How to Design a Coding Project Without Tutorials: A Step-by-Step Guide
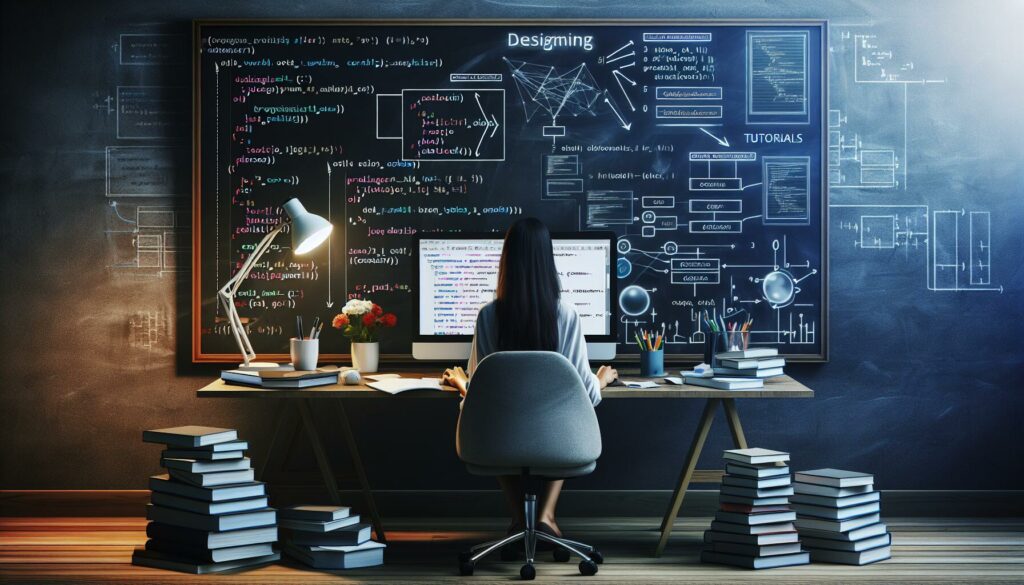
As you progress in your coding journey, there comes a time when you need to step away from tutorials and start creating projects on your own. This transition can be challenging, but it’s a crucial step in becoming a proficient programmer. In this comprehensive guide, we’ll walk you through the process of designing and implementing a coding project without relying on tutorials, helping you develop essential skills for real-world programming.
1. Define Your Project Idea
The first step in creating a coding project is to come up with a clear idea of what you want to build. This process involves several sub-steps:
1.1. Brainstorm Project Ideas
Start by generating a list of potential project ideas. Consider:
- Problems you want to solve
- Apps or tools you wish existed
- Improvements to existing applications
- Personal interests or hobbies that could benefit from a custom solution
1.2. Evaluate Feasibility
Once you have a list of ideas, assess each one based on:
- Your current skill level
- Time commitment required
- Available resources
- Potential for learning and growth
1.3. Choose Your Project
Select the idea that best balances your interests, skills, and learning objectives. Remember, it’s often better to start with a smaller, achievable project than to get overwhelmed by an overly ambitious one.
2. Research and Planning
With your project idea in mind, it’s time to dive into research and planning.
2.1. Conduct Background Research
Research similar existing projects or solutions. This will help you:
- Understand common features and functionalities
- Identify potential challenges
- Get inspiration for your own implementation
2.2. Define Project Requirements
Create a list of features and functionalities your project should have. Categorize them into:
- Must-have features (MVP – Minimum Viable Product)
- Nice-to-have features (for future iterations)
2.3. Choose Your Tech Stack
Based on your project requirements and personal preferences, decide on:
- Programming language(s)
- Frameworks or libraries
- Database (if required)
- Hosting platform (if applicable)
2.4. Create a Project Roadmap
Break down your project into smaller, manageable tasks. This could include:
- Setting up the development environment
- Designing the user interface
- Implementing core functionalities
- Testing and debugging
- Deployment and maintenance
3. Design Your Project Architecture
Before diving into coding, it’s crucial to design your project’s architecture. This step will help you organize your code and make future modifications easier.
3.1. Create a High-Level Design
Sketch out the main components of your project and how they interact. This could include:
- User interface components
- Backend services
- Data storage
- External APIs or services
3.2. Design Data Models
If your project involves data storage, design your data models. Consider:
- What data needs to be stored
- Relationships between different data entities
- Data validation rules
3.3. Plan Your API (if applicable)
If your project includes a backend API, plan out the endpoints and their functionalities. Consider:
- RESTful design principles
- Authentication and authorization
- Data formats (e.g., JSON)
4. Set Up Your Development Environment
With your plan in place, it’s time to set up your development environment.
4.1. Install Necessary Tools
Install the required software for your chosen tech stack, such as:
- Code editor or IDE
- Version control system (e.g., Git)
- Programming language runtime
- Package managers
4.2. Initialize Your Project
Set up your project structure:
- Create a new directory for your project
- Initialize version control
- Set up a basic project structure
4.3. Configure Development Tools
Set up tools to improve your development workflow:
- Linters for code quality
- Auto-formatters for consistent code style
- Task runners or build tools
5. Start Coding
Now that you have a solid plan and setup, it’s time to start coding your project.
5.1. Begin with Core Functionality
Start by implementing the most crucial features of your project. This might include:
- Setting up the basic application structure
- Implementing key algorithms or logic
- Creating essential user interface components
5.2. Use Version Control Effectively
As you code, make sure to:
- Commit changes frequently
- Use descriptive commit messages
- Create branches for different features or experiments
5.3. Write Clean, Maintainable Code
Focus on writing code that is:
- Well-organized and modular
- Properly commented and documented
- Following best practices and design patterns for your chosen language and framework
6. Overcome Challenges and Learn
As you work on your project, you’ll inevitably face challenges. Here’s how to approach them:
6.1. Break Down Complex Problems
When faced with a difficult task:
- Break it down into smaller, manageable steps
- Solve each step individually
- Combine the solutions to address the larger problem
6.2. Research and Learn Independently
When you encounter something unfamiliar:
- Consult official documentation
- Search for relevant articles or blog posts
- Explore code examples on platforms like GitHub
- Ask questions on forums like Stack Overflow, but try to solve the problem yourself first
6.3. Experiment and Iterate
Don’t be afraid to try different approaches:
- Implement a basic solution first, then refine it
- Use console logging or debugging tools to understand what’s happening in your code
- Learn from your mistakes and improve your solutions
7. Test Your Project
As you build your project, it’s crucial to test it thoroughly to ensure it works as expected.
7.1. Implement Unit Tests
Write unit tests for individual components or functions:
- Use testing frameworks appropriate for your language (e.g., Jest for JavaScript, pytest for Python)
- Test edge cases and potential failure scenarios
- Aim for high test coverage of your core functionality
7.2. Perform Integration Testing
Test how different parts of your application work together:
- Ensure components interact correctly
- Test data flow between different parts of your application
- Verify that your application works with external services or APIs as expected
7.3. Conduct User Testing
If possible, have others test your application:
- Observe how users interact with your project
- Gather feedback on usability and features
- Identify and fix any bugs or issues that arise during testing
8. Refine and Optimize
With a working version of your project, it’s time to refine and optimize it.
8.1. Refactor Your Code
Look for opportunities to improve your code:
- Remove duplicated code
- Improve naming conventions for better readability
- Optimize algorithms for better performance
- Apply design patterns where appropriate
8.2. Enhance User Experience
Focus on improving the user experience:
- Refine the user interface design
- Improve application responsiveness
- Add helpful error messages and user feedback
8.3. Optimize Performance
Look for ways to make your application faster and more efficient:
- Identify and resolve performance bottlenecks
- Optimize database queries
- Implement caching where appropriate
- Minimize network requests and payload sizes
9. Document Your Project
Proper documentation is crucial for maintaining and sharing your project.
9.1. Write a README File
Create a comprehensive README that includes:
- Project description and purpose
- Installation instructions
- Usage guide
- List of features
- Known issues or limitations
- Future development plans
9.2. Document Your Code
Ensure your code is well-documented:
- Add comments to explain complex logic or algorithms
- Use docstrings or similar tools to document functions and classes
- Keep documentation up-to-date as you make changes
9.3. Create User Documentation
If your project is intended for other users, create user documentation:
- Write a user manual or guide
- Create tutorials or walkthrough videos
- Provide FAQs or troubleshooting guides
10. Deploy and Share Your Project
With your project complete, it’s time to share it with the world.
10.1. Choose a Hosting Platform
Select an appropriate platform to host your project:
- GitHub Pages for static websites
- Heroku, DigitalOcean, or AWS for web applications
- App stores for mobile applications
10.2. Set Up Continuous Integration/Continuous Deployment (CI/CD)
Implement CI/CD to automate your deployment process:
- Use tools like Jenkins, Travis CI, or GitHub Actions
- Automate testing and deployment when pushing to specific branches
- Ensure your production environment closely matches your development environment
10.3. Share Your Project
Promote your project to potential users or collaborators:
- Share on social media or developer forums
- Write a blog post about your development process
- Present your project at local meetups or conferences
Conclusion
Designing and implementing a coding project without tutorials is a challenging but rewarding experience. It allows you to apply your skills, learn new concepts, and create something truly unique. By following this step-by-step guide, you’ll be well-equipped to tackle your next project independently, building your confidence and expertise as a programmer.
Remember, the key to success is persistence and continuous learning. Don’t be discouraged by setbacks or challenges – they’re all part of the learning process. Embrace the opportunity to problem-solve and grow as a developer. With each project you complete, you’ll become more proficient and better prepared for real-world programming challenges.
As you progress in your coding journey, consider sharing your experiences and knowledge with others. Contributing to open-source projects, writing tutorials, or mentoring beginners are great ways to reinforce your learning and give back to the programming community.
Now, armed with this guide, it’s time to embark on your next coding adventure. Choose a project that excites you, start planning, and begin bringing your ideas to life through code. Happy coding!