How to Approach System Design Questions with Confidence
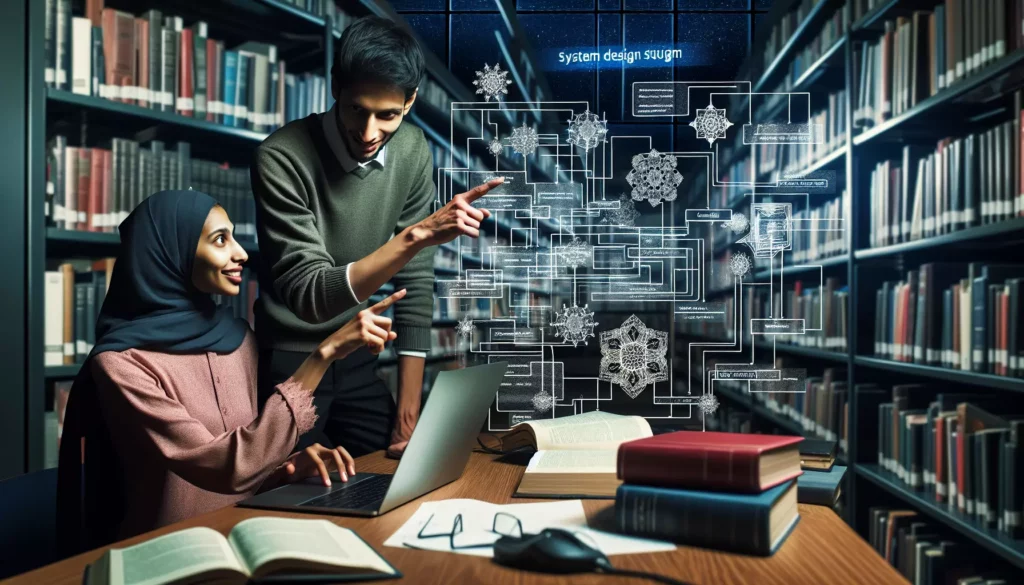
System design questions are a crucial component of technical interviews, especially for senior software engineering positions and roles at major tech companies. These questions assess a candidate’s ability to design large-scale distributed systems, which is an essential skill for building robust and scalable applications. In this comprehensive guide, we’ll explore strategies to approach system design questions with confidence, helping you ace your next technical interview.
Understanding the Importance of System Design Questions
Before diving into the strategies, it’s crucial to understand why system design questions are so important in technical interviews:
- Real-world application: System design closely mimics the challenges engineers face in their day-to-day work.
- Scalability assessment: These questions evaluate a candidate’s ability to design systems that can handle growth and increased load.
- Problem-solving skills: They test a candidate’s analytical thinking and ability to break down complex problems.
- Communication skills: System design interviews assess how well candidates can explain their thought process and decisions.
- Technical breadth: These questions cover a wide range of topics, from databases to networking and distributed systems.
The Four-Step Approach to System Design Questions
To tackle system design questions effectively, we recommend following a structured four-step approach:
1. Clarify Requirements and Scope
The first step in approaching any system design question is to clarify the requirements and scope of the problem. This involves asking questions to understand the system’s goals, constraints, and expected functionality.
- What are the core features of the system?
- What is the expected scale (number of users, data volume, etc.)?
- What are the performance requirements (latency, throughput)?
- Are there any specific constraints or assumptions we should consider?
Example dialogue:
Interviewer: "Design a URL shortening service like bit.ly."
You: "Sure, I'd be happy to design a URL shortening service. Before we begin, I'd like to clarify a few things:
1. What's the expected scale of the service? How many URLs do we expect to shorten per day?
2. Do we need to support custom short URLs?
3. What's the expected lifespan of a shortened URL?
4. Do we need to track any analytics, like click-through rates?"
Interviewer: "Good questions. Let's assume we need to handle about 100 million URLs per day. Custom short URLs are not required for now. Shortened URLs should be valid for at least 5 years. Basic analytics like click counts would be useful."
You: "Thank you for the clarification. This helps set the scope for our design."
2. Outline High-Level Design
Once you have a clear understanding of the requirements, sketch out a high-level design of the system. This should include the main components and their interactions.
- Identify the major components of the system
- Describe how these components interact with each other
- Discuss the flow of data through the system
For our URL shortening service example, a high-level design might include:
- Web servers to handle incoming requests
- Application servers to process URL shortening and redirection logic
- Database to store URL mappings
- Cache layer for faster retrieval of frequently accessed URLs
- Load balancer to distribute traffic across multiple servers
You might sketch out a diagram to illustrate this high-level design:
[User] <--> [Load Balancer] <--> [Web Servers] <--> [Application Servers] <--> [Cache]
^
|
v
[Database]
3. Deep Dive into Core Components
After outlining the high-level design, dive deeper into the core components of the system. This is where you can demonstrate your technical knowledge and problem-solving skills.
- Discuss the data model and database schema
- Explain the URL shortening algorithm
- Describe the caching strategy
- Address scalability and performance considerations
For our URL shortening service, we might discuss:
Data Model:
Table: shortened_urls
Columns:
- id: bigint (primary key)
- original_url: varchar(2048)
- short_code: varchar(10)
- created_at: timestamp
- expiration_date: timestamp
- click_count: int
URL Shortening Algorithm:
We could use a base62 encoding of the auto-incrementing ID to generate short codes. This allows for 62^7 (about 3.5 trillion) unique URLs with 7-character codes.
function generateShortCode(id) {
const base62Chars = "0123456789ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz";
let shortCode = "";
while (id > 0) {
shortCode = base62Chars[id % 62] + shortCode;
id = Math.floor(id / 62);
}
return shortCode.padStart(7, '0');
}
Caching Strategy:
We could use a distributed cache like Redis to store frequently accessed URL mappings. This would reduce the load on the database and improve response times.
Scalability Considerations:
- Use database sharding to distribute data across multiple database servers
- Implement a consistent hashing algorithm for cache node selection
- Use CDN for faster content delivery across different geographic regions
4. Discuss Trade-offs and Potential Improvements
In the final step, discuss any trade-offs in your design and potential areas for improvement. This demonstrates your ability to critically evaluate your own work and consider alternative approaches.
- Identify potential bottlenecks in the system
- Discuss alternative design choices and their pros/cons
- Suggest improvements or additional features
For our URL shortening service, we might discuss:
- Trade-off between using a longer short code (for more unique URLs) vs. shorter, more user-friendly codes
- Potential for collision in the URL shortening algorithm and strategies to mitigate it
- Balancing between cache size and database load
- Implementing a cleanup mechanism for expired URLs
- Adding security features like rate limiting to prevent abuse
Common Pitfalls to Avoid
When approaching system design questions, be aware of these common pitfalls:
- Jumping to implementation details too quickly: Focus on the high-level design first before diving into specifics.
- Ignoring scalability: Always consider how your system will handle growth and increased load.
- Overlooking edge cases: Consider scenarios like system failures, data consistency issues, and extreme load conditions.
- Poor time management: Allocate your time wisely across all aspects of the design.
- Not asking clarifying questions: Don’t hesitate to ask for more information or clarification when needed.
Essential System Design Concepts to Master
To excel in system design interviews, it’s crucial to have a solid understanding of fundamental concepts. Here are some key areas to focus on:
1. Scalability
Understand different scaling strategies:
- Vertical Scaling (Scale Up): Adding more power to an existing machine.
- Horizontal Scaling (Scale Out): Adding more machines to the system.
2. Load Balancing
Familiarize yourself with load balancing algorithms and their use cases:
- Round Robin
- Least Connections
- IP Hash
3. Caching
Understand different caching strategies and their applications:
- Write-through cache
- Write-back cache
- Cache eviction policies (LRU, LFU, FIFO)
4. Database Sharding
Learn about database sharding techniques:
- Horizontal partitioning
- Vertical partitioning
- Directory-based sharding
5. Consistency Models
Understand different consistency models in distributed systems:
- Strong consistency
- Eventual consistency
- CAP theorem
6. Message Queues
Learn about the role of message queues in distributed systems:
- Decoupling components
- Asynchronous processing
- Load leveling
Practice Makes Perfect
Like any skill, mastering system design takes practice. Here are some ways to improve your system design skills:
- Study existing systems: Analyze the architecture of popular services like Twitter, Netflix, or Uber.
- Design systems on your own: Practice designing systems for various scenarios, even if you’re not in an interview setting.
- Participate in open-source projects: Contributing to large-scale open-source projects can provide valuable experience in real-world system design.
- Read technical blogs and papers: Stay updated with the latest trends and best practices in system design.
- Discuss with peers: Engage in discussions about system design with colleagues or in online forums.
Conclusion
Approaching system design questions with confidence requires a combination of structured thinking, technical knowledge, and practice. By following the four-step approach outlined in this guide – clarifying requirements, outlining high-level design, diving deep into core components, and discussing trade-offs – you’ll be well-equipped to tackle even the most challenging system design questions.
Remember, the key to success in system design interviews is not just about arriving at the perfect solution, but demonstrating your problem-solving process, technical knowledge, and ability to make informed decisions under constraints. With consistent practice and a solid understanding of fundamental concepts, you can approach system design questions with confidence and ace your next technical interview.
Keep honing your skills, stay curious about new technologies and architectures, and don’t be afraid to think big when designing systems. Happy designing!