How to Debug Like a Pro: Master the Art of Troubleshooting Your Code
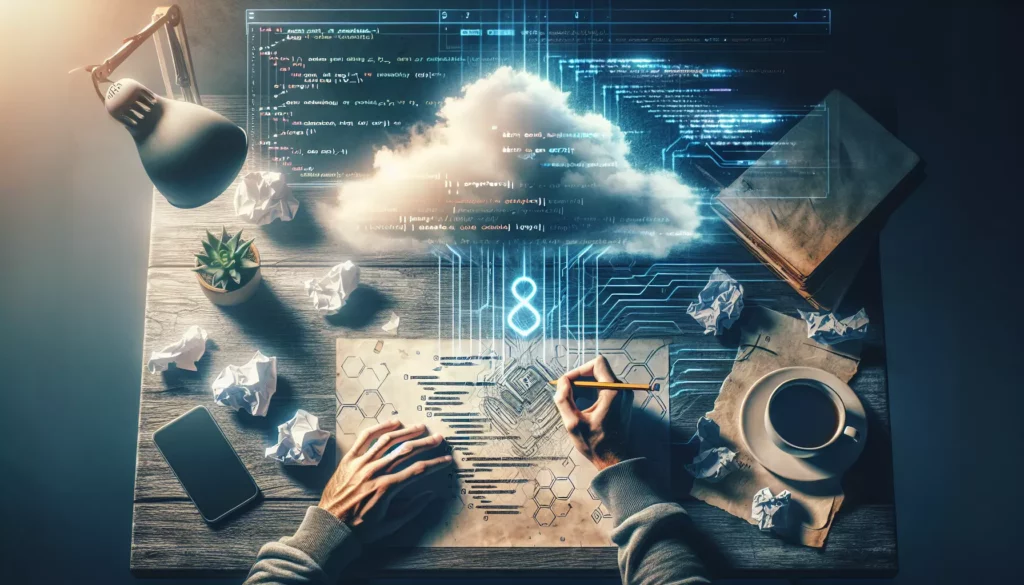
Debugging is an essential skill for every programmer, from beginners to seasoned professionals. It’s the process of identifying, isolating, and fixing errors or “bugs” in your code. While it can be frustrating at times, mastering the art of debugging can significantly improve your coding efficiency and problem-solving abilities. In this comprehensive guide, we’ll explore various techniques, tools, and best practices to help you debug like a pro.
1. Understanding the Importance of Debugging
Before diving into the specifics of debugging techniques, it’s crucial to understand why debugging is so important in the software development process:
- Code Quality: Debugging helps improve the overall quality of your code by identifying and eliminating errors.
- Time Efficiency: Effective debugging skills can save you countless hours of frustration and wasted effort.
- Problem-Solving: Debugging enhances your analytical and problem-solving skills, which are valuable in all aspects of programming.
- Learning Opportunity: Each debugging session is a chance to learn more about your code, the programming language, and potential pitfalls.
2. Common Types of Bugs
To debug effectively, it’s helpful to understand the different types of bugs you might encounter:
- Syntax Errors: These are errors in the structure of your code that prevent it from compiling or running.
- Runtime Errors: These occur when your code is executing and encounters an unexpected condition.
- Logical Errors: These are the trickiest to spot, as the code runs without crashing but produces incorrect results.
- Semantic Errors: These occur when the code doesn’t do what you intended it to do, even if it runs without errors.
3. Essential Debugging Techniques
3.1. Print Debugging
One of the simplest yet effective debugging techniques is print debugging. This involves adding print statements to your code to track the flow of execution and inspect variable values at different points.
Example in Python:
def calculate_sum(a, b):
print(f"Calculating sum of {a} and {b}")
result = a + b
print(f"Result: {result}")
return result
total = calculate_sum(5, 3)
print(f"Total: {total}")
While simple, print debugging can be powerful for understanding the flow of your program and identifying where things might be going wrong.
3.2. Using a Debugger
Most modern Integrated Development Environments (IDEs) come with built-in debuggers. These tools allow you to:
- Set breakpoints in your code
- Step through your code line by line
- Inspect variable values at runtime
- Modify variable values during execution
Learning to use a debugger effectively can significantly speed up your debugging process. Here’s a basic example of how you might use a debugger in Python using the `pdb` module:
import pdb
def complex_function(x, y):
result = x * y
pdb.set_trace() # This will pause execution and start the debugger
return result * 2
complex_function(5, 3)
When you run this code, it will pause at the `pdb.set_trace()` line, allowing you to inspect variables and step through the code.
3.3. Rubber Duck Debugging
This technique involves explaining your code, line by line, to an inanimate object (traditionally a rubber duck). The act of verbalizing your code often helps you spot errors or inconsistencies that you might have overlooked while coding silently.
3.4. Divide and Conquer
When dealing with a large codebase, the divide and conquer approach can be very effective. This involves:
- Isolating the bug to a specific section of code
- Commenting out portions of code to narrow down the problem area
- Gradually adding back code until the bug reappears
3.5. Log File Analysis
For larger applications, especially those running on servers, log file analysis is crucial. Implement comprehensive logging in your application and learn to parse and analyze these logs effectively.
4. Advanced Debugging Strategies
4.1. Unit Testing
While not strictly a debugging technique, implementing unit tests can help prevent bugs and make debugging easier when issues do arise. By testing individual components of your code in isolation, you can quickly identify which parts are functioning correctly and which aren’t.
Example of a simple unit test in Python using the `unittest` module:
import unittest
def add_numbers(a, b):
return a + b
class TestAddNumbers(unittest.TestCase):
def test_add_positive_numbers(self):
self.assertEqual(add_numbers(2, 3), 5)
def test_add_negative_numbers(self):
self.assertEqual(add_numbers(-1, -1), -2)
if __name__ == '__main__':
unittest.main()
4.2. Version Control Debugging
Utilizing version control systems like Git can be incredibly helpful in debugging. You can:
- Use `git bisect` to find the commit that introduced a bug
- Compare different versions of your code to identify changes that might have caused issues
- Revert to a previous working state if necessary
4.3. Remote Debugging
For applications running on remote servers or in different environments, remote debugging can be essential. Many IDEs and debuggers support remote debugging, allowing you to debug code running on a different machine as if it were running locally.
4.4. Memory Profiling
For performance issues or memory leaks, memory profiling tools can be invaluable. These tools help you understand how your program is using memory and can identify areas where memory usage is inefficient.
In Python, you can use the `memory_profiler` module:
from memory_profiler import profile
@profile
def my_function():
a = [1] * (10 ** 6)
b = [2] * (2 * 10 ** 7)
del b
return a
if __name__ == '__main__':
my_function()
Running this script with `python -m memory_profiler script.py` will give you a line-by-line analysis of memory usage.
5. Debugging Tools and IDEs
Familiarizing yourself with debugging tools and features in popular IDEs can significantly enhance your debugging capabilities:
- PyCharm: Offers an excellent debugger for Python with features like conditional breakpoints and watch windows.
- Visual Studio Code: Provides debugging support for multiple languages and has a user-friendly interface.
- Eclipse: Popular for Java development, it offers robust debugging features including thread debugging.
- Chrome DevTools: Essential for debugging JavaScript in web applications.
6. Best Practices for Effective Debugging
6.1. Reproduce the Bug
Before you start debugging, ensure you can consistently reproduce the bug. This might involve creating a minimal test case that demonstrates the issue.
6.2. Read the Error Message
Error messages often contain valuable information about what went wrong and where. Take the time to read and understand them thoroughly.
6.3. Check Recent Changes
If a bug suddenly appears, review recent changes to your code. The problem is often in the most recently modified sections.
6.4. Use Assertions
Assertions can help catch bugs early by verifying that certain conditions are met during program execution.
def divide_numbers(a, b):
assert b != 0, "Cannot divide by zero"
return a / b
result = divide_numbers(10, 0) # This will raise an AssertionError
6.5. Keep a Debugging Log
Maintain a log of your debugging process, including hypotheses, tests performed, and results. This can help you track your progress and avoid repeating steps.
6.6. Take Breaks
Debugging can be mentally taxing. Taking regular breaks can help you maintain focus and often leads to fresh insights when you return to the problem.
7. Debugging in Different Programming Paradigms
7.1. Object-Oriented Programming (OOP)
When debugging OOP code, pay special attention to:
- Object state and how it changes over time
- Inheritance hierarchies and method overriding
- Proper encapsulation and access control
7.2. Functional Programming
In functional programming, focus on:
- Ensuring functions are pure (no side effects)
- Properly handling immutable data structures
- Correct application of higher-order functions
7.3. Asynchronous Programming
Debugging asynchronous code can be challenging. Key areas to focus on include:
- Proper handling of promises or async/await
- Race conditions and timing issues
- Callback hell and proper error propagation
8. Debugging in Different Environments
8.1. Web Development
For web development, familiarize yourself with:
- Browser Developer Tools for frontend debugging
- Network tab for API and request/response debugging
- Console logging for JavaScript debugging
8.2. Mobile Development
Mobile app debugging often involves:
- Using emulators or physical devices for testing
- Platform-specific debugging tools (e.g., Xcode for iOS, Android Studio for Android)
- Remote debugging for web-based mobile apps
8.3. Server-Side Debugging
When debugging server-side applications:
- Use logging extensively
- Implement proper error handling and reporting
- Utilize server monitoring tools for performance debugging
9. Advanced Topics in Debugging
9.1. Debugging Multithreaded Applications
Multithreaded applications introduce additional complexity in debugging. Key considerations include:
- Race conditions and deadlocks
- Thread-safe data structures
- Using thread-aware debuggers
9.2. Debugging Distributed Systems
Debugging distributed systems requires:
- Understanding of network protocols and communication patterns
- Distributed tracing tools
- Analyzing system-wide logs and metrics
9.3. Performance Debugging
When debugging performance issues:
- Use profiling tools to identify bottlenecks
- Analyze time complexity of algorithms
- Consider caching and optimization techniques
10. Continuous Improvement in Debugging Skills
Becoming a pro at debugging is an ongoing process. Here are some ways to continuously improve your skills:
- Practice Regularly: Take on challenging coding problems and debug your solutions.
- Learn from Others: Participate in code reviews and pair programming sessions.
- Stay Updated: Keep up with new debugging tools and techniques in your programming language and environment.
- Contribute to Open Source: Debugging issues in large, complex projects can provide valuable experience.
- Teach Others: Explaining debugging concepts to others can deepen your own understanding.
Conclusion
Debugging is both an art and a science. It requires patience, analytical thinking, and a systematic approach. By mastering the techniques and tools discussed in this guide, you’ll be well on your way to debugging like a pro. Remember, every bug you encounter is an opportunity to learn and improve your skills. Happy debugging!