How to Avoid Syntax Errors and Common Beginner Pitfalls
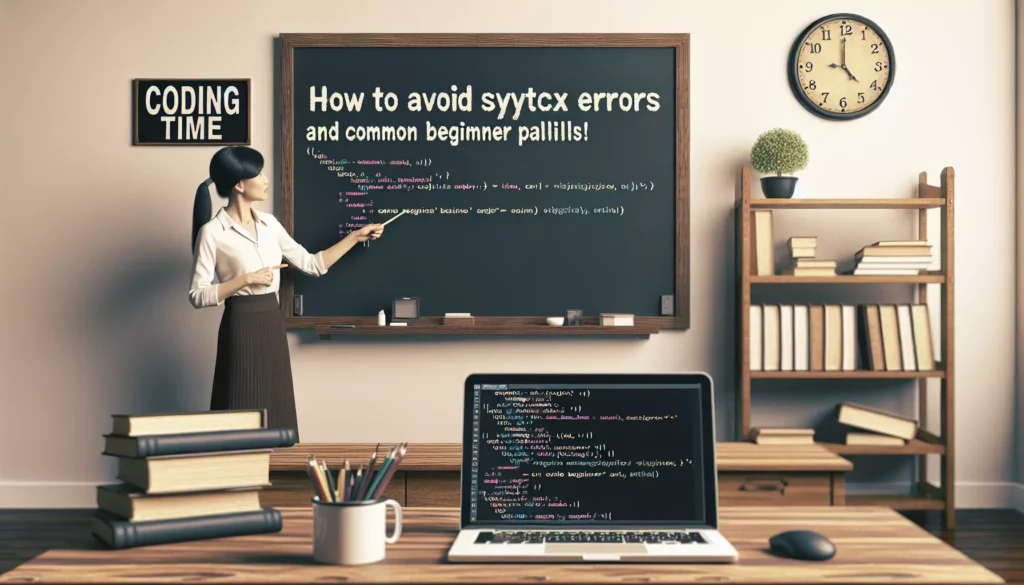
As a beginner programmer, you’re likely to encounter various challenges along your coding journey. One of the most common hurdles you’ll face is dealing with syntax errors and other common pitfalls that can slow down your progress and lead to frustration. In this comprehensive guide, we’ll explore strategies to help you avoid these issues and become a more efficient and confident coder.
Understanding Syntax Errors
Before we dive into how to avoid syntax errors, it’s essential to understand what they are. Syntax errors occur when you violate the rules of the programming language you’re using. These errors prevent your code from compiling or running correctly. Some common examples of syntax errors include:
- Missing or misplaced parentheses, brackets, or curly braces
- Forgetting to end statements with semicolons (in languages that require them)
- Misspelling keywords or variable names
- Using incorrect capitalization in case-sensitive languages
- Improper indentation (in languages like Python where indentation is significant)
Strategies to Avoid Syntax Errors
1. Use a Good Code Editor or IDE
One of the best ways to catch syntax errors early is to use a modern code editor or Integrated Development Environment (IDE) that offers features like syntax highlighting, auto-completion, and real-time error detection. These tools can help you identify and fix errors as you type, saving you time and frustration later on.
Some popular options include:
- Visual Studio Code (for multiple languages)
- PyCharm (for Python)
- IntelliJ IDEA (for Java)
- Sublime Text (for multiple languages)
2. Pay Attention to Parentheses and Brackets
Mismatched or missing parentheses, brackets, and curly braces are common sources of syntax errors. To avoid these issues:
- Always close parentheses, brackets, and curly braces immediately after opening them
- Use proper indentation to make your code structure clear
- Take advantage of your editor’s bracket matching feature
- Consider using a linter or formatter to automatically check and correct bracket issues
3. Be Consistent with Naming Conventions
Consistency in naming variables, functions, and classes can help you avoid syntax errors and make your code more readable. Follow these guidelines:
- Use descriptive names that clearly indicate the purpose of the variable or function
- Stick to a consistent naming convention (e.g., camelCase or snake_case)
- Avoid using reserved keywords as variable or function names
- Be mindful of case sensitivity in your chosen programming language
4. Pay Attention to Semicolons and Statement Terminators
In languages that require semicolons to terminate statements (like JavaScript, Java, or C++), forgetting to add them is a common source of syntax errors. To avoid this:
- Make it a habit to add semicolons at the end of each statement
- Use a linter or code formatter that can automatically add missing semicolons
- If you’re using a language that doesn’t require semicolons (like Python), be consistent in your use of newlines to separate statements
5. Use Proper Indentation
Proper indentation is crucial for readability and, in some languages like Python, it’s a syntax requirement. To maintain correct indentation:
- Use consistent indentation throughout your code (e.g., 2 or 4 spaces per level)
- Utilize your editor’s auto-indent feature
- For languages where indentation is significant, pay extra attention to nested blocks and function definitions
6. Comment Your Code
While comments don’t directly prevent syntax errors, they can help you understand your code better and catch logical errors. Good commenting practices include:
- Writing clear, concise comments that explain the purpose of complex code blocks
- Using inline comments sparingly to clarify non-obvious lines of code
- Updating comments when you modify the corresponding code
- Avoiding over-commenting obvious code
Common Beginner Pitfalls and How to Avoid Them
Beyond syntax errors, there are several common pitfalls that beginners often encounter. Let’s explore these issues and discuss strategies to avoid them.
1. Not Understanding Variable Scope
Variable scope refers to the part of the program where a variable is accessible. Misunderstanding scope can lead to unexpected behavior in your code.
To avoid scope-related issues:
- Learn the scoping rules of your programming language
- Use descriptive variable names to avoid confusion
- Minimize the use of global variables
- Be cautious when using variables with the same name in different scopes
Here’s an example of scope in Python:
def outer_function():
x = 10 # x is local to outer_function
def inner_function():
nonlocal x # This allows us to modify the outer x
x = 20
inner_function()
print(x) # This will print 20
outer_function()
2. Ignoring Error Messages
Error messages are your friends! They provide valuable information about what’s wrong with your code. Many beginners make the mistake of ignoring or not fully reading error messages.
To make the most of error messages:
- Read the entire error message carefully
- Pay attention to the line number and file name in the error message
- If you don’t understand the error, search online for explanations and solutions
- Use debuggers to step through your code and identify the source of the error
3. Not Breaking Down Problems into Smaller Steps
Trying to solve complex problems in one go can be overwhelming and lead to errors. Instead, break down the problem into smaller, manageable steps.
To improve your problem-solving approach:
- Start by outlining the main steps of your solution
- Implement and test each step individually
- Use functions to encapsulate distinct pieces of functionality
- Write pseudocode before actual code to clarify your thought process
4. Neglecting to Test Code Thoroughly
Many beginners assume their code works after testing it with a single input. This can lead to bugs that only appear under certain conditions.
To improve your testing practices:
- Test your code with various inputs, including edge cases
- Write unit tests for individual functions
- Use assertions to check for expected behavior
- Consider learning about test-driven development (TDD)
Here’s a simple example of using assertions in Python:
def divide(a, b):
assert b != 0, "Cannot divide by zero"
return a / b
# This will raise an AssertionError
try:
result = divide(10, 0)
except AssertionError as e:
print(f"Caught an error: {e}")
5. Copying and Pasting Code Without Understanding
While it’s tempting to copy and paste code from online sources, doing so without understanding how it works can lead to issues down the line.
To avoid this pitfall:
- Try to understand the code before using it in your project
- Modify the code to fit your specific needs
- Use comments to explain complex pieces of code you’ve borrowed
- Give credit to the original source when appropriate
6. Not Using Version Control
Version control systems like Git are essential tools for managing your code and collaborating with others. Many beginners overlook the importance of version control.
To start using version control effectively:
- Learn the basics of Git (or another version control system)
- Commit your changes regularly with meaningful commit messages
- Use branches to experiment with new features without affecting your main codebase
- Consider using platforms like GitHub or GitLab to store your repositories and collaborate with others
7. Neglecting Code Organization and Structure
As your projects grow, maintaining a clear and organized code structure becomes crucial. Poor organization can lead to confusion and errors.
To improve your code organization:
- Use meaningful file and directory names
- Group related functionality into separate modules or classes
- Follow the principle of separation of concerns
- Use consistent formatting and style throughout your project
8. Not Seeking Help When Stuck
Many beginners hesitate to ask for help when they encounter difficulties. Remember, everyone was a beginner once, and seeking help is a crucial part of the learning process.
To get help effectively:
- Search for solutions online before asking (but don’t spend too long if you can’t find an answer)
- Clearly describe your problem and what you’ve tried when asking for help
- Utilize resources like Stack Overflow, Reddit programming communities, or Discord channels
- Consider finding a mentor or joining a coding community
Tools and Resources to Help You Avoid Errors and Pitfalls
There are numerous tools and resources available to help you write better code and avoid common errors. Here are some recommendations:
1. Linters and Code Formatters
Linters analyze your code for potential errors and style violations, while code formatters automatically format your code to adhere to a consistent style. Some popular options include:
- ESLint (for JavaScript)
- Pylint (for Python)
- RuboCop (for Ruby)
- Prettier (a code formatter for multiple languages)
2. Debuggers
Debuggers allow you to step through your code line by line, inspect variables, and identify the source of errors. Most modern IDEs come with built-in debuggers, but some standalone options include:
- GDB (GNU Debugger) for C and C++
- pdb (Python Debugger)
- Chrome DevTools for JavaScript
3. Online Coding Platforms
Platforms like AlgoCademy provide interactive coding environments where you can practice coding, solve problems, and receive immediate feedback. These platforms often include features like:
- Step-by-step tutorials
- AI-powered assistance
- Instant code execution and testing
- A community of learners and mentors
4. Documentation and Learning Resources
Familiarizing yourself with official documentation and high-quality learning resources can help you avoid errors and understand best practices. Some valuable resources include:
- Official language documentation (e.g., Python.org, MDN for JavaScript)
- Online courses platforms like Coursera, edX, and Udacity
- Programming books (both physical and e-books)
- YouTube tutorials and coding channels
Conclusion
Avoiding syntax errors and common beginner pitfalls is an essential skill for any programmer. By following the strategies outlined in this guide, using appropriate tools and resources, and maintaining a growth mindset, you can significantly reduce the number of errors in your code and become a more proficient programmer.
Remember that making mistakes is a natural part of the learning process. Don’t get discouraged when you encounter errors or challenges. Instead, view them as opportunities to learn and improve your skills. With practice and persistence, you’ll find that you’re making fewer errors and writing more efficient, readable code.
Keep coding, stay curious, and don’t hesitate to seek help when you need it. Happy coding!