How to Come Up with a Solution and Design an Algorithm During a Coding Interview
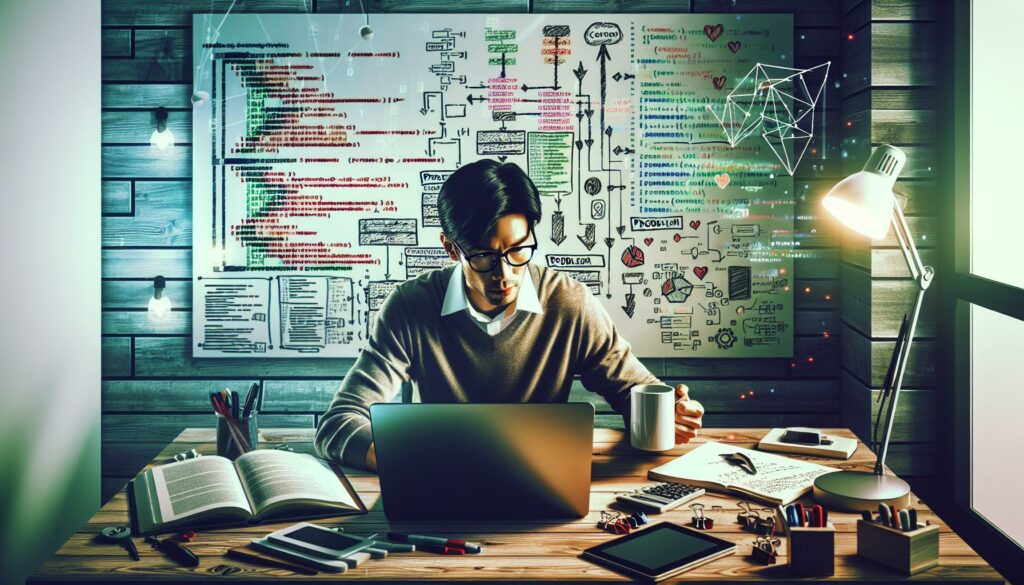
Coding interviews can be nerve-wracking experiences, especially when you’re faced with the challenge of coming up with a solution and designing an algorithm on the spot. However, with the right approach and preparation, you can tackle these challenges with confidence. In this comprehensive guide, we’ll walk you through the process of developing solutions and designing algorithms during coding interviews, providing you with valuable strategies and techniques to help you succeed.
1. Understanding the Problem
Before diving into solution design, it’s crucial to fully understand the problem at hand. This step is often overlooked, but it’s essential for developing an effective algorithm.
1.1. Active Listening and Clarification
When the interviewer presents the problem, listen carefully and take notes if necessary. Don’t hesitate to ask clarifying questions to ensure you understand all aspects of the problem. Some key points to clarify include:
- Input format and constraints
- Expected output format
- Any special conditions or edge cases
- Performance requirements (time and space complexity)
1.2. Repeat the Problem
After the interviewer explains the problem, repeat it back in your own words. This serves two purposes:
- It confirms your understanding of the problem.
- It gives the interviewer a chance to correct any misunderstandings.
1.3. Identify the Core Problem
Try to distill the problem to its essence. What is the fundamental task you need to accomplish? Identifying the core problem can help you recognize similarities to problems you’ve solved before or point you towards relevant algorithms and data structures.
2. Brainstorming and Exploring Approaches
Once you have a clear understanding of the problem, it’s time to start exploring potential solutions.
2.1. Start with a Naive Approach
Begin by considering the simplest, most straightforward solution, even if it’s not the most efficient. This serves several purposes:
- It gives you a starting point for further optimization.
- It demonstrates to the interviewer that you can at least solve the problem.
- It often reveals insights about the problem that can lead to more efficient solutions.
2.2. Consider Multiple Approaches
Don’t settle for the first solution that comes to mind. Try to think of at least two or three different approaches. This shows the interviewer your ability to think critically and consider multiple perspectives.
2.3. Think Aloud
As you brainstorm, vocalize your thoughts. This gives the interviewer insight into your problem-solving process and allows them to provide hints if you’re stuck. It also demonstrates your communication skills, which are crucial in a team environment.
2.4. Use Examples
Work through small examples to test your ideas and gain insights. This can help you identify edge cases and potential pitfalls in your approach.
3. Selecting an Approach
After exploring multiple approaches, it’s time to choose the most promising one to develop further.
3.1. Evaluate Trade-offs
Consider the pros and cons of each approach, focusing on:
- Time complexity
- Space complexity
- Code simplicity and maintainability
- Scalability
3.2. Discuss with the Interviewer
Share your thoughts on the different approaches with the interviewer. They may provide valuable feedback or guide you towards a particular solution.
3.3. Make a Decision
Choose the approach that best balances efficiency and simplicity, given the constraints of the problem.
4. Designing the Algorithm
With an approach selected, it’s time to design your algorithm in more detail.
4.1. Break Down the Problem
Divide the problem into smaller, manageable sub-problems. This makes the overall problem less daunting and allows you to focus on solving one piece at a time.
4.2. Use Pseudocode
Before diving into actual code, write out your algorithm in pseudocode. This allows you to focus on the logic without getting bogged down in syntax details. Here’s an example of how you might write pseudocode for a simple sorting algorithm:
function sortArray(arr):
for i from 0 to length(arr) - 1:
for j from i + 1 to length(arr):
if arr[i] > arr[j]:
swap arr[i] and arr[j]
return arr
4.3. Consider Edge Cases
Think about potential edge cases and how your algorithm will handle them. Common edge cases include:
- Empty input
- Input with a single element
- Very large inputs
- Inputs with duplicate elements
4.4. Optimize
Look for opportunities to optimize your algorithm. This might involve:
- Using more efficient data structures
- Eliminating redundant operations
- Applying specific algorithmic techniques (e.g., two-pointer technique, sliding window)
5. Implementing the Solution
With your algorithm designed, it’s time to implement it in code.
5.1. Choose the Right Data Structures
Select appropriate data structures that align with your algorithm’s needs. Common choices include:
- Arrays and Lists for sequential data
- Hash Tables for fast lookup
- Stacks and Queues for specific processing orders
- Trees and Graphs for hierarchical or networked data
5.2. Write Clean, Readable Code
Even under time pressure, strive to write clean, well-organized code. This includes:
- Using meaningful variable and function names
- Breaking down complex operations into helper functions
- Adding comments to explain non-obvious parts of your code
5.3. Test As You Go
Don’t wait until you’ve written the entire solution to start testing. Test each component as you implement it. This can help catch errors early and demonstrate your testing mindset to the interviewer.
6. Analyzing and Improving Your Solution
Once you have a working solution, take some time to analyze and improve it.
6.1. Analyze Time and Space Complexity
Determine the time and space complexity of your solution. Be prepared to explain your analysis to the interviewer. For example:
function findDuplicate(arr):
seen = {}
for num in arr:
if num in seen:
return num
seen[num] = true
return -1
# Time Complexity: O(n) where n is the length of the array
# Space Complexity: O(n) for the hash table
6.2. Consider Alternative Solutions
If time allows, discuss alternative solutions with the interviewer. This demonstrates your ability to think critically about trade-offs between different approaches.
6.3. Optimize Further
Look for opportunities to further optimize your solution. This might involve:
- Reducing the number of passes through the data
- Using bit manipulation techniques
- Applying mathematical properties to simplify calculations
7. Common Algorithmic Techniques
Familiarizing yourself with common algorithmic techniques can give you a significant advantage in coding interviews. Here are some techniques you should be comfortable with:
7.1. Two Pointer Technique
This technique involves using two pointers to traverse a data structure, often moving at different speeds or in different directions. It’s particularly useful for array and linked list problems.
Example: Finding the middle of a linked list
function findMiddle(head):
slow = head
fast = head
while fast and fast.next:
slow = slow.next
fast = fast.next.next
return slow
# This algorithm uses two pointers: 'slow' moves one step at a time,
# while 'fast' moves two steps. When 'fast' reaches the end,
# 'slow' will be at the middle.
7.2. Sliding Window
The sliding window technique is used to perform operations on a specific window of elements in an array or string. It’s often used to solve substring or subarray problems efficiently.
Example: Finding the maximum sum subarray of size k
function maxSubarraySum(arr, k):
if len(arr) < k:
return None
window_sum = sum(arr[:k])
max_sum = window_sum
for i in range(k, len(arr)):
window_sum = window_sum - arr[i-k] + arr[i]
max_sum = max(max_sum, window_sum)
return max_sum
# This algorithm maintains a window of size k and slides it over the array,
# updating the sum and maximum efficiently.
7.3. Divide and Conquer
This technique involves breaking a problem into smaller subproblems, solving them independently, and then combining the results. It’s the basis for algorithms like merge sort and quick sort.
Example: Merge Sort
function mergeSort(arr):
if len(arr) <= 1:
return arr
mid = len(arr) // 2
left = mergeSort(arr[:mid])
right = mergeSort(arr[mid:])
return merge(left, right)
function merge(left, right):
result = []
i, j = 0, 0
while i < len(left) and j < len(right):
if left[i] <= right[j]:
result.append(left[i])
i += 1
else:
result.append(right[j])
j += 1
result.extend(left[i:])
result.extend(right[j:])
return result
# This algorithm divides the array into halves, sorts them recursively,
# and then merges the sorted halves.
7.4. Dynamic Programming
Dynamic programming is a method for solving complex problems by breaking them down into simpler subproblems. It’s especially useful for optimization problems.
Example: Fibonacci sequence with memoization
function fibonacci(n, memo={}):
if n in memo:
return memo[n]
if n <= 1:
return n
memo[n] = fibonacci(n-1, memo) + fibonacci(n-2, memo)
return memo[n]
# This algorithm uses memoization to store previously computed Fibonacci numbers,
# avoiding redundant calculations and significantly improving efficiency.
7.5. Greedy Algorithms
Greedy algorithms make the locally optimal choice at each step, aiming to find a global optimum. They don’t always produce the best solution, but when they do, they’re often very efficient.
Example: Activity Selection Problem
function activitySelection(start, finish):
n = len(start)
activities = sorted(zip(start, finish), key=lambda x: x[1])
selected = [activities[0]]
last_finish = activities[0][1]
for i in range(1, n):
if activities[i][0] >= last_finish:
selected.append(activities[i])
last_finish = activities[i][1]
return selected
# This algorithm greedily selects activities that finish earliest and don't overlap
# with previously selected activities.
8. Practice and Preparation
Becoming proficient at designing algorithms during coding interviews requires practice and preparation. Here are some tips to help you improve:
8.1. Solve Problems Regularly
Make solving coding problems a regular part of your routine. Platforms like LeetCode, HackerRank, and CodeSignal offer a wide variety of problems to practice with.
8.2. Time Yourself
Practice solving problems under time constraints to simulate interview conditions. This will help you manage your time effectively during actual interviews.
8.3. Review and Learn from Solutions
After solving a problem, take the time to review other solutions. This can expose you to different approaches and help you learn new techniques.
8.4. Mock Interviews
Participate in mock interviews with friends or use platforms that offer mock interview services. This will help you get comfortable with explaining your thought process out loud and coding under observation.
8.5. Study Core Computer Science Concepts
Ensure you have a solid understanding of fundamental data structures and algorithms. This knowledge forms the building blocks for solving more complex problems.
9. Handling Interview Pressure
Even with thorough preparation, the pressure of a coding interview can be challenging. Here are some strategies to help you stay calm and focused:
9.1. Take a Deep Breath
If you feel overwhelmed, take a moment to breathe deeply. This can help calm your nerves and clear your mind.
9.2. Communicate Clearly
Don’t be afraid to think out loud or ask for clarification. Clear communication can help you organize your thoughts and may even prompt the interviewer to provide helpful hints.
9.3. Start with What You Know
If you’re unsure how to approach a problem, start with the parts you do know. This can help build your confidence and may lead you to insights about the overall solution.
9.4. Use the Whiteboard or Text Editor Effectively
Organize your thoughts visually by using diagrams or pseudocode on the whiteboard or in the text editor. This can help you and the interviewer follow your thought process more easily.
9.5. Learn from Mistakes
If you make a mistake or get stuck, don’t panic. Use it as an opportunity to demonstrate your problem-solving skills and ability to learn quickly.
Conclusion
Designing algorithms during a coding interview is a skill that improves with practice and preparation. By understanding the problem thoroughly, exploring multiple approaches, designing your algorithm step-by-step, and implementing it cleanly, you can tackle even challenging problems with confidence.
Remember that the interview process is not just about finding the perfect solution, but also about demonstrating your problem-solving skills, coding ability, and communication skills. Stay calm, think logically, and don’t be afraid to engage with the interviewer throughout the process.
With consistent practice and the strategies outlined in this guide, you’ll be well-equipped to handle algorithm design challenges in your next coding interview. Good luck!