How to Ask the Right Questions in a Coding Interview to Solve Problems Faster
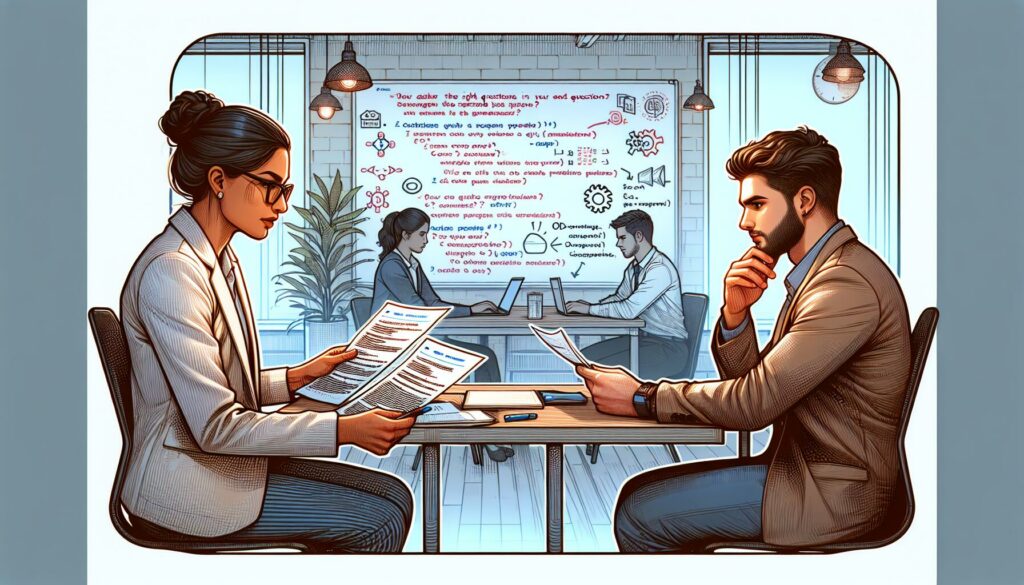
When faced with a coding interview, your ability to solve problems efficiently isn’t just about your coding skills—it’s also about how well you can gather information and clarify the problem at hand. Asking the right questions can be the key to unlocking a swift and accurate solution. In this comprehensive guide, we’ll explore why asking questions is crucial, what types of questions you should ask, and how this approach can dramatically improve your problem-solving speed and accuracy in coding interviews.
The Importance of Asking Questions in Coding Interviews
Many candidates make the mistake of diving straight into coding without fully understanding the problem. This can lead to wasted time, incorrect solutions, and unnecessary stress. By asking thoughtful questions, you can:
- Clarify ambiguities in the problem statement
- Uncover hidden requirements or constraints
- Demonstrate your analytical thinking and communication skills
- Build rapport with the interviewer
- Gain valuable insights that can guide your solution
Remember, interviewers often intentionally provide vague or incomplete problem descriptions to see how you handle uncertainty and gather information.
How Asking Questions Prevents Wasted Time
Jumping into coding without a clear understanding of the problem can lead to several time-wasting pitfalls:
- Solving the wrong problem: You might misinterpret the requirements and solve something entirely different from what was asked.
- Overlooking edge cases: Without clarification, you might miss critical edge cases that your solution needs to handle.
- Choosing an inefficient approach: Lack of information about constraints or input size can lead to selecting a suboptimal algorithm.
- Overengineering: You might add unnecessary complexity to your solution by making incorrect assumptions about the problem’s scope.
- Rewriting code: Realizing you’ve misunderstood something halfway through coding often means starting over, wasting precious interview time.
By asking the right questions upfront, you can avoid these pitfalls and streamline your problem-solving process.
Essential Questions to Ask in a Coding Interview
Here’s a comprehensive list of questions you should consider asking when presented with a new problem in a coding interview:
1. Input Clarification
- What is the exact format of the input?
- What data types should I expect for each input?
- Are there any constraints on the input values (e.g., positive integers only, maximum string length)?
- How large can the input be? What’s the maximum size I should consider?
- Can the input be empty or null?
- For array or list inputs, can they contain duplicates?
- For string inputs, should I consider only ASCII characters or Unicode as well?
2. Output Expectations
- What exactly should the function return?
- In what format should the output be presented?
- For multiple possible solutions, should I return all of them or just one?
- How should I handle error cases or invalid inputs?
3. Constraints and Edge Cases
- Are there any time complexity requirements for the solution?
- Are there any space complexity limitations?
- How should I handle edge cases like empty inputs, single-element inputs, or extremely large inputs?
- Are there any specific edge cases you want me to consider?
4. Assumptions and Clarifications
- Can I assume the input is always valid, or do I need to validate it?
- Are there any implicit assumptions I should be aware of?
- Is it okay to modify the input, or should I work with a copy?
- For graph or tree problems: Is the graph/tree guaranteed to be connected? Can there be cycles?
5. Problem-Specific Questions
- For sorting problems: Does the order of equal elements matter (stable sort)?
- For search problems: Is the input sorted or can I assume any order?
- For optimization problems: Is it okay to use additional data structures, or should I optimize for space?
6. Clarifying the Problem Statement
- Can you provide an example input and the expected output for that input?
- Are there any specific cases or scenarios I should pay special attention to?
- Is this problem similar to any well-known algorithms or data structures?
How to Ask Questions Effectively
Asking questions is an art. Here are some tips to help you ask questions effectively during a coding interview:
- Start broad, then narrow down: Begin with general questions about the problem before diving into specifics.
- Use the problem statement as a guide: Analyze each part of the problem statement and ask for clarification on anything that’s unclear.
- Prioritize your questions: Ask the most critical questions first, in case time runs short.
- Listen actively: Pay close attention to the interviewer’s responses, as they may contain valuable hints or additional information.
- Confirm your understanding: After receiving answers, summarize your understanding to ensure you’ve interpreted the information correctly.
- Be respectful of time: While it’s important to ask questions, be mindful of the interview’s time constraints.
Example: Applying the Question-Asking Strategy
Let’s walk through an example of how asking the right questions can help you solve a problem more efficiently. Suppose you’re given the following problem statement:
“Implement a function to find the kth largest element in an array.”
Here’s how you might approach this with questions:
- You: “Can you clarify what you mean by the kth largest element? Is it the kth distinct largest element, or can we have duplicates?”
- Interviewer: “Consider duplicates as distinct elements. So in the array [5,5,4,3,2], 5 would be both the 1st and 2nd largest element.”
- You: “Understood. What are the constraints on the input array? Can it be empty? What’s the maximum size we should consider?”
- Interviewer: “The array will always have at least one element, and you can assume its maximum length is 10^5.”
- You: “Got it. And what about the value of k? Can it be larger than the array size?”
- Interviewer: “K will always be a valid index, so it will be between 1 and the array length, inclusive.”
- You: “Alright. Are there any constraints on the elements in the array? Are they integers, floating-point numbers, or something else?”
- Interviewer: “They will be integers, and you can assume they fit within the range of a 32-bit integer.”
- You: “Thank you. Is there any specific time complexity requirement for the solution?”
- Interviewer: “Good question. We’re looking for a solution that’s better than O(n log n) in the average case.”
- You: “I see. One last question: is it okay to modify the input array, or should I work with a copy?”
- Interviewer: “You can modify the input array if needed.”
With these clarifications, you now have a much clearer picture of the problem and its constraints. This information guides you towards an efficient solution:
- You know you need to handle duplicates, so a simple sorting approach might work but wouldn’t meet the time complexity requirement.
- The constraint on time complexity suggests using a selection algorithm like QuickSelect, which has an average-case time complexity of O(n).
- You can modify the input array, which allows for in-place partitioning in the QuickSelect algorithm.
- You don’t need to worry about empty arrays or invalid k values, simplifying your code.
Armed with this information, you can now confidently implement a solution using the QuickSelect algorithm, knowing that it meets all the stated requirements and constraints.
Implementing the Solution
Based on the information gathered through our questions, here’s how we might implement the solution:
import random
def findKthLargest(nums, k):
def quickSelect(l, r, k):
pivot = random.randint(l, r)
nums[pivot], nums[r] = nums[r], nums[pivot]
store = l
for i in range(l, r):
if nums[i] > nums[r]:
nums[store], nums[i] = nums[i], nums[store]
store += 1
nums[r], nums[store] = nums[store], nums[r]
if k == r - store + 1:
return nums[store]
elif k < r - store + 1:
return quickSelect(store + 1, r, k)
else:
return quickSelect(l, store - 1, k - (r - store + 1))
return quickSelect(0, len(nums) - 1, k)
# Example usage
arr = [3, 2, 1, 5, 6, 4]
k = 2
result = findKthLargest(arr, k)
print(f"The {k}th largest element is: {result}")
This implementation uses the QuickSelect algorithm, which has an average-case time complexity of O(n), meeting the interviewer’s requirement for a solution better than O(n log n). It also handles duplicates correctly and modifies the input array in-place, as we confirmed was allowed.
The Impact of Asking the Right Questions
By asking the right questions, we were able to:
- Clarify the exact problem requirements (handling duplicates, valid k values)
- Understand the input constraints (array size, element types)
- Identify the performance expectations (better than O(n log n))
- Confirm what modifications were allowed (in-place array modification)
This information directly guided us to an optimal solution, saving time and ensuring we met all the interviewer’s criteria. Without these questions, we might have wasted time implementing a less efficient sorting-based solution or unnecessarily complicating our code to handle edge cases that weren’t actually part of the problem.
Conclusion
Asking the right questions in a coding interview is a crucial skill that can significantly improve your problem-solving speed and accuracy. By clarifying assumptions, understanding constraints, and identifying potential edge cases upfront, you can avoid common pitfalls and focus on implementing the most appropriate solution.
Remember, interviewers are not just evaluating your coding skills but also your problem-solving approach and communication abilities. Demonstrating that you can ask insightful questions and gather relevant information effectively is a valuable trait that sets you apart as a strong candidate.
As you prepare for coding interviews, practice incorporating this question-asking strategy into your problem-solving routine. Start with the list of essential questions provided in this article and adapt them to each specific problem you encounter. With time and practice, you’ll develop the intuition to ask the most relevant questions quickly, setting yourself up for success in any coding interview scenario.
By mastering the art of asking the right questions, you’ll not only perform better in interviews but also develop a valuable skill that will serve you well throughout your programming career. After all, understanding the problem thoroughly is often half the battle in software development, and the ability to gather requirements effectively is essential for any successful programmer.