How to Dry Run Code with Sample Test Cases During a Coding Interview
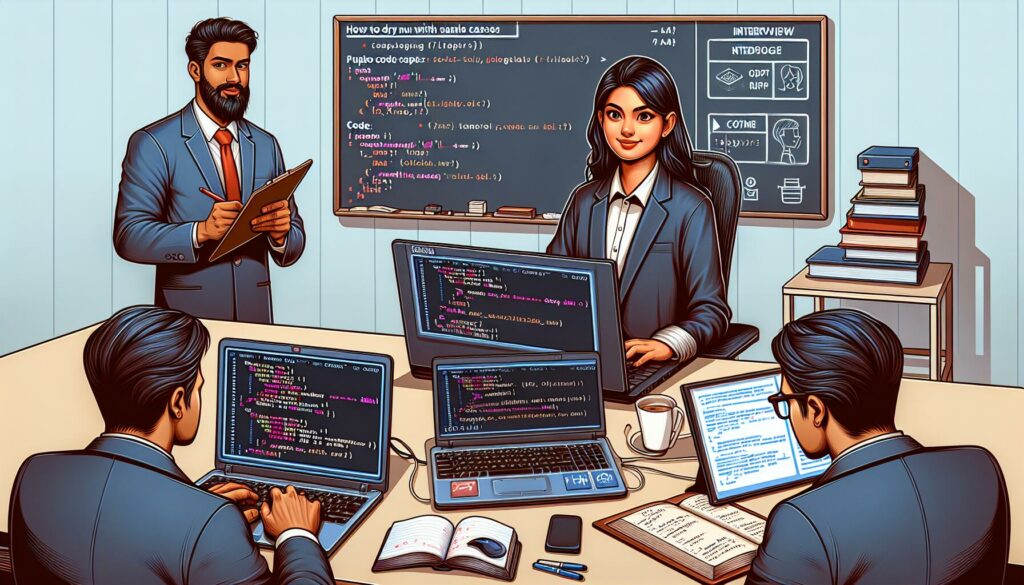
When preparing for a coding interview, one of the most crucial skills to master is the ability to dry run your code with sample test cases. This technique not only helps you validate your solution but also demonstrates your problem-solving prowess to the interviewer. In this comprehensive guide, we’ll explore the art of dry running code, its importance in coding interviews, and how to effectively use sample test cases to showcase your skills.
Table of Contents
- What is Dry Running?
- The Importance of Dry Running in Coding Interviews
- A Step-by-Step Guide to Dry Running Code
- Creating Effective Sample Test Cases
- Common Pitfalls to Avoid
- Practice Techniques to Improve Your Dry Running Skills
- Real-World Examples of Dry Running in Action
- Tools and Resources for Dry Running Practice
- Advanced Techniques for Complex Algorithms
- Understanding the Interviewer’s Perspective
- Conclusion
1. What is Dry Running?
Dry running, also known as manual code simulation or tracing, is the process of executing a program mentally or on paper, without actually running it on a computer. This technique involves stepping through the code line by line, keeping track of variable values, and simulating the program’s behavior to understand its logic and identify potential issues.
In the context of coding interviews, dry running serves as a powerful tool to:
- Verify the correctness of your solution
- Identify edge cases and potential bugs
- Demonstrate your understanding of the algorithm to the interviewer
- Improve your problem-solving skills and code comprehension
2. The Importance of Dry Running in Coding Interviews
Coding interviews at top tech companies like Google, Amazon, Facebook, Apple, and Netflix (often referred to as FAANG) are designed to assess not just your coding skills but also your problem-solving abilities and attention to detail. Here’s why dry running is crucial in these high-stakes interviews:
- Demonstrates thoroughness: By dry running your code, you show the interviewer that you’re meticulous and committed to producing high-quality, bug-free solutions.
- Reveals thought process: Walking through your code step-by-step gives the interviewer insight into your problem-solving approach and logical thinking.
- Catches errors early: Dry running helps you identify and fix bugs before submitting your final solution, improving your chances of success.
- Builds confidence: Successfully dry running your code can boost your confidence during the interview, leading to better overall performance.
- Simulates real-world scenarios: In actual software development, you’ll often need to review and understand code without running it, making dry running an essential skill beyond interviews.
3. A Step-by-Step Guide to Dry Running Code
Follow these steps to effectively dry run your code during a coding interview:
- Prepare your environment: Have a pen and paper ready, or use a whiteboard if provided.
- Choose a sample input: Select a simple, representative input to start with.
- Initialize variables: Write down the initial values of all variables.
- Step through the code: Go through each line of code, updating variable values and noting any output.
- Track control flow: Pay attention to loops, conditionals, and function calls.
- Maintain a call stack: For recursive functions, keep track of the call stack and return values.
- Note intermediate results: Write down important intermediate values to help you follow the logic.
- Verify the output: Compare your final result with the expected output.
- Analyze edge cases: Consider boundary conditions and special inputs.
- Explain your process: Articulate your thoughts and reasoning to the interviewer as you go.
4. Creating Effective Sample Test Cases
Developing a set of comprehensive test cases is crucial for thorough dry running. Here are some tips for creating effective sample test cases:
- Start with simple cases: Begin with straightforward inputs to verify basic functionality.
- Include edge cases: Test boundary conditions, such as empty inputs, single-element arrays, or maximum/minimum values.
- Consider special cases: Think about inputs that might trigger specific conditions in your code.
- Use varied data types: If applicable, test with different data types (integers, floats, strings, etc.).
- Cover all code paths: Ensure your test cases exercise all branches of your code.
- Include large inputs: Test with larger datasets to verify time and space complexity.
- Negative testing: Include invalid inputs to check error handling.
Example: For a function that finds the maximum element in an array, consider these test cases:
- Simple case: [1, 3, 5, 2, 4]
- Single element: [42]
- Empty array: []
- All identical elements: [7, 7, 7, 7]
- Negative numbers: [-5, -2, -8, -1]
- Mixed positive and negative: [-3, 0, 5, -1, 10]
- Large numbers: [1000000, 2000000, 3000000]
5. Common Pitfalls to Avoid
When dry running code during an interview, be aware of these common mistakes:
- Rushing through the process: Take your time to carefully step through each line of code.
- Overlooking edge cases: Don’t forget to consider boundary conditions and special inputs.
- Ignoring variable scope: Pay attention to variable scopes, especially in nested functions or loops.
- Mishandling reference types: Be careful when dealing with objects or arrays passed by reference.
- Neglecting off-by-one errors: Double-check loop conditions and array indices.
- Assuming correct input: Consider how your code handles invalid or unexpected inputs.
- Forgetting to reset variables: In loops or recursive calls, ensure variables are properly reset or updated.
- Losing track of the call stack: For recursive functions, maintain a clear picture of the call stack.
- Skipping error handling: Don’t overlook how your code handles potential errors or exceptions.
- Failing to communicate: Remember to explain your thought process to the interviewer as you dry run the code.
6. Practice Techniques to Improve Your Dry Running Skills
To excel at dry running during coding interviews, consider incorporating these practice techniques into your preparation routine:
- Regular code tracing exercises: Dedicate time to practicing dry runs on various algorithms and data structures.
- Peer review sessions: Partner with a friend or study group to review each other’s code and practice explaining your thought process.
- Time-boxed challenges: Set a timer and practice dry running code under time pressure to simulate interview conditions.
- Variety in problem types: Practice with a diverse range of problems, including array manipulation, string processing, tree traversals, and graph algorithms.
- Incremental complexity: Start with simple problems and gradually increase the complexity as you improve.
- Video tutorials: Watch online tutorials that demonstrate effective dry running techniques.
- Mock interviews: Participate in mock coding interviews to get real-time feedback on your dry running skills.
- Code review practice: Regularly review open-source code or solutions from coding platforms to improve your code comprehension skills.
- Teach others: Explaining algorithms and dry running processes to others can deepen your understanding and improve your communication skills.
- Visualization tools: Use online algorithm visualizers to reinforce your mental model of how different algorithms work.
7. Real-World Examples of Dry Running in Action
Let’s walk through a real-world example of dry running a simple algorithm during a coding interview. Consider the following problem:
Write a function to reverse a string in-place.
Here’s a possible solution in Python:
def reverse_string(s):
left, right = 0, len(s) - 1
s = list(s)
while left < right:
s[left], s[right] = s[right], s[left]
left += 1
right -= 1
return ''.join(s)
Now, let’s dry run this code with the input string “hello”:
- Initialize variables:
- s = [‘h’, ‘e’, ‘l’, ‘l’, ‘o’]
- left = 0
- right = 4
- First iteration of the while loop:
- Swap s[0] and s[4]: [‘o’, ‘e’, ‘l’, ‘l’, ‘h’]
- left = 1, right = 3
- Second iteration:
- Swap s[1] and s[3]: [‘o’, ‘l’, ‘l’, ‘e’, ‘h’]
- left = 2, right = 2
- While loop condition (left < right) is now false, so we exit the loop
- Join the characters and return the result: “olleh”
By walking through this process, you demonstrate to the interviewer that you understand how the algorithm works and can verify its correctness.
8. Tools and Resources for Dry Running Practice
To enhance your dry running skills, consider using these tools and resources:
- Online coding platforms: Websites like LeetCode, HackerRank, and CodeSignal offer a wide range of coding problems with built-in test cases.
- Visualization tools: Use algorithm visualizers like VisuAlgo or Algorithm Visualizer to better understand how different algorithms work.
- Debuggers: Practice using debuggers in your preferred IDE to step through code line by line.
- Whiteboard simulators: Online tools like ExcaliDraw or AWW App can help you practice whiteboarding remotely.
- Books on algorithms: “Cracking the Coding Interview” by Gayle Laakmann McDowell and “Introduction to Algorithms” by Cormen et al. are excellent resources for learning about algorithms and problem-solving techniques.
- Online courses: Platforms like Coursera, edX, and Udacity offer courses on algorithms and data structures that often include exercises in code tracing and analysis.
- Code review tools: Familiarize yourself with code review platforms like GitHub or GitLab to practice reading and understanding others’ code.
- Community forums: Participate in coding communities on platforms like Stack Overflow or Reddit’s r/cscareerquestions to discuss problem-solving approaches and dry running techniques.
- Mock interview platforms: Websites like Pramp or interviewing.io offer free peer-to-peer mock coding interviews to practice your skills in a realistic setting.
- YouTube tutorials: Watch coding interview preparation videos that demonstrate effective dry running and problem-solving techniques.
9. Advanced Techniques for Complex Algorithms
As you progress in your coding interview preparation, you’ll encounter more complex algorithms that require advanced dry running techniques. Here are some strategies to handle these challenging scenarios:
- Recursive algorithms: Maintain a clear call stack diagram, showing each recursive call and its parameters. Use indentation to represent the depth of recursion.
- Dynamic programming: Create a table to track the values of subproblems as you build up to the final solution. This helps visualize the memoization process.
- Graph algorithms: Draw the graph and update node/edge properties as you step through the algorithm. Use different colors or markers to represent visited nodes or current paths.
- Bit manipulation: Write out the binary representations of numbers and show how each operation affects the bits. Use a table to track changes in individual bit positions.
- Backtracking algorithms: Use a tree diagram to represent the decision space, and mark the paths explored and backtracked.
- Sliding window techniques: Visualize the window using brackets or highlighting, and show how it moves through the data structure.
- Two-pointer techniques: Use arrows or markers to represent the pointers and show how they move through the data structure.
- Divide and conquer algorithms: Draw diagrams to show how the problem is divided into subproblems and how the results are combined.
- State machines: Create a state diagram and track the transitions between states as you process the input.
- Complex data structures: For algorithms involving trees, heaps, or other complex structures, draw and update the structure as you step through the code.
10. Understanding the Interviewer’s Perspective
To make the most of your dry running demonstration during a coding interview, it’s essential to understand what the interviewer is looking for. Here are some key points to keep in mind:
- Clear communication: The interviewer wants to see that you can articulate your thought process clearly and concisely.
- Problem-solving approach: Demonstrate your ability to break down complex problems into manageable steps.
- Attention to detail: Show that you’re thorough and can catch potential issues before they become bugs.
- Adaptability: Be open to feedback and able to adjust your approach if the interviewer suggests alternatives.
- Time management: Balance the time spent on dry running with other aspects of the interview, such as initial problem-solving and code writing.
- Error handling: Address how your code handles edge cases and potential errors.
- Code optimization: If time allows, discuss potential optimizations or alternative approaches.
- Real-world applicability: Relate your dry running process to real-world software development scenarios.
- Curiosity and enthusiasm: Show genuine interest in the problem and eagerness to explore different aspects of the solution.
- Collaboration: Treat the dry running process as a collaborative effort with the interviewer, inviting their input and questions.
11. Conclusion
Mastering the art of dry running code with sample test cases is a crucial skill for success in coding interviews, particularly when aiming for positions at top tech companies. By following the techniques and strategies outlined in this guide, you’ll be well-equipped to demonstrate your problem-solving abilities, attention to detail, and coding proficiency.
Remember that dry running is not just about verifying your code’s correctness; it’s an opportunity to showcase your thought process, communication skills, and thoroughness as a developer. Regular practice, coupled with a deep understanding of algorithms and data structures, will help you build confidence and excel in your coding interviews.
As you continue to hone your skills, don’t forget to leverage the wealth of resources available, from online coding platforms to mock interview services. With dedication and consistent practice, you’ll be well-prepared to tackle even the most challenging coding interviews and take a significant step towards landing your dream job in the tech industry.