How to Design a URL Shortener in a System Design Interview
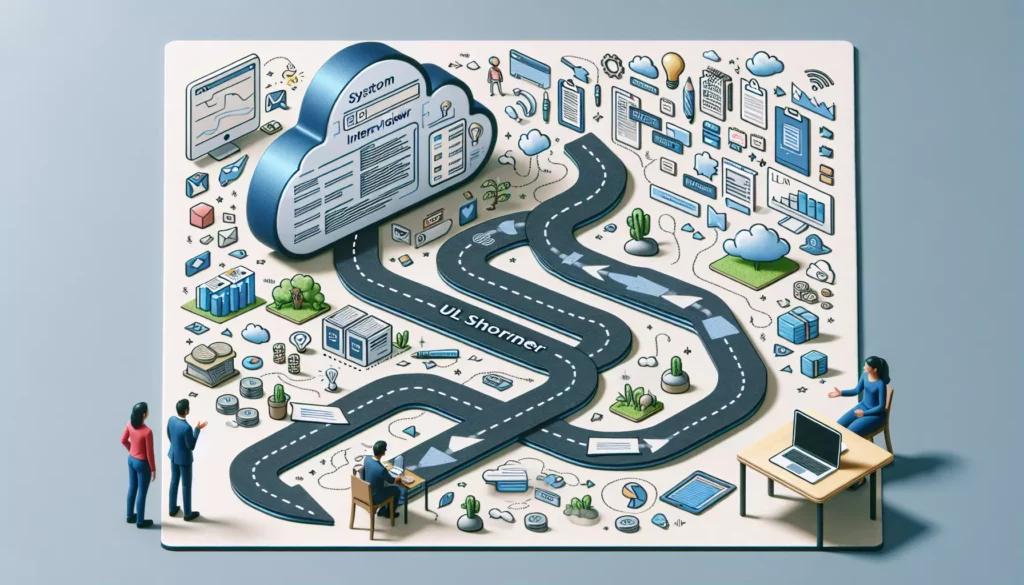
System design interviews are a crucial part of the technical interview process, especially for senior software engineering positions at major tech companies. One common problem that interviewers often present is designing a URL shortener service. This challenge tests a candidate’s ability to architect a scalable, efficient, and robust system that can handle high traffic and provide quick redirects. In this comprehensive guide, we’ll walk through the process of designing a URL shortener, covering all the essential aspects you need to consider to ace your system design interview.
1. Understanding the Problem
Before diving into the solution, it’s crucial to clarify the requirements and constraints of the system. Here are some key questions to ask the interviewer:
- What’s the expected scale of the system? (e.g., number of URLs shortened per day, number of redirects per second)
- What’s the desired length of the shortened URL?
- Do we need to support custom short URLs?
- Should the shortened URLs expire? If so, after how long?
- Do we need analytics for the shortened URLs (e.g., click tracking)?
- What’s the expected read-to-write ratio?
- Do we need to handle concurrent requests for the same long URL?
For this discussion, let’s assume the following requirements:
- The system should handle 100 million new URL shortenings per day
- The system should handle 10 billion redirects per day
- The shortened URL should be as short as possible
- The system should support custom short URLs
- URLs should not expire
- Basic analytics (click count) should be supported
- The read-to-write ratio is expected to be 100:1
2. High-Level Design
At a high level, our URL shortener system will consist of the following components:
- API Gateway: Handles incoming requests and routes them to the appropriate service
- URL Shortening Service: Generates short URLs and stores the mapping
- Redirection Service: Handles redirect requests for shortened URLs
- Database: Stores the mapping between short and long URLs
- Cache: Improves read performance by caching frequently accessed URLs
- Analytics Service: Tracks and stores click data for shortened URLs
System Architecture Diagram
<ASCII diagram>
+-------------+ +-------------------+ +------------------+
| | | | | |
| Clients +---->+ API Gateway +---->+ URL Shortening |
| | | | | Service |
+-------------+ +-------------------+ +------------------+
| |
| |
v v
+-------------------+ +------------------+
| | | |
| Redirection | | Database |
| Service | | |
+-------------------+ +------------------+
| ^
| |
v |
+-------------------+ +------------------+
| | | |
| Cache | | Analytics |
| | | Service |
+-------------------+ +------------------+
</ASCII diagram>
3. Detailed Component Design
3.1 URL Shortening Algorithm
The core of our system is the algorithm that generates short URLs. We have several options:
- Base62 Encoding: Convert a unique integer ID to a base62 string (a-z, A-Z, 0-9). This approach is simple and generates short URLs, but it reveals information about the order of URL creation.
- MD5 Hashing: Generate an MD5 hash of the long URL and take the first few characters. This approach can lead to collisions, which need to be handled.
- Counter-based Approach: Use a distributed counter to generate unique IDs and convert them to base62. This approach ensures uniqueness but requires additional infrastructure for the counter.
For our system, let’s use the counter-based approach with base62 encoding. Here’s a simple implementation in Python:
import string
def encode(num, alphabet=string.ascii_letters + string.digits):
if num == 0:
return alphabet[0]
arr = []
base = len(alphabet)
while num:
num, rem = divmod(num, base)
arr.append(alphabet[rem])
arr.reverse()
return ''.join(arr)
def decode(string, alphabet=string.ascii_letters + string.digits):
base = len(alphabet)
strlen = len(string)
num = 0
idx = 0
for char in string:
power = (strlen - (idx + 1))
num += alphabet.index(char) * (base ** power)
idx += 1
return num
3.2 Database Schema
We’ll need a database to store the mapping between short and long URLs. Here’s a simple schema:
CREATE TABLE url_mappings (
id BIGINT PRIMARY KEY AUTO_INCREMENT,
short_url VARCHAR(10) UNIQUE NOT NULL,
long_url TEXT NOT NULL,
user_id BIGINT,
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP,
click_count BIGINT DEFAULT 0
);
This schema allows us to store the necessary information and supports basic analytics (click count). We’ll use a distributed NoSQL database like Cassandra for better scalability and performance.
3.3 API Design
Our system will expose the following API endpoints:
-
Shorten URL
POST /shorten Content-Type: application/json { "long_url": "https://www.example.com/very/long/url", "custom_alias": "myurl" // optional }
Response:
{ "short_url": "https://short.url/abc123", "long_url": "https://www.example.com/very/long/url", "expiration_date": null }
-
Redirect
GET /{short_url}
This endpoint will return a 301 (Permanent Redirect) to the original long URL.
-
Get URL Analytics
GET /analytics/{short_url}
Response:
{ "short_url": "https://short.url/abc123", "long_url": "https://www.example.com/very/long/url", "click_count": 42, "created_at": "2023-05-01T12:00:00Z" }
4. Data Flow
4.1 URL Shortening Flow
- The client sends a POST request to the API Gateway with the long URL and optional custom alias.
- The API Gateway forwards the request to the URL Shortening Service.
- The URL Shortening Service checks if the long URL already exists in the database.
- If it exists, return the existing short URL.
- If it doesn’t exist:
- Generate a new unique ID using the distributed counter.
- Encode the ID to create a short URL.
- Store the mapping in the database.
- Return the new short URL to the client.
4.2 URL Redirection Flow
- The client sends a GET request to the API Gateway with the short URL.
- The API Gateway forwards the request to the Redirection Service.
- The Redirection Service checks the cache for the short URL mapping.
- If found in cache, increment the click count and return a 301 redirect to the long URL.
- If not found in cache:
- Query the database for the long URL.
- If found, add the mapping to the cache, increment the click count, and return a 301 redirect.
- If not found, return a 404 Not Found error.
5. Scalability and Performance Considerations
5.1 Database Sharding
To handle the high volume of data, we’ll need to shard our database. We can use consistent hashing to distribute the data across multiple database nodes. The sharding key could be the first few characters of the short URL.
5.2 Caching Strategy
We’ll use a distributed cache like Redis to improve read performance. The cache will store the most frequently accessed URL mappings. We can use a Least Recently Used (LRU) eviction policy to manage the cache size.
5.3 Load Balancing
We’ll use load balancers in front of our API Gateway and each service to distribute traffic evenly across multiple instances. This will help handle the high volume of requests and improve fault tolerance.
5.4 Rate Limiting
To prevent abuse, we’ll implement rate limiting at the API Gateway level. This will restrict the number of requests a client can make within a given time frame.
6. Security Considerations
6.1 Input Validation
We need to validate and sanitize all input to prevent malicious URLs or injection attacks. This includes checking the length and format of both long and short URLs.
6.2 URL Encryption
For added security, we can encrypt the long URLs before storing them in the database. This will protect sensitive information in case of a data breach.
6.3 Authentication and Authorization
If we want to support user accounts and custom aliases, we’ll need to implement an authentication system. This will also allow us to enforce per-user rate limits and provide personalized analytics.
7. Monitoring and Analytics
To ensure the health and performance of our system, we should implement comprehensive monitoring and analytics:
- System metrics: CPU usage, memory usage, disk I/O, network traffic
- Application metrics: Request latency, error rates, cache hit/miss ratios
- Business metrics: Number of URLs shortened, number of redirects, top referrers
We can use tools like Prometheus for metrics collection and Grafana for visualization.
8. Potential Improvements and Extensions
8.1 URL Expiration
We can add an expiration feature for URLs, automatically deleting them after a certain period or when they reach a click limit. This would require adding an expiration field to our database schema and implementing a cleanup process.
8.2 Advanced Analytics
We could expand our analytics capabilities to include geographic data, device types, and referrer information. This would require additional data collection and storage considerations.
8.3 API Key Management
For B2B scenarios, we could implement an API key system to allow businesses to programmatically create and manage short URLs.
8.4 Bulk URL Shortening
We could add support for shortening multiple URLs in a single request, which would be useful for large-scale operations.
9. Conclusion
Designing a URL shortener system involves carefully considering various aspects such as scalability, performance, security, and data management. The solution presented here provides a solid foundation that can handle high traffic and offers room for future expansion.
During a system design interview, it’s crucial to demonstrate your thought process and ability to make trade-offs. Be prepared to discuss alternative approaches and their pros and cons. Remember to start with clarifying requirements, present a high-level design, and then dive into specific components as time allows.
By thoroughly understanding the components and considerations involved in designing a URL shortener, you’ll be well-prepared to tackle this common system design interview question and showcase your architectural skills.