Dynamic programming often involves breaking down a problem into smaller subproblems. Trial and error can help in identifying the correct subproblems and establishing the relationship between them.
def fibonacci_dp(n):
if n <= 1:
return n
dp = [0] * (n + 1)
dp[1] = 1
for i in range(2, n + 1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
# Example usage
print(fibonacci_dp(10)) # Output: 55
Through trial and error, you might refine this solution to use less space by only keeping track of the last two values, rather than the entire sequence.
3. Divide and Conquer
In divide and conquer algorithms, trial and error can help in determining the most effective way to divide the problem and combine the solutions.
def merge_sort(arr):
if len(arr) <= 1:
return arr
mid = len(arr) // 2
left = merge_sort(arr[:mid])
right = merge_sort(arr[mid:])
return merge(left, right)
def merge(left, right):
result = []
i, j = 0, 0
while i < len(left) and j < len(right):
if left[i] <= right[j]:
result.append(left[i])
i += 1
else:
result.append(right[j])
j += 1
result.extend(left[i:])
result.extend(right[j:])
return result
# Example usage
print(merge_sort([38, 27, 43, 3, 9, 82, 10])) # Output: [3, 9, 10, 27, 38, 43, 82]
Through trial and error, you might experiment with different pivot selection strategies in quicksort or explore ways to optimize the merge operation in merge sort.
Overcoming Common Challenges in Trial and Error
While embracing trial and error is crucial, it’s not without its challenges. Here are some common obstacles you might face and strategies to overcome them:
1. Frustration and Discouragement
Challenge: Repeated failures can lead to frustration and discouragement.
Solution: Reframe failures as learning opportunities. Keep a log of your attempts and what you’ve learned from each. Celebrate small victories and incremental progress.
2. Getting Stuck in Local Optima
Challenge: Sometimes, minor improvements can prevent you from seeing radically different and potentially better solutions.
Solution: Periodically step back and reconsider the problem from scratch. Try approaching the problem from a completely different angle or using a different algorithmic paradigm.
3. Inefficient Use of Time
Challenge: Unstructured trial and error can be time-consuming and inefficient.
Solution: Set time limits for each approach you try. Use a systematic method like the scientific method: form a hypothesis, test it, analyze results, and refine your approach based on findings.
4. Overlooking Theoretical Foundations
Challenge: Focusing too much on trial and error might lead to neglecting theoretical analysis and established algorithmic principles.
Solution: Balance practical experimentation with theoretical study. After each trial, reflect on how your findings align with or challenge established theories and principles in computer science.
Leveraging AI and Tools in Trial and Error
Modern technology offers various tools that can enhance your trial and error process in algorithm development:
1. AI-Powered Code Suggestions
Tools like GitHub Copilot or TabNine can provide intelligent code suggestions, helping you explore different implementations more quickly.
2. Automated Testing Frameworks
Utilize testing frameworks to automate the process of running your algorithm against various inputs and edge cases.
import unittest
def is_prime(n):
if n < 2:
return False
for i in range(2, int(n**0.5) + 1):
if n % i == 0:
return False
return True
class TestPrime(unittest.TestCase):
def test_prime_numbers(self):
self.assertTrue(is_prime(2))
self.assertTrue(is_prime(3))
self.assertTrue(is_prime(5))
self.assertTrue(is_prime(17))
self.assertTrue(is_prime(97))
def test_non_prime_numbers(self):
self.assertFalse(is_prime(1))
self.assertFalse(is_prime(4))
self.assertFalse(is_prime(15))
self.assertFalse(is_prime(100))
if __name__ == "__main__":
unittest.main()
3. Performance Profiling Tools
Use profiling tools to identify performance bottlenecks in your code, guiding your optimization efforts.
4. Version Control Systems
Leverage version control systems like Git to track different versions of your algorithm, making it easier to compare and revert changes.
Conclusion: Embracing the Journey of Algorithm Development
Embracing trial and error in algorithm development is not just about finding the right solution; it’s about embarking on a journey of continuous learning and improvement. As you tackle complex problems, remember that each attempt, successful or not, contributes to your growth as a programmer.
Key takeaways for effective trial and error in algorithm development include:
- Start simple and iterate
- Analyze and learn from each attempt
- Use visualization and benchmarking tools
- Pay attention to edge cases
- Collaborate and seek feedback
- Balance practical experimentation with theoretical knowledge
- Leverage modern tools and AI to enhance your process
By incorporating these strategies into your approach to algorithm development, you’ll not only improve your problem-solving skills but also build the resilience and creativity necessary to excel in technical interviews and real-world programming challenges.
Remember, the goal is not just to solve the problem at hand, but to develop a robust problem-solving mindset that will serve you throughout your programming career. Embrace the process, learn from each iteration, and enjoy the intellectual challenge of algorithm development. Happy coding!