How to Develop Problem-Solving Skills Through Iterative Practice
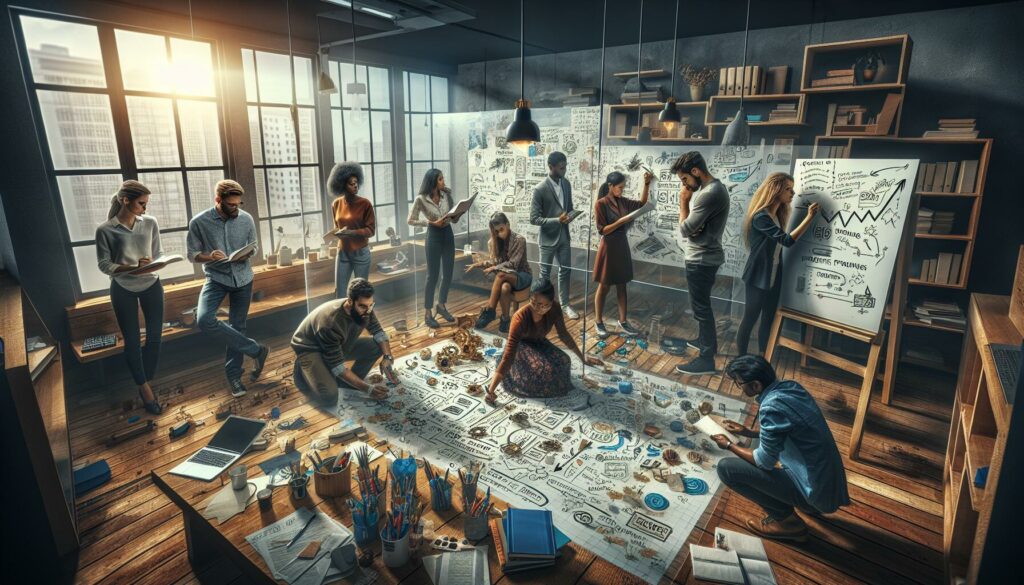
In the world of coding and software development, problem-solving skills are paramount. Whether you’re a beginner just starting your journey or an experienced developer preparing for technical interviews at major tech companies, the ability to approach and solve complex problems efficiently is a skill that can set you apart. At AlgoCademy, we believe that developing these skills through iterative practice is one of the most effective ways to improve your coding abilities and prepare for success in the tech industry.
Understanding the Importance of Problem-Solving Skills
Before diving into the methods of developing problem-solving skills, it’s crucial to understand why they are so important in the field of programming:
- Real-world application: Most programming tasks involve solving problems, from debugging code to designing efficient algorithms.
- Technical interviews: Companies like FAANG (Facebook, Amazon, Apple, Netflix, Google) heavily emphasize problem-solving skills in their hiring processes.
- Career advancement: Strong problem-solving abilities can lead to faster career progression and more challenging, rewarding projects.
- Innovation: The ability to solve complex problems creatively can lead to groundbreaking solutions and technological advancements.
The Iterative Practice Approach
Iterative practice is a method of learning and improvement that involves repeated attempts at solving problems, with each iteration building upon the knowledge gained from previous attempts. This approach is particularly effective for developing problem-solving skills in coding for several reasons:
- It reinforces concepts through repetition
- It allows for gradual increase in problem complexity
- It encourages learning from mistakes and refining solutions
- It mimics real-world scenarios where problems often require multiple attempts
Steps to Develop Problem-Solving Skills Through Iterative Practice
1. Start with the Basics
Begin your journey by mastering the fundamental concepts of programming and algorithmic thinking. This includes:
- Basic data structures (arrays, linked lists, stacks, queues)
- Common algorithms (sorting, searching)
- Time and space complexity analysis
AlgoCademy offers interactive tutorials and exercises that cover these foundational topics, allowing you to build a strong base for more advanced problem-solving.
2. Practice Regularly
Consistency is key when it comes to developing problem-solving skills. Set aside dedicated time each day or week to work on coding problems. Even 30 minutes a day can make a significant difference over time.
3. Use a Structured Approach
When tackling a problem, follow a structured approach:
- Understand the problem thoroughly
- Break it down into smaller, manageable parts
- Plan your solution
- Implement the solution
- Test and refine
This methodical approach will help you develop a systematic way of thinking about problems, which is crucial for success in technical interviews and real-world programming tasks.
4. Gradually Increase Difficulty
Start with simpler problems and progressively move to more complex ones. AlgoCademy’s platform is designed to guide you through this progression, offering problems of increasing difficulty as you improve your skills.
5. Learn Multiple Solutions
For each problem you solve, try to come up with multiple solutions. This practice will help you think creatively and understand the trade-offs between different approaches. Consider factors such as:
- Time complexity
- Space complexity
- Readability
- Scalability
6. Analyze and Learn from Mistakes
When you encounter a problem you can’t solve or make mistakes in your solution, don’t get discouraged. Instead, use it as a learning opportunity:
- Analyze where you went wrong
- Study the correct solution
- Understand the underlying concepts
- Try to solve the problem again from scratch
7. Utilize AI-Powered Assistance
AlgoCademy’s AI-powered assistance can provide hints, explanations, and step-by-step guidance when you’re stuck. This feature can help you learn new problem-solving techniques and understand complex concepts more easily.
8. Participate in Coding Challenges
Engage in coding competitions or challenges. These timed events can help you:
- Improve your ability to solve problems under pressure
- Learn from other participants
- Expose yourself to a wide variety of problem types
9. Review and Reflect
Regularly review the problems you’ve solved and reflect on your progress. This can help you identify areas for improvement and reinforce your learning.
10. Collaborate and Discuss
Engage with other learners through forums, study groups, or pair programming sessions. Discussing problems and solutions with others can provide new perspectives and deepen your understanding.
Advanced Techniques for Problem-Solving Skill Development
As you progress in your journey, consider incorporating these advanced techniques to further enhance your problem-solving abilities:
1. Implement from Scratch
Try implementing common data structures and algorithms from scratch. This exercise will deepen your understanding of how these fundamental tools work and improve your ability to use them in problem-solving.
For example, you could implement a binary search tree:
class Node:
def __init__(self, value):
self.value = value
self.left = None
self.right = None
class BinarySearchTree:
def __init__(self):
self.root = None
def insert(self, value):
if not self.root:
self.root = Node(value)
else:
self._insert_recursive(self.root, value)
def _insert_recursive(self, node, value):
if value < node.value:
if node.left is None:
node.left = Node(value)
else:
self._insert_recursive(node.left, value)
else:
if node.right is None:
node.right = Node(value)
else:
self._insert_recursive(node.right, value)
def search(self, value):
return self._search_recursive(self.root, value)
def _search_recursive(self, node, value):
if node is None or node.value == value:
return node
if value < node.value:
return self._search_recursive(node.left, value)
return self._search_recursive(node.right, value)
2. Time-Boxing
Set a specific time limit for solving a problem. This practice can help you:
- Improve your ability to work under time constraints (crucial for technical interviews)
- Learn to prioritize and focus on the most important aspects of a problem
- Develop the skill of quickly identifying potential solutions
3. Solve Problems in Multiple Programming Languages
Once you’re comfortable with one programming language, try solving problems in others. This practice will:
- Broaden your understanding of programming concepts
- Make you more adaptable to different coding environments
- Help you appreciate the strengths and weaknesses of different languages
4. Teach Others
Explaining concepts and solutions to others is an excellent way to solidify your own understanding. Consider:
- Writing blog posts about problem-solving techniques
- Creating video tutorials
- Mentoring less experienced programmers
5. Analyze Real-World Systems
Study the architecture and design of real-world systems. This can help you understand how complex problems are solved at scale. For example, you could research:
- How social media platforms handle millions of concurrent users
- How e-commerce sites manage inventory and transactions
- How streaming services deliver content efficiently
Common Problem-Solving Patterns
As you practice, you’ll start to recognize common patterns that can be applied to a wide range of problems. Familiarizing yourself with these patterns can significantly improve your problem-solving skills:
1. Two Pointers
This technique is often used for searching pairs in a sorted array or linked list. It involves maintaining two pointers that move through the data structure based on certain conditions.
Example: Finding a pair of numbers in a sorted array that sum to a target value.
def find_pair_with_sum(arr, target):
left = 0
right = len(arr) - 1
while left < right:
current_sum = arr[left] + arr[right]
if current_sum == target:
return (arr[left], arr[right])
elif current_sum < target:
left += 1
else:
right -= 1
return None
2. Sliding Window
This pattern is useful for problems involving arrays or strings where you need to find or calculate something among all subarrays or substrings of a certain size.
Example: Finding the maximum sum subarray of a fixed size k.
def max_sum_subarray(arr, k):
if len(arr) < k:
return None
window_sum = sum(arr[:k])
max_sum = window_sum
for i in range(len(arr) - k):
window_sum = window_sum - arr[i] + arr[i + k]
max_sum = max(max_sum, window_sum)
return max_sum
3. Fast and Slow Pointers
This technique is often used in linked list problems, particularly for detecting cycles or finding the middle element.
Example: Detecting a cycle in a linked list.
def has_cycle(head):
if not head or not head.next:
return False
slow = head
fast = head.next
while slow != fast:
if not fast or not fast.next:
return False
slow = slow.next
fast = fast.next.next
return True
4. Merge Intervals
This pattern is used when dealing with intervals or ranges, often to find overlapping intervals or merge them.
Example: Merging overlapping intervals.
def merge_intervals(intervals):
intervals.sort(key=lambda x: x[0])
merged = []
for interval in intervals:
if not merged or merged[-1][1] < interval[0]:
merged.append(interval)
else:
merged[-1][1] = max(merged[-1][1], interval[1])
return merged
5. Dynamic Programming
This is a powerful technique for solving optimization problems by breaking them down into simpler subproblems.
Example: Calculating the nth Fibonacci number using dynamic programming.
def fibonacci(n):
if n <= 1:
return n
dp = [0] * (n + 1)
dp[1] = 1
for i in range(2, n + 1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
Preparing for Technical Interviews
As you develop your problem-solving skills, you’ll be better prepared for technical interviews at top tech companies. Here are some additional tips to help you succeed:
1. Practice Whiteboard Coding
Many technical interviews involve coding on a whiteboard or in a shared online editor. Practice writing code without the assistance of an IDE to simulate this environment.
2. Verbalize Your Thought Process
Interviewers are often as interested in your problem-solving approach as they are in the final solution. Practice explaining your thoughts out loud as you work through problems.
3. Study Company-Specific Questions
Research and practice problems that are commonly asked by the companies you’re interested in. AlgoCademy offers curated problem sets tailored to specific companies to help you prepare.
4. Mock Interviews
Conduct mock interviews with friends, mentors, or through online platforms. This can help you get comfortable with the interview format and receive feedback on your performance.
5. Review Computer Science Fundamentals
Ensure you have a solid understanding of fundamental computer science concepts, including:
- Data structures
- Algorithms
- Object-oriented programming
- System design
Conclusion
Developing strong problem-solving skills is a journey that requires dedication, practice, and perseverance. By following the iterative practice approach and utilizing the resources and tools provided by platforms like AlgoCademy, you can significantly improve your ability to tackle complex coding challenges.
Remember that the key to success is consistent practice and a willingness to learn from both successes and failures. As you progress, you’ll find that your problem-solving skills not only prepare you for technical interviews but also make you a more effective and valuable programmer in any professional setting.
Keep challenging yourself, stay curious, and never stop learning. With time and effort, you’ll develop the problem-solving skills necessary to excel in the world of programming and tackle even the most complex coding challenges with confidence.