How to Efficiently Use Stack and Queue Data Structures
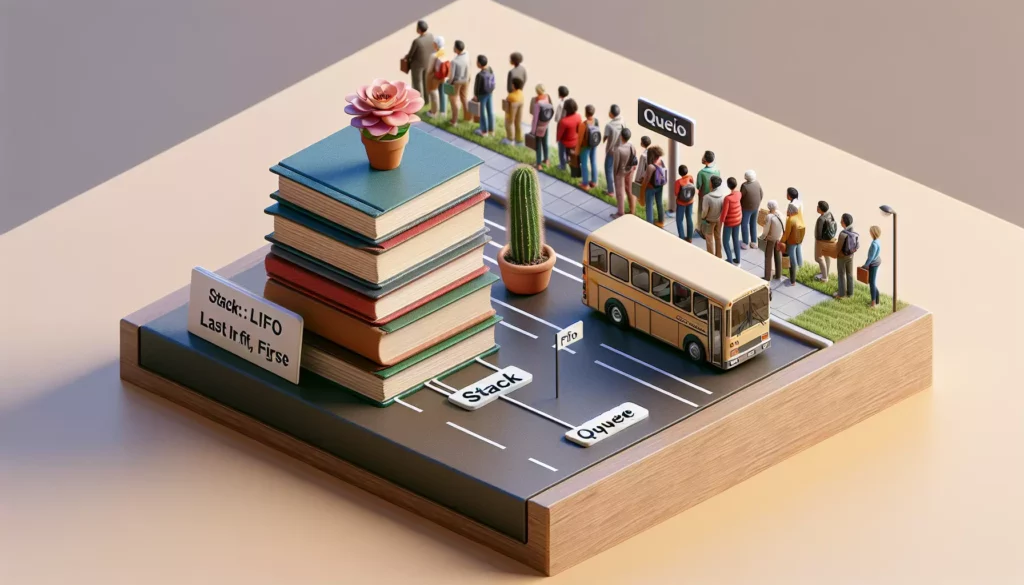
In the world of computer science and programming, data structures play a crucial role in organizing and managing information efficiently. Among these, stacks and queues are fundamental concepts that every programmer should master. Whether you’re a beginner just starting your coding journey or an experienced developer preparing for technical interviews at top tech companies, understanding how to use stacks and queues effectively can significantly enhance your problem-solving skills and algorithmic thinking.
In this comprehensive guide, we’ll dive deep into the world of stack and queue data structures, exploring their characteristics, implementations, and practical applications. By the end of this article, you’ll have a solid grasp of these essential tools and be able to leverage them in your coding projects and interview preparations.
Table of Contents
- Understanding Stacks
- Implementing Stacks
- Understanding Queues
- Implementing Queues
- Real-world Applications of Stacks and Queues
- Efficiency Considerations
- Common Problems and Solutions
- Interview Tips and Tricks
- Conclusion
1. Understanding Stacks
A stack is a linear data structure that follows the Last-In-First-Out (LIFO) principle. This means that the last element added to the stack will be the first one to be removed. Think of a stack of plates: you add plates to the top of the stack and remove them from the top as well.
Key Operations of a Stack
- Push: Add an element to the top of the stack
- Pop: Remove the top element from the stack
- Peek (or Top): View the top element without removing it
- isEmpty: Check if the stack is empty
- Size: Get the number of elements in the stack
Stacks are particularly useful in scenarios where you need to keep track of the most recent elements or when you need to reverse the order of elements.
2. Implementing Stacks
Stacks can be implemented using various underlying data structures, such as arrays or linked lists. Let’s look at both implementations in Python:
Stack Implementation using a List (Array)
class Stack:
def __init__(self):
self.items = []
def push(self, item):
self.items.append(item)
def pop(self):
if not self.is_empty():
return self.items.pop()
def peek(self):
if not self.is_empty():
return self.items[-1]
def is_empty(self):
return len(self.items) == 0
def size(self):
return len(self.items)
# Usage example
stack = Stack()
stack.push(1)
stack.push(2)
stack.push(3)
print(stack.pop()) # Output: 3
print(stack.peek()) # Output: 2
print(stack.size()) # Output: 2
Stack Implementation using a Linked List
class Node:
def __init__(self, data):
self.data = data
self.next = None
class Stack:
def __init__(self):
self.top = None
self.size = 0
def push(self, item):
new_node = Node(item)
new_node.next = self.top
self.top = new_node
self.size += 1
def pop(self):
if not self.is_empty():
item = self.top.data
self.top = self.top.next
self.size -= 1
return item
def peek(self):
if not self.is_empty():
return self.top.data
def is_empty(self):
return self.top is None
def get_size(self):
return self.size
# Usage example
stack = Stack()
stack.push(1)
stack.push(2)
stack.push(3)
print(stack.pop()) # Output: 3
print(stack.peek()) # Output: 2
print(stack.get_size()) # Output: 2
Both implementations have their advantages. The list-based implementation is simpler and leverages Python’s built-in list operations, while the linked list implementation provides more control over memory allocation and can be more efficient for large datasets.
3. Understanding Queues
A queue is another linear data structure that follows the First-In-First-Out (FIFO) principle. This means that the first element added to the queue will be the first one to be removed. Think of a queue as a line of people waiting for a service: the person who arrives first gets served first.
Key Operations of a Queue
- Enqueue: Add an element to the rear of the queue
- Dequeue: Remove the front element from the queue
- Front: View the front element without removing it
- isEmpty: Check if the queue is empty
- Size: Get the number of elements in the queue
Queues are particularly useful in scenarios where you need to process elements in the order they arrive, such as task scheduling or breadth-first search algorithms.
4. Implementing Queues
Like stacks, queues can be implemented using arrays or linked lists. Let’s look at both implementations in Python:
Queue Implementation using a List (Array)
class Queue:
def __init__(self):
self.items = []
def enqueue(self, item):
self.items.append(item)
def dequeue(self):
if not self.is_empty():
return self.items.pop(0)
def front(self):
if not self.is_empty():
return self.items[0]
def is_empty(self):
return len(self.items) == 0
def size(self):
return len(self.items)
# Usage example
queue = Queue()
queue.enqueue(1)
queue.enqueue(2)
queue.enqueue(3)
print(queue.dequeue()) # Output: 1
print(queue.front()) # Output: 2
print(queue.size()) # Output: 2
Queue Implementation using a Linked List
class Node:
def __init__(self, data):
self.data = data
self.next = None
class Queue:
def __init__(self):
self.front = None
self.rear = None
self.size = 0
def enqueue(self, item):
new_node = Node(item)
if self.is_empty():
self.front = self.rear = new_node
else:
self.rear.next = new_node
self.rear = new_node
self.size += 1
def dequeue(self):
if not self.is_empty():
item = self.front.data
self.front = self.front.next
if self.front is None:
self.rear = None
self.size -= 1
return item
def get_front(self):
if not self.is_empty():
return self.front.data
def is_empty(self):
return self.front is None
def get_size(self):
return self.size
# Usage example
queue = Queue()
queue.enqueue(1)
queue.enqueue(2)
queue.enqueue(3)
print(queue.dequeue()) # Output: 1
print(queue.get_front()) # Output: 2
print(queue.get_size()) # Output: 2
As with stacks, the choice between list-based and linked list implementations depends on the specific requirements of your application. The linked list implementation can be more efficient for large queues, especially when frequent enqueue and dequeue operations are needed.
5. Real-world Applications of Stacks and Queues
Understanding the practical applications of stacks and queues can help you recognize when to use them in your own projects. Here are some common use cases for each:
Stack Applications
- Function Call Stack: Programming languages use a stack to keep track of function calls and local variables.
- Undo Mechanism: Many applications use a stack to implement undo functionality.
- Expression Evaluation: Stacks are used to evaluate arithmetic expressions, especially in postfix notation.
- Backtracking Algorithms: Stacks are useful in backtracking algorithms, such as maze solving or game tree traversal.
- Browser History: Web browsers use a stack to implement the back button functionality.
Queue Applications
- Task Scheduling: Operating systems use queues to manage processes and tasks.
- Breadth-First Search: Queues are essential in implementing breadth-first search algorithms for graphs.
- Printer Spooler: Print jobs are typically managed using a queue.
- Message Buffers: In communication systems, queues are used to buffer messages between sender and receiver.
- Handling of Requests: Web servers often use queues to manage incoming HTTP requests.
6. Efficiency Considerations
When working with stacks and queues, it’s important to consider the time complexity of various operations. Here’s a breakdown of the time complexities for common operations:
Stack Time Complexities
- Push: O(1)
- Pop: O(1)
- Peek: O(1)
- isEmpty: O(1)
- Size: O(1)
Queue Time Complexities
- Enqueue: O(1)
- Dequeue: O(1) for linked list implementation, O(n) for array implementation
- Front: O(1)
- isEmpty: O(1)
- Size: O(1)
Note that the array implementation of a queue has O(n) time complexity for the dequeue operation because it requires shifting all elements. This can be optimized using a circular queue or a double-ended queue (deque).
7. Common Problems and Solutions
To solidify your understanding of stacks and queues, let’s look at some common problems and their solutions:
Problem 1: Valid Parentheses
Given a string containing just the characters ‘(‘, ‘)’, ‘{‘, ‘}’, ‘[‘ and ‘]’, determine if the input string is valid. An input string is valid if:
- Open brackets must be closed by the same type of brackets.
- Open brackets must be closed in the correct order.
Solution using a stack:
def is_valid(s):
stack = []
mapping = {")": "(", "}": "{", "]": "["}
for char in s:
if char in mapping:
if not stack or stack[-1] != mapping[char]:
return False
stack.pop()
else:
stack.append(char)
return len(stack) == 0
# Test cases
print(is_valid("()")) # Output: True
print(is_valid("()[]{}")) # Output: True
print(is_valid("(]")) # Output: False
print(is_valid("([)]")) # Output: False
print(is_valid("{[]}")) # Output: True
Problem 2: Implement Queue using Stacks
Implement a first in first out (FIFO) queue using only two stacks. The implemented queue should support all the functions of a normal queue (enqueue, dequeue, front, empty).
Solution:
class MyQueue:
def __init__(self):
self.stack1 = [] # For enqueue
self.stack2 = [] # For dequeue
def enqueue(self, x):
self.stack1.append(x)
def dequeue(self):
if not self.stack2:
while self.stack1:
self.stack2.append(self.stack1.pop())
if self.stack2:
return self.stack2.pop()
return None
def front(self):
if not self.stack2:
while self.stack1:
self.stack2.append(self.stack1.pop())
if self.stack2:
return self.stack2[-1]
return None
def empty(self):
return len(self.stack1) == 0 and len(self.stack2) == 0
# Test the implementation
queue = MyQueue()
queue.enqueue(1)
queue.enqueue(2)
print(queue.front()) # Output: 1
print(queue.dequeue()) # Output: 1
print(queue.empty()) # Output: False
8. Interview Tips and Tricks
When preparing for technical interviews, especially for top tech companies, it’s crucial to have a solid understanding of stacks and queues. Here are some tips to help you excel in stack and queue-related interview questions:
- Understand the basics: Make sure you can explain the fundamental concepts of stacks and queues, including their operations and time complexities.
- Practice implementations: Be comfortable implementing stacks and queues using both arrays and linked lists.
- Recognize patterns: Learn to identify problems that can be solved efficiently using stacks or queues.
- Think about edge cases: Consider empty stacks/queues, single-element structures, and other boundary conditions.
- Optimize space and time: Be prepared to discuss trade-offs between different implementations and how to optimize for specific scenarios.
- Explain your thought process: As you solve problems, articulate your reasoning and approach clearly.
- Practice common problems: Familiarize yourself with classic stack and queue problems, such as valid parentheses, implement queue using stacks, and stack min/max.
- Consider real-world applications: Be ready to discuss practical use cases of stacks and queues in software systems.
Remember, interviewers are often more interested in your problem-solving approach and communication skills than in perfect code. Practice explaining your solutions out loud, and don’t hesitate to ask clarifying questions during the interview.
9. Conclusion
Mastering stack and queue data structures is essential for any programmer looking to enhance their problem-solving skills and excel in technical interviews. These fundamental concepts form the building blocks for more complex algorithms and data structures, and their applications span across various domains of computer science and software engineering.
By understanding the characteristics, implementations, and efficient use of stacks and queues, you’ll be better equipped to tackle a wide range of programming challenges. Remember to practice regularly, implement these structures from scratch, and solve related problems to reinforce your understanding.
As you continue your journey in coding education and skills development, keep exploring more advanced data structures and algorithms. The knowledge and problem-solving techniques you gain from working with stacks and queues will serve as a solid foundation for your growth as a programmer and your success in technical interviews at top tech companies.
Happy coding, and may your stacks always be balanced and your queues efficiently managed!