How to Debug Code During a Coding Interview: A Comprehensive Guide
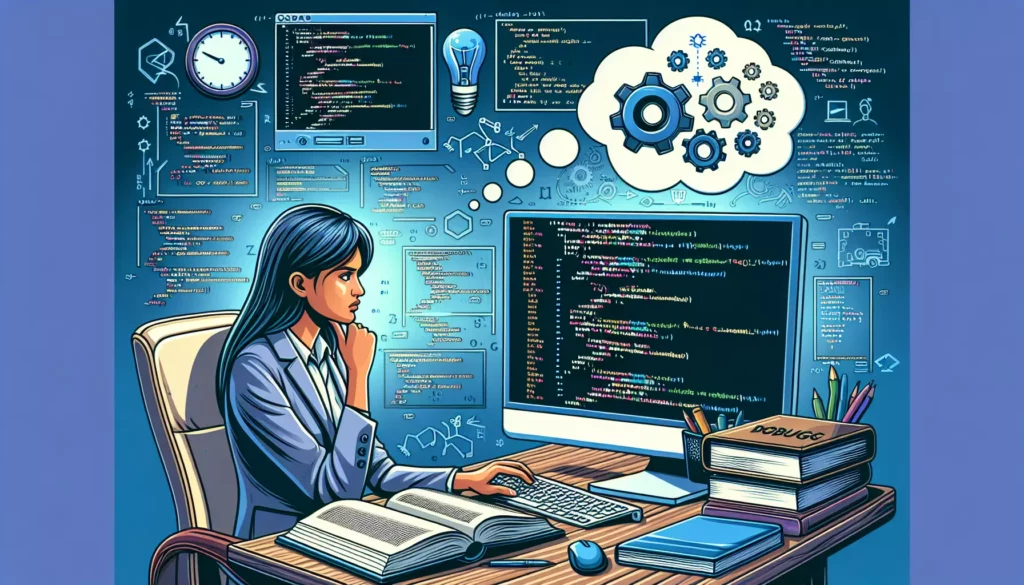
Debugging code is an essential skill for any programmer, and it becomes even more critical during a coding interview. The ability to quickly identify and fix errors in your code can make the difference between success and failure in these high-pressure situations. In this comprehensive guide, we’ll explore effective strategies and techniques for debugging code during a coding interview, helping you approach this challenge with confidence and precision.
1. Understanding the Importance of Debugging in Coding Interviews
Before we dive into specific debugging techniques, it’s crucial to understand why debugging skills are so valued in coding interviews:
- Problem-solving abilities: Debugging showcases your analytical thinking and ability to troubleshoot complex issues.
- Attention to detail: Identifying and fixing bugs demonstrates your meticulousness and code quality standards.
- Real-world simulation: Debugging during an interview mimics real-world scenarios where developers often spend significant time fixing issues.
- Communication skills: Explaining your debugging process allows interviewers to assess your ability to articulate technical concepts clearly.
2. Preparing for Debugging Scenarios
To excel at debugging during a coding interview, preparation is key. Here are some steps you can take to sharpen your debugging skills:
2.1. Practice Debugging Exercises
Regularly engage in debugging exercises to familiarize yourself with common error types and their solutions. Platforms like AlgoCademy offer interactive coding challenges that include debugging scenarios, allowing you to hone your skills in a realistic environment.
2.2. Study Common Error Types
Familiarize yourself with common error types in your preferred programming language. These may include:
- Syntax errors
- Runtime errors
- Logical errors
- Type errors
- Off-by-one errors
2.3. Learn Your IDE’s Debugging Tools
Most Integrated Development Environments (IDEs) come with powerful debugging tools. Learn how to use features like breakpoints, step-through execution, and variable inspection in your preferred IDE.
3. Debugging Techniques for Coding Interviews
When faced with a debugging challenge during a coding interview, consider employing the following techniques:
3.1. Read the Error Message Carefully
Error messages often provide valuable information about the nature and location of the problem. Take the time to read and understand the error message before attempting to fix the issue.
3.2. Use Print Statements Strategically
Inserting print statements at key points in your code can help you track the flow of execution and identify where things go wrong. For example:
def calculate_sum(numbers):
print("Entering calculate_sum function")
total = 0
for num in numbers:
print(f"Current number: {num}, Total: {total}")
total += num
print(f"Final total: {total}")
return total
result = calculate_sum([1, 2, 3, 4, 5])
print(f"Result: {result}")
3.3. Employ the Divide and Conquer Approach
If you’re dealing with a complex function or algorithm, try breaking it down into smaller parts and testing each part individually. This can help isolate the source of the problem more quickly.
3.4. Use Debugger Tools
If available, make use of debugger tools to step through your code line by line. This allows you to observe the state of variables and the flow of execution in real-time.
3.5. Check for Off-by-One Errors
Off-by-one errors are common in loops and array operations. Always double-check your loop conditions and array indices. For example:
def print_array_elements(arr):
for i in range(len(arr)): # Correct: range(len(arr))
print(arr[i])
# Incorrect: range(len(arr) + 1) would cause an index out of range error
3.6. Verify Input Validation
Ensure that your function handles various input types and edge cases correctly. Consider adding input validation to prevent unexpected behavior:
def calculate_average(numbers):
if not numbers:
return 0 # Handle empty list case
if not all(isinstance(num, (int, float)) for num in numbers):
raise ValueError("All elements must be numbers")
return sum(numbers) / len(numbers)
3.7. Use Rubber Duck Debugging
Explain your code and the problem you’re facing out loud, as if you’re explaining it to someone else (or a rubber duck). This process can often help you spot issues you might have overlooked.
4. Common Debugging Pitfalls to Avoid
When debugging during a coding interview, be aware of these common pitfalls:
4.1. Assuming the Problem is Complicated
Often, bugs are caused by simple oversights. Don’t immediately assume the issue is complex; start by checking for simple errors like typos or incorrect variable names.
4.2. Ignoring Warning Messages
Pay attention to warning messages, even if your code seems to run correctly. Warnings can indicate potential issues that may cause problems later.
4.3. Overcomplicating the Solution
When fixing a bug, aim for the simplest solution that addresses the problem. Overengineering can introduce new bugs and make your code harder to understand.
4.4. Not Testing After Fixes
Always test your code after making changes to ensure the bug is truly fixed and that you haven’t introduced new issues.
5. Communicating Your Debugging Process
During a coding interview, it’s not just about fixing the bug; it’s also about demonstrating your problem-solving process. Here’s how to effectively communicate your debugging approach:
5.1. Think Aloud
Verbalize your thought process as you debug. This gives the interviewer insight into your problem-solving approach and allows them to provide hints if needed.
5.2. Explain Your Reasoning
When you make changes to the code, explain why you’re making those specific changes and what you expect them to accomplish.
5.3. Ask Clarifying Questions
If you’re unsure about any aspect of the problem or the expected behavior, don’t hesitate to ask for clarification. This shows that you’re thorough and committed to understanding the problem fully.
5.4. Discuss Alternative Approaches
If you consider multiple ways to fix a bug, briefly discuss the pros and cons of each approach. This demonstrates your ability to evaluate different solutions.
6. Advanced Debugging Techniques
As you become more comfortable with basic debugging, consider incorporating these advanced techniques into your skillset:
6.1. Binary Search Debugging
For bugs that are hard to locate, use a binary search approach. Comment out half of your code and see if the bug persists. Keep narrowing down the problematic section by halving the search area each time.
6.2. Log-Based Debugging
Implement a simple logging system to track the state of your program at various points. This can be particularly useful for asynchronous or time-dependent bugs:
import logging
logging.basicConfig(level=logging.DEBUG)
def complex_function(x, y):
logging.debug(f"Entering complex_function with x={x}, y={y}")
result = x * y
logging.debug(f"Result calculated: {result}")
return result
complex_function(5, 7)
6.3. Exception Handling for Debugging
Use try-except blocks to catch and handle exceptions, providing more information about where and why errors occur:
def divide_numbers(a, b):
try:
result = a / b
return result
except ZeroDivisionError:
print("Error: Division by zero")
return None
except TypeError:
print("Error: Invalid type for division")
return None
print(divide_numbers(10, 0)) # Handles ZeroDivisionError
print(divide_numbers(10, "2")) # Handles TypeError
6.4. Using Assertions
Incorporate assertions to check for conditions that should always be true in your code. This can help catch logical errors early:
def calculate_rectangle_area(length, width):
assert length > 0 and width > 0, "Length and width must be positive"
return length * width
print(calculate_rectangle_area(5, 3)) # Works correctly
print(calculate_rectangle_area(-1, 3)) # Raises an AssertionError
7. Debugging Different Types of Errors
Different types of errors require different debugging approaches. Here’s how to tackle some common error types:
7.1. Syntax Errors
Syntax errors are usually the easiest to fix, as they’re caught by the compiler or interpreter. Pay attention to error messages, which often point directly to the line causing the issue.
7.2. Runtime Errors
Runtime errors occur during program execution. Use try-except blocks to catch these errors and provide more informative error messages:
def access_list_element(lst, index):
try:
return lst[index]
except IndexError:
print(f"Error: Index {index} is out of range for list of length {len(lst)}")
return None
my_list = [1, 2, 3]
print(access_list_element(my_list, 5)) # Handles IndexError
7.3. Logical Errors
Logical errors are the trickiest to debug as the program runs without crashing but produces incorrect results. Use print statements or logging to track the program’s state and identify where it deviates from expected behavior.
7.4. Infinite Loops
To debug infinite loops, add a counter or a timeout mechanism to force the loop to exit after a certain number of iterations or time:
import time
def potentially_infinite_loop(max_iterations=1000, timeout=5):
start_time = time.time()
iterations = 0
while True:
# Your loop logic here
iterations += 1
if iterations >= max_iterations:
print(f"Loop exited after {iterations} iterations")
break
if time.time() - start_time > timeout:
print(f"Loop timed out after {timeout} seconds")
break
potentially_infinite_loop()
8. Debugging Tools and Resources
Familiarize yourself with these debugging tools and resources to enhance your debugging capabilities:
8.1. IDE Debuggers
Most modern IDEs come with built-in debuggers. Learn to use features like:
- Breakpoints
- Step-through execution
- Variable watches
- Call stack inspection
8.2. Online Debugging Tools
Utilize online tools for quick debugging and code sharing:
- Python Tutor: Visualize code execution step-by-step
- JSFiddle: Debug and share JavaScript, HTML, and CSS code
- GDB Online: Online GDB compiler and debugger
8.3. Version Control Systems
Use version control systems like Git to track changes and revert to previous working versions if needed.
8.4. Documentation and Community Resources
Consult language documentation and community forums for insights into common bugs and their solutions:
- Stack Overflow
- Language-specific documentation (e.g., Python docs, MDN for JavaScript)
- GitHub issue trackers for libraries and frameworks
9. Practice Exercises for Debugging
To improve your debugging skills, try these practice exercises:
9.1. Find the Bug
Identify and fix the bug in the following code:
def fibonacci(n):
if n <= 1:
return n
else:
return fibonacci(n-1) + fibonacci(n-2)
print(fibonacci(10)) # Expected output: 55
print(fibonacci(0)) # Expected output: 0
9.2. Debug the Sorting Algorithm
Find and fix the issue in this bubble sort implementation:
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n-i-1):
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
return arr
print(bubble_sort([64, 34, 25, 12, 22, 11, 90]))
9.3. Fix the Recursive Function
Debug the following recursive function to correctly calculate the factorial:
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n)
print(factorial(5)) # Expected output: 120
print(factorial(0)) # Expected output: 1
10. Conclusion
Debugging is an essential skill for any programmer, and it’s particularly crucial during coding interviews. By mastering the techniques and strategies outlined in this guide, you’ll be well-equipped to tackle debugging challenges with confidence and efficiency.
Remember that debugging is not just about fixing errors; it’s about understanding how your code works, identifying potential issues, and implementing robust solutions. As you continue to practice and refine your debugging skills, you’ll find that you become a more effective problem-solver and a more valuable asset to any development team.
Keep in mind that platforms like AlgoCademy offer a wealth of resources and practice problems to help you hone your debugging skills in preparation for coding interviews. By regularly engaging with debugging exercises and staying up-to-date with best practices, you’ll be well-prepared to showcase your problem-solving abilities and technical expertise during your next coding interview.