How to Effectively Analyze Coding Interview Questions
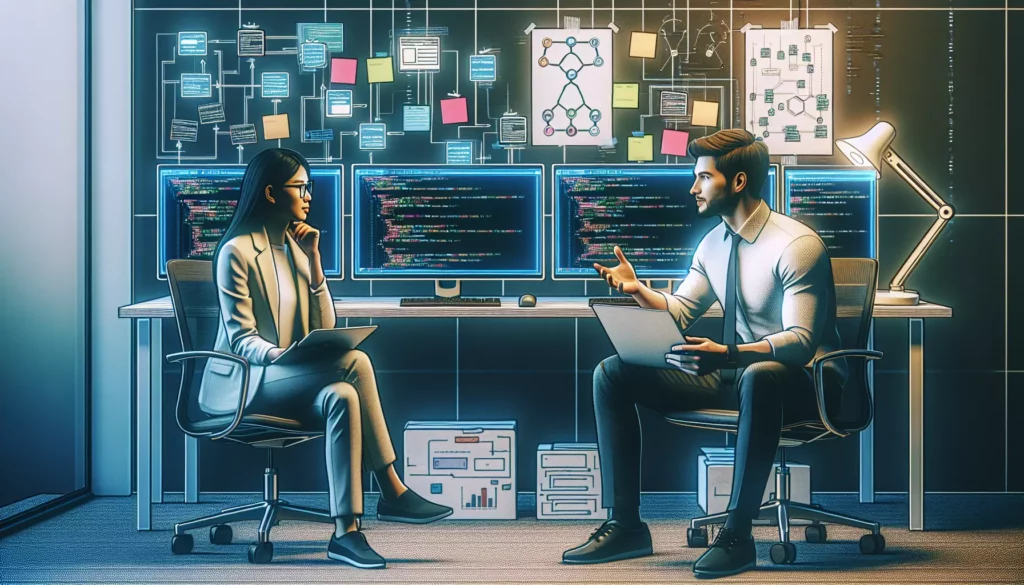
In the competitive world of tech hiring, coding interviews have become a crucial hurdle for aspiring software engineers. Whether you’re aiming for a position at a FAANG company (Facebook, Amazon, Apple, Netflix, Google) or any other tech firm, your ability to analyze and solve coding problems efficiently can make or break your chances. This comprehensive guide will walk you through the process of effectively analyzing coding interview questions, helping you approach these challenges with confidence and strategy.
1. Understanding the Importance of Analysis
Before diving into the specifics of how to analyze coding questions, it’s crucial to understand why this skill is so important. Proper analysis of a problem can:
- Save time during the actual coding process
- Help you avoid common pitfalls and edge cases
- Demonstrate your problem-solving skills to the interviewer
- Lead to more efficient and optimized solutions
- Reduce stress and increase your confidence during the interview
Remember, interviewers are often more interested in your thought process than the final code itself. A well-structured analysis showcases your ability to think critically and approach problems methodically.
2. The 5-Step Analysis Framework
To effectively analyze coding interview questions, we recommend following a 5-step framework:
- Clarify the problem
- Identify inputs and outputs
- Consider constraints and edge cases
- Develop a high-level approach
- Analyze time and space complexity
Let’s explore each of these steps in detail.
2.1. Clarify the Problem
The first step in analyzing any coding question is to ensure you fully understand what’s being asked. This involves:
- Reading the question carefully, multiple times if necessary
- Asking clarifying questions to the interviewer
- Restating the problem in your own words to confirm understanding
For example, if given a question about finding the “kth largest element in an array,” you might ask:
- Is the array sorted or unsorted?
- Can there be duplicate elements?
- What should be returned if k is larger than the array size?
Clarifying these points early can save you time and prevent misunderstandings later in the process.
2.2. Identify Inputs and Outputs
Once you understand the problem, clearly define:
- What are the inputs to the function?
- What should the function return?
- What are the types and structures of these inputs and outputs?
For instance, in the “kth largest element” problem:
- Inputs: An array of integers and an integer k
- Output: The kth largest integer in the array
Clearly defining these helps you structure your function signature and guides your thinking about the solution.
2.3. Consider Constraints and Edge Cases
This step involves thinking about:
- What are the constraints on the input? (e.g., size limits, value ranges)
- What are possible edge cases or special scenarios?
- How should the function handle invalid inputs?
For our example problem, you might consider:
- What if the array is empty?
- What if k is 0 or negative?
- What if all elements in the array are the same?
- What’s the maximum size of the array?
Addressing these scenarios demonstrates thoroughness and attention to detail.
2.4. Develop a High-Level Approach
Before writing any code, outline your approach to solving the problem. This might involve:
- Brainstorming different algorithms or data structures that could be useful
- Sketching out a flow diagram or pseudocode
- Considering multiple approaches and their trade-offs
For finding the kth largest element, you might consider approaches like:
- Sorting the array and returning the kth element from the end
- Using a min-heap of size k
- Using the QuickSelect algorithm
Discuss these approaches with your interviewer, explaining the pros and cons of each.
2.5. Analyze Time and Space Complexity
For each approach you consider, analyze its time and space complexity. This helps you and the interviewer understand the efficiency of your solution. Consider:
- Best-case, average-case, and worst-case time complexity
- Space complexity, including any additional data structures used
- How the complexity scales with input size
For example, sorting the array would have a time complexity of O(n log n), while the QuickSelect algorithm has an average-case time complexity of O(n) but a worst-case of O(n²).
3. Practical Example: Two Sum Problem
Let’s apply our 5-step framework to a classic coding interview question: the Two Sum problem.
Problem Statement: Given an array of integers and a target sum, return indices of two numbers in the array such that they add up to the target.
Step 1: Clarify the Problem
Questions to ask:
- Can there be multiple pairs that sum to the target?
- Should we return only one pair or all pairs?
- Are the numbers in the array unique?
- Can we use the same element twice?
Assumptions (based on typical expectations):
- Return only one pair of indices
- Each input would have exactly one solution
- We cannot use the same element twice
Step 2: Identify Inputs and Outputs
Inputs:
- An array of integers
- A target sum (integer)
Output:
- An array of two integers (indices of the two numbers)
Step 3: Consider Constraints and Edge Cases
- What if the array is empty or has only one element?
- Can the array contain negative numbers?
- What’s the range of the numbers in the array?
- What if there’s no solution?
Step 4: Develop a High-Level Approach
We’ll consider two approaches:
1. Brute Force Approach:
- Use nested loops to check every pair of numbers
- Return the indices if their sum equals the target
2. Hash Table Approach:
- Use a hash table to store complements
- Iterate through the array once, checking for complements
Step 5: Analyze Time and Space Complexity
Brute Force Approach:
- Time Complexity: O(n²) – we check every pair of numbers
- Space Complexity: O(1) – we only use a constant amount of extra space
Hash Table Approach:
- Time Complexity: O(n) – we only need to iterate through the array once
- Space Complexity: O(n) – in the worst case, we might need to store n-1 numbers in the hash table
Implementation
Let’s implement the more efficient Hash Table approach:
def two_sum(nums, target):
complement_dict = {}
for i, num in enumerate(nums):
complement = target - num
if complement in complement_dict:
return [complement_dict[complement], i]
complement_dict[num] = i
return [] # No solution found
This solution demonstrates how a thorough analysis leads to an efficient implementation.
4. Common Pitfalls to Avoid
When analyzing coding interview questions, be wary of these common mistakes:
- Rushing to code: Don’t start coding immediately. Take time to analyze and plan your approach.
- Ignoring constraints: Always consider the given constraints and how they might affect your solution.
- Overlooking edge cases: Think about special scenarios that might break a naive implementation.
- Failing to communicate: Share your thought process with the interviewer. They want to understand how you think.
- Fixating on one approach: Be open to alternative solutions, especially if your initial approach is suboptimal.
- Neglecting time and space complexity: Always analyze the efficiency of your solution.
- Misunderstanding the problem: Don’t hesitate to ask clarifying questions if anything is unclear.
5. Advanced Analysis Techniques
As you become more comfortable with basic analysis, consider incorporating these advanced techniques:
5.1. Pattern Recognition
Many coding problems fall into recognizable categories. Familiarize yourself with common problem types such as:
- Two-pointer techniques
- Sliding window problems
- Dynamic programming
- Graph traversal (DFS, BFS)
- Divide and conquer algorithms
Recognizing these patterns can help you quickly identify potential solution strategies.
5.2. Algorithmic Paradigms
Understanding broader algorithmic paradigms can guide your problem-solving approach:
- Greedy algorithms
- Divide and conquer
- Dynamic programming
- Backtracking
Each paradigm has its strengths and is suited to different types of problems.
5.3. Time-Space Tradeoffs
Often, you can trade time complexity for space complexity or vice versa. Consider multiple solutions and discuss these tradeoffs with your interviewer. For example:
- Using extra space to reduce time complexity (e.g., hash tables for lookups)
- In-place algorithms that save space but might be more time-consuming
5.4. Asymptotic Analysis
Go beyond basic Big O notation. Consider:
- Amortized analysis for data structures like dynamic arrays
- Average-case vs worst-case scenarios
- Lower bounds and how close your solution is to the optimal
5.5. Problem Transformation
Sometimes, transforming the problem into a known, solved problem can lead to efficient solutions. This requires:
- A broad knowledge of classic computer science problems
- The ability to see analogies between different problem domains
- Creative thinking to map your current problem onto known solutions
6. Practicing Your Analysis Skills
Improving your ability to analyze coding questions requires consistent practice. Here are some strategies to enhance your skills:
6.1. Solve Diverse Problems
Expose yourself to a wide range of problem types. Resources like LeetCode, HackerRank, and AlgoExpert offer thousands of coding challenges across various difficulty levels and topics.
6.2. Time Your Analysis
In real interviews, you’ll need to analyze problems quickly. Practice timing yourself:
- Spend 5-10 minutes on problem analysis before coding
- Try to cover all five steps of the analysis framework within this time
6.3. Mock Interviews
Participate in mock interviews where you can practice verbalizing your analysis. Platforms like Pramp or interviewing.io offer peer-to-peer mock interviews.
6.4. Review and Reflect
After solving a problem:
- Review your initial analysis. Was it accurate and comprehensive?
- Compare your solution with other approaches. What did you miss?
- Reflect on how you could improve your analysis for similar problems in the future
6.5. Study Solution Approaches
When you encounter a problem you can’t solve, don’t just look at the code solution. Study the approach and analysis behind the solution. This helps you internalize problem-solving patterns.
7. Adapting Your Analysis to Different Interview Formats
Coding interviews come in various formats, and you may need to adjust your analysis approach accordingly:
7.1. Whiteboard Interviews
In traditional whiteboard interviews:
- Focus on clear, structured thinking
- Use diagrams and pseudocode to illustrate your analysis
- Practice writing and explaining simultaneously
7.2. Online Coding Interviews
For remote interviews with shared coding environments:
- Type out your analysis steps as comments before coding
- Use code comments to explain your thought process
- Be prepared to run and test your code
7.3. Take-Home Assignments
For longer, project-style interviews:
- Document your analysis thoroughly
- Consider multiple approaches and justify your choices
- Pay extra attention to edge cases and error handling
8. Conclusion
Effective analysis of coding interview questions is a skill that can significantly improve your performance in technical interviews. By following the 5-step framework outlined in this guide and consistently practicing your analysis skills, you’ll be better prepared to tackle a wide range of coding challenges.
Remember, the goal of analysis is not just to solve the problem, but to demonstrate your problem-solving process, communication skills, and technical knowledge. Even if you don’t immediately see the optimal solution, a well-structured analysis can impress interviewers and guide you towards an effective approach.
As you continue to develop your skills, you’ll find that strong analytical abilities not only help in interviews but also make you a more effective software engineer in your day-to-day work. Keep practicing, stay curious, and approach each problem as an opportunity to showcase your analytical prowess.