How to Approach String Manipulation Questions: A Comprehensive Guide
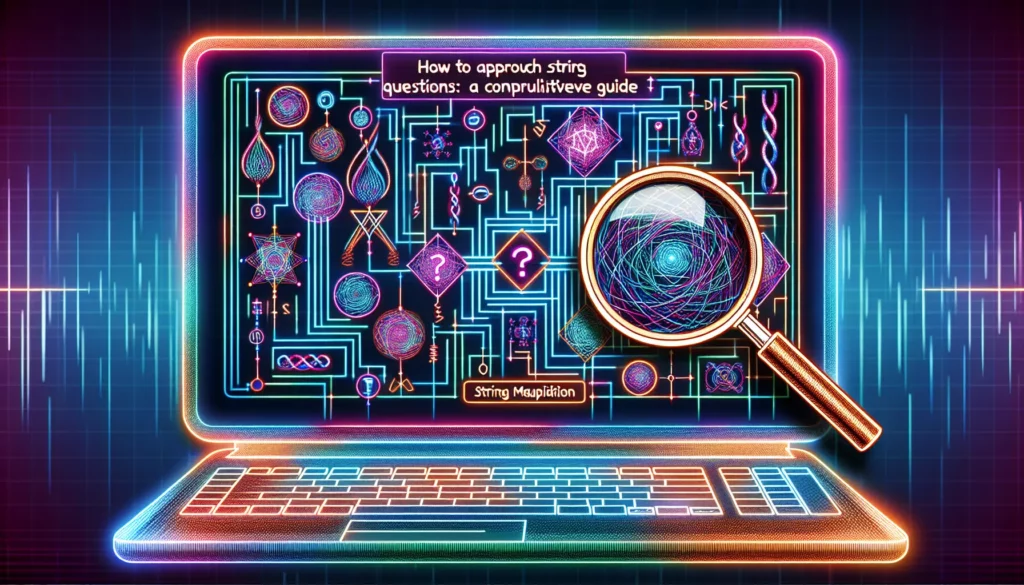
String manipulation is a fundamental skill in programming and a common topic in coding interviews, especially for positions at major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, and Google). Whether you’re a beginner learning to code or an experienced developer preparing for technical interviews, mastering string manipulation techniques is crucial. In this comprehensive guide, we’ll explore various approaches to tackle string manipulation questions effectively.
Understanding String Manipulation
Before diving into specific techniques, it’s essential to understand what string manipulation entails. String manipulation refers to the process of working with and modifying text data. This can include operations such as:
- Concatenating strings
- Extracting substrings
- Searching for patterns
- Replacing characters or substrings
- Reversing strings
- Changing case (uppercase/lowercase)
- Removing whitespace
- Splitting strings into arrays
These operations form the building blocks for solving more complex string manipulation problems.
Common String Manipulation Techniques
1. Two-Pointer Technique
The two-pointer technique is a powerful approach for many string manipulation problems. It involves using two pointers to traverse the string, often moving in opposite directions or at different speeds.
Example: Reversing a String
function reverseString(s) {
let left = 0;
let right = s.length - 1;
let chars = s.split("");
while (left < right) {
// Swap characters
[chars[left], chars[right]] = [chars[right], chars[left]];
left++;
right--;
}
return chars.join("");
}
console.log(reverseString("hello")); // Output: "olleh"
2. Sliding Window
The sliding window technique is useful for problems that involve finding subarrays or substrings that meet certain conditions. It involves maintaining a “window” that expands or contracts as you iterate through the string.
Example: Longest Substring Without Repeating Characters
function lengthOfLongestSubstring(s) {
let maxLength = 0;
let start = 0;
let charMap = new Map();
for (let end = 0; end < s.length; end++) {
if (charMap.has(s[end])) {
start = Math.max(start, charMap.get(s[end]) + 1);
}
charMap.set(s[end], end);
maxLength = Math.max(maxLength, end - start + 1);
}
return maxLength;
}
console.log(lengthOfLongestSubstring("abcabcbb")); // Output: 3
3. Hash Tables
Hash tables (or objects in JavaScript) are excellent for problems that involve counting characters, checking for anagrams, or storing character frequencies.
Example: Valid Anagram
function isAnagram(s, t) {
if (s.length !== t.length) return false;
const charCount = {};
for (let char of s) {
charCount[char] = (charCount[char] || 0) + 1;
}
for (let char of t) {
if (!charCount[char]) return false;
charCount[char]--;
}
return true;
}
console.log(isAnagram("anagram", "nagaram")); // Output: true
4. Stack
Stacks are useful for problems involving matching parentheses, backspace operations, or maintaining a history of operations.
Example: Valid Parentheses
function isValid(s) {
const stack = [];
const openBrackets = "({[";
const closeBrackets = ")}]";
const bracketPairs = {
")": "(",
"}": "{",
"]": "["
};
for (let char of s) {
if (openBrackets.includes(char)) {
stack.push(char);
} else if (closeBrackets.includes(char)) {
if (stack.pop() !== bracketPairs[char]) {
return false;
}
}
}
return stack.length === 0;
}
console.log(isValid("()[]{}")); // Output: true
Advanced String Manipulation Techniques
1. Regular Expressions
Regular expressions (regex) are powerful tools for pattern matching and text manipulation. They can significantly simplify complex string operations.
Example: Extracting Email Addresses
function extractEmails(text) {
const emailRegex = /[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}/g;
return text.match(emailRegex) || [];
}
const text = "Contact us at support@example.com or info@company.co.uk for more information.";
console.log(extractEmails(text));
// Output: ["support@example.com", "info@company.co.uk"]
2. Dynamic Programming
Dynamic programming can be applied to string manipulation problems that involve finding optimal substructures or require memoization to avoid redundant computations.
Example: Longest Palindromic Substring
function longestPalindrome(s) {
if (s.length < 2) return s;
let start = 0;
let maxLength = 1;
function expandAroundCenter(left, right) {
while (left >= 0 && right < s.length && s[left] === s[right]) {
const currentLength = right - left + 1;
if (currentLength > maxLength) {
start = left;
maxLength = currentLength;
}
left--;
right++;
}
}
for (let i = 0; i < s.length; i++) {
expandAroundCenter(i, i); // Odd-length palindromes
expandAroundCenter(i, i + 1); // Even-length palindromes
}
return s.substring(start, start + maxLength);
}
console.log(longestPalindrome("babad")); // Output: "bab" or "aba"
3. Trie Data Structure
Tries are efficient data structures for storing and searching strings, especially useful for problems involving prefix matching or autocomplete functionality.
Example: Implementing a Trie
class TrieNode {
constructor() {
this.children = {};
this.isEndOfWord = false;
}
}
class Trie {
constructor() {
this.root = new TrieNode();
}
insert(word) {
let node = this.root;
for (let char of word) {
if (!node.children[char]) {
node.children[char] = new TrieNode();
}
node = node.children[char];
}
node.isEndOfWord = true;
}
search(word) {
let node = this.root;
for (let char of word) {
if (!node.children[char]) {
return false;
}
node = node.children[char];
}
return node.isEndOfWord;
}
startsWith(prefix) {
let node = this.root;
for (let char of prefix) {
if (!node.children[char]) {
return false;
}
node = node.children[char];
}
return true;
}
}
const trie = new Trie();
trie.insert("apple");
console.log(trie.search("apple")); // Output: true
console.log(trie.search("app")); // Output: false
console.log(trie.startsWith("app")); // Output: true
Best Practices for Approaching String Manipulation Questions
1. Clarify the Problem
Before diving into coding, make sure you fully understand the problem requirements:
- Ask about input constraints (e.g., character set, string length)
- Clarify any ambiguities in the problem statement
- Discuss edge cases and expected behavior
2. Consider Time and Space Complexity
Always think about the efficiency of your solution:
- Analyze the time complexity of your algorithm
- Consider the space complexity, especially for large inputs
- Discuss potential trade-offs between time and space
3. Start with a Brute Force Approach
It’s often helpful to start with a simple, straightforward solution:
- Implement a naive approach to solve the problem
- Use this as a baseline for optimization
- Discuss the limitations of the brute force method
4. Optimize Your Solution
Look for ways to improve your initial solution:
- Identify redundant operations or calculations
- Consider using appropriate data structures (e.g., hash tables, tries)
- Apply relevant algorithms or techniques (e.g., two-pointer, sliding window)
5. Test Your Code
Always test your solution with various inputs:
- Start with simple test cases
- Include edge cases (e.g., empty string, single character)
- Consider large inputs to test for performance
6. Explain Your Approach
During an interview, communicate your thought process:
- Explain your reasoning for choosing a particular approach
- Discuss alternative solutions you considered
- Highlight the trade-offs of different approaches
Common String Manipulation Problems
To help you practice and apply these techniques, here are some common string manipulation problems you might encounter in coding interviews:
- Reverse a string
- Check if a string is a palindrome
- Find the longest substring without repeating characters
- Implement string compression
- Check if two strings are anagrams
- Find the first non-repeating character in a string
- Implement a basic string matching algorithm
- Convert a string to integer (atoi)
- Implement wildcard pattern matching
- Generate all permutations of a string
Conclusion
Mastering string manipulation is essential for success in coding interviews and real-world programming tasks. By understanding and applying the techniques discussed in this guide, you’ll be well-equipped to tackle a wide range of string-related problems.
Remember that practice is key to improving your skills. Regularly solve string manipulation problems, analyze different approaches, and strive to optimize your solutions. As you gain more experience, you’ll develop intuition for choosing the most appropriate technique for each problem.
Platforms like AlgoCademy offer interactive coding tutorials and resources specifically designed to help you improve your algorithmic thinking and problem-solving skills. Take advantage of these tools to practice string manipulation questions and prepare for technical interviews at top tech companies.
With dedication and consistent practice, you’ll be well-prepared to handle string manipulation questions in any coding interview or real-world programming scenario. Good luck with your coding journey!