How to Build a Web Application from Scratch: A Comprehensive Guide
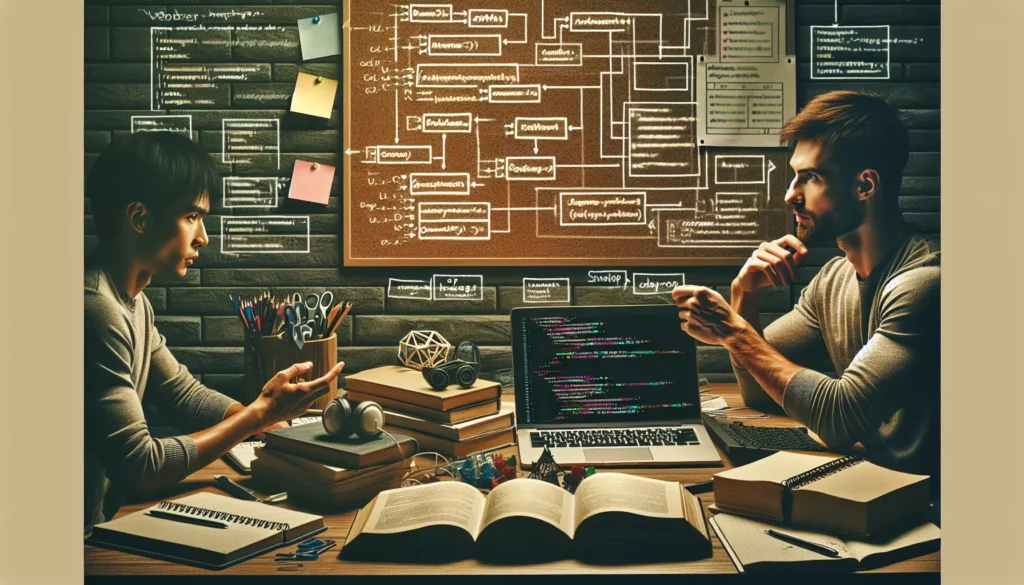
Building a web application from scratch can seem like a daunting task, especially for beginners. However, with the right approach and tools, it can be an exciting and rewarding journey. In this comprehensive guide, we’ll walk you through the process of creating a web application from the ground up, covering everything from planning and design to development and deployment.
Table of Contents
- Planning Your Web Application
- Designing the User Interface
- Building the Frontend
- Developing the Backend
- Setting Up the Database
- Creating APIs
- Implementing User Authentication
- Testing Your Web Application
- Deploying Your Web Application
- Maintaining and Scaling Your Web Application
1. Planning Your Web Application
Before diving into coding, it’s crucial to have a clear plan for your web application. This stage involves defining your project’s goals, target audience, and core features.
Define Your Project Goals
Start by answering these questions:
- What problem does your web application solve?
- Who is your target audience?
- What are the core features of your application?
- What makes your application unique?
Create User Stories
User stories help you understand your application from the user’s perspective. They typically follow this format:
“As a [type of user], I want to [perform an action] so that [achieve a goal].”
Choose Your Tech Stack
Based on your project requirements, choose the technologies you’ll use. A typical stack might include:
- Frontend: HTML, CSS, JavaScript (React, Vue, or Angular)
- Backend: Node.js, Python (Django or Flask), Ruby on Rails, or Java
- Database: MySQL, PostgreSQL, MongoDB, or Firebase
- Hosting: AWS, Google Cloud, Heroku, or DigitalOcean
2. Designing the User Interface
A well-designed user interface (UI) is crucial for user engagement and satisfaction. Here are the steps to design your UI:
Create Wireframes
Wireframes are low-fidelity sketches of your application’s layout. They help you visualize the structure of your pages without getting bogged down in details.
Design Mockups
Mockups are high-fidelity designs that represent the final look of your application. Use tools like Figma, Adobe XD, or Sketch to create detailed mockups.
Develop a Style Guide
A style guide ensures consistency across your application. It should include:
- Color palette
- Typography
- Button styles
- Icon set
- Spacing and layout guidelines
3. Building the Frontend
The frontend is what users interact with directly. It’s responsible for presenting data and capturing user input.
Set Up Your Development Environment
Install the necessary tools:
- Node.js and npm (Node Package Manager)
- A code editor (VS Code, Sublime Text, or WebStorm)
- Git for version control
Choose a Frontend Framework
Popular frontend frameworks include:
- React
- Vue.js
- Angular
For this guide, let’s use React as an example.
Set Up Your React Project
Create a new React project using Create React App:
npx create-react-app my-web-app
cd my-web-app
npm start
Create Components
Break down your UI into reusable components. Here’s an example of a simple React component:
import React from 'react';
function Header() {
return (
<header>
<h1>My Web Application</h1>
<nav>
<ul>
<li><a href="#home">Home</a></li>
<li><a href="#about">About</a></li>
<li><a href="#contact">Contact</a></li>
</ul>
</nav>
</header>
);
}
export default Header;
Implement Routing
Use React Router to handle navigation in your application:
npm install react-router-dom
// In your App.js
import { BrowserRouter as Router, Route, Switch } from 'react-router-dom';
import Home from './components/Home';
import About from './components/About';
import Contact from './components/Contact';
function App() {
return (
<Router>
<Switch>
<Route exact path="/" component={Home} />
<Route path="/about" component={About} />
<Route path="/contact" component={Contact} />
</Switch>
</Router>
);
}
Style Your Components
Use CSS or a CSS-in-JS solution like styled-components to style your components:
npm install styled-components
// In your component file
import styled from 'styled-components';
const Button = styled.button`
background-color: #4CAF50;
border: none;
color: white;
padding: 15px 32px;
text-align: center;
text-decoration: none;
display: inline-block;
font-size: 16px;
margin: 4px 2px;
cursor: pointer;
`;
function MyComponent() {
return <Button>Click me!</Button>;
}
4. Developing the Backend
The backend handles the server-side logic, data processing, and database interactions.
Choose a Backend Framework
Popular backend frameworks include:
- Express.js (Node.js)
- Django (Python)
- Ruby on Rails (Ruby)
- Spring Boot (Java)
For this guide, we’ll use Express.js with Node.js.
Set Up Your Express.js Project
mkdir backend
cd backend
npm init -y
npm install express
Create Your Server
Create a file named server.js
:
const express = require('express');
const app = express();
const port = process.env.PORT || 3000;
app.get('/', (req, res) => {
res.send('Hello World!');
});
app.listen(port, () => {
console.log(`Server running on port ${port}`);
});
Implement Routes
Create routes to handle different HTTP requests:
app.get('/api/users', (req, res) => {
// Logic to fetch users
});
app.post('/api/users', (req, res) => {
// Logic to create a new user
});
app.put('/api/users/:id', (req, res) => {
// Logic to update a user
});
app.delete('/api/users/:id', (req, res) => {
// Logic to delete a user
});
5. Setting Up the Database
Choose a database that suits your application’s needs. For this guide, we’ll use MongoDB, a popular NoSQL database.
Install MongoDB
Follow the installation instructions for your operating system from the official MongoDB website.
Connect to MongoDB
Install the MongoDB driver for Node.js:
npm install mongodb
Connect to MongoDB in your server.js file:
const MongoClient = require('mongodb').MongoClient;
const uri = "mongodb://localhost:27017/myapp";
MongoClient.connect(uri, { useNewUrlParser: true, useUnifiedTopology: true }, (err, client) => {
if (err) {
console.error('Failed to connect to MongoDB:', err);
return;
}
console.log('Connected to MongoDB');
const db = client.db('myapp');
// Use the database in your application
});
Create Database Schema
Define the structure of your data. For example, a user schema might look like this:
const userSchema = {
username: String,
email: String,
password: String,
createdAt: Date
};
6. Creating APIs
APIs (Application Programming Interfaces) allow your frontend to communicate with your backend.
Design RESTful APIs
Follow RESTful principles when designing your APIs. Here’s an example of a user API:
- GET /api/users – Retrieve all users
- GET /api/users/:id – Retrieve a specific user
- POST /api/users – Create a new user
- PUT /api/users/:id – Update a user
- DELETE /api/users/:id – Delete a user
Implement API Endpoints
Create routes in your Express.js application to handle these API requests:
const express = require('express');
const router = express.Router();
router.get('/users', async (req, res) => {
try {
const users = await db.collection('users').find().toArray();
res.json(users);
} catch (error) {
res.status(500).json({ message: error.message });
}
});
router.post('/users', async (req, res) => {
try {
const newUser = await db.collection('users').insertOne(req.body);
res.status(201).json(newUser);
} catch (error) {
res.status(400).json({ message: error.message });
}
});
// Implement other CRUD operations similarly
module.exports = router;
7. Implementing User Authentication
User authentication is crucial for protecting user data and personalizing the user experience.
Choose an Authentication Strategy
Common authentication strategies include:
- Session-based authentication
- Token-based authentication (JWT)
- OAuth
Implement JWT Authentication
Let’s implement JWT (JSON Web Token) authentication:
npm install jsonwebtoken bcryptjs
// In your auth.js file
const jwt = require('jsonwebtoken');
const bcrypt = require('bcryptjs');
async function register(req, res) {
try {
const { username, email, password } = req.body;
const hashedPassword = await bcrypt.hash(password, 10);
const user = await db.collection('users').insertOne({
username,
email,
password: hashedPassword
});
const token = jwt.sign({ id: user.insertedId }, 'your-secret-key', { expiresIn: '1h' });
res.status(201).json({ token });
} catch (error) {
res.status(400).json({ message: error.message });
}
}
async function login(req, res) {
try {
const { email, password } = req.body;
const user = await db.collection('users').findOne({ email });
if (!user || !await bcrypt.compare(password, user.password)) {
return res.status(400).json({ message: 'Invalid credentials' });
}
const token = jwt.sign({ id: user._id }, 'your-secret-key', { expiresIn: '1h' });
res.json({ token });
} catch (error) {
res.status(400).json({ message: error.message });
}
}
module.exports = { register, login };
8. Testing Your Web Application
Testing is crucial to ensure your application works as expected and to catch bugs early.
Unit Testing
Use Jest for unit testing your React components and backend functions:
npm install --save-dev jest
// In your component.test.js file
import React from 'react';
import { render, screen } from '@testing-library/react';
import MyComponent from './MyComponent';
test('renders learn react link', () => {
render(<MyComponent />);
const linkElement = screen.getByText(/learn react/i);
expect(linkElement).toBeInTheDocument();
});
Integration Testing
Use tools like Cypress for end-to-end testing:
npm install --save-dev cypress
// In your cypress/integration/sample_spec.js file
describe('My First Test', () => {
it('Visits the app', () => {
cy.visit('http://localhost:3000')
cy.contains('Learn React').click()
cy.url().should('include', '/learn')
})
})
9. Deploying Your Web Application
Once your application is ready, it’s time to deploy it to a production environment.
Choose a Hosting Provider
Popular hosting options include:
- Heroku
- AWS (Amazon Web Services)
- Google Cloud Platform
- DigitalOcean
Deploy to Heroku
Here’s a basic guide to deploying your application to Heroku:
- Sign up for a Heroku account
- Install the Heroku CLI
- Login to Heroku:
heroku login
- Create a new Heroku app:
heroku create
- Add a Procfile to your project root:
web: node server.js
- Commit your changes:
git add . && git commit -m "Prepare for deployment"
- Push to Heroku:
git push heroku main
10. Maintaining and Scaling Your Web Application
After deployment, you need to maintain and potentially scale your application.
Monitor Performance
Use tools like New Relic or Datadog to monitor your application’s performance and identify bottlenecks.
Implement Caching
Use caching mechanisms like Redis to improve performance:
npm install redis
const redis = require('redis');
const client = redis.createClient();
app.get('/api/users', (req, res) => {
client.get('users', async (err, users) => {
if (err) throw err;
if (users) {
res.json(JSON.parse(users));
} else {
const users = await db.collection('users').find().toArray();
client.setex('users', 3600, JSON.stringify(users));
res.json(users);
}
});
});
Implement Load Balancing
As your application grows, you may need to distribute traffic across multiple servers. Use tools like Nginx or HAProxy for load balancing.
Regularly Update Dependencies
Keep your dependencies up to date to ensure security and performance:
npm update
Conclusion
Building a web application from scratch is a complex but rewarding process. By following this guide, you’ve learned the essential steps: from planning and designing to developing, testing, and deploying your application. Remember, building a great web application is an iterative process. Continuously gather user feedback, monitor performance, and make improvements to create a successful and valuable product.
As you continue your journey in web development, don’t forget to keep learning and staying updated with the latest technologies and best practices. Happy coding!