How to Effectively Explain Your Code During a Coding Interview
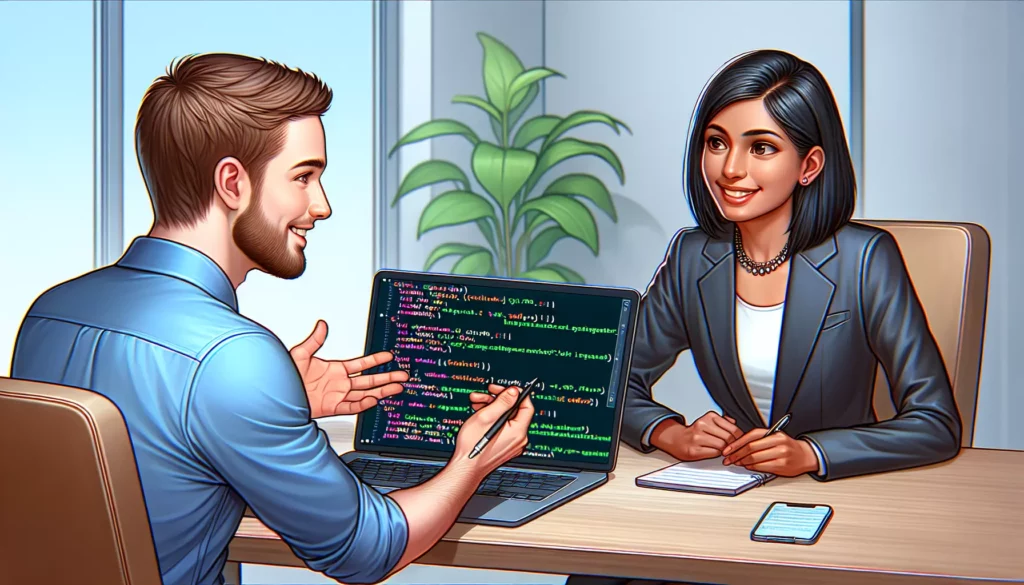
Coding interviews can be nerve-wracking experiences, especially when you’re asked to explain your code to the interviewer. However, the ability to articulate your thought process and explain your code clearly is a crucial skill that can set you apart from other candidates. In this comprehensive guide, we’ll explore effective strategies to help you explain your code during a coding interview, ensuring that you showcase your problem-solving skills and technical knowledge in the best possible light.
1. Understand the Importance of Code Explanation
Before diving into the specifics of how to explain your code, it’s essential to understand why this skill is so valuable to interviewers:
- Demonstrates communication skills: Clear code explanation shows that you can effectively communicate complex technical concepts.
- Reveals thought process: It gives insight into how you approach problem-solving and your ability to think critically.
- Shows code comprehension: Explaining your code proves that you understand what you’ve written and aren’t just memorizing solutions.
- Highlights collaboration potential: Good explanations indicate that you can work well in a team and contribute to code reviews.
2. Prepare Before the Interview
Effective code explanation starts well before the interview itself. Here are some preparation strategies:
2.1. Practice Regularly
Regularly solve coding problems and practice explaining your solutions out loud. This can help you become more comfortable with verbalizing your thought process.
2.2. Study Common Algorithms and Data Structures
Familiarize yourself with fundamental algorithms and data structures. Understanding these concepts deeply will make it easier to explain how you’re applying them in your code.
2.3. Review Your Own Code
Regularly review and refactor your old code. This practice will help you identify areas where you can improve your coding style and make your code more explainable.
3. Start with a High-Level Overview
When you begin explaining your code, start with a high-level overview of your approach. This sets the context for the detailed explanation that follows.
3.1. Explain the Problem
Begin by restating the problem in your own words. This shows that you’ve understood the requirements correctly.
3.2. Outline Your Approach
Briefly describe the overall strategy you’ve chosen to solve the problem. Mention any key algorithms or data structures you’re using.
3.3. Discuss Time and Space Complexity
If applicable, mention the time and space complexity of your solution. This demonstrates your understanding of algorithmic efficiency.
4. Walk Through Your Code Step-by-Step
After providing a high-level overview, dive into the details of your code:
4.1. Explain in Logical Chunks
Break your code into logical sections and explain each part separately. This makes your explanation easier to follow.
4.2. Use Clear and Concise Language
Avoid jargon unless you’re sure the interviewer is familiar with it. Use simple, clear language to explain complex concepts.
4.3. Highlight Key Decision Points
Emphasize important decision points in your code, explaining why you chose one approach over another.
5. Provide Context and Reasoning
As you explain your code, provide context for your decisions:
5.1. Explain Your Thought Process
Share the reasoning behind your approach. What led you to choose this particular solution?
5.2. Discuss Trade-offs
If there were multiple ways to solve the problem, explain why you chose your specific approach and what trade-offs you considered.
5.3. Mention Potential Optimizations
If you see ways to optimize your code further, mention them. This shows that you’re thinking critically about your solution.
6. Use Visual Aids When Appropriate
Sometimes, visual representations can greatly enhance your explanation:
6.1. Draw Diagrams
For complex algorithms or data structures, consider drawing diagrams to illustrate your points.
6.2. Use Examples
Walk through an example input and how your code processes it. This can make your explanation more concrete and easier to follow.
7. Be Open to Questions and Feedback
Interviewers often ask questions or provide feedback during your explanation:
7.1. Listen Carefully
Pay close attention to the interviewer’s questions and comments. They may be guiding you towards improvements or testing your ability to consider different scenarios.
7.2. Respond Thoughtfully
Take a moment to think before responding to questions. It’s okay to pause and consider your answer.
7.3. Be Open to Suggestions
If the interviewer suggests improvements, show that you’re receptive to feedback and willing to learn.
8. Practice with Real-World Examples
Let’s practice explaining code with a real-world example. Consider the following Python function that implements a binary search algorithm:
def binary_search(arr, target):
left = 0
right = len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
Here’s how you might explain this code during an interview:
“This function implements a binary search algorithm to find a target value in a sorted array. Let me walk you through the code step-by-step:
First, we initialize two pointers, ‘left’ and ‘right’, to the start and end of the array respectively. We’ll use these to keep track of the search range.
The main logic is in the while loop, which continues as long as ‘left’ is less than or equal to ‘right’. This ensures we keep searching as long as there’s a valid range to search in.
Inside the loop, we calculate the middle index ‘mid’ by taking the average of ‘left’ and ‘right’. We use integer division to ensure we get a whole number index.
We then compare the value at the middle index with our target. There are three possibilities:
- If we’ve found the target, we return the current index.
- If the middle value is less than the target, we know the target must be in the right half of the array, so we update ‘left’ to search the right half.
- Otherwise, the middle value is greater than the target, so we update ‘right’ to search the left half.
This process continues, halving the search range each time, until we either find the target or the search range becomes invalid (left > right).
If we exit the loop without finding the target, we return -1 to indicate the target isn’t in the array.
The time complexity of this algorithm is O(log n) because we’re halving the search range in each iteration. The space complexity is O(1) as we’re only using a constant amount of extra space for our variables.
One potential optimization would be to use left + (right – left) // 2 to calculate the middle index, which can prevent integer overflow for very large arrays.”
9. Common Pitfalls to Avoid
When explaining your code, be aware of these common mistakes:
9.1. Rushing Through the Explanation
Take your time to explain thoroughly. It’s better to be clear and comprehensive than to rush and miss important details.
9.2. Assuming Too Much Knowledge
Don’t assume the interviewer knows every detail about the problem or your approach. Explain things clearly, even if they seem obvious to you.
9.3. Ignoring Edge Cases
Make sure to mention how your code handles edge cases or potential error scenarios. This shows attention to detail and thorough thinking.
9.4. Being Defensive
If the interviewer points out an issue or suggests an improvement, don’t get defensive. Show that you’re open to feedback and eager to learn.
10. Handling Challenges During Code Explanation
Sometimes, you might face challenges while explaining your code. Here’s how to handle them:
10.1. If You Get Stuck
If you find yourself struggling to explain a part of your code, take a deep breath and pause. It’s okay to say something like, “Let me take a moment to gather my thoughts on this part.” Then, try to break down the problematic section into smaller, more manageable pieces.
10.2. If You Realize a Mistake
If you notice an error in your code during the explanation, don’t panic. Acknowledge the mistake, explain why it’s an issue, and describe how you would fix it. This shows honesty and the ability to catch and correct errors.
10.3. If Asked About Alternative Approaches
If the interviewer asks about alternative ways to solve the problem, be prepared to discuss them. Even if you didn’t implement these alternatives, showing that you’re aware of them demonstrates breadth of knowledge.
11. Tailoring Your Explanation to the Audience
Remember that your explanation should be tailored to your audience:
11.1. Technical Level
Try to gauge the technical level of your interviewer. If they’re highly technical, you can go into more depth. If they seem less technical, focus more on high-level concepts and the problem-solving approach.
11.2. Company Culture
If you’re aware of the company’s tech stack or coding practices, try to align your explanation with their approach. This shows that you’ve done your research and can fit into their environment.
12. Practicing Code Explanation
Improving your code explanation skills requires practice. Here are some ways to hone this skill:
12.1. Mock Interviews
Conduct mock interviews with friends, mentors, or through online platforms. This will give you practice explaining your code in a pressure situation.
12.2. Code Reviews
Participate in code reviews, either at work or in open-source projects. Explaining your code to other developers is excellent practice for interviews.
12.3. Teaching Others
Try teaching coding concepts to others, whether through tutoring, writing blog posts, or creating video tutorials. Teaching is an excellent way to improve your explanation skills.
Conclusion
Explaining your code effectively during a coding interview is a skill that can significantly boost your chances of success. By starting with a high-level overview, walking through your code step-by-step, providing context for your decisions, and being open to questions and feedback, you can showcase not just your coding abilities, but also your communication skills and problem-solving approach.
Remember, interviewers are not just looking at the correctness of your code, but also at how you think, how you communicate, and how you approach problems. By mastering the art of code explanation, you’re demonstrating that you’re not just a good coder, but a valuable potential team member who can contribute to discussions, code reviews, and collaborative problem-solving.
With practice and preparation, you can turn the code explanation part of your interview from a challenge into an opportunity to shine. Keep coding, keep explaining, and keep improving. Your future self will thank you when you’re confidently explaining your brilliant solution in your next coding interview!