How to Combat the Feeling of Overwhelm When Facing a New Problem
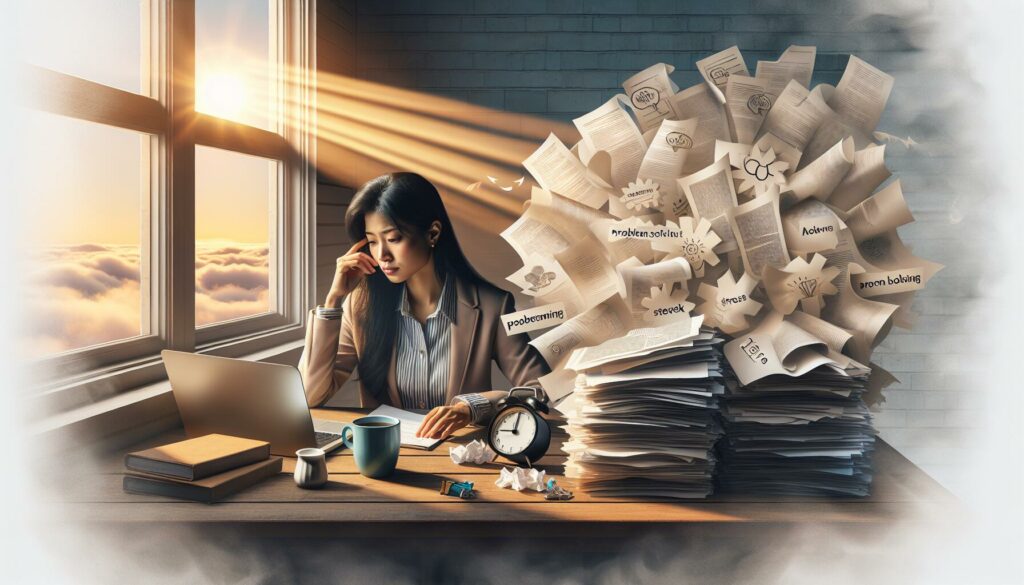
In the world of coding and programming, encountering new problems is a daily occurrence. Whether you’re a beginner just starting your journey or an experienced developer tackling complex algorithms, the feeling of overwhelm can strike at any moment. This sensation can be particularly acute when preparing for technical interviews at major tech companies, often referred to as FAANG (Facebook, Amazon, Apple, Netflix, Google). However, with the right mindset and strategies, you can overcome this overwhelm and approach new problems with confidence. In this comprehensive guide, we’ll explore effective techniques to combat the feeling of overwhelm when facing new coding challenges.
1. Understand the Root of Overwhelm
Before we dive into strategies, it’s crucial to understand why we feel overwhelmed in the first place. Overwhelm often stems from:
- The complexity of the problem
- Fear of failure
- Time pressure
- Lack of confidence in one’s abilities
- Uncertainty about where to start
Recognizing these factors can help you address them more effectively and develop targeted strategies to overcome them.
2. Break Down the Problem
One of the most effective ways to combat overwhelm is to break down the problem into smaller, manageable parts. This technique, often referred to as “divide and conquer,” can make even the most daunting tasks seem more approachable.
Steps to break down a problem:
- Identify the main components of the problem
- Break these components into smaller sub-tasks
- Prioritize the sub-tasks
- Tackle each sub-task one at a time
For example, if you’re faced with implementing a complex sorting algorithm, you might break it down like this:
- Understand the algorithm’s concept
- Implement the basic structure
- Write the main logic
- Handle edge cases
- Optimize for efficiency
- Test and debug
By focusing on one step at a time, you’ll find the overall task much less overwhelming.
3. Start with What You Know
When facing a new problem, it’s easy to get caught up in what you don’t know. Instead, start with what you do know. This approach can help build confidence and provide a solid foundation for tackling the unknown parts of the problem.
Tips for starting with what you know:
- Identify familiar concepts or patterns in the problem
- Write down any relevant information or constraints given in the problem statement
- List out the tools, data structures, or algorithms you think might be useful
- Begin with a simple implementation, even if it’s not the most efficient solution
Remember, every complex problem has simpler components. By starting with what you know, you create a bridge to the unknown parts of the problem.
4. Utilize the Power of Pseudocode
Pseudocode is a powerful tool for combating overwhelm. It allows you to outline your approach without getting bogged down in syntax details. This high-level planning can help you see the big picture and identify potential challenges before you start coding.
Benefits of using pseudocode:
- Clarifies your thinking
- Helps identify logical errors early
- Makes it easier to explain your approach to others
- Provides a roadmap for your actual code implementation
Here’s an example of how you might use pseudocode to plan out a binary search algorithm:
function binarySearch(array, target):
set left to 0
set right to length of array - 1
while left <= right:
calculate mid as (left + right) / 2
if array[mid] equals target:
return mid
else if array[mid] < target:
set left to mid + 1
else:
set right to mid - 1
return -1 // target not found
By outlining your approach in pseudocode, you can focus on the logic without worrying about syntax, making the problem feel more manageable.
5. Embrace the Learning Process
It’s important to remember that feeling overwhelmed is a natural part of the learning process. Embracing this can help you shift your perspective from one of stress to one of opportunity.
Ways to embrace the learning process:
- View challenges as opportunities to grow
- Celebrate small victories along the way
- Keep a learning journal to track your progress
- Share your struggles and successes with peers or mentors
- Remember that even experienced developers face new challenges regularly
By reframing overwhelm as a sign of growth, you can approach new problems with a more positive mindset.
6. Utilize Available Resources
In the age of information, there’s no need to face problems alone. Utilizing available resources can significantly reduce feelings of overwhelm and provide valuable guidance.
Resources to consider:
- Online coding platforms like AlgoCademy
- Documentation and official guides
- Stack Overflow and other Q&A forums
- Coding communities and forums
- Textbooks and online courses
- Mentors or more experienced peers
Remember, using resources isn’t cheating—it’s an essential skill for any programmer. The key is to use these resources to understand the problem better, not just to find a quick solution.
7. Practice Time Management
Effective time management can significantly reduce feelings of overwhelm. By allocating your time wisely, you can ensure that you’re making steady progress without burning out.
Time management techniques:
- Use the Pomodoro Technique: Work for 25 minutes, then take a 5-minute break
- Set realistic goals for each coding session
- Use time-blocking to allocate specific periods for different tasks
- Take regular breaks to prevent mental fatigue
- Use a timer when working on particularly challenging problems to avoid getting stuck
Remember, the goal is not to work non-stop, but to work efficiently and sustainably.
8. Develop a Problem-Solving Framework
Having a structured approach to problem-solving can make new challenges feel less daunting. Develop a framework that you can apply to any problem you encounter.
A sample problem-solving framework:
- Understand the problem: Read the problem statement carefully and clarify any ambiguities
- Plan your approach: Break down the problem and outline your solution
- Write pseudocode: Sketch out your algorithm in plain language
- Implement your solution: Translate your pseudocode into actual code
- Test and debug: Run your code with various inputs and fix any issues
- Optimize: Look for ways to improve your solution’s efficiency
- Reflect: Consider what you learned and how you can apply it to future problems
By following a consistent framework, you create a familiar structure even when facing unfamiliar problems.
9. Practice, Practice, Practice
The more you expose yourself to new problems, the less overwhelming they become. Regular practice not only improves your coding skills but also builds your confidence in tackling unfamiliar challenges.
Ways to incorporate regular practice:
- Solve a coding problem every day
- Participate in coding challenges or competitions
- Work on personal projects that push your boundaries
- Contribute to open-source projects
- Review and refactor old code to reinforce concepts
Remember, the goal of practice is not just to solve problems, but to become comfortable with the problem-solving process itself.
10. Cultivate a Growth Mindset
Lastly, cultivating a growth mindset can significantly impact how you approach new challenges. A growth mindset is the belief that abilities can be developed through dedication and hard work.
Ways to cultivate a growth mindset:
- Embrace challenges as opportunities to learn
- View effort as the path to mastery
- Learn from criticism and feedback
- Find inspiration in the success of others
- Use the word “yet” when facing difficulties (e.g., “I don’t understand this yet”)
With a growth mindset, you’ll be better equipped to handle the inevitable challenges and setbacks that come with learning to code.
Conclusion
Feeling overwhelmed when facing new coding problems is a common experience, even for seasoned developers. However, by implementing these strategies—breaking down problems, starting with what you know, using pseudocode, embracing the learning process, utilizing resources, managing your time effectively, developing a problem-solving framework, practicing regularly, and cultivating a growth mindset—you can transform overwhelm into opportunity.
Remember, every complex algorithm or system you admire was once a daunting challenge for its creators. By persistently applying these techniques and maintaining a positive attitude, you’ll not only overcome the feeling of overwhelm but also develop the resilience and problem-solving skills that are crucial for success in the world of programming.
As you continue your coding journey, whether you’re preparing for technical interviews at FAANG companies or working on personal projects, embrace each new problem as a chance to grow. With time and practice, what once seemed overwhelming will become an exciting challenge, propelling you forward in your programming career.