How to Develop Algorithmic Intuition and Solve Coding Problems Instantly
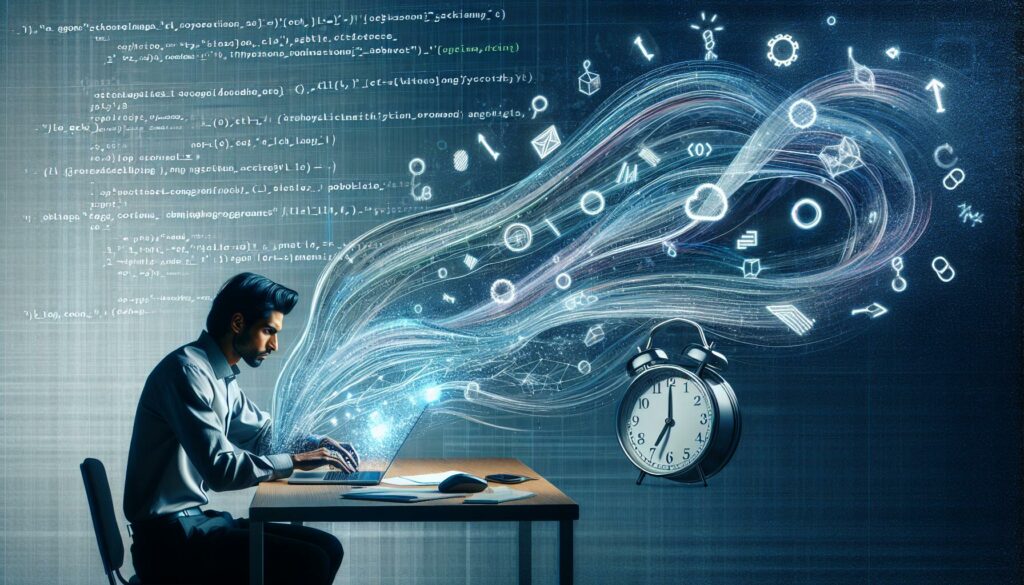
In the world of programming and software development, the ability to solve coding problems quickly and efficiently is a highly sought-after skill. Whether you’re preparing for technical interviews at top tech companies or simply aiming to become a better programmer, developing algorithmic intuition is crucial. This blog post will explore various strategies and techniques to help you build this intuition, enabling you to tackle coding challenges with confidence and ease.
Understanding Algorithmic Intuition
Before diving into the methods of developing algorithmic intuition, it’s essential to understand what it means. Algorithmic intuition refers to the ability to quickly recognize patterns, identify appropriate problem-solving techniques, and apply the right algorithms to solve a given problem. It’s not about memorizing solutions but rather about developing a deep understanding of algorithmic principles and problem-solving strategies.
The Importance of Consistent Practice
Like any skill, developing algorithmic intuition requires consistent practice. Here are some ways to incorporate regular practice into your routine:
1. Daily Coding Challenges
Set aside time each day to solve at least one coding problem. Platforms like LeetCode, HackerRank, and CodeSignal offer a wide variety of problems to choose from. Start with easier problems and gradually increase the difficulty as you become more comfortable.
2. Participate in Coding Contests
Regular participation in coding contests can help you improve your problem-solving skills under time pressure. Websites like Codeforces and TopCoder host frequent competitions that cater to different skill levels.
3. Implement Algorithms from Scratch
Try implementing common algorithms and data structures from scratch. This exercise will deepen your understanding of how these algorithms work and why they’re efficient for certain problems.
Problem Categorization and Pattern Recognition
One of the keys to developing algorithmic intuition is the ability to categorize problems and recognize common patterns. Here’s how you can improve in this area:
1. Create a Problem-Solving Framework
Develop a systematic approach to problem-solving. This could include steps like:
- Understanding the problem statement
- Identifying the input and output requirements
- Recognizing constraints and edge cases
- Brainstorming potential solutions
- Analyzing time and space complexity
- Implementing and testing the solution
2. Classify Problems by Type
As you solve more problems, try to classify them into categories such as:
- Array manipulation
- String processing
- Tree traversal
- Graph algorithms
- Dynamic programming
- Greedy algorithms
This categorization will help you quickly identify the type of problem you’re dealing with and recall relevant techniques.
3. Study Problem-Solving Patterns
Familiarize yourself with common problem-solving patterns like:
- Two-pointer technique
- Sliding window
- Depth-First Search (DFS) and Breadth-First Search (BFS)
- Binary search
- Divide and conquer
Understanding these patterns will allow you to apply them to a wide range of problems.
Reflection and Analysis
Developing algorithmic intuition isn’t just about solving problems; it’s also about reflecting on your solutions and analyzing different approaches. Here are some strategies to enhance your reflection process:
1. Analyze Multiple Solutions
After solving a problem, don’t stop at your first working solution. Explore alternative approaches and compare their efficiency. This practice will broaden your problem-solving toolkit and help you recognize when to apply different techniques.
2. Study Optimal Solutions
Even if you solve a problem correctly, take the time to study the most optimal solution. Understand why it’s more efficient and try to internalize the thought process behind it.
3. Keep a Problem-Solving Journal
Maintain a journal where you document the problems you’ve solved, the approaches you’ve used, and any insights you’ve gained. This record will serve as a valuable resource for review and reinforcement.
Building a Strong Foundation
To develop robust algorithmic intuition, it’s crucial to have a solid understanding of fundamental concepts. Here are some areas to focus on:
1. Master Basic Data Structures
Ensure you have a deep understanding of common data structures such as:
- Arrays and strings
- Linked lists
- Stacks and queues
- Trees and graphs
- Hash tables
- Heaps
Knowing when and how to use these data structures efficiently is key to solving many algorithmic problems.
2. Understand Time and Space Complexity
Develop a strong grasp of Big O notation and the ability to analyze the time and space complexity of your algorithms. This skill will help you compare different solutions and choose the most efficient one.
3. Learn Common Algorithmic Techniques
Familiarize yourself with fundamental algorithmic techniques such as:
- Recursion
- Dynamic programming
- Greedy algorithms
- Backtracking
- Divide and conquer
Understanding these techniques will provide you with a diverse set of tools to approach various problems.
Leveraging Visual Aids and Mental Models
Visual representations and mental models can significantly enhance your algorithmic intuition. Here are some ways to incorporate them into your learning process:
1. Use Flowcharts and Diagrams
When approaching a new problem, try to visualize the solution using flowcharts or diagrams. This practice can help you identify the logical steps required to solve the problem and spot potential issues or optimizations.
2. Animate Algorithms
Use animation tools or create your own animations to visualize how algorithms work. Seeing the step-by-step process of an algorithm in action can greatly improve your understanding and intuition.
3. Develop Mental Models
Create mental models or analogies for different algorithmic concepts. For example, you might think of a depth-first search as exploring a maze by always choosing the leftmost unexplored path and backtracking when you hit a dead end.
Practical Examples: Instant Insights Through Algorithmic Intuition
Let’s explore some examples where understanding an algorithm’s behavior leads to immediate insights into the solution:
Example 1: Two Sum Problem
Problem: Given an array of integers and a target sum, find two numbers in the array that add up to the target.
Intuition: If you understand how hash tables work, you can instantly recognize that this problem can be solved efficiently using a single pass through the array and a hash table to store complements.
def two_sum(nums, target):
complement_map = {}
for i, num in enumerate(nums):
complement = target - num
if complement in complement_map:
return [complement_map[complement], i]
complement_map[num] = i
return []
This solution has a time complexity of O(n) and a space complexity of O(n), which is optimal for this problem.
Example 2: Detecting a Cycle in a Linked List
Problem: Determine if a linked list contains a cycle.
Intuition: Understanding the Floyd’s Cycle-Finding Algorithm (also known as the “tortoise and hare” algorithm) provides an immediate insight into an elegant solution.
def has_cycle(head):
if not head or not head.next:
return False
slow = head
fast = head.next
while slow != fast:
if not fast or not fast.next:
return False
slow = slow.next
fast = fast.next.next
return True
This algorithm uses two pointers moving at different speeds. If there’s a cycle, the fast pointer will eventually catch up to the slow pointer.
Example 3: Finding the Longest Palindromic Substring
Problem: Given a string, find the longest palindromic substring.
Intuition: Understanding the concept of expanding around the center leads to an efficient solution without the need for complex dynamic programming.
def longest_palindrome(s):
if not s:
return ""
start = 0
max_length = 1
def expand_around_center(left, right):
while left >= 0 and right < len(s) and s[left] == s[right]:
left -= 1
right += 1
return right - left - 1
for i in range(len(s)):
len1 = expand_around_center(i, i)
len2 = expand_around_center(i, i + 1)
length = max(len1, len2)
if length > max_length:
start = i - (length - 1) // 2
max_length = length
return s[start:start + max_length]
This solution has a time complexity of O(n^2) and a space complexity of O(1), which is more efficient than many dynamic programming approaches for this problem.
Continuous Learning and Improvement
Developing algorithmic intuition is an ongoing process that requires dedication and continuous learning. Here are some additional tips to help you on your journey:
1. Read and Analyze Code
Regularly read and analyze code written by experienced developers. This practice will expose you to different coding styles, problem-solving approaches, and efficient implementations.
2. Teach Others
Explaining algorithmic concepts to others can solidify your understanding and reveal gaps in your knowledge. Consider starting a study group, writing blog posts, or creating tutorial videos.
3. Stay Updated with New Algorithms
Keep abreast of new algorithmic developments and techniques. The field of computer science is constantly evolving, and new algorithms or optimizations may provide better solutions to existing problems.
4. Solve Real-World Problems
Apply your algorithmic skills to real-world projects or open-source contributions. This practical experience will help you understand how algorithms are used in production environments and the trade-offs involved in their implementation.
Conclusion
Developing algorithmic intuition is a challenging but rewarding journey that can significantly enhance your problem-solving skills and make you a more effective programmer. By consistently practicing, categorizing problems, reflecting on your solutions, and building a strong foundation in fundamental concepts, you can train your mind to recognize patterns and apply the right algorithms instinctively.
Remember that building intuition takes time and patience. Don’t get discouraged if you don’t see immediate results. Keep practicing, stay curious, and embrace the learning process. With dedication and the right approach, you’ll find yourself solving coding problems with increasing speed and confidence.
As you continue to develop your algorithmic intuition, you’ll not only become better prepared for technical interviews but also gain a deeper appreciation for the elegance and power of efficient algorithms. This skill will serve you well throughout your career in software development, enabling you to design and implement more efficient and scalable solutions to complex problems.
So, start your journey today. Pick a problem, analyze it, solve it, and reflect on your solution. With each problem you tackle, you’ll be one step closer to mastering the art of algorithmic thinking and problem-solving.