How to Debug Code and Fix Errors Efficiently: A Comprehensive Guide
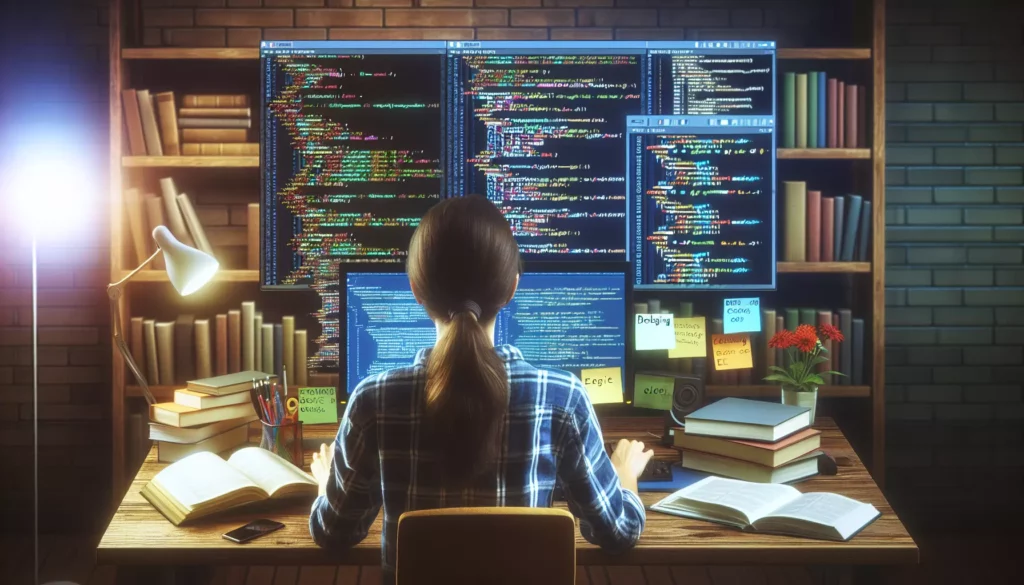
Debugging is an essential skill for every programmer, regardless of their experience level. It’s the process of identifying, isolating, and fixing errors or bugs in your code. Efficient debugging can save you countless hours of frustration and help you become a more proficient developer. In this comprehensive guide, we’ll explore various techniques, tools, and best practices to help you debug code and fix errors efficiently.
Table of Contents
- Understanding Debugging
- Common Types of Errors
- Debugging Techniques
- Debugging Tools
- Best Practices for Efficient Debugging
- Advanced Debugging Techniques
- Debugging in Specific Programming Languages
- Debugging Frameworks and Libraries
- Debugging in Real-World Scenarios
- Conclusion
1. Understanding Debugging
Debugging is more than just fixing errors; it’s a systematic approach to problem-solving in programming. It involves identifying the root cause of an issue, understanding its impact, and implementing a solution. Effective debugging requires a combination of analytical thinking, patience, and a deep understanding of the programming language and environment you’re working in.
The debugging process typically involves the following steps:
- Reproducing the error
- Isolating the problem
- Identifying the cause
- Fixing the bug
- Testing the solution
- Documenting the process and solution
By following these steps, you can approach debugging in a structured manner, making it easier to tackle even the most complex issues.
2. Common Types of Errors
Before diving into debugging techniques, it’s essential to understand the different types of errors you might encounter. Here are the most common categories:
Syntax Errors
Syntax errors occur when your code violates the rules of the programming language. These are usually caught by the compiler or interpreter and are relatively easy to fix. Examples include missing semicolons, mismatched parentheses, or incorrect indentation.
Runtime Errors
Runtime errors occur during program execution and can cause the program to crash or behave unexpectedly. These include null pointer exceptions, divide by zero errors, and array index out of bounds errors.
Logical Errors
Logical errors are the most challenging to debug because the program may run without crashing, but produce incorrect results. These errors stem from flaws in the program’s logic or algorithm implementation.
Semantic Errors
Semantic errors occur when the code is syntactically correct but doesn’t make sense in the context of the program. For example, using the wrong variable name or performing an operation on the wrong data type.
3. Debugging Techniques
Now that we understand the types of errors, let’s explore some effective debugging techniques:
Print Debugging
One of the simplest and most widely used debugging techniques is print debugging. This involves adding print statements to your code to display variable values, function calls, or other relevant information. While it’s not the most sophisticated method, it can be very effective for quick troubleshooting.
Example in Python:
def calculate_sum(a, b):
print(f"Calculating sum of {a} and {b}")
result = a + b
print(f"Result: {result}")
return result
total = calculate_sum(5, 3)
print(f"Total: {total}")
Rubber Duck Debugging
This technique involves explaining your code line by line to an inanimate object (like a rubber duck) or a person who may not be familiar with programming. The act of verbalizing your code often helps you spot errors or inconsistencies in your logic.
Divide and Conquer
When dealing with complex code, try to isolate the problem by commenting out sections of code or breaking the program into smaller, testable units. This can help you narrow down the location of the bug.
Binary Search Debugging
For large codebases, use a binary search approach to locate the bug. Start by commenting out half of the code. If the bug persists, it’s in the active half. If not, it’s in the commented-out half. Repeat this process until you isolate the problematic section.
Debugging by Induction
Start with a small, working piece of code and gradually add complexity. This helps you identify at which point the bug is introduced, making it easier to isolate and fix.
4. Debugging Tools
Modern integrated development environments (IDEs) and programming languages offer powerful debugging tools that can significantly streamline the debugging process. Here are some essential tools to master:
Integrated Debuggers
Most IDEs come with built-in debuggers that allow you to set breakpoints, step through code line by line, and inspect variable values in real-time. Popular IDEs with robust debugging capabilities include:
- Visual Studio Code
- PyCharm
- IntelliJ IDEA
- Eclipse
- Xcode
Browser Developer Tools
For web development, browser developer tools are indispensable. They allow you to inspect HTML, CSS, and JavaScript, set breakpoints, and analyze network requests. Chrome DevTools and Firefox Developer Tools are particularly powerful.
Logging Frameworks
Logging frameworks provide a more sophisticated alternative to print debugging. They allow you to log messages at different severity levels and can be easily enabled or disabled. Popular logging frameworks include:
- Log4j (Java)
- Winston (JavaScript)
- Loguru (Python)
Profilers
Profilers help identify performance bottlenecks in your code by measuring execution time and resource usage. They’re particularly useful for optimizing algorithms and improving overall program efficiency.
Memory Analyzers
For languages with manual memory management, memory analyzers help detect memory leaks and optimize memory usage. Tools like Valgrind for C/C++ are invaluable for this purpose.
5. Best Practices for Efficient Debugging
To make your debugging process more efficient, consider adopting these best practices:
Write Testable Code
Design your code with testing in mind. Use modular design principles and write small, focused functions that are easy to test and debug.
Use Version Control
Utilize version control systems like Git to track changes in your code. This allows you to revert to a working state if you introduce new bugs while debugging.
Implement Logging
Incorporate a logging system into your code from the start. This will provide valuable information for debugging, especially in production environments.
Write Unit Tests
Develop a comprehensive suite of unit tests for your code. This helps catch bugs early and makes it easier to isolate issues when they occur.
Use Assertions
Implement assertions in your code to catch unexpected conditions early. This can help prevent subtle bugs from propagating through your program.
Keep Your Development Environment Updated
Ensure your IDE, compiler, and libraries are up to date. Outdated tools may introduce bugs or lack important debugging features.
Document Your Code
Well-documented code is easier to debug. Use clear variable names, add comments to explain complex logic, and maintain up-to-date documentation.
6. Advanced Debugging Techniques
As you become more proficient in debugging, you can explore more advanced techniques to tackle complex issues:
Remote Debugging
Remote debugging allows you to debug code running on a different machine or environment. This is particularly useful for server-side applications or embedded systems.
Time-Travel Debugging
Some advanced debuggers offer time-travel or reverse debugging capabilities, allowing you to step backwards through code execution. This can be incredibly powerful for understanding complex bugs.
Conditional Breakpoints
Set breakpoints that only trigger under specific conditions. This is useful when debugging issues that only occur in certain scenarios.
Dynamic Analysis
Use dynamic analysis tools to detect runtime errors, memory leaks, and performance issues. Tools like Valgrind for C/C++ or the Java Flight Recorder fall into this category.
Static Code Analysis
Employ static code analysis tools to identify potential bugs, security vulnerabilities, and code quality issues before runtime. Tools like SonarQube or ESLint can be integrated into your development workflow.
7. Debugging in Specific Programming Languages
While many debugging principles are universal, each programming language has its own set of tools and best practices. Let’s look at some language-specific debugging tips:
Python Debugging
- Use the built-in
pdb
module for interactive debugging - Leverage the
ipdb
package for an enhanced debugging experience - Utilize the
logging
module for structured logging
Example of using pdb:
import pdb
def complex_function(x, y):
result = x * y
pdb.set_trace() # Debugger will pause here
return result * 2
complex_function(5, 3)
JavaScript Debugging
- Use
console.log()
,console.warn()
, andconsole.error()
for logging - Leverage browser developer tools for client-side debugging
- Use the
debugger
statement to create breakpoints in your code
Example of using the debugger statement:
function calculateTotal(price, quantity) {
let subtotal = price * quantity;
debugger; // Execution will pause here when dev tools are open
let tax = subtotal * 0.08;
return subtotal + tax;
}
calculateTotal(10, 5);
Java Debugging
- Use the Java Debug Wire Protocol (JDWP) for remote debugging
- Leverage JUnit for unit testing and debugging
- Use Java Flight Recorder for performance analysis and debugging
C/C++ Debugging
- Use GDB (GNU Debugger) for comprehensive debugging capabilities
- Leverage Valgrind for memory leak detection and profiling
- Use static analysis tools like Clang Static Analyzer
8. Debugging Frameworks and Libraries
When working with frameworks and libraries, debugging can become more challenging due to the added complexity. Here are some tips for debugging popular frameworks:
React Debugging
- Use the React Developer Tools browser extension
- Leverage the Error Boundaries feature to catch and handle errors
- Use PropTypes for type checking in development
Angular Debugging
- Use the Augury browser extension for debugging Angular applications
- Leverage Angular’s built-in dependency injection for easier testing and debugging
- Use the
ng.probe()
command in the browser console for runtime inspection
Django Debugging
- Use Django Debug Toolbar for detailed request/response information
- Leverage Django’s built-in logging capabilities
- Use Django extensions for additional debugging tools
Spring Boot Debugging
- Use Spring Boot Actuator for monitoring and managing your application
- Leverage Spring Boot DevTools for automatic restarts and live reload
- Use Spring Boot Admin for managing and monitoring Spring Boot applications
9. Debugging in Real-World Scenarios
Real-world debugging often involves complex systems and environments. Here are some strategies for debugging in production and large-scale applications:
Debugging in Production
- Implement comprehensive logging and monitoring
- Use feature flags to control the rollout of new features
- Implement canary releases to detect issues early
- Use distributed tracing for microservices architectures
Debugging Concurrency Issues
- Use thread dump analysis to identify deadlocks
- Leverage specialized tools like Java VisualVM for visualizing thread states
- Implement proper synchronization and use concurrent data structures
Debugging Network Issues
- Use network monitoring tools like Wireshark
- Implement proper error handling and retries for network operations
- Use tools like cURL or Postman for API debugging
Debugging Performance Issues
- Use profiling tools to identify bottlenecks
- Implement caching strategies where appropriate
- Optimize database queries and indexing
- Use load testing tools to simulate high traffic scenarios
10. Conclusion
Debugging is an essential skill that every programmer must master. By understanding different types of errors, employing various debugging techniques, and leveraging the right tools, you can significantly improve your ability to identify and fix issues in your code.
Remember that effective debugging is not just about fixing errors; it’s about understanding your code deeply and continuously improving your programming skills. As you gain experience, you’ll develop an intuition for common pitfalls and learn to write more robust, error-resistant code from the start.
Keep practicing, stay curious, and don’t be afraid to dive deep into your code when debugging. With time and experience, you’ll become more efficient at solving even the most complex programming challenges.
Happy debugging!