How to Design a File Storage System in a System Design Interview
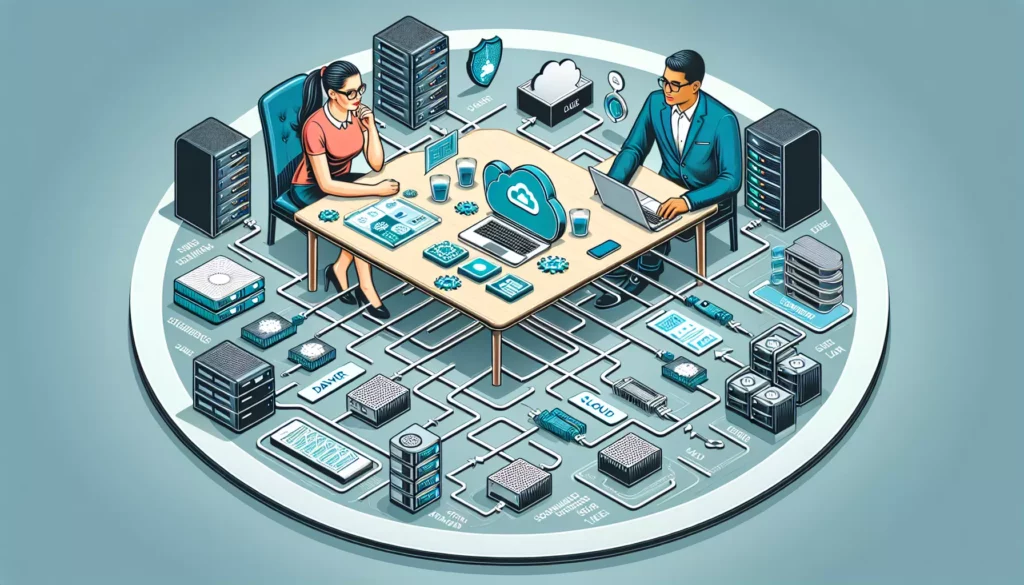
When preparing for technical interviews at top tech companies, system design questions are a crucial component that can make or break your chances. One common system design problem you might encounter is designing a file storage system. This comprehensive guide will walk you through the process of tackling this challenge in a system design interview, providing you with the knowledge and confidence to impress your interviewers.
Understanding the Problem
Before diving into the solution, it’s essential to clarify the requirements and constraints of the file storage system. Here are some key questions to ask the interviewer:
- What is the scale of the system? (e.g., number of users, file sizes, total storage capacity)
- What are the primary use cases? (e.g., personal storage, enterprise file sharing, media streaming)
- What are the performance requirements? (e.g., read/write latency, throughput)
- Are there any specific features needed? (e.g., file versioning, access control, encryption)
- What are the reliability and availability requirements?
- Are there any budget or hardware constraints?
By asking these questions, you demonstrate your ability to gather requirements and think critically about the problem at hand.
High-Level Design
Once you have a clear understanding of the requirements, you can start outlining the high-level design of the file storage system. Here’s a basic architecture to consider:
- Client Interface: This could be a web application, mobile app, or API that allows users to interact with the storage system.
- Load Balancer: Distributes incoming requests across multiple servers to ensure high availability and optimal performance.
- Application Servers: Handle user authentication, file metadata management, and coordinate file operations.
- Metadata Database: Stores information about files, users, and permissions.
- Storage Nodes: The actual servers or devices that store the file data.
- Caching Layer: Improves read performance for frequently accessed files.
- Content Delivery Network (CDN): Enhances performance for geographically distributed users.
Detailed Component Design
1. Client Interface
The client interface should provide a user-friendly way to interact with the file storage system. This could include:
- File upload and download functionality
- File organization (folders, tags)
- Search capabilities
- Sharing and collaboration features
- Access control management
For the API design, consider using RESTful endpoints for various operations:
POST /files - Upload a new file
GET /files/{fileId} - Download a file
PUT /files/{fileId} - Update file metadata
DELETE /files/{fileId} - Delete a file
GET /files - List files (with pagination and filtering)
POST /folders - Create a new folder
GET /search?q={query} - Search for files
2. Load Balancer
Implement a load balancer to distribute incoming requests across multiple application servers. This ensures high availability and helps manage traffic spikes. You can use various load balancing algorithms, such as:
- Round Robin
- Least Connections
- IP Hash
- Weighted Round Robin
Popular load balancing solutions include Nginx, HAProxy, or cloud-provided services like AWS Elastic Load Balancing.
3. Application Servers
Application servers handle the core logic of the file storage system. Key responsibilities include:
- User authentication and authorization
- File metadata management
- Coordinating file upload and download operations
- Implementing business logic (e.g., versioning, sharing)
- Interacting with the metadata database and storage nodes
Consider using a microservices architecture to separate concerns and improve scalability. For example:
- Authentication Service
- File Metadata Service
- Storage Coordination Service
- Search Service
- Sharing and Collaboration Service
4. Metadata Database
The metadata database stores information about files, users, and permissions. This could be implemented using a relational database like PostgreSQL or a NoSQL database like MongoDB, depending on the specific requirements and scale of the system.
Key tables or collections might include:
- Users
- Files
- Folders
- Permissions
- Versions
- Shares
Here’s a simplified example of a File table schema:
CREATE TABLE Files (
id UUID PRIMARY KEY,
name VARCHAR(255) NOT NULL,
size BIGINT NOT NULL,
content_type VARCHAR(100),
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP,
updated_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP,
owner_id UUID REFERENCES Users(id),
parent_folder_id UUID REFERENCES Folders(id),
storage_node_id UUID,
is_deleted BOOLEAN DEFAULT FALSE
);
5. Storage Nodes
Storage nodes are responsible for storing the actual file data. There are several approaches to implement storage nodes:
- Distributed File System: Use technologies like HDFS (Hadoop Distributed File System) or GlusterFS to distribute files across multiple nodes.
- Object Storage: Utilize object storage solutions like Amazon S3, Google Cloud Storage, or OpenStack Swift.
- Block Storage: Use block storage devices for high-performance requirements, such as Amazon EBS or local SSDs.
To ensure data durability and availability, implement replication or erasure coding across multiple storage nodes and data centers.
6. Caching Layer
Implement a caching layer to improve read performance for frequently accessed files. This can be achieved using in-memory caching solutions like Redis or Memcached. Consider caching:
- File metadata
- File content for small, frequently accessed files
- User session data
- Access control lists (ACLs)
Implement cache invalidation strategies to ensure data consistency between the cache and the primary storage.
7. Content Delivery Network (CDN)
For improved performance and reduced latency, especially for geographically distributed users, integrate a CDN into your file storage system. Popular CDN providers include:
- Cloudflare
- Akamai
- Amazon CloudFront
- Google Cloud CDN
CDNs can cache static content and even large files at edge locations closer to end-users, significantly improving download speeds and reducing the load on your primary infrastructure.
Scalability Considerations
To ensure your file storage system can handle growth and increasing demands, consider the following scalability strategies:
1. Horizontal Scaling
Design your system to scale horizontally by adding more machines to the resource pool. This applies to:
- Application servers
- Storage nodes
- Database servers (if using a distributed database)
Use auto-scaling groups to automatically adjust the number of instances based on load.
2. Database Sharding
As the metadata database grows, implement database sharding to distribute data across multiple database servers. You can shard based on:
- User ID
- File ID
- Date ranges
Ensure your sharding strategy allows for easy rebalancing and minimizes cross-shard queries.
3. Consistent Hashing
Use consistent hashing to distribute files across storage nodes. This allows for easier scaling and rebalancing of data as you add or remove storage nodes.
4. Asynchronous Processing
Implement asynchronous processing for time-consuming tasks to improve system responsiveness. Examples include:
- File upload processing (e.g., virus scanning, metadata extraction)
- Large file downloads
- Search indexing
Use message queues like RabbitMQ or Apache Kafka to manage asynchronous tasks.
Reliability and Fault Tolerance
To ensure high availability and data durability, implement the following reliability measures:
1. Data Replication
Replicate data across multiple storage nodes and data centers. Consider using techniques like:
- Master-slave replication
- Multi-master replication
- Quorum-based replication
2. Regular Backups
Implement a robust backup strategy, including:
- Full backups
- Incremental backups
- Off-site backup storage
3. Failure Detection and Recovery
Implement health checks and automatic failover mechanisms to detect and recover from node failures. This includes:
- Load balancer health checks
- Database failover
- Storage node failure handling
4. Data Integrity Checks
Regularly perform data integrity checks to detect and correct data corruption. This can include:
- Checksums
- Periodic file audits
- Data scrubbing
Security Considerations
Ensure the security of your file storage system by implementing:
1. Encryption
- Encrypt data in transit using TLS/SSL
- Implement at-rest encryption for stored files
- Use envelope encryption for key management
2. Access Control
- Implement fine-grained access control lists (ACLs)
- Use role-based access control (RBAC) for system management
- Enforce the principle of least privilege
3. Authentication and Authorization
- Implement strong user authentication (e.g., multi-factor authentication)
- Use OAuth 2.0 or OpenID Connect for third-party integrations
- Implement token-based authentication for API access
4. Auditing and Monitoring
- Log all system access and file operations
- Implement real-time monitoring and alerting for suspicious activities
- Regularly review and analyze audit logs
Performance Optimization
To ensure optimal performance of your file storage system, consider the following optimizations:
1. Caching Strategies
- Implement multi-level caching (e.g., application-level, database-level, CDN)
- Use read-through and write-through caching patterns
- Implement cache warming for predictable access patterns
2. Content Delivery Optimization
- Use dynamic CDN routing based on user location
- Implement adaptive bitrate streaming for media files
- Use HTTP/2 or HTTP/3 for improved connection efficiency
3. Database Optimization
- Implement database indexing strategies
- Use database query caching
- Optimize database schema and query patterns
4. File Chunking and Parallel Processing
- Implement file chunking for large file uploads and downloads
- Use parallel processing for file operations on large files
- Implement resumable file transfers
Monitoring and Maintenance
To ensure the ongoing health and performance of your file storage system, implement comprehensive monitoring and maintenance processes:
1. System Monitoring
- Monitor server resource utilization (CPU, memory, disk, network)
- Track application-level metrics (request rates, error rates, latencies)
- Implement distributed tracing for complex requests
- Use tools like Prometheus, Grafana, or cloud-native monitoring solutions
2. Alerting
- Set up alerts for critical system events and performance thresholds
- Implement an on-call rotation for handling urgent issues
- Use tools like PagerDuty or OpsGenie for alert management
3. Capacity Planning
- Regularly review system usage and growth trends
- Project future capacity needs based on historical data
- Plan for infrastructure upgrades and expansions
4. Regular Maintenance
- Schedule routine system updates and patches
- Perform regular database maintenance (e.g., index rebuilding, statistics updates)
- Conduct periodic security audits and penetration testing
Conclusion
Designing a file storage system for a system design interview requires a comprehensive understanding of various components and considerations. By following this guide, you’ll be well-equipped to tackle this challenge and demonstrate your ability to design scalable, reliable, and performant systems.
Remember to:
- Start by clarifying requirements and constraints
- Present a high-level design before diving into details
- Consider scalability, reliability, and security aspects
- Discuss performance optimizations and monitoring strategies
- Be prepared to make trade-offs based on specific requirements
With practice and a structured approach, you’ll be able to confidently navigate system design interviews and showcase your skills to potential employers in the tech industry.