How to Avoid Tunnel Vision: Recognizing When to Change Course in a Coding Interview
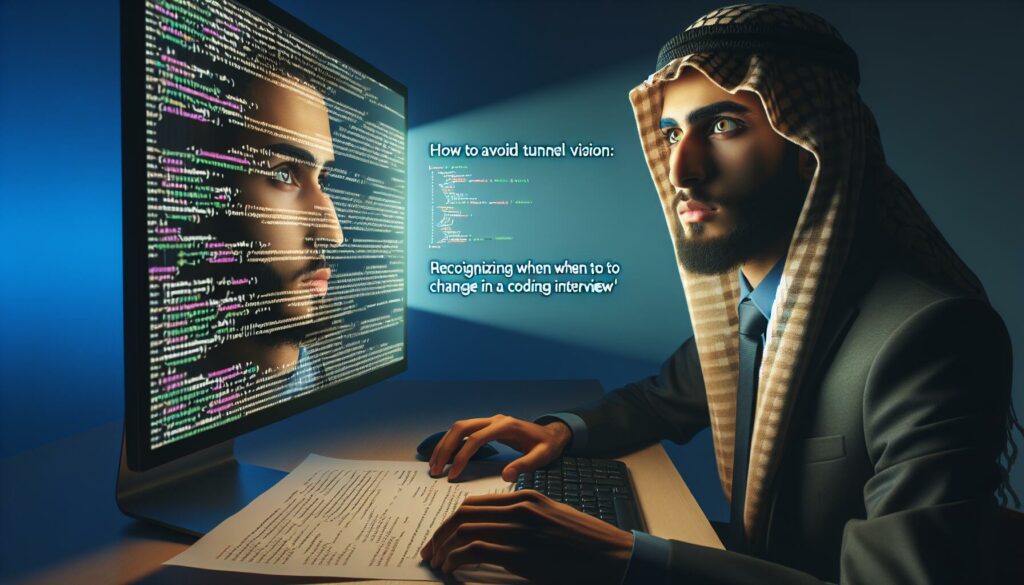
Coding interviews can be intense, high-pressure situations where your problem-solving skills are put to the test. One of the most crucial abilities you can develop for these interviews is knowing when to change course. This skill involves recognizing when your current approach isn’t working and being able to pivot to a new solution without losing momentum. In this comprehensive guide, we’ll explore how to avoid tunnel vision during coding interviews, provide strategies for recognizing when it’s time to switch gears, and share real-life examples of successful pivots.
Understanding Tunnel Vision in Coding Interviews
Tunnel vision in a coding interview occurs when a candidate becomes so focused on a particular approach to solving a problem that they fail to consider alternative solutions, even when their current method is proving ineffective. This can lead to wasted time, increased stress, and ultimately, a failure to solve the problem within the given timeframe.
Some common signs of tunnel vision include:
- Spending too much time debugging a specific implementation without progress
- Ignoring hints or suggestions from the interviewer
- Feeling stuck but continuing to pursue the same approach
- Losing track of time while working on a suboptimal solution
Strategies for Recognizing When to Change Course
Developing the ability to recognize when it’s time to pivot is crucial for success in coding interviews. Here are some strategies to help you identify when it’s time to change your approach:
1. Set Mental Checkpoints
Before diving into a problem, set mental checkpoints at regular intervals (e.g., every 5-10 minutes). When you reach a checkpoint, take a moment to assess your progress and the viability of your current approach.
2. Listen to Your Interviewer
Interviewers often provide subtle hints or ask leading questions when they notice you’re heading down an unproductive path. Pay close attention to their feedback and be open to changing direction based on their input.
3. Analyze the Complexity of Your Solution
Regularly evaluate the time and space complexity of your current approach. If you realize that your solution won’t meet the efficiency requirements, it’s a clear sign that you need to consider alternatives.
4. Track Your Progress
Keep a mental note of the milestones you’ve achieved in solving the problem. If you find yourself making little to no progress for an extended period, it might be time to reconsider your strategy.
5. Trust Your Instincts
If you have a nagging feeling that your current approach is overly complicated or doesn’t align with the problem’s requirements, don’t ignore it. Trust your instincts and be willing to explore other options.
How to Pivot Effectively
Once you’ve recognized the need to change course, the next challenge is to pivot effectively without losing valuable time or becoming discouraged. Here are some tips for making a smooth transition to a new approach:
1. Take a Step Back
Before abandoning your current solution entirely, take a moment to analyze what you’ve learned from your initial attempt. This reflection can often provide insights that will inform your new approach.
2. Communicate with Your Interviewer
Explain your thought process to the interviewer, including why you believe your current approach isn’t working and what alternative solutions you’re considering. This demonstrates your problem-solving skills and ability to adapt.
3. Start with a High-Level Approach
Instead of immediately diving into code, begin by outlining a high-level approach for your new solution. This can help you avoid getting bogged down in implementation details too quickly.
4. Reuse Relevant Parts
If possible, identify any parts of your initial solution that can be repurposed for your new approach. This can save time and demonstrate your ability to iterate on ideas.
5. Stay Calm and Focused
Changing course can be stressful, but it’s important to remain calm and focused. Remember that the ability to pivot is a valuable skill that interviewers appreciate.
Real-Life Examples of Successful Pivots
To illustrate the importance of recognizing when to change course, let’s look at some real-life examples of candidates who successfully pivoted during coding interviews:
Example 1: The Two Sum Problem
A candidate was given the classic “Two Sum” problem: Given an array of integers and a target sum, find two numbers in the array that add up to the target.
Initial Approach:
def two_sum(nums, target):
for i in range(len(nums)):
for j in range(i + 1, len(nums)):
if nums[i] + nums[j] == target:
return [i, j]
return []
The candidate began with a brute-force approach using nested loops. After implementing this solution, they realized its time complexity was O(n^2), which wasn’t optimal for large inputs.
Pivot:
Recognizing the need for a more efficient solution, the candidate explained their thought process to the interviewer and proposed using a hash table to improve time complexity.
def two_sum(nums, target):
num_map = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_map:
return [num_map[complement], i]
num_map[num] = i
return []
This pivot demonstrated the candidate’s ability to recognize an inefficient solution and quickly adapt to a more optimal approach with O(n) time complexity.
Example 2: Binary Tree Level Order Traversal
Another candidate was asked to implement a level order traversal of a binary tree.
Initial Approach:
The candidate started by implementing a depth-first search (DFS) solution, thinking it would be simpler:
def level_order_traversal(root):
result = []
def dfs(node, level):
if not node:
return
if len(result) == level:
result.append([])
result[level].append(node.val)
dfs(node.left, level + 1)
dfs(node.right, level + 1)
dfs(root, 0)
return result
While this solution works, the candidate realized it was more complex than necessary and didn’t directly reflect the level-by-level nature of the problem.
Pivot:
After explaining their thought process to the interviewer, the candidate decided to switch to a breadth-first search (BFS) approach using a queue:
from collections import deque
def level_order_traversal(root):
if not root:
return []
result = []
queue = deque([(root, 0)])
while queue:
node, level = queue.popleft()
if len(result) == level:
result.append([])
result[level].append(node.val)
if node.left:
queue.append((node.left, level + 1))
if node.right:
queue.append((node.right, level + 1))
return result
This pivot showcased the candidate’s ability to recognize a more intuitive and straightforward solution to the problem.
Example 3: Longest Palindromic Substring
A candidate was asked to find the longest palindromic substring in a given string.
Initial Approach:
The candidate began with a brute-force approach, checking all possible substrings:
def longest_palindrome(s):
def is_palindrome(sub):
return sub == sub[::-1]
max_length = 0
longest = ""
for i in range(len(s)):
for j in range(i, len(s)):
substring = s[i:j+1]
if is_palindrome(substring) and len(substring) > max_length:
max_length = len(substring)
longest = substring
return longest
After implementing this solution, the candidate realized its time complexity was O(n^3), which was highly inefficient for longer strings.
Pivot:
Recognizing the need for optimization, the candidate explained to the interviewer that they wanted to try a more efficient approach using dynamic programming:
def longest_palindrome(s):
n = len(s)
dp = [[False] * n for _ in range(n)]
start = 0
max_length = 1
# All substrings of length 1 are palindromes
for i in range(n):
dp[i][i] = True
# Check for substrings of length 2
for i in range(n - 1):
if s[i] == s[i + 1]:
dp[i][i + 1] = True
start = i
max_length = 2
# Check for lengths greater than 2
for length in range(3, n + 1):
for i in range(n - length + 1):
j = i + length - 1
if s[i] == s[j] and dp[i + 1][j - 1]:
dp[i][j] = True
if length > max_length:
start = i
max_length = length
return s[start:start + max_length]
This pivot demonstrated the candidate’s ability to recognize an inefficient solution and apply a more advanced algorithm to solve the problem optimally.
The Importance of Practice and Preparation
Developing the ability to recognize when to change course and pivot effectively during a coding interview is a skill that improves with practice. Here are some ways to hone this skill:
1. Mock Interviews
Participate in mock interviews with peers or mentors. Ask them to provide feedback on your problem-solving approach and your ability to pivot when necessary.
2. Timed Practice Problems
Solve coding problems with a timer to simulate the time pressure of a real interview. This will help you develop a sense of when you’re spending too much time on an ineffective approach.
3. Study Multiple Solutions
For each problem you encounter, study multiple solution approaches. This will broaden your problem-solving toolkit and make it easier to pivot to alternative solutions during an interview.
4. Reflect on Your Problem-Solving Process
After solving each problem, take time to reflect on your problem-solving process. Identify moments where you could have pivoted earlier or more effectively.
5. Learn from Others
Watch coding interview videos or read interview experiences from successful candidates. Pay attention to how they handle situations where they need to change their approach.
Conclusion
The ability to recognize when to change course and pivot effectively is a crucial skill for success in coding interviews. By developing strategies to identify when your current approach isn’t working, practicing smooth transitions to new solutions, and learning from real-life examples, you can enhance your problem-solving abilities and perform better under pressure.
Remember that changing course isn’t a sign of failure – it’s a demonstration of your adaptability, critical thinking, and commitment to finding the best solution. Embrace the pivot as an opportunity to showcase your skills and impress your interviewer.
As you continue to practice and refine your coding interview skills, focus on developing a flexible mindset that allows you to explore multiple approaches to each problem. With time and experience, you’ll become more adept at recognizing when to change course and executing effective pivots, setting yourself up for success in even the most challenging coding interviews.