How to Embrace Abstract Thinking When Coding from Scratch
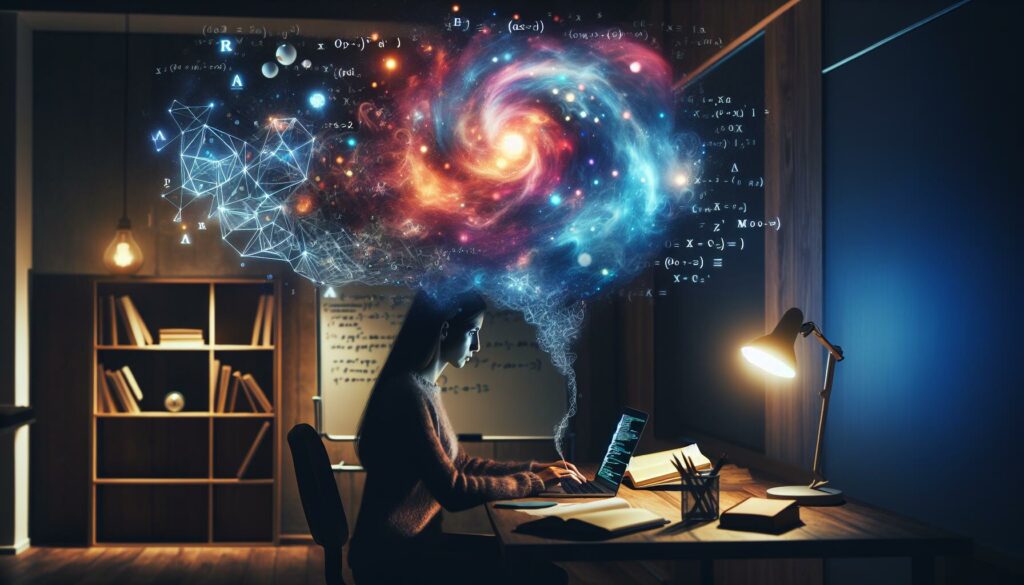
In the world of programming, the ability to think abstractly is a superpower. It’s the skill that separates good coders from great ones, enabling them to tackle complex problems with elegance and efficiency. Whether you’re a beginner just starting your coding journey or an experienced developer looking to level up your skills, embracing abstract thinking can revolutionize your approach to coding from scratch. In this comprehensive guide, we’ll explore the concept of abstract thinking in programming, its importance, and practical strategies to cultivate this crucial skill.
Understanding Abstract Thinking in Programming
Abstract thinking in programming is the ability to conceptualize complex ideas and systems without getting bogged down in the minute details. It involves seeing the bigger picture, identifying patterns, and creating mental models that represent the essence of a problem or solution. This cognitive skill allows programmers to:
- Break down complex problems into manageable components
- Design scalable and flexible software architectures
- Create reusable code and modular systems
- Understand and implement advanced algorithms and data structures
- Adapt to new programming paradigms and technologies more easily
By embracing abstract thinking, you’re not just writing code; you’re crafting solutions that can stand the test of time and scale.
The Importance of Abstract Thinking in Coding
Abstract thinking is crucial in coding for several reasons:
1. Problem-Solving Efficiency
When you think abstractly, you can quickly identify the core of a problem without getting distracted by superficial details. This leads to more efficient problem-solving and faster development cycles.
2. Code Reusability
Abstract thinkers are more likely to create generalized solutions that can be applied to a wide range of scenarios, promoting code reusability and reducing redundancy.
3. Improved Collaboration
Abstract thinking facilitates better communication among team members by providing a common language to discuss high-level concepts and system designs.
4. Innovation
By thinking abstractly, programmers can envision novel solutions and approaches that may not be immediately obvious when focusing solely on concrete implementations.
5. Adaptability
As technology evolves, abstract thinkers can more easily adapt their skills to new languages, frameworks, and paradigms by recognizing underlying principles and patterns.
Strategies to Embrace Abstract Thinking When Coding from Scratch
Now that we understand the importance of abstract thinking, let’s explore practical strategies to cultivate this skill when coding from scratch:
1. Start with Pseudocode
Before diving into actual code, begin by writing pseudocode. This high-level description of your algorithm or program flow allows you to focus on the logic and structure without getting caught up in syntax details.
Example pseudocode for a simple sorting algorithm:
function sort(list):
for each element in list:
compare with next element
if out of order:
swap elements
repeat until no swaps are needed
return sorted list
2. Use Diagrams and Visual Representations
Visual aids can help you conceptualize abstract ideas. Use flowcharts, UML diagrams, or even simple sketches to represent your program’s structure, data flow, or object relationships.
3. Practice Data Abstraction
Focus on the essential characteristics of data and hide unnecessary details. This concept is fundamental in object-oriented programming and helps in creating more manageable and modular code.
Example of data abstraction in Python:
class Car:
def __init__(self, make, model, year):
self.__make = make
self.__model = model
self.__year = year
def get_description(self):
return f"{self.__year} {self.__make} {self.__model}"
# Usage
my_car = Car("Toyota", "Corolla", 2022)
print(my_car.get_description()) # Outputs: 2022 Toyota Corolla
4. Implement Design Patterns
Design patterns are reusable solutions to common programming problems. By studying and implementing these patterns, you’ll develop a more abstract understanding of software design principles.
Example of the Singleton pattern in Java:
public class Singleton {
private static Singleton instance;
private Singleton() {}
public static Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
}
// Usage
Singleton obj1 = Singleton.getInstance();
Singleton obj2 = Singleton.getInstance();
System.out.println(obj1 == obj2); // Outputs: true
5. Practice Functional Decomposition
Break down complex problems into smaller, more manageable functions. This approach encourages you to think about your code in terms of abstract operations rather than specific implementations.
6. Explore Different Programming Paradigms
Familiarize yourself with various programming paradigms such as object-oriented, functional, and procedural programming. Each paradigm offers a different perspective on problem-solving and can enhance your abstract thinking skills.
7. Implement Abstract Data Types (ADTs)
Create and use abstract data types to separate the logical properties of data structures from their implementation details. This practice reinforces the concept of thinking in terms of operations and behaviors rather than concrete data representations.
Example of an ADT in C++:
class Stack {
private:
vector<int> elements;
public:
void push(int element) {
elements.push_back(element);
}
int pop() {
if (elements.empty()) {
throw runtime_error("Stack is empty");
}
int top = elements.back();
elements.pop_back();
return top;
}
bool isEmpty() {
return elements.empty();
}
};
// Usage
Stack myStack;
myStack.push(10);
myStack.push(20);
cout << myStack.pop(); // Outputs: 20
8. Study and Implement Algorithms
Algorithms are the epitome of abstract thinking in programming. By studying and implementing various algorithms, you’ll develop a deeper understanding of problem-solving patterns and data manipulation techniques.
9. Practice Code Refactoring
Regularly refactor your code to improve its structure without changing its functionality. This exercise helps you identify abstract patterns and relationships within your codebase.
10. Engage in Pair Programming
Collaborating with other programmers can expose you to different thinking styles and approaches to problem-solving, broadening your perspective and enhancing your abstract thinking skills.
Applying Abstract Thinking to Real-World Coding Scenarios
Let’s explore how abstract thinking can be applied to real-world coding scenarios:
Scenario 1: Building a Content Management System (CMS)
When approaching the development of a CMS from scratch, abstract thinking allows you to:
- Conceptualize the system as a set of interacting modules (e.g., user management, content creation, publishing, and templating)
- Design abstract interfaces for each module, focusing on their responsibilities rather than implementation details
- Create a flexible data model that can accommodate various content types without requiring significant code changes
- Implement a plugin architecture that allows for easy extension of functionality
Example of an abstract interface for a content module:
interface ContentManager {
public function createContent($type, $data);
public function updateContent($id, $data);
public function deleteContent($id);
public function getContent($id);
public function listContent($filters);
}
Scenario 2: Developing a Data Processing Pipeline
When creating a data processing pipeline from scratch, abstract thinking enables you to:
- Visualize the pipeline as a series of transformations on abstract data streams
- Design modular components that can be easily rearranged or replaced
- Create generic interfaces for data sources and sinks, allowing for easy addition of new input/output types
- Implement a plugin system for custom data transformations
Example of an abstract pipeline structure:
class Pipeline {
private $sources = [];
private $transformations = [];
private $sinks = [];
public function addSource(DataSource $source) {
$this->sources[] = $source;
}
public function addTransformation(Transformation $transform) {
$this->transformations[] = $transform;
}
public function addSink(DataSink $sink) {
$this->sinks[] = $sink;
}
public function execute() {
foreach ($this->sources as $source) {
$data = $source->getData();
foreach ($this->transformations as $transform) {
$data = $transform->apply($data);
}
foreach ($this->sinks as $sink) {
$sink->output($data);
}
}
}
}
Overcoming Challenges in Abstract Thinking
While embracing abstract thinking can greatly enhance your coding skills, it’s not without its challenges. Here are some common obstacles and strategies to overcome them:
1. Difficulty in Visualizing Complex Systems
Strategy: Start with simple diagrams and gradually add complexity. Use tools like mind mapping software or whiteboarding sessions to externalize your thoughts.
2. Overabstraction
Strategy: Balance abstraction with concrete implementations. Regularly validate your abstract models against real-world use cases to ensure they remain practical and useful.
3. Analysis Paralysis
Strategy: Set time limits for planning and design phases. Use iterative development approaches to refine your abstract models as you implement and test concrete code.
4. Communicating Abstract Ideas
Strategy: Practice explaining your abstract concepts using analogies and real-world examples. Develop a shared vocabulary with your team for discussing high-level concepts.
5. Maintaining Focus on the Big Picture
Strategy: Regularly step back from implementation details to review and refine your overall system architecture. Schedule dedicated time for high-level thinking and design reviews.
Tools and Resources for Enhancing Abstract Thinking Skills
To further develop your abstract thinking abilities, consider exploring the following tools and resources:
- UML Modeling Tools: Software like Lucidchart or Draw.io can help you create visual representations of your abstract ideas.
- Design Pattern Catalogs: Books like “Design Patterns: Elements of Reusable Object-Oriented Software” provide a wealth of abstract solutions to common programming problems.
- Functional Programming Languages: Languages like Haskell or Lisp can help you think more abstractly about computation and data transformation.
- Algorithm Visualization Tools: Websites like VisuAlgo can help you understand abstract algorithmic concepts through interactive visualizations.
- Code Katas and Coding Challenges: Platforms like LeetCode or HackerRank offer problems that exercise your abstract problem-solving skills.
- Open Source Projects: Contributing to large-scale open source projects can expose you to complex system designs and abstract architectural patterns.
Conclusion: Embracing the Power of Abstract Thinking
Embracing abstract thinking when coding from scratch is a transformative journey that can significantly elevate your programming skills. By focusing on high-level concepts, patterns, and relationships, you’ll be better equipped to tackle complex problems, design scalable systems, and adapt to the ever-evolving landscape of technology.
Remember that developing abstract thinking skills is an ongoing process. It requires practice, patience, and a willingness to step back from the immediate details of your code. As you continue to hone this skill, you’ll find yourself not just writing programs, but crafting elegant solutions that stand the test of time and complexity.
So, the next time you sit down to code from scratch, take a moment to think abstractly. Visualize the big picture, identify the core concepts, and let your abstract thinking guide you towards creating not just functional code, but truly inspired software. With time and practice, you’ll find that abstract thinking becomes second nature, opening up new realms of possibility in your programming journey.