How to Build a Personal Coding Project That Impresses Interviewers
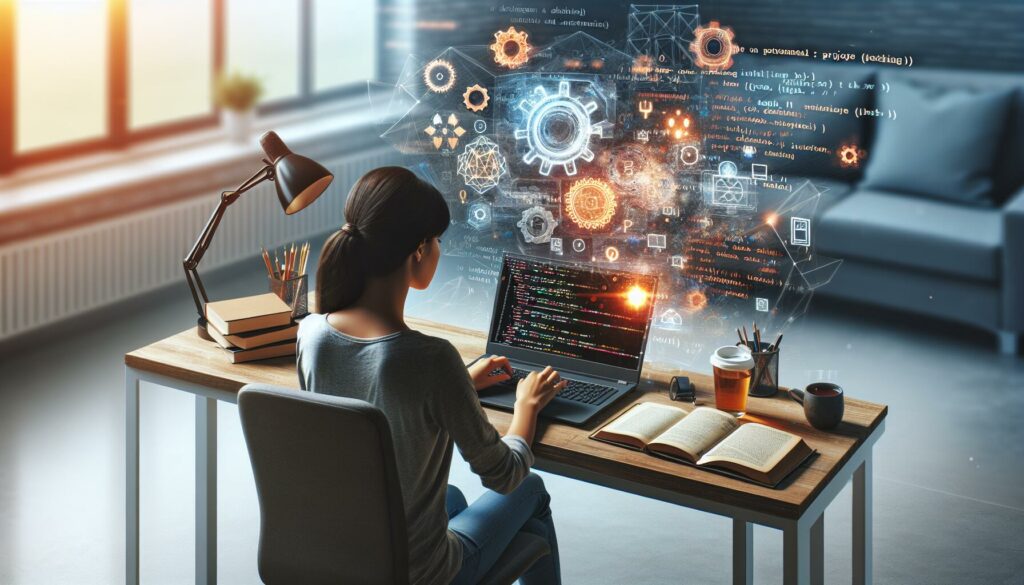
In the competitive world of tech hiring, having a standout personal coding project can be the key to catching an interviewer’s eye and landing your dream job. Whether you’re a seasoned developer or just starting your coding journey, creating a project that showcases your skills, creativity, and problem-solving abilities is crucial. This comprehensive guide will walk you through the process of building a personal coding project that will impress interviewers and help you stand out from the crowd.
1. Choose a Project That Aligns with Your Interests and Career Goals
The first step in creating an impressive personal project is selecting an idea that genuinely excites you. Your passion for the project will shine through during interviews and demonstrate your enthusiasm for coding. Consider the following factors when choosing your project:
- Your target industry or role
- Technologies you want to learn or showcase
- Problems you’d like to solve
- Personal interests or hobbies that could be enhanced with technology
For example, if you’re interested in working for a social media company, you might create a unique social networking app with a twist. If you’re passionate about finance, you could develop a personal budgeting tool with advanced features.
2. Plan Your Project Thoroughly
Before diving into coding, take the time to plan your project meticulously. This step will help you stay organized and demonstrate your ability to think through complex problems. Your project plan should include:
- A clear project description and goals
- User stories or feature requirements
- A basic system architecture or design
- A timeline with milestones
- A list of technologies and tools you’ll use
Consider using project management tools like Trello or Asana to keep track of your progress and showcase your organizational skills to potential employers.
3. Choose the Right Tech Stack
Selecting an appropriate tech stack for your project is crucial. Consider the following factors:
- Relevance to your target industry or role
- Scalability and performance requirements
- Your familiarity with the technologies
- Community support and documentation availability
For example, if you’re building a web application, you might choose a popular stack like MERN (MongoDB, Express.js, React, Node.js) or MEAN (MongoDB, Express.js, Angular, Node.js). If you’re focusing on mobile development, consider using React Native or Flutter for cross-platform apps.
4. Implement Core Functionality First
Start by implementing the core features of your project. This approach allows you to have a working prototype quickly and helps you stay motivated. Follow these steps:
- Set up your development environment
- Create a basic project structure
- Implement the most critical features
- Test thoroughly as you go
For example, if you’re building a task management app, start with basic CRUD (Create, Read, Update, Delete) operations for tasks before adding more advanced features like task prioritization or team collaboration.
5. Focus on Clean, Maintainable Code
Writing clean, well-organized code is essential for impressing interviewers. Follow these best practices:
- Use consistent naming conventions
- Write clear, concise comments
- Follow the DRY (Don’t Repeat Yourself) principle
- Implement proper error handling
- Use version control (e.g., Git) from the start
Here’s an example of clean, well-commented JavaScript code:
// Function to calculate the factorial of a number
function calculateFactorial(n) {
// Check if the input is valid
if (n < 0 || !Number.isInteger(n)) {
throw new Error("Input must be a non-negative integer");
}
// Base case: factorial of 0 or 1 is 1
if (n === 0 || n === 1) {
return 1;
}
// Recursive case: n! = n * (n-1)!
return n * calculateFactorial(n - 1);
}
// Example usage
try {
const result = calculateFactorial(5);
console.log(`Factorial of 5 is: ${result}`);
} catch (error) {
console.error(`Error: ${error.message}`);
}
6. Incorporate Advanced Features and Technologies
To make your project stand out, consider incorporating advanced features or cutting-edge technologies. This demonstrates your ability to learn and apply new concepts. Some ideas include:
- Implementing machine learning algorithms
- Using cloud services (e.g., AWS, Google Cloud, or Azure)
- Integrating with popular APIs
- Implementing real-time features using WebSockets
- Adding voice or image recognition capabilities
For example, if you’re building a recipe app, you could use machine learning to suggest recipes based on a user’s preferences or implement image recognition to identify ingredients from photos.
7. Prioritize User Experience and Design
A great user experience (UX) and appealing design can significantly enhance your project’s impact. Consider the following:
- Create an intuitive user interface
- Implement responsive design for various screen sizes
- Use appropriate color schemes and typography
- Optimize for performance and fast loading times
- Include accessibility features
Tools like Figma or Adobe XD can help you create mockups and prototypes before implementing the design in code.
8. Write Comprehensive Tests
Testing is a crucial aspect of software development that many personal projects overlook. Implementing thorough testing demonstrates your commitment to quality and reliability. Include the following types of tests:
- Unit tests for individual functions and components
- Integration tests for interactions between different parts of your application
- End-to-end tests to simulate user interactions
- Performance tests to ensure your application can handle expected loads
Here’s an example of a simple unit test using Jest for a JavaScript function:
// Function to be tested
function add(a, b) {
return a + b;
}
// Test suite
describe("add function", () => {
test("adds two positive numbers correctly", () => {
expect(add(2, 3)).toBe(5);
});
test("adds a positive and a negative number correctly", () => {
expect(add(5, -3)).toBe(2);
});
test("adds two negative numbers correctly", () => {
expect(add(-2, -4)).toBe(-6);
});
});
9. Document Your Project Thoroughly
Comprehensive documentation is essential for showcasing your project and demonstrating your communication skills. Include the following in your documentation:
- A detailed README file explaining your project’s purpose, features, and setup instructions
- API documentation (if applicable)
- Code comments explaining complex logic or algorithms
- A changelog to track version history and updates
- Troubleshooting guides or FAQs
Consider using tools like Swagger for API documentation or JSDoc for generating documentation from your code comments.
10. Deploy and Host Your Project
Make your project accessible to interviewers by deploying and hosting it online. This step demonstrates your ability to take a project from development to production. Consider the following options:
- Use platforms like Heroku, Netlify, or Vercel for easy deployment
- Set up a continuous integration/continuous deployment (CI/CD) pipeline
- Implement monitoring and logging to track performance and errors
- Secure your application with HTTPS and proper authentication
For example, you could use GitHub Actions to set up a CI/CD pipeline that automatically deploys your application to Heroku whenever you push changes to your main branch.
11. Showcase Your Project Effectively
Once your project is complete, it’s crucial to present it effectively to potential employers. Consider the following strategies:
- Create a polished GitHub repository with a detailed README
- Include screenshots or GIFs demonstrating your project’s functionality
- Record a brief demo video showcasing key features
- Write a blog post or article explaining your development process and lessons learned
- Share your project on relevant social media platforms or forums
12. Prepare to Discuss Your Project in Interviews
Be ready to talk about your project in depth during interviews. Prepare answers to common questions such as:
- What inspired you to create this project?
- What were the biggest challenges you faced, and how did you overcome them?
- How did you decide on the technologies and architecture you used?
- What would you do differently if you were to start the project again?
- How would you scale the project to handle a larger user base?
Practice explaining your project concisely, focusing on the most impressive aspects and the problems you solved.
13. Continuously Improve and Iterate
Even after your initial release, continue to improve and expand your project. This demonstrates your commitment to growth and your ability to maintain and evolve a codebase. Consider the following:
- Regularly update dependencies and address security vulnerabilities
- Implement user feedback and suggestions
- Add new features or optimize existing ones
- Refactor code to improve performance or readability
- Explore integrations with other tools or services
14. Leverage Your Project for Networking
Use your personal project as a networking tool to connect with other developers and potential employers. Consider these strategies:
- Contribute to open-source projects related to the technologies you used
- Attend hackathons or coding events to showcase your project
- Join online communities or forums related to your project’s domain
- Reach out to developers or companies working on similar projects for feedback or collaboration opportunities
15. Reflect on Your Learning Journey
Take time to reflect on what you’ve learned throughout the process of building your project. This reflection can be valuable during interviews and for your personal growth. Consider keeping a development journal or blog to document your progress, challenges, and insights.
Conclusion
Building a personal coding project that impresses interviewers requires careful planning, execution, and presentation. By following the steps outlined in this guide, you’ll be well-equipped to create a project that showcases your skills, creativity, and problem-solving abilities. Remember that the process of building the project is just as important as the final product, as it demonstrates your ability to learn, adapt, and overcome challenges – all valuable traits that employers seek in potential hires.
As you embark on your project, keep in mind that persistence and passion are key. Don’t be afraid to tackle challenging problems or explore new technologies. The more you push yourself, the more impressive your project will be to potential employers. And most importantly, enjoy the process of creation and learning – your enthusiasm will shine through in interviews and set you apart from other candidates.
By creating a standout personal coding project, you’re not just building a portfolio piece; you’re investing in your future career success. So, roll up your sleeves, fire up your favorite IDE, and start coding your way to your dream job in the tech industry!