How to Develop Mental Toughness for Coding Challenges
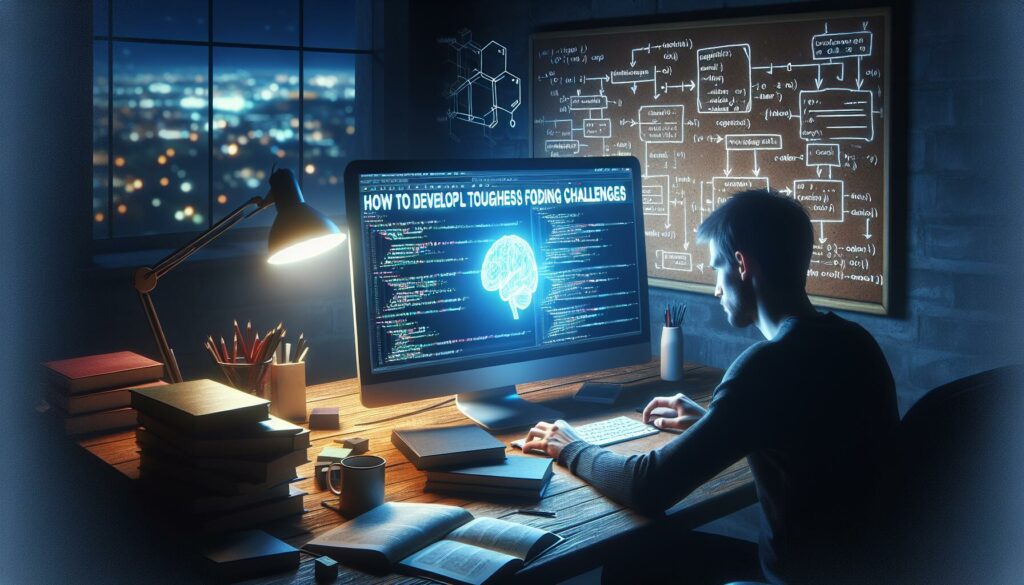
In the world of programming and software development, facing coding challenges is an inevitable part of the journey. Whether you’re a beginner learning the ropes or an experienced developer preparing for technical interviews at top tech companies, mental toughness is a crucial skill that can make or break your success. This article will explore various strategies and techniques to help you develop the mental resilience needed to tackle coding challenges head-on and emerge victorious.
Understanding Mental Toughness in Coding
Mental toughness in coding refers to the ability to maintain focus, persevere through difficulties, and perform at your best even under pressure. It’s about developing a mindset that embraces challenges as opportunities for growth rather than insurmountable obstacles. This skill is especially important when facing complex algorithms, debugging tricky issues, or participating in high-stakes coding interviews.
The Importance of Mental Toughness in Coding Challenges
Coding challenges, particularly those encountered in technical interviews for major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), can be incredibly demanding. They often require a combination of problem-solving skills, algorithmic thinking, and the ability to perform under time constraints. Mental toughness can be the deciding factor between success and failure in these high-pressure situations.
Strategies to Develop Mental Toughness
1. Embrace the Growth Mindset
Adopting a growth mindset is fundamental to developing mental toughness. This concept, popularized by psychologist Carol Dweck, emphasizes the belief that abilities and intelligence can be developed through dedication and hard work. In the context of coding challenges:
- View mistakes as learning opportunities rather than failures
- Celebrate the process of problem-solving, not just the final solution
- Embrace challenges as chances to grow your skills
2. Practice Regularly
Consistent practice is key to building mental toughness. The more you expose yourself to coding challenges, the more comfortable and confident you’ll become. Consider the following approaches:
- Set aside dedicated time each day for coding practice
- Use platforms like AlgoCademy to access a variety of coding challenges
- Participate in coding competitions or hackathons to test your skills in a competitive environment
3. Simulate Interview Conditions
To prepare for the pressure of technical interviews, try to recreate similar conditions during your practice sessions:
- Set time limits for solving problems
- Practice explaining your thought process out loud
- Have a friend or mentor act as an interviewer
4. Develop a Problem-Solving Framework
Having a structured approach to problem-solving can provide a sense of control and reduce anxiety when facing challenging problems. A common framework includes:
- Understand the problem thoroughly
- Plan your approach
- Implement the solution
- Test and refine
5. Learn from Failures
Failure is an inevitable part of the learning process. Developing mental toughness means being able to bounce back from setbacks and use them as stepping stones to improvement:
- Analyze your mistakes to understand where you went wrong
- Identify patterns in the types of problems you struggle with
- Develop targeted strategies to address your weaknesses
6. Practice Mindfulness and Stress Management
Mindfulness techniques can help you stay calm and focused during challenging coding sessions:
- Try deep breathing exercises when you feel overwhelmed
- Practice meditation to improve focus and reduce anxiety
- Take short breaks to reset your mind when stuck on a problem
7. Cultivate a Supportive Community
Surrounding yourself with like-minded individuals can provide motivation, support, and valuable insights:
- Join coding forums or study groups
- Participate in pair programming sessions
- Seek mentorship from more experienced developers
Specific Techniques for Tackling Coding Challenges
1. Break Down Complex Problems
When faced with a daunting coding challenge, break it down into smaller, manageable parts:
- Identify the core components of the problem
- Solve each component individually
- Integrate the solutions to form a complete answer
2. Use Pseudocode
Before diving into actual coding, outline your solution using pseudocode:
// Pseudocode example for a simple sorting algorithm
1. Loop through the array
2. Compare each element with the next
3. If the current element is greater than the next
3.1 Swap the elements
4. Repeat steps 1-3 until no more swaps are needed
This approach helps clarify your thinking and provides a roadmap for implementation.
3. Implement Time Management Strategies
In timed coding challenges, effective time management is crucial:
- Allocate specific time slots for understanding the problem, planning, coding, and testing
- Use the Pomodoro Technique: work in focused 25-minute intervals with short breaks in between
- If stuck on a problem, move on and return to it later if time permits
4. Utilize Rubber Duck Debugging
This technique involves explaining your code or problem to an inanimate object (traditionally a rubber duck):
- Verbalize your thought process and code logic
- This often helps identify errors or logical flaws in your approach
- Can be particularly useful in interview settings where you’re expected to think aloud
5. Practice Active Recall
Instead of passively reading solutions, actively engage with the material:
- After solving a problem, try to recreate the solution without referring to your previous work
- Explain the solution to someone else or write a blog post about it
- Revisit problems after a few days or weeks to reinforce your understanding
Common Coding Challenge Types and How to Approach Them
1. Array Manipulation
Array problems are common in coding interviews. Key strategies include:
- Understanding basic operations like insertion, deletion, and traversal
- Familiarizing yourself with common patterns like two-pointer technique or sliding window
- Practicing in-place modifications to optimize space complexity
Example problem: Two Sum
def two_sum(nums, target):
seen = {}
for i, num in enumerate(nums):
complement = target - num
if complement in seen:
return [seen[complement], i]
seen[num] = i
return []
2. String Manipulation
String problems often test your ability to handle edge cases and optimize for efficiency:
- Be comfortable with string methods in your chosen language
- Consider using data structures like hash tables for optimization
- Pay attention to issues like case sensitivity and special characters
Example problem: Valid Anagram
def is_anagram(s, t):
return sorted(s) == sorted(t)
3. Linked Lists
Linked list problems often involve manipulating pointers and handling edge cases:
- Practice implementing basic operations like insertion, deletion, and reversal
- Be comfortable with both singly and doubly linked lists
- Use the two-pointer technique for problems involving cycle detection or finding the middle element
Example problem: Reverse a Linked List
def reverse_list(head):
prev = None
current = head
while current:
next_node = current.next
current.next = prev
prev = current
current = next_node
return prev
4. Tree and Graph Traversal
These problems test your understanding of data structures and recursive thinking:
- Master both depth-first search (DFS) and breadth-first search (BFS) algorithms
- Understand the differences between various tree types (binary, binary search, n-ary)
- Practice implementing graph algorithms like Dijkstra’s or A*
Example problem: Binary Tree Inorder Traversal
def inorder_traversal(root):
result = []
def inorder(node):
if node:
inorder(node.left)
result.append(node.val)
inorder(node.right)
inorder(root)
return result
5. Dynamic Programming
Dynamic programming problems can be particularly challenging and require a systematic approach:
- Identify the base cases and recurrence relation
- Practice both top-down (memoization) and bottom-up (tabulation) approaches
- Focus on optimizing space complexity after achieving a working solution
Example problem: Fibonacci Sequence
def fibonacci(n):
if n
Overcoming Common Mental Blocks in Coding Challenges
1. Imposter Syndrome
Many developers, even experienced ones, suffer from imposter syndrome – the feeling that they’re not as competent as others perceive them to be. To combat this:
- Keep a record of your achievements and progress
- Remind yourself that everyone starts as a beginner
- Focus on personal growth rather than comparing yourself to others
2. Analysis Paralysis
Sometimes, the fear of making a mistake can lead to inaction. To overcome this:
- Start with a brute force solution to build momentum
- Set a time limit for planning before diving into coding
- Remember that any solution is better than no solution in a coding challenge
3. Perfectionism
While attention to detail is important, perfectionism can be counterproductive in time-constrained situations. To manage this:
- Focus on meeting the core requirements first
- Use comments to note areas for potential optimization
- Practice time management to ensure you complete the challenge
4. Fear of Failure
The fear of failure can be paralyzing, especially in high-stakes situations like job interviews. To address this:
- Reframe failure as a learning opportunity
- Practice positive self-talk and affirmations
- Prepare thoroughly to build confidence
Leveraging Tools and Resources
Developing mental toughness for coding challenges doesn’t mean you have to go it alone. There are numerous tools and resources available to support your journey:
1. Online Coding Platforms
Platforms like AlgoCademy offer a structured approach to learning and practicing coding challenges:
- Access a wide range of problems categorized by difficulty and topic
- Benefit from step-by-step guidance and explanations
- Track your progress and identify areas for improvement
2. AI-Powered Assistance
Many platforms now offer AI-powered tools to enhance your learning experience:
- Get personalized problem recommendations based on your skill level
- Receive hints and explanations tailored to your approach
- Analyze your code for efficiency and suggest optimizations
3. Community Forums and Discussion Boards
Engaging with the coding community can provide valuable insights and support:
- Discuss different approaches to solving problems
- Learn from others’ experiences and mistakes
- Find motivation and accountability partners
4. Technical Interview Preparation Resources
For those aiming for positions at top tech companies, specialized resources can be invaluable:
- Books like “Cracking the Coding Interview” by Gayle Laakmann McDowell
- Mock interview platforms that simulate real interview conditions
- Company-specific preparation guides and practice questions
Conclusion
Developing mental toughness for coding challenges is a journey that requires dedication, practice, and the right mindset. By embracing a growth mindset, consistently challenging yourself, and utilizing the strategies and resources outlined in this article, you can build the resilience needed to tackle even the most daunting coding problems.
Remember that mental toughness is not about never feeling stressed or overwhelmed – it’s about developing the skills to push through these feelings and perform at your best. As you continue to practice and face challenges head-on, you’ll find your confidence and problem-solving abilities growing stronger with each obstacle you overcome.
Whether you’re preparing for technical interviews at top tech companies or simply looking to improve your coding skills, the mental toughness you develop will serve you well throughout your programming career. Embrace the journey, celebrate your progress, and keep pushing your limits. With persistence and the right approach, you’ll be well-equipped to face any coding challenge that comes your way.