How to Create and Use Custom Data Structures in Programming
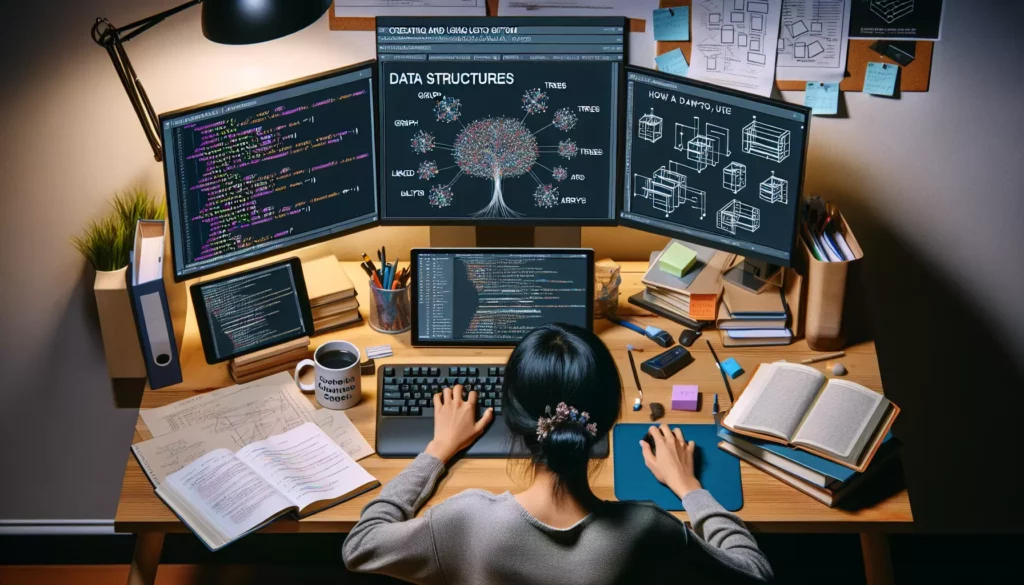
In the world of programming, data structures play a crucial role in organizing and managing information efficiently. While most programming languages come with built-in data structures like arrays, lists, and dictionaries, there are times when you need to create custom data structures tailored to your specific needs. In this comprehensive guide, we’ll explore the process of creating and using custom data structures, providing you with the knowledge and skills to enhance your programming toolkit.
Table of Contents
- Understanding Data Structures
- Benefits of Custom Data Structures
- Planning Your Custom Data Structure
- Implementing Custom Data Structures
- Example: Creating a Linked List
- Using Custom Data Structures
- Optimizing Custom Data Structures
- Testing and Debugging
- Real-World Applications
- Best Practices for Custom Data Structures
- Conclusion
1. Understanding Data Structures
Before diving into creating custom data structures, it’s essential to have a solid understanding of what data structures are and why they’re important in programming.
What are Data Structures?
Data structures are specialized formats for organizing, storing, and managing data in computer programs. They provide efficient ways to access and modify data, allowing developers to create more efficient and scalable applications.
Common Built-in Data Structures
Most programming languages come with several built-in data structures, including:
- Arrays
- Linked Lists
- Stacks
- Queues
- Trees
- Graphs
- Hash Tables
These structures serve various purposes and have different time and space complexities for different operations.
2. Benefits of Custom Data Structures
While built-in data structures cover many use cases, there are several reasons why you might want to create custom data structures:
- Tailored Functionality: Custom data structures can be designed to meet specific requirements of your application.
- Improved Performance: By optimizing for your specific use case, custom structures can outperform generic ones.
- Better Memory Management: Custom structures allow fine-grained control over memory allocation and usage.
- Enhanced Readability: Well-designed custom structures can make your code more intuitive and easier to understand.
- Learning Opportunity: Creating custom data structures deepens your understanding of data organization and algorithmic thinking.
3. Planning Your Custom Data Structure
Before implementing a custom data structure, it’s crucial to plan it thoroughly. Here are the steps to follow:
Define the Purpose
Clearly articulate what problem your custom data structure will solve. What operations should it support? What are the performance requirements?
Choose the Underlying Structure
Decide on the basic building blocks of your structure. Will it be based on arrays, linked nodes, or a combination of existing structures?
Outline the API
Define the methods and properties your data structure will expose. This includes operations for adding, removing, and accessing data.
Consider Edge Cases
Think about potential edge cases and how your structure will handle them. This includes empty states, maximum capacity, and error conditions.
4. Implementing Custom Data Structures
Now that you have a plan, it’s time to implement your custom data structure. Here’s a general approach:
Choose a Programming Language
Select a language that supports the features you need. Object-oriented languages like Java, C++, or Python are often good choices for implementing custom data structures.
Define the Structure
Create a class or struct to represent your data structure. Include the necessary fields to store data and maintain the structure’s state.
Implement Core Methods
Start with the basic operations like adding, removing, and accessing elements. These form the foundation of your data structure.
Add Helper Methods
Implement additional methods to support the core functionality, such as checking if the structure is empty or getting its size.
Optimize and Refine
As you implement, look for opportunities to optimize performance and improve code readability.
5. Example: Creating a Linked List
Let’s walk through an example of creating a custom linked list in Python. This will demonstrate the process of implementing a custom data structure.
Define the Node Class
First, we’ll create a Node class to represent individual elements in our linked list:
class Node:
def __init__(self, data):
self.data = data
self.next = None
Implement the LinkedList Class
Now, let’s create the LinkedList class with basic operations:
class LinkedList:
def __init__(self):
self.head = None
def append(self, data):
new_node = Node(data)
if not self.head:
self.head = new_node
return
current = self.head
while current.next:
current = current.next
current.next = new_node
def prepend(self, data):
new_node = Node(data)
new_node.next = self.head
self.head = new_node
def delete(self, data):
if not self.head:
return
if self.head.data == data:
self.head = self.head.next
return
current = self.head
while current.next:
if current.next.data == data:
current.next = current.next.next
return
current = current.next
def display(self):
elements = []
current = self.head
while current:
elements.append(current.data)
current = current.next
print(" -> ".join(map(str, elements)))
Using the Custom LinkedList
Here’s how you can use the custom LinkedList:
# Create a new linked list
my_list = LinkedList()
# Add elements
my_list.append(1)
my_list.append(2)
my_list.append(3)
my_list.prepend(0)
# Display the list
my_list.display() # Output: 0 -> 1 -> 2 -> 3
# Delete an element
my_list.delete(2)
# Display the updated list
my_list.display() # Output: 0 -> 1 -> 3
This example demonstrates the basic structure and usage of a custom data structure. You can expand on this by adding more methods or optimizing the existing ones.
6. Using Custom Data Structures
Once you’ve created a custom data structure, it’s important to know how to effectively use it in your programs. Here are some tips:
Integration with Existing Code
When introducing a custom data structure into an existing project, ensure it integrates smoothly with the current codebase. This may involve creating adapters or wrappers to make your structure compatible with existing interfaces.
Documentation
Provide clear documentation for your custom data structure. Include information on:
- The purpose and use cases of the structure
- Available methods and their parameters
- Time and space complexity of operations
- Any limitations or specific behaviors to be aware of
Example Usage
Offer example code snippets demonstrating how to use your custom data structure effectively. This helps other developers (or yourself in the future) understand how to leverage the structure’s capabilities.
7. Optimizing Custom Data Structures
Creating an efficient custom data structure often involves iterative optimization. Here are some strategies to improve your custom data structure:
Time Complexity Analysis
Analyze the time complexity of your operations. Look for bottlenecks and consider alternative algorithms or data organization to improve performance.
Space Optimization
Evaluate the memory usage of your structure. Can you reduce the memory footprint without significantly impacting performance?
Lazy Initialization
Consider implementing lazy initialization for resource-intensive components of your structure. This can help improve startup time and reduce memory usage for unused features.
Caching
Implement caching mechanisms for frequently accessed data or computed results to improve overall performance.
Concurrency
If your data structure will be used in a multi-threaded environment, consider implementing thread-safe operations or providing synchronization mechanisms.
8. Testing and Debugging
Thorough testing is crucial when developing custom data structures. Here’s how to approach testing and debugging:
Unit Testing
Create comprehensive unit tests for each method of your data structure. Test both typical use cases and edge cases.
import unittest
class TestLinkedList(unittest.TestCase):
def setUp(self):
self.list = LinkedList()
def test_append(self):
self.list.append(1)
self.assertEqual(self.list.head.data, 1)
def test_prepend(self):
self.list.prepend(1)
self.list.prepend(2)
self.assertEqual(self.list.head.data, 2)
def test_delete(self):
self.list.append(1)
self.list.append(2)
self.list.delete(1)
self.assertEqual(self.list.head.data, 2)
if __name__ == '__main__':
unittest.main()
Performance Testing
Conduct performance tests to ensure your data structure meets the required efficiency standards. This may involve benchmarking against built-in structures or alternative implementations.
Edge Case Testing
Pay special attention to edge cases such as empty structures, maximum capacity, and boundary conditions.
Debugging Techniques
Use debugging tools and techniques specific to your programming language. This may include:
- Print statements for simple debugging
- Integrated Development Environment (IDE) debuggers for step-by-step execution
- Profiling tools to identify performance bottlenecks
9. Real-World Applications
Custom data structures find applications in various domains. Here are some real-world scenarios where custom data structures can be particularly useful:
Graph-based Social Networks
Implementing a custom graph structure to represent social connections, allowing for efficient friend recommendations and relationship analysis.
Game Development
Creating specialized data structures for game state management, collision detection, or AI decision trees.
Financial Systems
Developing custom structures for high-frequency trading algorithms or real-time financial data processing.
Geographic Information Systems (GIS)
Implementing spatial data structures like quadtrees or R-trees for efficient geographic queries.
Text Editors and IDEs
Creating rope data structures or piece tables for efficient text manipulation in large documents.
10. Best Practices for Custom Data Structures
To ensure your custom data structures are effective and maintainable, follow these best practices:
Keep It Simple
Start with a simple implementation and add complexity only when necessary. Overengineering can lead to difficult-to-maintain code.
Follow SOLID Principles
Apply SOLID principles of object-oriented design to create more robust and flexible data structures:
- Single Responsibility Principle
- Open-Closed Principle
- Liskov Substitution Principle
- Interface Segregation Principle
- Dependency Inversion Principle
Use Generic Programming
If your programming language supports generics, use them to create more flexible and reusable data structures.
Implement Standard Interfaces
Implement standard interfaces (e.g., Iterable, Comparable) to make your custom structure more interoperable with existing code and libraries.
Consistent Naming Conventions
Use clear and consistent naming for methods and properties. Follow the conventions of your programming language and development team.
Error Handling
Implement robust error handling and provide informative error messages to help users of your data structure diagnose issues.
Version Control
Use version control systems like Git to track changes to your data structure implementation over time.
Conclusion
Creating and using custom data structures is a powerful skill that can significantly enhance your programming capabilities. By understanding the principles behind data structures, carefully planning your implementation, and following best practices, you can create efficient and tailored solutions for complex programming challenges.
Remember that the process of creating custom data structures is iterative. As you gain more experience and encounter new challenges, you’ll refine your approach and develop more sophisticated structures.
Custom data structures are not just about solving specific problems; they’re about thinking critically about data organization and manipulation. This skill is invaluable in algorithmic thinking and problem-solving, key components of advanced programming and technical interviews.
As you continue your journey in programming, don’t hesitate to experiment with custom data structures. They can be the key to unlocking new levels of efficiency and elegance in your code. Happy coding!