How to Break Down Complex Problems into Solvable Chunks: A Programmer’s Guide
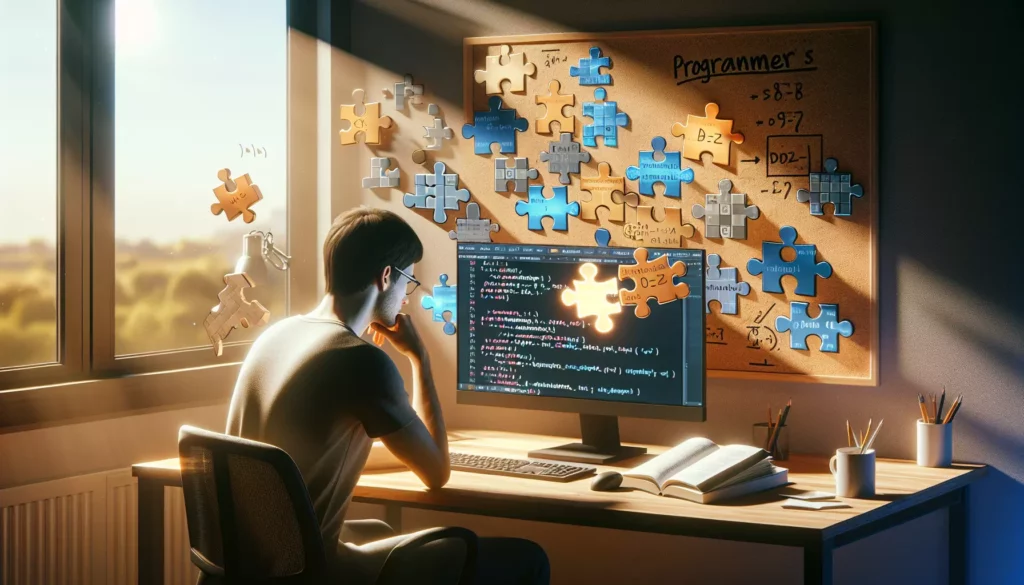
In the world of programming and software development, tackling complex problems is an everyday challenge. Whether you’re a beginner just starting your coding journey or an experienced developer preparing for technical interviews at top tech companies, the ability to break down intricate problems into manageable pieces is an invaluable skill. This article will explore effective strategies for dissecting complex problems, with a focus on algorithmic thinking and problem-solving techniques that are crucial for success in coding interviews and real-world programming scenarios.
Understanding the Importance of Problem Decomposition
Before diving into specific techniques, it’s essential to understand why breaking down complex problems is so crucial in programming:
- Manageability: Large, complex problems can be overwhelming. Breaking them down makes them more approachable and less daunting.
- Focus: Working on smaller chunks allows you to concentrate on specific aspects of the problem without losing sight of the bigger picture.
- Modularity: Decomposed problems often lead to modular code, which is easier to understand, test, and maintain.
- Collaboration: When working in teams, divided tasks can be distributed more effectively among team members.
- Problem-solving practice: Regularly breaking down problems enhances your overall problem-solving skills, which is crucial for technical interviews and professional growth.
Strategies for Breaking Down Complex Problems
1. Understand the Problem Thoroughly
Before attempting to break down a problem, ensure you have a clear understanding of what needs to be solved. This involves:
- Reading the problem statement carefully, multiple times if necessary
- Identifying the inputs and expected outputs
- Recognizing any constraints or special conditions
- Asking clarifying questions (especially important in interview settings)
For example, if you’re tackling a problem like finding the longest palindromic substring in a given string, make sure you understand what constitutes a palindrome, whether the solution needs to handle empty strings or single-character inputs, and if there are any time or space complexity requirements.
2. Identify the Core Components
Once you understand the problem, try to identify its main components or sub-problems. For the palindromic substring problem, you might break it down into:
- A function to check if a given substring is a palindrome
- A method to generate all possible substrings
- A way to keep track of the longest palindrome found
3. Use the Divide and Conquer Approach
The divide and conquer strategy involves breaking a problem into smaller, more manageable sub-problems, solving them independently, and then combining the solutions. This approach is particularly useful for recursive problems and algorithms like merge sort or quick sort.
For instance, when implementing merge sort:
- Divide: Split the array into two halves
- Conquer: Recursively sort the two halves
- Combine: Merge the sorted halves
def merge_sort(arr):
if len(arr) <= 1:
return arr
mid = len(arr) // 2
left = merge_sort(arr[:mid])
right = merge_sort(arr[mid:])
return merge(left, right)
def merge(left, right):
result = []
i, j = 0, 0
while i < len(left) and j < len(right):
if left[i] < right[j]:
result.append(left[i])
i += 1
else:
result.append(right[j])
j += 1
result.extend(left[i:])
result.extend(right[j:])
return result
4. Use Abstraction and Modularization
Abstraction involves hiding complex implementation details behind simpler interfaces. By creating functions or classes that encapsulate specific functionalities, you can work with higher-level concepts and focus on solving one part of the problem at a time.
For example, when implementing a graph algorithm like Dijkstra’s shortest path, you might create separate modules for:
- Graph representation (e.g., adjacency list or matrix)
- Priority queue implementation
- The main Dijkstra algorithm logic
class Graph:
def __init__(self):
self.nodes = {}
def add_edge(self, from_node, to_node, weight):
if from_node not in self.nodes:
self.nodes[from_node] = {}
self.nodes[from_node][to_node] = weight
class PriorityQueue:
# Implementation details...
def dijkstra(graph, start):
# Implementation using Graph and PriorityQueue
# ...
5. Use Pseudocode and Flowcharts
Before diving into actual code, it can be helpful to sketch out your approach using pseudocode or flowcharts. This allows you to focus on the logic and structure of your solution without getting bogged down in syntax details.
Pseudocode for finding the maximum element in an array might look like this:
function find_max(array):
if array is empty:
return null
max_element = first element of array
for each element in array:
if element > max_element:
max_element = element
return max_element
6. Implement Incrementally
Once you have broken down the problem and have a plan, start implementing your solution incrementally. Begin with the simplest sub-problem or component and gradually build up to the full solution. This approach allows you to:
- Test and verify each part of your solution as you go
- Identify and fix issues early in the development process
- Maintain a sense of progress, which can be motivating when dealing with complex problems
For example, when implementing a solution to find all permutations of a string, you might start with:
- Implementing a function to swap two characters in a string
- Creating a helper function to generate permutations for a fixed first character
- Building the main function that uses the helper to generate all permutations
def swap(string, i, j):
chars = list(string)
chars[i], chars[j] = chars[j], chars[i]
return ''.join(chars)
def permute_helper(string, left, right, results):
if left == right:
results.append(string)
else:
for i in range(left, right + 1):
string = swap(string, left, i)
permute_helper(string, left + 1, right, results)
string = swap(string, left, i) # backtrack
def permute(string):
results = []
permute_helper(string, 0, len(string) - 1, results)
return results
Advanced Techniques for Problem Decomposition
1. Dynamic Programming
Dynamic Programming (DP) is a powerful technique for solving complex problems by breaking them down into simpler subproblems. It’s particularly useful for optimization problems and can significantly reduce time complexity in certain scenarios.
Key steps in applying DP:
- Identify if the problem has overlapping subproblems
- Define the recurrence relation
- Decide on a memoization strategy (top-down) or tabulation (bottom-up)
- Implement the solution, often using arrays or matrices to store intermediate results
Example: Fibonacci sequence using DP
def fibonacci(n):
if n <= 1:
return n
dp = [0] * (n + 1)
dp[1] = 1
for i in range(2, n + 1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
2. Greedy Algorithms
Greedy algorithms make locally optimal choices at each step with the hope of finding a global optimum. While not always guaranteed to find the best solution, they can be effective for certain problems and are often simpler to implement than more complex algorithms.
Steps to apply a greedy approach:
- Identify the optimal substructure of the problem
- Determine the greedy choice at each step
- Prove that the greedy choice is safe (i.e., it doesn’t eliminate the optimal solution)
- Implement the solution
Example: Coin change problem (assuming coins of denominations that allow for a greedy approach)
def coin_change(amount, coins):
coins.sort(reverse=True) # Sort coins in descending order
result = []
for coin in coins:
while amount >= coin:
result.append(coin)
amount -= coin
return result if amount == 0 else None # Return None if exact change isn't possible
3. Sliding Window Technique
The sliding window technique is particularly useful for problems involving arrays or strings where you need to find or calculate something among all subarrays or substrings of a certain size. It can often reduce the time complexity from O(n²) to O(n).
Steps to apply the sliding window technique:
- Identify the window size (fixed or variable)
- Compute the result for the first window
- Slide the window by one element and update the result
- Repeat step 3 until the end of the array/string
Example: Finding the maximum sum subarray of size k
def max_subarray_sum(arr, k):
if len(arr) < k:
return None
window_sum = sum(arr[:k])
max_sum = window_sum
for i in range(k, len(arr)):
window_sum = window_sum - arr[i-k] + arr[i]
max_sum = max(max_sum, window_sum)
return max_sum
4. Two-Pointer Technique
The two-pointer technique involves using two pointers to traverse an array or string, often moving in opposite directions or at different speeds. This approach can be particularly effective for problems involving searching, reversing, or finding pairs in sorted arrays.
Common two-pointer patterns:
- Opposite directional: One pointer starts at the beginning, the other at the end
- Same directional: Both pointers move in the same direction, but at different speeds
- Sliding window: A variation where the two pointers define the boundaries of a window
Example: Reversing a string in-place
def reverse_string(s):
left, right = 0, len(s) - 1
while left < right:
s[left], s[right] = s[right], s[left]
left += 1
right -= 1
return s
Problem-Solving in Technical Interviews
When facing complex problems in technical interviews, especially for positions at top tech companies, it’s crucial to approach problem-solving systematically. Here are some tips to help you break down and solve interview problems effectively:
1. Clarify the Problem
Before jumping into a solution, make sure you fully understand the problem:
- Ask questions about input formats, constraints, and edge cases
- Discuss any assumptions you’re making
- Use examples to confirm your understanding
2. Think Aloud
Interviewers are interested in your thought process, not just the final solution. As you break down the problem:
- Verbalize your thoughts and ideas
- Explain why you’re considering certain approaches
- Discuss trade-offs between different solutions
3. Start with a Brute Force Approach
Often, it’s helpful to start with a simple, inefficient solution:
- Demonstrates that you can solve the problem
- Provides a baseline for optimization
- Allows you to start coding while thinking about more efficient solutions
4. Optimize Incrementally
After implementing a basic solution, look for ways to optimize:
- Identify bottlenecks in your current approach
- Consider using appropriate data structures (e.g., hash tables for O(1) lookup)
- Apply relevant algorithms or techniques (e.g., two-pointer, sliding window)
5. Test Your Solution
Before declaring your solution complete:
- Walk through your code with a simple example
- Test edge cases (empty input, single element, maximum values)
- Discuss the time and space complexity of your solution
Conclusion
Breaking down complex problems into solvable chunks is a fundamental skill for programmers and a critical component of success in technical interviews. By understanding various problem-solving strategies, from basic decomposition techniques to advanced algorithmic approaches, you can tackle even the most challenging coding problems with confidence.
Remember, the key to mastering this skill is practice. Regularly engage with diverse programming challenges, analyze different problem-solving approaches, and reflect on your solutions. Platforms like AlgoCademy offer a wealth of resources, including interactive coding tutorials and AI-powered assistance, to help you hone your problem-solving skills and prepare for technical interviews at top tech companies.
As you continue to develop your abilities in breaking down and solving complex problems, you’ll not only improve your chances of success in interviews but also become a more effective and efficient programmer in your professional career. The strategies and techniques discussed in this article provide a solid foundation, but remember that problem-solving is as much an art as it is a science. With time and practice, you’ll develop your own intuition and approach to tackling complex programming challenges.