How to Build Projects That Stand Out to Employers: A Comprehensive Guide
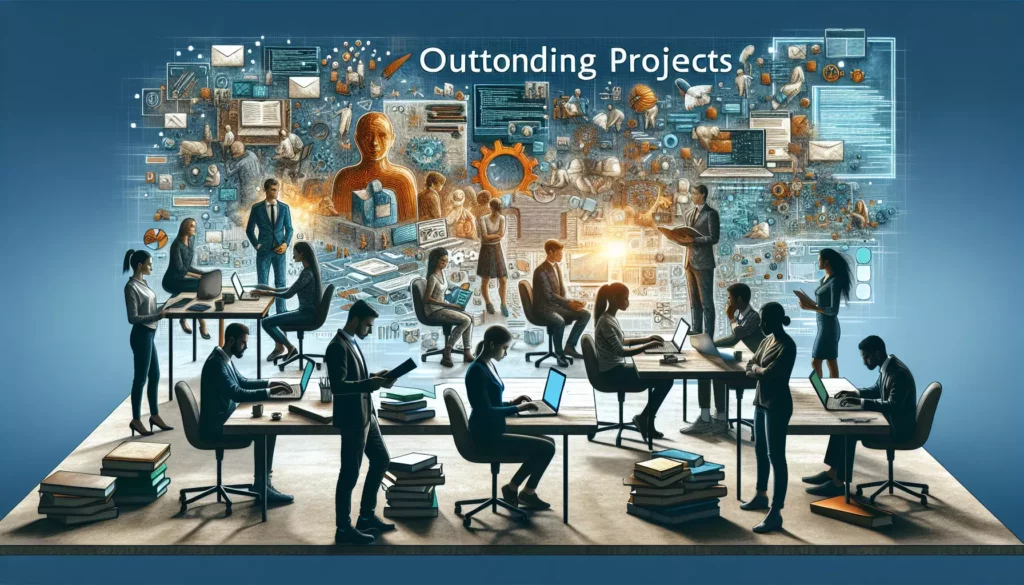
In today’s competitive job market, having a strong portfolio of projects is crucial for aspiring developers and programmers. Employers are increasingly looking beyond just academic qualifications and are placing greater emphasis on practical skills and real-world experience. This is where personal projects come into play. They not only showcase your technical abilities but also demonstrate your passion, creativity, and problem-solving skills. In this comprehensive guide, we’ll explore how to build projects that truly stand out to employers, helping you land your dream job in the tech industry.
1. Understanding the Importance of Personal Projects
Before diving into the specifics of building standout projects, it’s essential to understand why they matter so much to employers:
- Practical Skills Demonstration: Projects show that you can apply your knowledge in real-world scenarios.
- Initiative and Passion: They demonstrate your enthusiasm for coding beyond academic requirements.
- Problem-Solving Abilities: Projects highlight your ability to overcome challenges and find solutions.
- Creativity: They showcase your innovative thinking and unique approaches to problems.
- Continuous Learning: Projects indicate your commitment to staying updated with the latest technologies and practices.
2. Choosing the Right Project Ideas
The first step in building standout projects is selecting the right ideas. Here are some strategies to help you choose:
2.1. Solve Real-World Problems
Look for problems in your daily life or community that could be solved with a software solution. These projects are often the most impressive because they demonstrate your ability to identify needs and create practical solutions.
2.2. Align with Industry Trends
Stay updated with the latest trends in technology and choose projects that incorporate cutting-edge tools and frameworks. This shows employers that you’re forward-thinking and adaptable.
2.3. Showcase Your Interests
Choose projects that align with your personal interests. Your passion will shine through, making the project more engaging for both you and potential employers.
2.4. Consider Scale and Complexity
While it’s great to have a mix of projects, ensure you have at least one or two complex, larger-scale projects that demonstrate your ability to handle substantial codebases and intricate systems.
3. Essential Elements of Standout Projects
To make your projects truly impressive, incorporate these key elements:
3.1. Clean, Well-Documented Code
Write clean, readable, and well-commented code. Use consistent naming conventions and follow best practices for the programming languages and frameworks you’re using. This demonstrates your professionalism and attention to detail.
3.2. Comprehensive README
Create a detailed README file that explains:
- The project’s purpose and functionality
- Technologies and tools used
- Installation and setup instructions
- Usage guidelines
- Future improvements or known issues
3.3. Version Control
Use Git for version control and maintain a clean commit history. This shows your ability to work collaboratively and manage code changes effectively.
3.4. Testing
Implement thorough testing for your projects. This can include unit tests, integration tests, and end-to-end tests. Testing demonstrates your commitment to code quality and reliability.
3.5. Deployment
Deploy your projects so they’re accessible online. This could be through platforms like Heroku, Netlify, or AWS. A live demo is much more impactful than just code on GitHub.
3.6. Responsive Design
For web projects, ensure your applications are responsive and work well on various devices and screen sizes. This shows your attention to user experience and modern web development practices.
4. Leveraging Advanced Technologies and Concepts
To make your projects stand out even more, consider incorporating advanced technologies and concepts:
4.1. Artificial Intelligence and Machine Learning
Integrate AI or ML components into your projects. This could be as simple as a recommendation system or as complex as a natural language processing application. Here’s a simple example of how you might implement a basic sentiment analysis using Python and the NLTK library:
import nltk
from nltk.sentiment import SentimentIntensityAnalyzer
# Download necessary NLTK data
nltk.download('vader_lexicon')
def analyze_sentiment(text):
sia = SentimentIntensityAnalyzer()
sentiment = sia.polarity_scores(text)
if sentiment['compound'] >= 0.05:
return "Positive"
elif sentiment['compound'] <= -0.05:
return "Negative"
else:
return "Neutral"
# Example usage
text = "I love this product! It's amazing and works perfectly."
result = analyze_sentiment(text)
print(f"Sentiment: {result}")
4.2. Microservices Architecture
If you’re building a larger application, consider using a microservices architecture. This demonstrates your understanding of scalable and maintainable system design.
4.3. Blockchain
Incorporate blockchain technology into a project, such as a simple cryptocurrency or a decentralized application (DApp). This shows your ability to work with cutting-edge technologies.
4.4. Cloud Services
Utilize cloud services like AWS, Google Cloud, or Azure in your projects. This could involve using serverless functions, cloud databases, or containerization with services like Docker and Kubernetes.
5. Optimizing Performance and Scalability
Employers are always impressed by projects that demonstrate an understanding of performance optimization and scalability. Here are some areas to focus on:
5.1. Database Optimization
Implement efficient database queries and indexing. Consider using caching mechanisms like Redis for frequently accessed data.
5.2. Asynchronous Programming
Utilize asynchronous programming techniques to improve the responsiveness of your applications. Here’s an example using async/await in JavaScript:
async function fetchData() {
try {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
return data;
} catch (error) {
console.error('Error fetching data:', error);
}
}
async function processData() {
const data = await fetchData();
// Process the data
console.log('Processed data:', data);
}
processData();
5.3. Load Balancing
For web applications, implement load balancing to distribute traffic across multiple servers. This demonstrates your understanding of handling high-traffic scenarios.
5.4. Code Optimization
Optimize your code for performance. This could involve using appropriate data structures, implementing efficient algorithms, and minimizing unnecessary computations.
6. Incorporating Security Best Practices
Security is a critical concern for employers. Showcase your awareness of security best practices in your projects:
6.1. Input Validation
Implement thorough input validation to prevent issues like SQL injection and cross-site scripting (XSS) attacks.
6.2. Authentication and Authorization
Implement secure user authentication and authorization systems. Consider using industry-standard protocols like OAuth 2.0 or JWT (JSON Web Tokens).
6.3. Data Encryption
Use encryption for sensitive data, both in transit and at rest. Here’s a simple example of how you might encrypt and decrypt data using Python’s cryptography library:
from cryptography.fernet import Fernet
def generate_key():
return Fernet.generate_key()
def encrypt_message(message, key):
f = Fernet(key)
encrypted_message = f.encrypt(message.encode())
return encrypted_message
def decrypt_message(encrypted_message, key):
f = Fernet(key)
decrypted_message = f.decrypt(encrypted_message).decode()
return decrypted_message
# Example usage
key = generate_key()
message = "This is a secret message"
encrypted = encrypt_message(message, key)
print("Encrypted:", encrypted)
decrypted = decrypt_message(encrypted, key)
print("Decrypted:", decrypted)
6.4. Regular Security Audits
Conduct regular security audits of your code and dependencies. Use tools like static code analyzers and vulnerability scanners to identify potential security issues.
7. Demonstrating Soft Skills Through Your Projects
While technical skills are crucial, employers also value soft skills. Here’s how you can showcase these through your projects:
7.1. Collaboration
Participate in open-source projects or collaborate with other developers. This demonstrates your ability to work in a team and contribute to larger codebases.
7.2. Communication
Write clear and concise documentation for your projects. This includes not only code comments but also user guides and API documentation if applicable.
7.3. Problem-Solving
Document the challenges you faced during project development and how you overcame them. This could be in the form of a blog post or a section in your project’s README.
7.4. Time Management
Set and meet project milestones. Use project management tools like Trello or GitHub Projects to track your progress and demonstrate your ability to manage tasks effectively.
8. Showcasing Your Projects Effectively
Building great projects is only half the battle. You also need to showcase them effectively to catch employers’ attention:
8.1. Create a Portfolio Website
Develop a personal portfolio website that highlights your best projects. Include screenshots, live demos, and brief descriptions of each project.
8.2. Leverage GitHub
Maintain an active GitHub profile. Pin your best repositories to your profile and ensure each project has a comprehensive README.
8.3. Write Blog Posts
Write detailed blog posts about your projects, explaining the development process, challenges faced, and lessons learned. This demonstrates your ability to communicate technical concepts effectively.
8.4. Create Video Demonstrations
Record short video demonstrations of your projects in action. This can be particularly effective for complex or interactive applications.
9. Continuous Learning and Improvement
The tech industry is constantly evolving, and employers value candidates who demonstrate a commitment to continuous learning. Here’s how you can showcase this:
9.1. Stay Updated with Latest Technologies
Regularly update your projects to use the latest versions of frameworks and libraries. This shows that you’re keeping up with industry trends.
9.2. Refactor and Improve
Continuously refactor and improve your existing projects. This demonstrates your commitment to code quality and your ability to learn from past experiences.
9.3. Learn from Feedback
Seek feedback on your projects from peers or mentors. Implement suggestions and document the improvements you’ve made based on feedback.
9.4. Contribute to Open Source
Contribute to open-source projects related to your field. This not only improves your skills but also demonstrates your ability to work with established codebases and collaborate with other developers.
10. Tailoring Projects to Specific Employers
While having a diverse portfolio is important, you can make your projects even more appealing by tailoring them to specific employers or industries:
10.1. Research the Company
Before applying to a specific company, research their products, technologies, and challenges. Try to build a project that addresses a similar problem or uses similar technologies.
10.2. Industry-Specific Projects
If you’re targeting a specific industry (e.g., finance, healthcare, e-commerce), build projects that demonstrate your understanding of that industry’s unique challenges and requirements.
10.3. Showcase Relevant Skills
If a job listing emphasizes certain skills or technologies, ensure you have projects that prominently feature these elements.
10.4. Solve Company-Specific Challenges
Some companies provide coding challenges or sample problems as part of their application process. Solve these challenges and include them in your portfolio, even if you’re not currently applying to that company.
Conclusion
Building projects that stand out to employers requires a combination of technical skills, creativity, and strategic thinking. By focusing on solving real-world problems, incorporating advanced technologies, and demonstrating both hard and soft skills, you can create a portfolio that truly impresses potential employers.
Remember, the key is not just to build projects, but to build projects that showcase your unique strengths and align with your career goals. Continuously learn, iterate on your projects, and don’t be afraid to take on challenging ideas. With persistence and dedication, you’ll develop a portfolio that not only stands out to employers but also represents your growth and potential as a developer.
As you embark on your project-building journey, keep in mind that every line of code you write is an opportunity to learn and improve. Embrace the challenges, celebrate the successes, and always strive to push your boundaries. Your standout projects are not just a means to impress employers; they’re a testament to your passion for coding and your potential to make a significant impact in the tech industry.