How to Create a Plan of Attack for Any Coding Problem
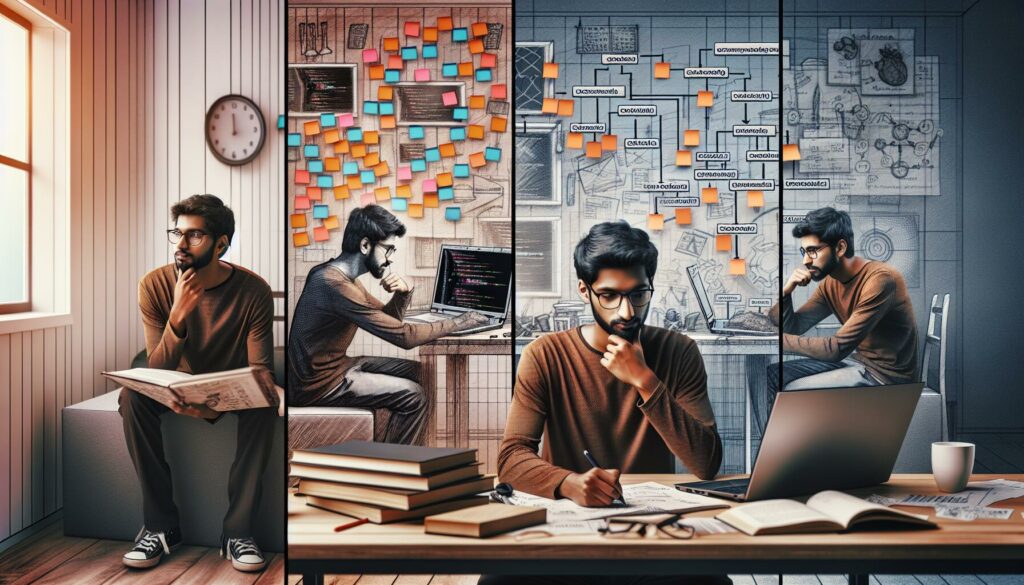
In the world of programming, encountering complex coding problems is a daily occurrence. Whether you’re a beginner just starting your journey or an experienced developer preparing for technical interviews at top tech companies, having a solid plan of attack is crucial. This comprehensive guide will walk you through the process of creating an effective strategy to tackle any coding challenge, helping you improve your problem-solving skills and boost your confidence as a programmer.
1. Understand the Problem
The first and most critical step in solving any coding problem is to thoroughly understand what is being asked. This phase involves several key actions:
1.1. Read the Problem Statement Carefully
Take your time to read the problem statement multiple times. Pay close attention to every detail, as even small nuances can significantly impact your solution approach.
1.2. Identify the Input and Output
Clearly define what inputs the problem provides and what output is expected. This helps in framing the problem and understanding the transformation required.
1.3. Clarify Any Ambiguities
If anything is unclear, don’t hesitate to ask questions or seek clarification. In a real-world scenario or during an interview, this shows your attention to detail and communication skills.
1.4. Consider Edge Cases
Think about potential edge cases or special scenarios that might affect your solution. This could include empty inputs, extremely large values, or unusual data types.
2. Analyze the Problem
Once you have a clear understanding of the problem, it’s time to analyze it more deeply:
2.1. Break Down the Problem
Divide the problem into smaller, manageable sub-problems. This technique, known as “divide and conquer,” makes complex problems more approachable.
2.2. Identify Patterns or Similarities
Look for patterns or similarities to problems you’ve solved before. Recognizing these can help you apply known solutions or algorithms.
2.3. Consider Multiple Approaches
Brainstorm different ways to solve the problem. Don’t settle for the first idea that comes to mind; explore various possibilities.
3. Plan Your Approach
With a thorough analysis complete, it’s time to plan your approach:
3.1. Choose an Algorithm or Data Structure
Based on your analysis, select an appropriate algorithm or data structure. Consider factors like time complexity, space complexity, and the specific requirements of the problem.
3.2. Outline Your Solution
Create a high-level outline of your solution. This can be in the form of pseudocode, flowcharts, or simply a step-by-step written plan.
3.3. Consider Time and Space Complexity
Evaluate the time and space complexity of your proposed solution. If it doesn’t meet the problem’s constraints, revisit your approach.
4. Implement the Solution
With a solid plan in place, it’s time to start coding:
4.1. Write Clean, Modular Code
Implement your solution using clean, well-organized code. Use meaningful variable names and break your code into functions or methods for better readability and maintainability.
4.2. Follow Best Practices
Adhere to coding best practices and style guidelines for the language you’re using. This includes proper indentation, comments where necessary, and following naming conventions.
4.3. Implement Step by Step
Follow your outlined plan, implementing one step at a time. This approach helps manage complexity and makes debugging easier.
5. Test Your Solution
Testing is a crucial part of the problem-solving process:
5.1. Start with Sample Inputs
Begin by testing your solution with the sample inputs provided in the problem statement. This ensures your code works for basic scenarios.
5.2. Test Edge Cases
Move on to testing the edge cases you identified earlier. This helps catch potential issues with your solution.
5.3. Use Additional Test Cases
Create additional test cases to thoroughly validate your solution. Consider using a mix of small and large inputs to test performance.
5.4. Debug and Refine
If you encounter any issues during testing, debug your code and refine your solution as necessary.
6. Optimize Your Solution
Once you have a working solution, consider optimizing it:
6.1. Analyze Time and Space Complexity
Revisit the time and space complexity of your implemented solution. Look for areas where you can improve efficiency.
6.2. Consider Alternative Approaches
Reflect on whether there are alternative approaches that could be more efficient or elegant. Sometimes, a completely different algorithm might yield better results.
6.3. Refactor Your Code
Clean up your code, removing any redundancies or unnecessary complexity. Aim for a solution that is both efficient and readable.
7. Document Your Solution
The final step is to document your solution:
7.1. Add Comments
Include clear, concise comments in your code to explain your thought process and any complex logic.
7.2. Write a Brief Explanation
Prepare a brief explanation of your approach, including why you chose certain algorithms or data structures, and any trade-offs you considered.
7.3. Discuss Complexity
Include a discussion of the time and space complexity of your solution, explaining how you arrived at these conclusions.
Example: Solving the Two Sum Problem
Let’s apply this plan of attack to a common coding problem: the Two Sum problem. This problem asks you to find two numbers in an array that add up to a target sum.
1. Understand the Problem
Problem Statement: Given an array of integers and a target sum, return the indices of two numbers in the array such that they add up to the target.
Input: An array of integers and a target sum
Output: The indices of two numbers that add up to the target
2. Analyze the Problem
We need to find a pair of numbers in the array. This suggests we might need to compare each number with every other number in the array.
3. Plan Your Approach
We could use a nested loop to check every pair of numbers, but this would be inefficient for large arrays. Instead, let’s use a hash map to store the complement of each number we’ve seen.
4. Implement the Solution
Here’s a Python implementation of this approach:
def two_sum(nums, target):
seen = {}
for i, num in enumerate(nums):
complement = target - num
if complement in seen:
return [seen[complement], i]
seen[num] = i
return [] # No solution found
5. Test Your Solution
Let’s test with a sample input:
nums = [2, 7, 11, 15]
target = 9
result = two_sum(nums, target)
print(result) # Should output [0, 1]
6. Optimize Your Solution
Our solution already has a time complexity of O(n) and a space complexity of O(n), which is optimal for this problem.
7. Document Your Solution
Here’s a brief explanation of the approach:
We use a hash map to store the numbers we’ve seen so far, with their indices as values. For each number, we calculate its complement (target – number) and check if it’s in the hash map. If it is, we’ve found our pair and return their indices. If not, we add the current number to the hash map and continue. This approach allows us to solve the problem in a single pass through the array, giving us a time complexity of O(n) and a space complexity of O(n) for the hash map.
Conclusion
Creating a plan of attack for coding problems is a skill that improves with practice. By following these steps – understanding the problem, analyzing it, planning your approach, implementing the solution, testing, optimizing, and documenting – you’ll be well-equipped to tackle any coding challenge that comes your way.
Remember, the key to becoming proficient at problem-solving is consistent practice. Platforms like AlgoCademy offer a wealth of resources, including interactive coding tutorials and AI-powered assistance, to help you hone your skills and prepare for technical interviews at top tech companies.
As you continue to practice, you’ll find that your ability to recognize patterns, choose appropriate data structures and algorithms, and implement efficient solutions will improve significantly. This systematic approach will not only help you in coding interviews but will also make you a more effective problem-solver in your day-to-day work as a programmer.
Keep challenging yourself with diverse problems, analyze your solutions, and learn from your mistakes. With time and dedication, you’ll develop the confidence and skills to approach any coding problem with a clear, effective plan of attack.