How to Avoid Overthinking During Coding Interviews: A Comprehensive Guide
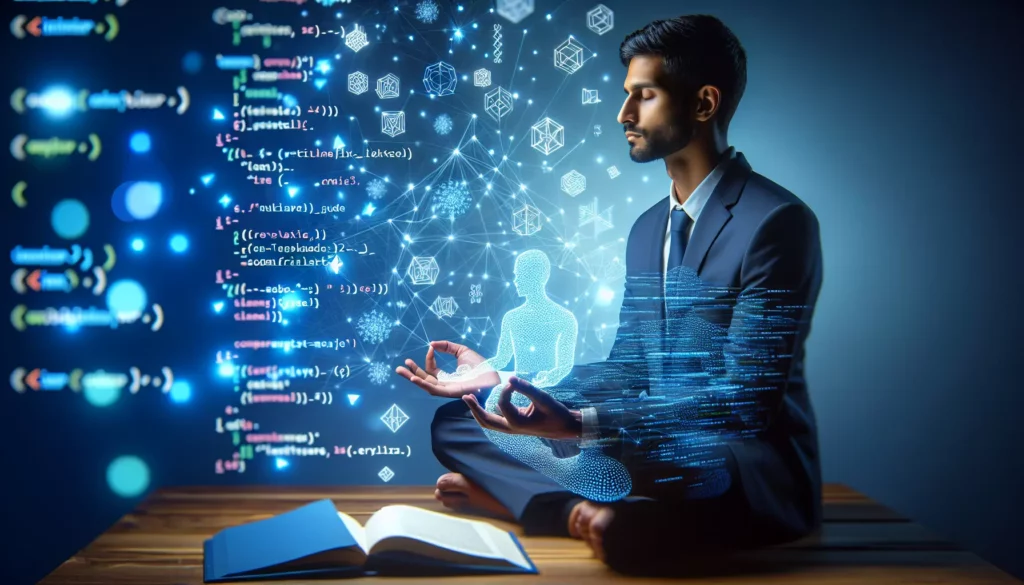
Coding interviews can be nerve-wracking experiences, especially when you’re aiming for positions at top tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google). One common pitfall that many candidates face is overthinking during these interviews. This tendency can lead to wasted time, increased stress, and ultimately, a suboptimal performance. In this comprehensive guide, we’ll explore effective strategies to help you avoid overthinking and perform at your best during coding interviews.
Understanding the Root of Overthinking
Before we dive into strategies, it’s essential to understand why overthinking occurs during coding interviews. Some common reasons include:
- Pressure to perform well
- Fear of making mistakes
- Desire to impress the interviewer
- Anxiety about time constraints
- Perfectionism
- Lack of confidence in one’s abilities
Recognizing these factors can help you address them more effectively and develop targeted strategies to overcome overthinking.
Strategies to Avoid Overthinking
1. Practice, Practice, Practice
One of the most effective ways to reduce overthinking is to build your confidence through consistent practice. The more familiar you are with various problem types and coding patterns, the less likely you are to second-guess yourself during the interview.
Consider the following approaches:
- Solve coding problems regularly on platforms like AlgoCademy, LeetCode, or HackerRank
- Participate in mock interviews with peers or mentors
- Time yourself while solving problems to simulate interview conditions
- Review and learn from your mistakes after each practice session
2. Develop a Structured Approach
Having a clear, step-by-step approach to problem-solving can help you stay focused and avoid getting lost in unnecessary details. Follow this general structure:
- Carefully read and understand the problem statement
- Ask clarifying questions to ensure you have all necessary information
- Discuss potential approaches and trade-offs with the interviewer
- Choose an approach and outline your solution
- Implement the solution, starting with a basic working version
- Test and optimize your code
By following a consistent structure, you’ll be less likely to overthink each step and more likely to make steady progress.
3. Start with a Brute Force Solution
Don’t get caught up in finding the most optimal solution right away. Begin with a brute force approach, even if it’s not the most efficient. This accomplishes several things:
- It demonstrates your problem-solving skills
- It gives you a working solution to build upon
- It helps you identify potential optimizations
- It shows the interviewer your thought process
Remember, it’s often easier to optimize a working solution than to come up with the perfect solution from scratch.
4. Think Aloud
Verbalizing your thoughts can help you stay focused and avoid getting stuck in your head. It also allows the interviewer to understand your thought process and provide guidance if needed. Some tips for effective think-aloud:
- Explain your reasoning for each decision
- Discuss trade-offs between different approaches
- Ask for feedback or confirmation when unsure
- Acknowledge when you’re stuck and describe what you’re trying to figure out
5. Use Pseudocode
Before diving into actual coding, write out your solution in pseudocode. This can help you organize your thoughts and catch potential issues early on, reducing the likelihood of overthinking during implementation. Here’s an example of how pseudocode might look for a simple problem:
// Problem: Find the maximum element in an array
function findMax(arr):
if arr is empty:
return null
max = first element of arr
for each element in arr:
if element > max:
max = element
return max
6. Break Down Complex Problems
When faced with a complex problem, break it down into smaller, more manageable subproblems. This approach can help prevent overwhelm and reduce the tendency to overthink. For example, if you’re asked to implement a complex data structure, you might break it down as follows:
- Define the basic structure (e.g., nodes, connections)
- Implement core operations (e.g., insert, delete)
- Add auxiliary functions (e.g., search, traverse)
- Optimize and handle edge cases
7. Set Time Limits for Each Step
Allocate specific time limits for different phases of your problem-solving process. This can help you avoid spending too much time on any single aspect. A sample time allocation might look like this:
- Understanding the problem and asking questions: 2-3 minutes
- Discussing potential approaches: 3-5 minutes
- Outlining the solution: 2-3 minutes
- Implementing the solution: 15-20 minutes
- Testing and optimizing: 5-10 minutes
Adjust these times based on the complexity of the problem and the total time available for the interview.
8. Embrace Imperfection
Remember that interviewers are often more interested in your problem-solving process than in a perfect solution. It’s okay to make mistakes or have less-than-optimal code. Focus on demonstrating your ability to think through problems, communicate effectively, and adapt to feedback.
9. Use Visualization Techniques
Visual aids can help clarify your thoughts and reduce overthinking. Consider using:
- Flowcharts to illustrate algorithm logic
- Diagrams to represent data structures
- Tables to track variable states
- Examples to work through edge cases
These visual tools can help you and the interviewer stay on the same page and catch potential issues early.
10. Practice Mindfulness
Incorporating mindfulness techniques can help you stay present and focused during the interview, reducing the tendency to overthink. Try these techniques:
- Take deep breaths to calm your nerves
- Practice grounding exercises to stay present
- Use positive self-talk to boost confidence
- Acknowledge anxious thoughts without dwelling on them
Common Pitfalls to Avoid
While working to avoid overthinking, be aware of these common pitfalls:
1. Analysis Paralysis
Avoid getting stuck in an endless loop of analyzing different approaches without making progress. If you find yourself spending too much time comparing options, choose one and move forward. You can always adjust your approach later if needed.
2. Premature Optimization
Don’t focus on optimizing your code before you have a working solution. It’s better to have a functional, less efficient solution that you can improve than to get stuck trying to create the perfect algorithm from the start.
3. Ignoring Interviewer Hints
Pay attention to any hints or guidance the interviewer provides. They may be trying to steer you in the right direction or help you avoid common pitfalls. Don’t overthink their intentions; take their input at face value and use it to your advantage.
4. Fixating on Minor Details
Avoid getting bogged down in minor implementation details or syntax. Focus on the overall logic and structure of your solution. If you’re unsure about a specific syntax or method name, it’s okay to ask the interviewer or use a placeholder.
5. Comparing Yourself to Others
Don’t waste mental energy wondering how other candidates might approach the problem or how you compare to them. Focus on showcasing your own skills and problem-solving abilities.
Preparing for Success
To set yourself up for success and minimize overthinking during coding interviews, consider these preparatory steps:
1. Build a Strong Foundation
Ensure you have a solid understanding of fundamental data structures, algorithms, and problem-solving techniques. Resources like AlgoCademy can help you build and reinforce these skills through interactive tutorials and practice problems.
2. Create a Study Plan
Develop a structured study plan that covers key topics and allows for regular practice. Include a mix of problem types and difficulty levels to ensure well-rounded preparation.
3. Simulate Interview Conditions
Practice under conditions that mimic real interviews as closely as possible. This might include:
- Using a whiteboard or simple text editor instead of an IDE
- Setting strict time limits for solving problems
- Having a friend or mentor act as the interviewer
- Practicing think-aloud problem-solving
4. Review Common Interview Questions
While you can’t predict exact interview questions, familiarizing yourself with common problem types can boost your confidence and reduce overthinking. Focus on areas like:
- Array and string manipulation
- Linked lists and trees
- Graph algorithms
- Dynamic programming
- Sorting and searching
5. Develop a Personal Problem-Solving Framework
Create a framework that works for you and practice applying it consistently. This might include steps like:
- Restating the problem in your own words
- Identifying input and output requirements
- Considering edge cases and constraints
- Sketching out a high-level approach
- Implementing and testing the solution incrementally
During the Interview
When you’re in the actual interview, keep these tips in mind to avoid overthinking:
1. Stay Calm and Composed
Take a deep breath and remind yourself that you’ve prepared for this. A calm mindset will help you think more clearly and avoid spiraling into overthinking.
2. Communicate Clearly
Express your thoughts clearly and concisely. If you’re unsure about something, ask for clarification. Clear communication can help prevent misunderstandings that might lead to overthinking.
3. Manage Your Time
Keep an eye on the clock and stick to your planned time allocations for each phase of problem-solving. This will help you maintain a steady pace and avoid getting stuck on any one aspect.
4. Be Open to Feedback
If the interviewer provides feedback or suggestions, be receptive and adaptable. Don’t overthink their intentions; use their input to improve your solution.
5. Focus on Progress, Not Perfection
Remember that the goal is to demonstrate your problem-solving skills, not to create a flawless solution. Focus on making steady progress and explaining your thought process along the way.
Conclusion
Overthinking during coding interviews is a common challenge, but it’s one that can be overcome with the right strategies and preparation. By practicing regularly, developing a structured approach, and focusing on clear communication, you can minimize overthinking and showcase your true abilities.
Remember that interviewers are often more interested in your problem-solving process than in perfect solutions. Embrace the challenge, stay calm, and trust in your preparation. With these strategies in mind, you’ll be well-equipped to tackle coding interviews with confidence and clarity.
Keep practicing, stay curious, and don’t let overthinking hold you back from achieving your goals in the world of software development. Happy coding, and best of luck in your future interviews!