How to Code Like a Craftsman: Focus on Detail and Quality
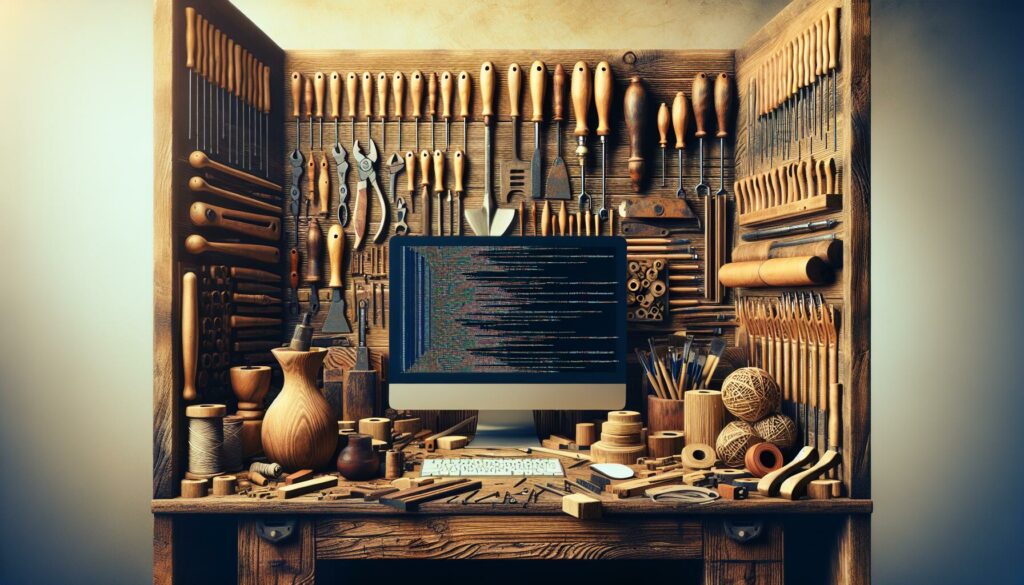
In the world of software development, writing code is more than just a technical skill—it’s an art form. The best programmers approach their craft with the same dedication and attention to detail as a master artisan. This mindset, often referred to as “coding like a craftsman,” emphasizes producing high-quality, well-structured, and maintainable code. In this comprehensive guide, we’ll explore the principles and practices that will help you elevate your coding skills and approach programming with the mindset of a true craftsman.
Understanding the Craftsman Mindset
Before diving into specific techniques, it’s essential to understand what it means to code like a craftsman. The craftsman mindset in programming involves:
- Taking pride in your work
- Striving for excellence in every line of code
- Continuously learning and improving your skills
- Paying attention to the smallest details
- Focusing on long-term maintainability and scalability
- Considering the impact of your code on the entire system
With this mindset as our foundation, let’s explore the key practices that will help you code like a craftsman.
1. Write Clean and Readable Code
Clean code is the hallmark of a skilled craftsman. It’s not just about making your code work; it’s about making it easy to understand and maintain. Here are some tips for writing clean code:
Use Meaningful Names
Choose descriptive and intention-revealing names for variables, functions, and classes. Avoid abbreviations and single-letter names unless they’re widely understood conventions.
// Poor naming
int d; // What does 'd' represent?
// Good naming
int daysUntilDeadline;
Keep Functions Small and Focused
Each function should do one thing and do it well. If a function is doing too much, break it down into smaller, more focused functions.
// Function that does too much
function processUserData(user) {
// Validate user input
// Update database
// Send confirmation email
// Log activity
}
// Better approach: separate concerns
function validateUserInput(user) { /* ... */ }
function updateUserDatabase(user) { /* ... */ }
function sendConfirmationEmail(user) { /* ... */ }
function logUserActivity(user) { /* ... */ }
function processUserData(user) {
validateUserInput(user);
updateUserDatabase(user);
sendConfirmationEmail(user);
logUserActivity(user);
}
Use Consistent Formatting
Adhere to a consistent coding style throughout your project. This includes indentation, bracket placement, and spacing. Many programming languages have established style guides you can follow.
Write Self-Documenting Code
Strive to make your code so clear that it doesn’t require extensive comments to explain what it does. Use expressive method names and clear variable names to make your intentions obvious.
2. Practice Test-Driven Development (TDD)
Test-Driven Development is a practice where you write tests for your code before writing the actual implementation. This approach helps ensure that your code works as intended and makes it easier to refactor and maintain over time.
The TDD Cycle
- Write a failing test that defines a function or improvements of a function
- Write the minimum amount of code to make the test pass
- Refactor the code to improve its structure without changing its behavior
Here’s a simple example of TDD in action:
// Step 1: Write a failing test
test('add function should return the sum of two numbers', () => {
expect(add(2, 3)).toBe(5);
});
// Step 2: Write the minimum code to make the test pass
function add(a, b) {
return a + b;
}
// Step 3: Refactor if necessary (in this case, the function is already simple and clear)
By following TDD, you ensure that your code is testable from the start and that you have a safety net when making changes or adding new features.
3. Embrace Code Reviews
Code reviews are an essential practice for maintaining code quality and fostering a culture of craftsmanship. They provide an opportunity for peer feedback, knowledge sharing, and catching potential issues before they make it into production.
Tips for Effective Code Reviews
- Be open to feedback and see it as an opportunity to improve
- Review code in small chunks to maintain focus and thoroughness
- Look for both technical correctness and adherence to coding standards
- Provide constructive feedback with specific suggestions for improvement
- Use code reviews as a learning opportunity for both the reviewer and the author
Remember, the goal of a code review is not to criticize but to collaborate and improve the overall quality of the codebase.
4. Refactor Regularly
Refactoring is the process of restructuring existing code without changing its external behavior. It’s a crucial practice for maintaining code quality over time and preventing technical debt.
When to Refactor
- When you notice code duplication
- When a function or class becomes too large or complex
- When you find code that’s difficult to understand or maintain
- When you’re adding a new feature that’s similar to existing functionality
- When you’re fixing a bug and realize the code structure contributed to the issue
Refactoring Techniques
Here are some common refactoring techniques:
- Extract Method: Move a code fragment into a separate method
- Rename Method: Change a method name to better reflect its purpose
- Extract Class: Move some behavior from one class to a new class
- Inline Method: Replace a method call with the method’s content
- Replace Conditional with Polymorphism: Replace conditional logic with polymorphic objects
Always ensure you have a good test suite in place before refactoring to catch any unintended changes in behavior.
5. Follow the SOLID Principles
The SOLID principles are a set of five design principles that help create more maintainable, flexible, and scalable software. Understanding and applying these principles is crucial for coding like a craftsman.
Single Responsibility Principle (SRP)
A class should have only one reason to change. In other words, a class should have only one job or responsibility.
// Violates SRP
class User {
constructor(name) {
this.name = name;
}
saveUser() {
// Save user to database
}
sendEmail() {
// Send email to user
}
}
// Follows SRP
class User {
constructor(name) {
this.name = name;
}
}
class UserRepository {
saveUser(user) {
// Save user to database
}
}
class EmailService {
sendEmail(user) {
// Send email to user
}
}
Open-Closed Principle (OCP)
Software entities should be open for extension but closed for modification. This means you should be able to extend a class’s behavior without modifying its existing code.
Liskov Substitution Principle (LSP)
Objects of a superclass should be replaceable with objects of its subclasses without affecting the correctness of the program.
Interface Segregation Principle (ISP)
Clients should not be forced to depend on interfaces they do not use. In other words, it’s better to have many specific interfaces rather than one general-purpose interface.
Dependency Inversion Principle (DIP)
High-level modules should not depend on low-level modules. Both should depend on abstractions. Abstractions should not depend on details. Details should depend on abstractions.
By following these principles, you create more modular, flexible, and maintainable code that’s easier to extend and modify over time.
6. Learn and Apply Design Patterns
Design patterns are reusable solutions to common problems in software design. They provide tested, proven development paradigms that can speed up the development process and make your code more robust and maintainable.
Common Design Patterns
- Singleton: Ensures a class has only one instance and provides a global point of access to it
- Factory Method: Defines an interface for creating an object, but lets subclasses decide which class to instantiate
- Observer: Defines a one-to-many dependency between objects so that when one object changes state, all its dependents are notified and updated automatically
- Strategy: Defines a family of algorithms, encapsulates each one, and makes them interchangeable
- Decorator: Attaches additional responsibilities to an object dynamically
Here’s an example of the Strategy pattern in action:
// Strategy interface
class PaymentStrategy {
pay(amount) {}
}
// Concrete strategies
class CreditCardPayment extends PaymentStrategy {
pay(amount) {
console.log(`Paid ${amount} using Credit Card`);
}
}
class PayPalPayment extends PaymentStrategy {
pay(amount) {
console.log(`Paid ${amount} using PayPal`);
}
}
// Context
class ShoppingCart {
constructor(paymentStrategy) {
this.paymentStrategy = paymentStrategy;
}
checkout(amount) {
this.paymentStrategy.pay(amount);
}
}
// Usage
const cart1 = new ShoppingCart(new CreditCardPayment());
cart1.checkout(100);
const cart2 = new ShoppingCart(new PayPalPayment());
cart2.checkout(200);
By learning and applying design patterns, you can solve complex problems more efficiently and create more flexible and reusable code.
7. Optimize for Performance and Efficiency
While clean and maintainable code is crucial, a true craftsman also considers performance and efficiency. Here are some tips to optimize your code:
Use Appropriate Data Structures
Choose the right data structure for your specific use case. For example, use a hash table for fast lookups, or a binary search tree for maintaining a sorted collection with efficient insertions and deletions.
Minimize Loops and Iterations
Look for opportunities to reduce the number of iterations in your code. Sometimes, you can combine multiple loops or use more efficient algorithms to achieve the same result with fewer operations.
Avoid Premature Optimization
Donald Knuth famously said, “Premature optimization is the root of all evil.” Focus on writing clear, correct code first, then optimize only when necessary and after profiling to identify actual bottlenecks.
Use Caching When Appropriate
For expensive computations or frequently accessed data, consider implementing caching mechanisms to improve performance.
Consider Time and Space Complexity
When designing algorithms, think about both time and space complexity. Sometimes, you may need to trade off one for the other depending on your specific requirements.
8. Document Your Code Effectively
While self-documenting code is ideal, sometimes additional documentation is necessary. Here are some tips for effective documentation:
Write Clear and Concise Comments
Use comments to explain why something is done, not what is done. The code itself should be clear enough to show what it’s doing.
Use Documentation Tools
Utilize documentation generation tools like JSDoc for JavaScript or Sphinx for Python to create comprehensive API documentation.
Keep Documentation Up-to-Date
Outdated documentation is often worse than no documentation. Make it a habit to update documentation whenever you make changes to the code.
Write Meaningful Commit Messages
Your version control commit messages are a form of documentation. Write clear, descriptive commit messages that explain the purpose of each change.
9. Continuously Learn and Improve
The field of software development is constantly evolving, and a true craftsman never stops learning. Here are some ways to keep improving your skills:
- Read books and articles about software development
- Attend conferences and meetups
- Participate in open-source projects
- Practice coding challenges on platforms like AlgoCademy
- Experiment with new languages and technologies
- Seek feedback from more experienced developers
Conclusion
Coding like a craftsman is about more than just writing functional code—it’s about creating software that is clean, efficient, maintainable, and built to last. By focusing on details and quality, following best practices, and continuously improving your skills, you can elevate your coding to the level of craftsmanship.
Remember, becoming a master craftsman is a journey, not a destination. It requires patience, practice, and a commitment to excellence. As you apply these principles and techniques in your daily work, you’ll find that your code becomes more elegant, your solutions more robust, and your skills more refined.
Whether you’re a beginner just starting out or an experienced developer looking to refine your craft, platforms like AlgoCademy can provide valuable resources and challenges to help you on your journey. With interactive coding tutorials, AI-powered assistance, and a focus on algorithmic thinking and problem-solving, AlgoCademy is an excellent tool for honing your skills and preparing for technical interviews at top tech companies.
So, embrace the craftsman mindset, focus on the details, strive for quality in every line of code you write, and watch as your programming skills transform from mere functionality to true artistry. Happy coding!