How to Approach Refactoring Existing Code: A Comprehensive Guide
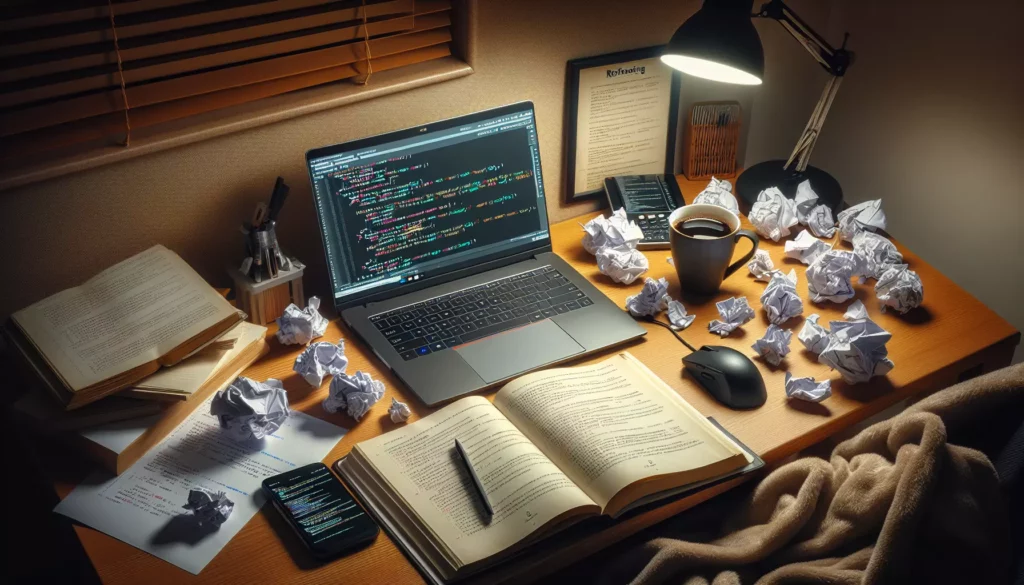
In the ever-evolving world of software development, maintaining and improving existing code is just as crucial as writing new code. This process, known as refactoring, is an essential skill for any programmer looking to enhance their abilities and create more efficient, readable, and maintainable software. In this comprehensive guide, we’ll explore the art of refactoring, discussing its importance, best practices, and how to approach it effectively.
What is Refactoring?
Refactoring is the process of restructuring existing code without changing its external behavior. The goal is to improve the code’s internal structure, making it easier to understand, maintain, and extend. This practice is crucial for several reasons:
- It improves code readability and reduces complexity
- It makes the code easier to maintain and update
- It can enhance performance and efficiency
- It helps in identifying and fixing bugs
- It facilitates the addition of new features
When Should You Refactor?
Knowing when to refactor is just as important as knowing how to refactor. Here are some signs that indicate it’s time to consider refactoring:
- Code duplication: If you find yourself copying and pasting code frequently, it’s a sign that you need to refactor to create reusable components or functions.
- Long methods or functions: Methods that are too long are often difficult to understand and maintain. Breaking them down into smaller, more focused functions can improve readability and maintainability.
- Large classes: Classes that have too many responsibilities violate the Single Responsibility Principle. Consider breaking them down into smaller, more focused classes.
- Frequent changes in the same area: If you find yourself constantly modifying the same piece of code, it might be a sign that the code is not flexible enough and needs refactoring.
- Difficulty in adding new features: If adding new features becomes increasingly difficult, it’s often a sign that the code structure needs improvement.
- Poor performance: If certain parts of your code are causing performance issues, refactoring can help optimize and improve efficiency.
The Refactoring Process
Now that we understand what refactoring is and when to do it, let’s dive into the process of refactoring. Here’s a step-by-step approach to guide you through the refactoring process:
1. Identify the Code to Refactor
The first step is to identify which parts of your code need refactoring. This could be based on the signs mentioned earlier, such as code duplication, long methods, or large classes. Tools like code analyzers and linters can help identify potential areas for improvement.
2. Ensure Proper Test Coverage
Before you start refactoring, it’s crucial to have a solid set of unit tests in place. These tests will serve as a safety net, ensuring that your refactoring efforts don’t introduce new bugs or change the existing functionality. If you don’t have adequate test coverage, write tests for the code you plan to refactor before making any changes.
3. Make Small, Incremental Changes
Refactoring should be done in small, manageable steps. This approach minimizes the risk of introducing errors and makes it easier to track and revert changes if necessary. After each small change, run your tests to ensure everything still works as expected.
4. Use Refactoring Techniques
There are many established refactoring techniques you can use. Some common ones include:
- Extract Method: Breaking down a large method into smaller, more focused methods
- Rename Method: Giving methods more descriptive names to improve readability
- Move Method: Moving a method to a more appropriate class
- Extract Class: Creating a new class from parts of an existing class
- Inline Method: Replacing a method call with the method’s content
- Replace Conditional with Polymorphism: Using polymorphism instead of complex conditional statements
5. Review and Test
After making changes, review your code to ensure it meets your quality standards. Run all tests again to verify that the functionality remains intact. It’s also a good practice to have your refactored code reviewed by peers.
6. Document Changes
Keep track of the changes you’ve made during the refactoring process. This documentation can be helpful for other developers who might work on the code in the future, and it can also serve as a reference for you if you need to revert changes or understand why certain decisions were made.
Best Practices for Refactoring
To make your refactoring efforts more effective, consider these best practices:
1. Follow the Boy Scout Rule
The Boy Scout Rule states that you should “leave the campground cleaner than you found it.” In the context of coding, this means always leaving the code a little better than you found it. Whenever you work on a piece of code, take a moment to make small improvements, even if they’re not directly related to your current task.
2. Use Version Control
Always use version control when refactoring. This allows you to easily track changes, revert if necessary, and collaborate with other developers. Make frequent, small commits with descriptive messages to maintain a clear history of your refactoring efforts.
3. Leverage IDE Features
Modern Integrated Development Environments (IDEs) offer powerful refactoring tools that can automate many common refactoring tasks. Familiarize yourself with these features to save time and reduce the risk of introducing errors.
4. Follow SOLID Principles
The SOLID principles are a set of five design principles that can guide you in creating more maintainable and extensible code. Keep these principles in mind when refactoring:
- Single Responsibility Principle (SRP)
- Open-Closed Principle (OCP)
- Liskov Substitution Principle (LSP)
- Interface Segregation Principle (ISP)
- Dependency Inversion Principle (DIP)
5. Maintain Coding Standards
Ensure that your refactored code adheres to your team’s coding standards and style guidelines. Consistency in code style across the project makes it easier for all team members to read and understand the code.
6. Communicate with Your Team
If you’re working on a team project, communicate your refactoring plans with your teammates. This helps avoid conflicts and ensures that everyone is on the same page regarding the changes being made.
Common Refactoring Scenarios
Let’s look at some common scenarios where refactoring can significantly improve code quality, along with examples of how to approach them:
1. Removing Code Duplication
Code duplication is a common issue that can lead to maintenance problems. Here’s an example of how you might refactor duplicated code:
Before refactoring:
function calculateAreaOfSquare(side) {
return side * side;
}
function calculateAreaOfRectangle(length, width) {
return length * width;
}
function calculateAreaOfCircle(radius) {
return Math.PI * radius * radius;
}
After refactoring:
function calculateArea(shape, ...dimensions) {
switch(shape) {
case 'square':
return dimensions[0] * dimensions[0];
case 'rectangle':
return dimensions[0] * dimensions[1];
case 'circle':
return Math.PI * dimensions[0] * dimensions[0];
default:
throw new Error('Unsupported shape');
}
}
2. Breaking Down Long Methods
Long methods can be difficult to understand and maintain. Here’s an example of breaking down a long method:
Before refactoring:
function processOrder(order) {
// Validate order
if (!order.items || order.items.length === 0) {
throw new Error('Order must have at least one item');
}
if (!order.shippingAddress) {
throw new Error('Shipping address is required');
}
// Calculate total
let total = 0;
for (let item of order.items) {
total += item.price * item.quantity;
}
// Apply discount
if (order.discountCode) {
let discount = getDiscountAmount(order.discountCode);
total -= discount;
}
// Calculate tax
let tax = total * 0.1; // Assume 10% tax rate
total += tax;
// Process payment
let paymentResult = processPayment(order.paymentDetails, total);
if (!paymentResult.success) {
throw new Error('Payment failed: ' + paymentResult.error);
}
// Create shipment
createShipment(order.items, order.shippingAddress);
return {
orderId: generateOrderId(),
total: total,
status: 'Processed'
};
}
After refactoring:
function processOrder(order) {
validateOrder(order);
let total = calculateTotal(order.items);
total = applyDiscount(total, order.discountCode);
total = addTax(total);
processPayment(order.paymentDetails, total);
createShipment(order.items, order.shippingAddress);
return createOrderSummary(total);
}
function validateOrder(order) {
if (!order.items || order.items.length === 0) {
throw new Error('Order must have at least one item');
}
if (!order.shippingAddress) {
throw new Error('Shipping address is required');
}
}
function calculateTotal(items) {
return items.reduce((total, item) => total + item.price * item.quantity, 0);
}
function applyDiscount(total, discountCode) {
if (discountCode) {
let discount = getDiscountAmount(discountCode);
return total - discount;
}
return total;
}
function addTax(total) {
return total * 1.1; // Assume 10% tax rate
}
function processPayment(paymentDetails, total) {
let paymentResult = processPayment(paymentDetails, total);
if (!paymentResult.success) {
throw new Error('Payment failed: ' + paymentResult.error);
}
}
function createOrderSummary(total) {
return {
orderId: generateOrderId(),
total: total,
status: 'Processed'
};
}
3. Simplifying Complex Conditionals
Complex conditional statements can make code hard to read and maintain. Here’s an example of simplifying a complex conditional:
Before refactoring:
function calculateDiscount(customer, orderTotal) {
if (customer.type === 'premium' && orderTotal > 100) {
return 0.1;
} else if (customer.type === 'premium' && orderTotal <= 100) {
return 0.05;
} else if (customer.type === 'regular' && orderTotal > 200) {
return 0.05;
} else if (customer.type === 'regular' && orderTotal <= 200 && orderTotal > 100) {
return 0.02;
} else {
return 0;
}
}
After refactoring:
function calculateDiscount(customer, orderTotal) {
const discountRules = [
{ type: 'premium', minOrder: 100, discount: 0.1 },
{ type: 'premium', minOrder: 0, discount: 0.05 },
{ type: 'regular', minOrder: 200, discount: 0.05 },
{ type: 'regular', minOrder: 100, discount: 0.02 },
{ type: 'regular', minOrder: 0, discount: 0 }
];
const rule = discountRules.find(rule =>
customer.type === rule.type && orderTotal > rule.minOrder
);
return rule ? rule.discount : 0;
}
Tools for Refactoring
While refactoring can be done manually, there are several tools available that can assist in the process:
1. IDE Refactoring Tools
Most modern IDEs come with built-in refactoring tools. These can automate many common refactoring tasks, such as renaming variables, extracting methods, and moving classes. Some popular IDEs with strong refactoring support include:
- IntelliJ IDEA (for Java)
- Visual Studio (for .NET languages)
- PyCharm (for Python)
- WebStorm (for JavaScript)
2. Static Code Analysis Tools
These tools analyze your code without executing it and can identify potential issues and areas for improvement. Some popular options include:
- SonarQube
- ESLint (for JavaScript)
- ReSharper (for .NET)
- Pylint (for Python)
3. Version Control Systems
While not specifically refactoring tools, version control systems like Git are essential for managing code changes during refactoring. They allow you to track changes, revert if necessary, and collaborate with team members.
4. Refactoring Browsers
These are specialized tools designed specifically for refactoring. While less common than IDE-integrated tools, they can be powerful for large-scale refactoring efforts. An example is the Refactoring Browser for Smalltalk.
Challenges in Refactoring
While refactoring is a valuable practice, it’s not without its challenges. Here are some common obstacles you might face and how to overcome them:
1. Time Constraints
Refactoring can be time-consuming, and in fast-paced development environments, it might be challenging to allocate time for it. To address this:
- Integrate refactoring into your regular development process
- Practice the Boy Scout Rule: always leave code a little better than you found it
- Prioritize refactoring tasks based on their impact and urgency
2. Risk of Introducing Bugs
There’s always a risk of introducing new bugs when modifying existing code. To mitigate this:
- Ensure comprehensive test coverage before refactoring
- Make small, incremental changes and test after each change
- Use automated refactoring tools when possible to reduce human error
3. Resistance from Team Members or Management
Sometimes, team members or management might resist refactoring efforts, viewing them as unnecessary or risky. To address this:
- Educate your team about the benefits of refactoring
- Demonstrate the impact of refactoring on code quality and development speed
- Start with small, low-risk refactoring efforts to build confidence
4. Large Legacy Codebases
Refactoring large, legacy codebases can be particularly challenging. To tackle this:
- Start with the most critical or frequently changed parts of the codebase
- Use the “Strangler Fig” pattern to gradually replace old code with new, refactored code
- Implement comprehensive testing before refactoring legacy code
Measuring the Success of Refactoring
To ensure that your refactoring efforts are effective, it’s important to measure their impact. Here are some ways to gauge the success of your refactoring:
1. Code Metrics
Use code quality metrics to measure improvements. Some useful metrics include:
- Cyclomatic complexity
- Lines of code
- Duplicated code percentage
- Code coverage
2. Performance Metrics
If performance improvement was a goal of your refactoring, measure relevant performance metrics such as:
- Execution time
- Memory usage
- Database query times
3. Development Speed
Track how refactoring impacts development speed:
- Time to implement new features
- Time to fix bugs
- Time spent on maintenance tasks
4. Developer Feedback
Collect feedback from developers working with the refactored code:
- Is the code easier to understand?
- Is it easier to add new features or fix bugs?
- Has the overall developer experience improved?
Conclusion
Refactoring is a crucial skill for any developer looking to improve code quality, maintainability, and efficiency. By following the approach and best practices outlined in this guide, you can effectively refactor existing code, leading to more robust and sustainable software projects.
Remember, refactoring is an ongoing process. It’s not about achieving perfection, but about continuous improvement. As you gain more experience with refactoring, you’ll develop a keen eye for code smells and opportunities for improvement, allowing you to write better code from the start and maintain high-quality codebases over time.
Whether you’re working on a personal project or as part of a large development team, incorporating refactoring into your regular development process will pay dividends in the long run. It will not only improve your code but also enhance your skills as a developer, making you more valuable in the ever-evolving field of software development.