How to Become a Data Scientist from a Programming Background: A Comprehensive Guide
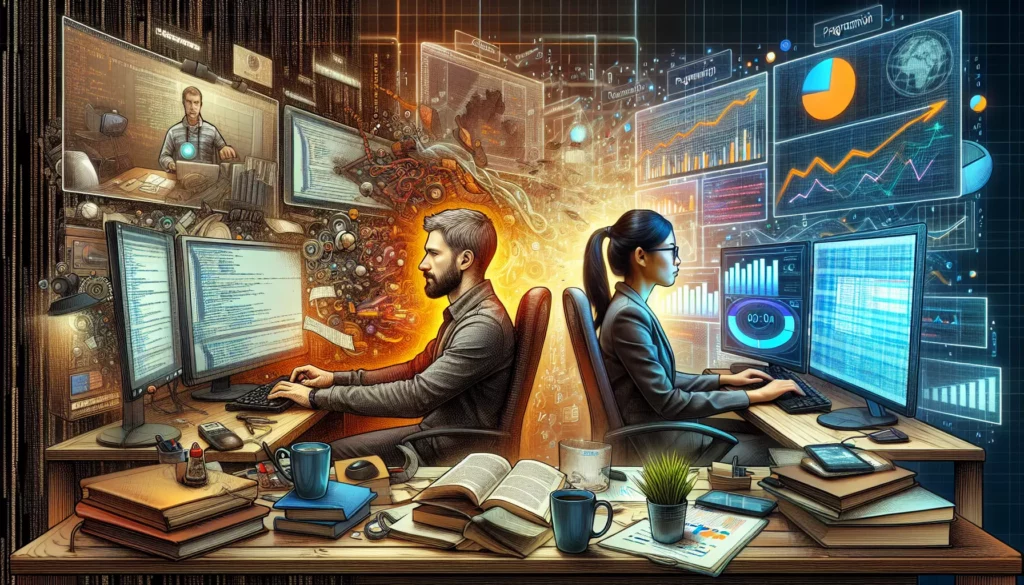
In today’s data-driven world, the role of a data scientist has become increasingly crucial across industries. For programmers looking to transition into this exciting field, the journey can be both challenging and rewarding. This comprehensive guide will walk you through the steps to become a data scientist, leveraging your existing programming skills and expanding your knowledge in key areas.
Table of Contents
- Understanding Data Science
- Assessing Your Current Skills
- Essential Skills for Data Scientists
- Building Your Data Science Foundation
- Mastering Data Analysis and Visualization
- Diving into Machine Learning
- Gaining Practical Experience
- Networking and Professional Development
- Landing Your First Data Science Job
- Continuous Learning and Growth
1. Understanding Data Science
Before embarking on your journey to become a data scientist, it’s essential to understand what data science entails. Data science is an interdisciplinary field that combines aspects of statistics, mathematics, computer science, and domain expertise to extract meaningful insights from data.
As a data scientist, you’ll be responsible for:
- Collecting, cleaning, and preprocessing data
- Analyzing data to identify patterns and trends
- Building predictive models using machine learning techniques
- Communicating findings to stakeholders through visualizations and reports
- Developing data-driven solutions to business problems
Your programming background gives you a head start in this field, as coding is a fundamental skill in data science. However, you’ll need to expand your knowledge and skillset to become a well-rounded data scientist.
2. Assessing Your Current Skills
Before diving into new areas of study, take stock of your current skills and identify areas for improvement. As a programmer, you likely have strengths in:
- Programming languages (e.g., Python, Java, C++)
- Software development principles
- Algorithmic thinking and problem-solving
- Version control (e.g., Git)
- Database management
These skills provide a solid foundation for your transition to data science. However, you may need to develop or enhance your knowledge in:
- Statistics and probability
- Linear algebra and calculus
- Data manipulation and analysis
- Machine learning algorithms
- Data visualization techniques
- Big data technologies
By identifying your strengths and areas for improvement, you can create a targeted learning plan to fill any knowledge gaps.
3. Essential Skills for Data Scientists
To succeed as a data scientist, you’ll need to develop a diverse set of skills. Here are the key areas to focus on:
3.1. Programming Languages
While you already have programming experience, it’s important to focus on languages commonly used in data science:
- Python: The most popular language for data science, with extensive libraries for data analysis and machine learning.
- R: Widely used for statistical computing and graphics.
- SQL: Essential for working with relational databases and querying large datasets.
3.2. Statistics and Mathematics
A strong foundation in statistics and mathematics is crucial for understanding data science concepts:
- Descriptive and inferential statistics
- Probability theory
- Linear algebra
- Calculus
- Optimization techniques
3.3. Data Manipulation and Analysis
Learn to work with various data formats and perform data cleaning, transformation, and analysis:
- Pandas for data manipulation in Python
- NumPy for numerical computing
- Data cleaning and preprocessing techniques
- Feature engineering
3.4. Machine Learning
Understand different machine learning algorithms and when to apply them:
- Supervised learning (e.g., regression, classification)
- Unsupervised learning (e.g., clustering, dimensionality reduction)
- Deep learning and neural networks
- Model evaluation and validation techniques
3.5. Data Visualization
Develop skills to create compelling visualizations that communicate insights effectively:
- Matplotlib and Seaborn for Python
- ggplot2 for R
- Interactive visualization tools (e.g., Plotly, Tableau)
3.6. Big Data Technologies
Familiarize yourself with tools for handling large-scale datasets:
- Hadoop ecosystem
- Apache Spark
- Distributed computing concepts
3.7. Domain Knowledge
Develop expertise in a specific industry or field to better understand the context of the data you’re working with.
4. Building Your Data Science Foundation
With a clear understanding of the skills needed, it’s time to start building your data science foundation. Here’s a step-by-step approach to get you started:
4.1. Strengthen Your Python Skills
If you’re not already proficient in Python, focus on mastering it for data science:
- Learn Python basics and advanced concepts
- Practice writing efficient and clean code
- Familiarize yourself with key data science libraries (NumPy, Pandas, Scikit-learn)
Here’s a simple example of using Pandas to read and display a CSV file:
import pandas as pd
# Read the CSV file
df = pd.read_csv('data.csv')
# Display the first 5 rows
print(df.head())
# Get basic information about the dataset
print(df.info())
4.2. Brush Up on Statistics and Mathematics
Strengthen your understanding of statistical concepts and mathematical foundations:
- Take online courses in statistics and probability
- Study linear algebra and calculus
- Practice applying statistical concepts to real-world problems
4.3. Learn Data Manipulation Techniques
Master the art of working with data using Pandas and NumPy:
- Practice data cleaning and preprocessing
- Learn to handle missing data and outliers
- Explore feature engineering techniques
Here’s an example of data manipulation using Pandas:
import pandas as pd
import numpy as np
# Create a sample dataset
data = {
'Name': ['Alice', 'Bob', 'Charlie', 'David'],
'Age': [25, 30, np.nan, 35],
'Salary': [50000, 60000, 55000, np.nan]
}
df = pd.DataFrame(data)
# Handle missing values
df['Age'].fillna(df['Age'].mean(), inplace=True)
df['Salary'].fillna(df['Salary'].median(), inplace=True)
# Create a new feature
df['Salary_Category'] = pd.cut(df['Salary'], bins=[0, 55000, 65000, np.inf], labels=['Low', 'Medium', 'High'])
print(df)
5. Mastering Data Analysis and Visualization
Data analysis and visualization are crucial skills for any data scientist. Here’s how to develop these skills:
5.1. Exploratory Data Analysis (EDA)
Learn to explore and understand your data through statistical and visual methods:
- Compute descriptive statistics
- Identify patterns and relationships in the data
- Detect anomalies and outliers
Here’s an example of basic EDA using Python:
import pandas as pd
import matplotlib.pyplot as plt
import seaborn as sns
# Load the dataset
df = pd.read_csv('dataset.csv')
# Display basic statistics
print(df.describe())
# Create a histogram
plt.figure(figsize=(10, 6))
df['Age'].hist(bins=20)
plt.title('Distribution of Age')
plt.xlabel('Age')
plt.ylabel('Frequency')
plt.show()
# Create a correlation heatmap
plt.figure(figsize=(12, 10))
sns.heatmap(df.corr(), annot=True, cmap='coolwarm')
plt.title('Correlation Heatmap')
plt.show()
5.2. Data Visualization Techniques
Develop your ability to create compelling visualizations:
- Master various chart types (e.g., bar charts, scatter plots, heatmaps)
- Learn to choose the right visualization for different data types
- Practice creating interactive visualizations
Here’s an example of creating a scatter plot with a regression line using Seaborn:
import seaborn as sns
import matplotlib.pyplot as plt
# Load a sample dataset
tips = sns.load_dataset('tips')
# Create a scatter plot with a regression line
plt.figure(figsize=(10, 6))
sns.regplot(x='total_bill', y='tip', data=tips)
plt.title('Relationship between Total Bill and Tip')
plt.xlabel('Total Bill')
plt.ylabel('Tip')
plt.show()
6. Diving into Machine Learning
Machine learning is a core component of data science. Here’s how to approach this vast field:
6.1. Understanding Machine Learning Fundamentals
- Learn the difference between supervised and unsupervised learning
- Understand common algorithms (e.g., linear regression, decision trees, k-means clustering)
- Study model evaluation metrics and techniques
6.2. Implementing Machine Learning Algorithms
Practice implementing various machine learning algorithms using libraries like Scikit-learn:
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LogisticRegression
from sklearn.metrics import accuracy_score, classification_report
# Assuming 'X' is your feature matrix and 'y' is your target variable
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Create and train the model
model = LogisticRegression()
model.fit(X_train, y_train)
# Make predictions
y_pred = model.predict(X_test)
# Evaluate the model
accuracy = accuracy_score(y_test, y_pred)
print(f"Accuracy: {accuracy}")
print(classification_report(y_test, y_pred))
6.3. Deep Learning and Neural Networks
Explore more advanced machine learning techniques:
- Study the basics of neural networks
- Learn to use deep learning frameworks like TensorFlow or PyTorch
- Implement and train deep learning models for various tasks
7. Gaining Practical Experience
Theory alone is not enough to become a proficient data scientist. You need hands-on experience to apply your skills effectively:
7.1. Work on Personal Projects
- Choose datasets that interest you and perform end-to-end analysis
- Participate in Kaggle competitions to solve real-world problems
- Create a portfolio showcasing your projects on GitHub
7.2. Contribute to Open Source Projects
- Find data science-related open source projects on GitHub
- Start with small contributions and gradually take on more complex tasks
- Collaborate with other data scientists and learn from their expertise
7.3. Internships and Part-time Opportunities
- Look for internships or part-time positions in data science roles
- Apply your skills to real-world business problems
- Gain experience working in a professional data science environment
8. Networking and Professional Development
Building a strong professional network can greatly accelerate your career transition:
8.1. Attend Data Science Conferences and Meetups
- Participate in local data science meetups
- Attend industry conferences to learn about the latest trends
- Network with other professionals in the field
8.2. Engage in Online Communities
- Join data science forums and discussion groups
- Participate in data science communities on platforms like Reddit or Stack Overflow
- Share your knowledge and learn from others
8.3. Build Your Online Presence
- Create a professional LinkedIn profile highlighting your data science skills
- Start a blog to share your insights and project experiences
- Engage with other data scientists on social media platforms
9. Landing Your First Data Science Job
With a strong foundation and practical experience, you’re ready to pursue your first data science role:
9.1. Tailor Your Resume and Cover Letter
- Highlight your relevant skills and projects
- Emphasize how your programming background adds value to data science roles
- Customize your application for each job opportunity
9.2. Prepare for Technical Interviews
- Practice coding challenges focused on data manipulation and analysis
- Review common data science interview questions
- Be prepared to explain your project experiences and methodologies
9.3. Consider Entry-level Positions
- Look for junior data scientist or data analyst roles
- Be open to positions that combine programming and data science skills
- Consider roles in industries where you have domain knowledge
10. Continuous Learning and Growth
The field of data science is constantly evolving, so it’s essential to commit to lifelong learning:
10.1. Stay Updated with the Latest Trends
- Follow data science blogs and publications
- Attend webinars and online courses to learn about new techniques and tools
- Experiment with emerging technologies in your personal projects
10.2. Pursue Advanced Education
- Consider pursuing a master’s degree in data science or a related field
- Take specialized courses to deepen your expertise in specific areas
- Obtain relevant certifications to validate your skills
10.3. Mentor Others and Share Your Knowledge
- Offer to mentor junior data scientists or aspiring professionals
- Present at conferences or write articles to share your experiences
- Contribute to the data science community through open-source projects or educational content
Conclusion
Transitioning from a programming background to a career in data science is an exciting journey that leverages your existing skills while opening up new opportunities. By following this comprehensive guide, you can systematically build the knowledge and experience needed to become a successful data scientist.
Remember that the path to becoming a data scientist is not linear, and everyone’s journey is unique. Stay curious, be persistent, and embrace the challenges along the way. With dedication and continuous learning, you’ll be well-equipped to thrive in the dynamic and rewarding field of data science.
As you progress in your data science career, don’t forget to give back to the community by sharing your knowledge and experiences with others. By contributing to the field’s growth, you’ll not only enhance your own skills but also help shape the future of data science.