How to Develop Intuition for Choosing the Right Algorithm
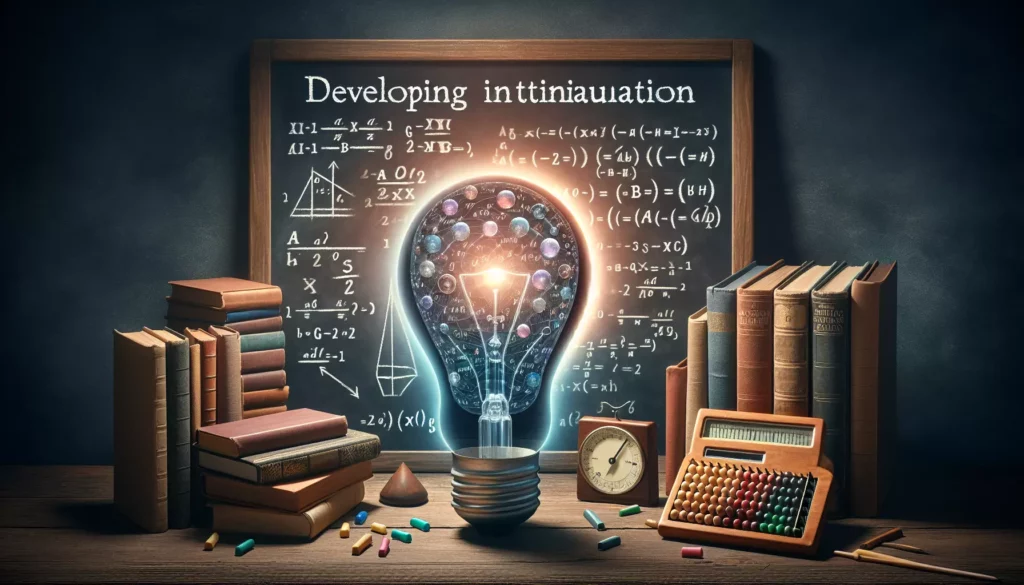
In the world of computer science and software development, the ability to choose the right algorithm for a given problem is a crucial skill. This intuition doesn’t come overnight; it’s developed through practice, experience, and a deep understanding of various algorithmic paradigms. In this comprehensive guide, we’ll explore how to cultivate this intuition, enabling you to make informed decisions when selecting algorithms for your coding projects.
Understanding the Importance of Algorithm Selection
Before we dive into the methods of developing algorithmic intuition, it’s essential to understand why choosing the right algorithm matters. The algorithm you select can significantly impact:
- Time complexity: How long your program takes to run
- Space complexity: How much memory your program uses
- Scalability: How well your solution performs as input size grows
- Code maintainability: How easy it is to understand and modify your code
Selecting an inappropriate algorithm can lead to inefficient solutions, poor performance, and even system failures in extreme cases. Therefore, developing a strong intuition for algorithm selection is not just a nice-to-have skill; it’s a necessity for any serious programmer.
Building Blocks of Algorithmic Intuition
To develop intuition for choosing the right algorithm, you need to have a solid foundation in several key areas:
1. Mastering Basic Data Structures
Understanding data structures is crucial because algorithms often rely on specific data structures to function efficiently. Familiarize yourself with:
- Arrays and Linked Lists
- Stacks and Queues
- Trees and Graphs
- Hash Tables
- Heaps
For each data structure, know its strengths, weaknesses, and common use cases. This knowledge will help you recognize which data structure might be most suitable for a given problem.
2. Grasping Algorithmic Paradigms
Algorithmic paradigms are general approaches to solving problems. The main paradigms include:
- Brute Force
- Divide and Conquer
- Dynamic Programming
- Greedy Algorithms
- Backtracking
Understanding these paradigms will help you categorize problems and identify potential solution strategies more quickly.
3. Analyzing Time and Space Complexity
Being able to analyze the efficiency of algorithms is crucial. Learn to:
- Use Big O notation to express time and space complexity
- Identify best-case, average-case, and worst-case scenarios
- Compare the efficiency of different algorithms
This skill will enable you to make informed decisions based on the performance characteristics of different algorithms.
Practical Steps to Develop Algorithmic Intuition
Now that we’ve covered the foundational knowledge, let’s explore practical steps you can take to develop your intuition for choosing the right algorithm.
1. Solve Diverse Problems
The more problems you solve, the better you’ll become at recognizing patterns and applying appropriate solutions. Here’s how to approach this:
- Start with easy problems and gradually increase difficulty
- Solve problems from various domains (e.g., string manipulation, graph theory, dynamic programming)
- Use platforms like LeetCode, HackerRank, or AlgoCademy to access a wide range of problems
As you solve more problems, you’ll start to see similarities between new problems and ones you’ve solved before, helping you choose appropriate algorithms more quickly.
2. Study Classic Algorithms
Familiarize yourself with well-known algorithms and their applications. Some essential algorithms to study include:
- Sorting algorithms (e.g., Quicksort, Mergesort, Heapsort)
- Search algorithms (e.g., Binary Search, Depth-First Search, Breadth-First Search)
- Graph algorithms (e.g., Dijkstra’s algorithm, Floyd-Warshall algorithm)
- String matching algorithms (e.g., KMP algorithm, Rabin-Karp algorithm)
Understanding these classic algorithms will give you a repertoire of tools to draw from when facing new problems.
3. Analyze and Compare Multiple Solutions
For each problem you solve, don’t stop at finding a single solution. Instead:
- Try to come up with multiple solutions using different approaches
- Analyze the time and space complexity of each solution
- Compare the trade-offs between different solutions
This practice will help you understand the strengths and weaknesses of different algorithmic approaches and when to use each one.
4. Implement Algorithms from Scratch
While it’s important to use built-in libraries and functions in real-world projects, implementing algorithms from scratch can deepen your understanding. Try to:
- Implement basic data structures (e.g., linked list, binary search tree) from scratch
- Code classic algorithms without relying on library functions
- Experiment with optimizations and variations of these algorithms
This hands-on experience will give you insights into how algorithms work under the hood, further developing your intuition.
5. Study Real-World Applications
Understanding how algorithms are used in real-world applications can help you develop intuition for practical scenarios. Consider:
- Studying case studies of how tech companies solve large-scale problems
- Analyzing the algorithms used in open-source projects
- Exploring how algorithms are applied in different fields (e.g., machine learning, computer graphics)
This broader perspective will help you understand the context in which different algorithms are most effective.
Developing a Problem-Solving Framework
As you gain more experience, it’s helpful to develop a systematic approach to problem-solving. Here’s a framework you can use:
1. Understand the Problem
- Clearly define the input and expected output
- Identify any constraints or special conditions
- Ask clarifying questions if needed
2. Analyze the Problem Characteristics
- Determine the size of the input
- Identify any patterns or structures in the data
- Consider the frequency of different operations (e.g., insertions, deletions, searches)
3. Consider Multiple Approaches
- Brainstorm different algorithmic paradigms that might apply
- Think about which data structures could be useful
- Consider both time and space complexity requirements
4. Choose the Most Appropriate Algorithm
- Evaluate the trade-offs between different approaches
- Consider the specific requirements of the problem (e.g., real-time processing, memory constraints)
- Make an informed decision based on your analysis
5. Implement and Optimize
- Code your chosen solution
- Test with various inputs, including edge cases
- Analyze the actual performance and optimize if necessary
By following this framework consistently, you’ll develop a structured approach to problem-solving that enhances your algorithmic intuition over time.
Common Pitfalls to Avoid
As you work on developing your intuition for choosing the right algorithm, be aware of these common pitfalls:
1. Overengineering
Sometimes, a simple solution is the best solution. Don’t always reach for complex algorithms when a straightforward approach will suffice. Consider the following example:
// Overengineered approach
function findMax(arr) {
return arr.reduce((max, current) => Math.max(max, current), arr[0]);
}
// Simple and efficient approach
function findMax(arr) {
let max = arr[0];
for (let i = 1; i < arr.length; i++) {
if (arr[i] > max) {
max = arr[i];
}
}
return max;
}
In this case, while the reduce method is more concise, the simple for loop is often more readable and potentially more efficient.
2. Premature Optimization
Don’t optimize before you need to. Start with a correct solution, then optimize if performance becomes an issue. As Donald Knuth famously said, “Premature optimization is the root of all evil.”
3. Ignoring Problem Constraints
Always consider the specific constraints of the problem. An algorithm that works well for small inputs might not scale for larger inputs. For example:
// Works for small n, but O(n^2) time complexity
function hasDuplicates(arr) {
for (let i = 0; i < arr.length; i++) {
for (let j = i + 1; j < arr.length; j++) {
if (arr[i] === arr[j]) return true;
}
}
return false;
}
// More efficient for large inputs, O(n) time complexity
function hasDuplicates(arr) {
const seen = new Set();
for (const num of arr) {
if (seen.has(num)) return true;
seen.add(num);
}
return false;
}
The second approach using a Set is much more efficient for large inputs.
4. Not Considering Space Complexity
While time complexity is often the primary concern, don’t forget about space complexity. Sometimes, trading space for time (or vice versa) can lead to a better overall solution.
Advanced Techniques for Developing Algorithmic Intuition
As you become more comfortable with the basics, consider these advanced techniques to further develop your intuition:
1. Study Algorithm Design Techniques
Familiarize yourself with advanced algorithm design techniques such as:
- Amortized analysis
- Randomized algorithms
- Approximation algorithms
- Online algorithms
Understanding these techniques will give you more tools to approach complex problems.
2. Explore Different Programming Paradigms
Different programming paradigms can offer new perspectives on problem-solving. Explore:
- Functional programming
- Object-oriented programming
- Declarative programming
Each paradigm has its strengths and can influence how you approach algorithmic problems.
3. Participate in Coding Competitions
Coding competitions can help you:
- Practice solving problems under time pressure
- Expose yourself to a wide variety of problem types
- Learn from other participants’ solutions
Platforms like Codeforces, TopCoder, and Google Code Jam offer regular competitions.
4. Contribute to Open Source Projects
Contributing to open source projects can provide real-world experience in:
- Analyzing existing codebases
- Optimizing algorithms for specific use cases
- Collaborating with other developers on algorithmic challenges
5. Read Research Papers
Staying up-to-date with the latest algorithmic research can provide insights into cutting-edge techniques. Some areas to explore include:
- Quantum algorithms
- Machine learning algorithms
- Distributed algorithms
Applying Algorithmic Intuition in Real-World Scenarios
Developing algorithmic intuition is not just about solving theoretical problems; it’s about applying this knowledge to real-world scenarios. Here are some examples of how algorithmic intuition can be applied in different domains:
1. Web Development
In web development, you might need to choose algorithms for:
- Efficient data retrieval from databases
- Optimizing server-side rendering
- Implementing caching mechanisms
For example, when implementing a search feature, you might choose between different string matching algorithms based on the size of the dataset and the frequency of searches.
2. Mobile App Development
In mobile app development, algorithmic choices can affect:
- App responsiveness
- Battery consumption
- Data synchronization efficiency
For instance, when implementing a news feed, you might need to balance between real-time updates and battery efficiency.
3. Data Science and Machine Learning
In data science and machine learning, algorithmic intuition is crucial for:
- Choosing appropriate machine learning models
- Optimizing data preprocessing steps
- Implementing efficient feature extraction techniques
For example, when working with large datasets, you might need to choose between exact and approximate algorithms for nearest neighbor search.
4. Game Development
In game development, algorithmic choices can impact:
- Game physics simulations
- Pathfinding for AI characters
- Procedural content generation
For instance, when implementing enemy AI, you might choose between different pathfinding algorithms based on the complexity of the game world and the number of AI agents.
Conclusion
Developing intuition for choosing the right algorithm is a journey that requires dedication, practice, and continuous learning. By building a strong foundation in data structures and algorithmic paradigms, solving diverse problems, and applying your knowledge to real-world scenarios, you can cultivate this valuable skill.
Remember that algorithmic intuition is not about memorizing solutions, but about understanding the underlying principles and trade-offs. As you gain experience, you’ll find yourself more quickly recognizing patterns and making informed decisions about which algorithms to apply in different situations.
Keep challenging yourself, stay curious, and don’t be afraid to experiment with different approaches. With time and practice, you’ll develop the intuition that sets apart great programmers and enables you to tackle complex computational problems with confidence and efficiency.