How to Avoid Common Mistakes in Coding Challenges
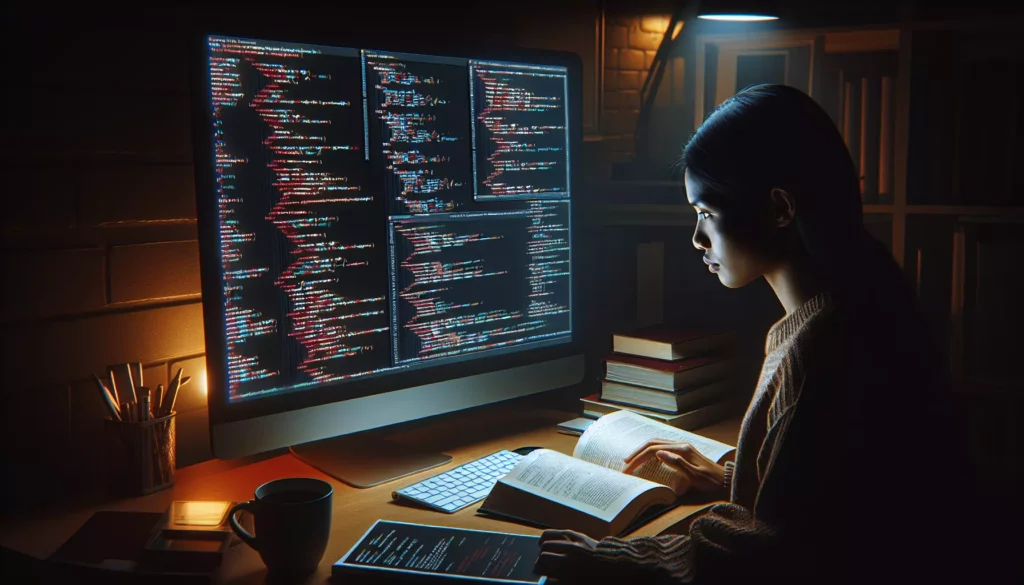
Coding challenges are an integral part of the journey for aspiring programmers and seasoned developers alike. Whether you’re preparing for a technical interview, participating in coding competitions, or simply honing your skills, these challenges can be both exciting and daunting. However, many programmers fall into common traps that can hinder their progress and success. In this comprehensive guide, we’ll explore the most frequent mistakes made during coding challenges and provide practical strategies to avoid them, helping you become a more proficient and confident coder.
1. Failing to Understand the Problem
One of the most critical mistakes in coding challenges is jumping into coding without fully grasping the problem at hand. This often leads to wasted time and incorrect solutions.
How to avoid this mistake:
- Read the problem statement carefully: Take your time to understand every detail of the problem. Don’t skim through it.
- Identify the input and output: Clearly define what the function should receive and return.
- Consider edge cases: Think about extreme or unusual inputs that might affect your solution.
- Ask clarifying questions: If something is unclear, don’t hesitate to ask for clarification. In real interviews, this shows critical thinking.
2. Neglecting to Plan Before Coding
Many programmers make the mistake of diving straight into coding without a clear plan or strategy. This can lead to disorganized code and inefficient solutions.
How to avoid this mistake:
- Outline your approach: Jot down the steps you’ll take to solve the problem.
- Consider multiple solutions: Think about different ways to approach the problem and choose the most efficient one.
- Use pseudocode: Write out your logic in plain language before translating it into actual code.
- Draw diagrams: Visual representations can help clarify complex problems and solutions.
3. Overlooking Time and Space Complexity
In coding challenges, especially those in technical interviews, the efficiency of your solution is often as important as its correctness. Ignoring time and space complexity can result in suboptimal solutions.
How to avoid this mistake:
- Analyze your algorithm: Consider the time and space complexity of your solution using Big O notation.
- Look for optimization opportunities: Can you reduce the number of loops or use more efficient data structures?
- Consider trade-offs: Sometimes, you may need to balance time complexity against space complexity.
- Practice with different problem types: Familiarize yourself with common algorithmic patterns and their complexities.
4. Ignoring Edge Cases and Input Validation
Failing to consider edge cases or validate input can lead to solutions that work for typical cases but fail under unusual circumstances.
How to avoid this mistake:
- Identify potential edge cases: Consider empty inputs, extremely large or small values, and other unusual scenarios.
- Implement input validation: Check for invalid inputs and handle them appropriately.
- Write test cases: Create a set of test cases that cover both typical and edge cases.
- Use defensive programming techniques: Anticipate and handle potential errors in your code.
5. Not Testing Your Code Thoroughly
Submitting a solution without proper testing is a recipe for disaster. Many programmers make the mistake of assuming their code works after testing it with one or two examples.
How to avoid this mistake:
- Start with small test cases: Begin with simple inputs to verify basic functionality.
- Progress to complex cases: Gradually introduce more complicated inputs and edge cases.
- Use a variety of test cases: Include different types of inputs to ensure comprehensive coverage.
- Implement unit tests: If time allows, write formal unit tests for your solution.
6. Overcomplicating the Solution
Sometimes, in an attempt to showcase their skills, programmers overcomplicate their solutions, making them harder to understand and more prone to errors.
How to avoid this mistake:
- Aim for simplicity: Start with the simplest solution that solves the problem correctly.
- Refactor for clarity: If your solution becomes complex, take time to simplify and clarify your code.
- Use appropriate data structures: Choose the right tools for the job, but don’t overcomplicate things unnecessarily.
- Follow the KISS principle: Keep It Simple, Stupid. Simplicity often leads to more robust and maintainable code.
7. Poor Naming Conventions and Code Organization
Unclear variable names and disorganized code can make your solution difficult to understand and debug, even if it’s functionally correct.
How to avoid this mistake:
- Use descriptive variable names: Choose names that clearly indicate the purpose of each variable.
- Follow consistent naming conventions: Stick to a single style (e.g., camelCase or snake_case) throughout your code.
- Organize your code logically: Group related functionality together and use appropriate indentation.
- Add comments when necessary: Use comments to explain complex logic or non-obvious decisions.
8. Neglecting to Handle Errors Gracefully
Failing to implement proper error handling can lead to crashes and unexpected behavior in your code.
How to avoid this mistake:
- Anticipate potential errors: Think about what could go wrong in your code and plan for it.
- Use try-catch blocks: Implement exception handling to gracefully manage errors.
- Provide meaningful error messages: When errors occur, give clear and helpful feedback.
- Fail gracefully: Ensure your program can recover or exit cleanly when errors occur.
9. Ignoring Code Efficiency and Optimization
While a brute-force solution might work for small inputs, it’s important to consider efficiency, especially for larger datasets.
How to avoid this mistake:
- Learn common optimization techniques: Familiarize yourself with techniques like memoization, dynamic programming, and efficient data structures.
- Profile your code: Use tools to identify performance bottlenecks in your solution.
- Consider space-time trade-offs: Sometimes, using more memory can significantly speed up your algorithm.
- Optimize iteratively: Start with a working solution, then refine it for better performance.
10. Not Explaining Your Thought Process
In interview settings, failing to communicate your thought process can be just as detrimental as providing an incorrect solution.
How to avoid this mistake:
- Think out loud: Verbalize your thought process as you work through the problem.
- Explain your decisions: Justify why you chose a particular approach or data structure.
- Discuss trade-offs: If there are multiple valid approaches, explain why you chose one over the others.
- Be open to feedback: If the interviewer suggests an alternative, consider it thoughtfully.
11. Failing to Manage Time Effectively
Poor time management during coding challenges can lead to incomplete or rushed solutions.
How to avoid this mistake:
- Read the instructions carefully: Understand the time constraints and requirements before starting.
- Allocate time for each phase: Budget time for understanding the problem, planning, coding, and testing.
- Use a timer: Keep track of how much time you’re spending on each part of the challenge.
- Know when to move on: If you’re stuck on a problem, consider moving to the next one and coming back later if time allows.
12. Not Leveraging Built-in Functions and Libraries
Reinventing the wheel by implementing functionality that already exists in standard libraries is a common mistake that wastes time and introduces potential bugs.
How to avoid this mistake:
- Know your language’s standard library: Familiarize yourself with built-in functions and data structures.
- Use appropriate libraries: If allowed, leverage well-known libraries for complex operations.
- Balance using libraries with demonstrating skills: In interviews, explain your choice to use a library function versus implementing it yourself.
- Stay updated on language features: Keep abreast of new features in your programming language that can simplify your code.
13. Neglecting Code Readability and Style
Writing code that works is important, but writing code that is easy to read and understand is equally crucial, especially in collaborative environments.
How to avoid this mistake:
- Follow style guidelines: Adhere to established style guides for your programming language.
- Use meaningful variable and function names: Choose names that clearly describe the purpose or content.
- Keep functions small and focused: Each function should do one thing and do it well.
- Use comments judiciously: Comment on why you’re doing something, not what you’re doing, if it’s not immediately clear from the code.
14. Failing to Consider Scalability
Solutions that work for small inputs may fail spectacularly when scaled up. Ignoring scalability can lead to inefficient solutions in real-world scenarios.
How to avoid this mistake:
- Think about larger inputs: Consider how your solution would perform with much larger datasets.
- Analyze asymptotic complexity: Understand how your algorithm’s performance grows with input size.
- Consider memory usage: Be mindful of space complexity, especially for large inputs.
- Test with varying input sizes: If possible, test your solution with small, medium, and large inputs to observe scaling behavior.
15. Not Learning from Mistakes
Perhaps the biggest mistake of all is not learning from the errors you make during coding challenges.
How to avoid this mistake:
- Review your solutions: After completing a challenge, take time to analyze your approach and identify areas for improvement.
- Study optimal solutions: Look at other efficient solutions to the same problem and understand their advantages.
- Keep a coding journal: Document the challenges you face and the lessons you learn from each coding session.
- Practice regularly: Consistent practice helps reinforce good habits and identify areas where you commonly make mistakes.
Practical Example: Avoiding Common Mistakes
Let’s look at a practical example of how to avoid common mistakes when solving a coding challenge. Consider the following problem:
Write a function that finds the longest palindromic substring in a given string.
Here’s an implementation that avoids common mistakes:
def longest_palindromic_substring(s):
if not s:
return "" # Handle empty input
def expand_around_center(left, right):
while left >= 0 and right < len(s) and s[left] == s[right]:
left -= 1
right += 1
return s[left + 1:right]
longest = ""
for i in range(len(s)):
# Check for odd-length palindromes
palindrome1 = expand_around_center(i, i)
# Check for even-length palindromes
palindrome2 = expand_around_center(i, i + 1)
# Update longest if a longer palindrome is found
if len(palindrome1) > len(longest):
longest = palindrome1
if len(palindrome2) > len(longest):
longest = palindrome2
return longest
# Test the function
print(longest_palindromic_substring("babad")) # Output: "bab" or "aba"
print(longest_palindromic_substring("cbbd")) # Output: "bb"
print(longest_palindromic_substring("")) # Output: "" (empty string)
print(longest_palindromic_substring("a")) # Output: "a"
This solution avoids common mistakes by:
- Understanding the problem: The function correctly identifies the longest palindromic substring.
- Planning before coding: The approach uses the efficient “expand around center” technique.
- Considering time complexity: This solution has O(n^2) time complexity, which is efficient for this problem.
- Handling edge cases: It correctly handles empty strings and single-character inputs.
- Using descriptive names: Function and variable names clearly indicate their purpose.
- Avoiding overcomplication: The solution is straightforward and easy to understand.
- Testing thoroughly: Multiple test cases are provided, including edge cases.
Conclusion
Mastering coding challenges is a journey of continuous learning and improvement. By being aware of these common mistakes and actively working to avoid them, you can significantly enhance your problem-solving skills and become a more effective programmer. Remember, the goal is not just to solve the problem, but to do so efficiently, clearly, and robustly.
As you continue to practice and participate in coding challenges, keep these tips in mind:
- Take time to fully understand the problem before coding.
- Plan your approach and consider multiple solutions.
- Think about efficiency and scalability from the start.
- Write clean, readable code with meaningful names and proper organization.
- Test your solution thoroughly, including edge cases.
- Communicate your thought process clearly, especially in interview settings.
- Learn from each challenge and continuously refine your skills.
By avoiding these common mistakes and following best practices, you’ll not only perform better in coding challenges but also develop habits that will serve you well throughout your programming career. Happy coding!