How to Approach Problems That Have Multiple Solutions in Programming
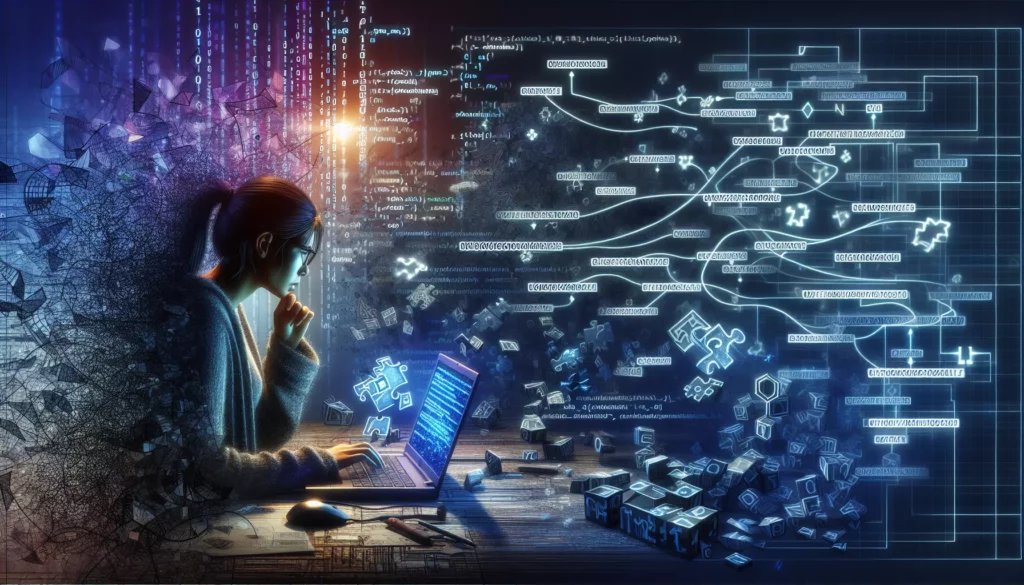
In the world of programming and software development, encountering problems with multiple solutions is a common occurrence. As aspiring developers or seasoned professionals, it’s crucial to develop the skills to navigate these scenarios effectively. This article will explore various strategies and techniques to approach problems that have multiple solutions, helping you become a more versatile and efficient problem-solver.
Understanding the Nature of Multi-Solution Problems
Before diving into specific approaches, it’s essential to understand why some problems have multiple solutions:
- Complexity: Complex problems often have various ways to break them down and solve them.
- Trade-offs: Different solutions may prioritize different aspects like performance, readability, or memory usage.
- Context: The best solution can vary depending on the specific context or requirements of the project.
- Creativity: Programming allows for creative problem-solving, leading to diverse approaches.
Step 1: Analyze the Problem Thoroughly
The first step in approaching any problem, especially those with multiple solutions, is to analyze it thoroughly:
- Understand the requirements: Clearly define what the problem is asking and what constraints exist.
- Identify input and output: Determine what data you’re working with and what results are expected.
- Consider edge cases: Think about potential extreme or unusual scenarios that your solution needs to handle.
- Break it down: Divide the problem into smaller, manageable sub-problems if possible.
Step 2: Brainstorm Multiple Approaches
Once you have a solid understanding of the problem, it’s time to brainstorm different approaches:
- Think creatively: Don’t limit yourself to the first solution that comes to mind.
- Consider different paradigms: Explore solutions using various programming paradigms (e.g., procedural, object-oriented, functional).
- Draw from past experiences: Recall similar problems you’ve solved before and how those solutions might apply.
- Research: Look up common algorithms or design patterns that might be relevant to your problem.
Step 3: Evaluate Each Approach
After generating multiple potential solutions, it’s crucial to evaluate each one:
- Time complexity: Analyze how the solution’s performance scales with input size.
- Space complexity: Consider the memory requirements of each approach.
- Readability and maintainability: Assess how easy the code will be to understand and modify in the future.
- Scalability: Determine if the solution can handle growth in data or user base.
- Testability: Consider how easily you can write unit tests for the solution.
Step 4: Implement a Solution
After evaluating your options, choose the most appropriate solution and implement it:
- Start with a basic implementation: Begin with a simple version that solves the core problem.
- Test as you go: Write unit tests to ensure your implementation works correctly.
- Refactor and optimize: Once you have a working solution, look for ways to improve it.
- Document your code: Add comments and documentation to explain your approach and any important decisions.
Step 5: Reflect and Learn
After implementing your chosen solution, take time to reflect on the process:
- Compare with other solutions: If possible, look at how others solved the same problem.
- Analyze trade-offs: Understand what you gained and what you sacrificed with your chosen approach.
- Consider alternatives: Think about how you might solve the problem differently in the future.
- Learn from the experience: Identify any new techniques or concepts you learned during the process.
Common Techniques for Approaching Multi-Solution Problems
Here are some specific techniques that can be helpful when dealing with problems that have multiple solutions:
1. Divide and Conquer
The divide and conquer approach involves breaking down a complex problem into smaller, more manageable sub-problems. This technique can be particularly useful when dealing with large-scale problems that seem overwhelming at first glance.
Example: Implementing a sorting algorithm like Merge Sort
def merge_sort(arr):
if len(arr) <= 1:
return arr
mid = len(arr) // 2
left = merge_sort(arr[:mid])
right = merge_sort(arr[mid:])
return merge(left, right)
def merge(left, right):
result = []
i, j = 0, 0
while i < len(left) and j < len(right):
if left[i] < right[j]:
result.append(left[i])
i += 1
else:
result.append(right[j])
j += 1
result.extend(left[i:])
result.extend(right[j:])
return result
2. Dynamic Programming
Dynamic programming is a method for solving complex problems by breaking them down into simpler subproblems. It’s particularly useful for optimization problems and can often provide efficient solutions to problems with overlapping subproblems.
Example: Calculating Fibonacci numbers
def fibonacci(n):
if n <= 1:
return n
fib = [0] * (n + 1)
fib[1] = 1
for i in range(2, n + 1):
fib[i] = fib[i-1] + fib[i-2]
return fib[n]
3. Greedy Algorithms
Greedy algorithms make the locally optimal choice at each step with the hope of finding a global optimum. While they don’t always produce the best solution, they can be efficient and effective for certain types of problems.
Example: Making change with the fewest coins
def make_change(amount, coins):
coins.sort(reverse=True)
change = []
for coin in coins:
while amount >= coin:
change.append(coin)
amount -= coin
return change
4. Brute Force
While often considered inefficient, brute force approaches can be useful for smaller problem sizes or as a starting point for more optimized solutions. They involve systematically enumerating all possible candidates for the solution and checking whether each candidate satisfies the problem statement.
Example: Finding all permutations of a string
def permutations(s):
if len(s) <= 1:
return [s]
perms = []
for i, char in enumerate(s):
for perm in permutations(s[:i] + s[i+1:]):
perms.append(char + perm)
return perms
5. Recursion
Recursive solutions involve a function calling itself to solve smaller instances of the same problem. This approach can lead to elegant and concise solutions for problems that have a natural recursive structure.
Example: Calculating factorial
def factorial(n):
if n == 0 or n == 1:
return 1
return n * factorial(n - 1)
Case Study: Solving the Longest Common Subsequence Problem
Let’s apply the concepts we’ve discussed to a real-world problem: finding the Longest Common Subsequence (LCS) of two strings. This problem has multiple solution approaches, each with its own trade-offs.
Problem Statement:
Given two sequences, find the length of the longest subsequence present in both of them. A subsequence is a sequence that appears in the same relative order, but not necessarily contiguous.
Approach 1: Recursive Solution
This approach uses recursion to break down the problem into smaller subproblems.
def lcs_recursive(X, Y, m, n):
if m == 0 or n == 0:
return 0
elif X[m-1] == Y[n-1]:
return 1 + lcs_recursive(X, Y, m-1, n-1)
else:
return max(lcs_recursive(X, Y, m, n-1), lcs_recursive(X, Y, m-1, n))
# Usage
X = "ABCDGH"
Y = "AEDFHR"
print(lcs_recursive(X, Y, len(X), len(Y)))
Pros: Simple to understand and implement.
Cons: Inefficient for large inputs due to redundant recursive calls.
Approach 2: Dynamic Programming Solution
This approach uses a table to store intermediate results, avoiding redundant calculations.
def lcs_dp(X, Y):
m, n = len(X), len(Y)
L = [[0] * (n + 1) for _ in range(m + 1)]
for i in range(1, m + 1):
for j in range(1, n + 1):
if X[i-1] == Y[j-1]:
L[i][j] = L[i-1][j-1] + 1
else:
L[i][j] = max(L[i-1][j], L[i][j-1])
return L[m][n]
# Usage
X = "ABCDGH"
Y = "AEDFHR"
print(lcs_dp(X, Y))
Pros: Efficient for large inputs, avoids redundant calculations.
Cons: Uses more memory than the recursive solution.
Approach 3: Dynamic Programming with Space Optimization
This approach optimizes the space usage of the dynamic programming solution.
def lcs_dp_optimized(X, Y):
m, n = len(X), len(Y)
L = [0] * (n + 1)
for i in range(1, m + 1):
prev = 0
for j in range(1, n + 1):
temp = L[j]
if X[i-1] == Y[j-1]:
L[j] = prev + 1
else:
L[j] = max(L[j], L[j-1])
prev = temp
return L[n]
# Usage
X = "ABCDGH"
Y = "AEDFHR"
print(lcs_dp_optimized(X, Y))
Pros: Efficient in both time and space complexity.
Cons: Slightly more complex to understand and implement.
Comparing the Approaches
- Time Complexity:
- Recursive: O(2^n) in the worst case
- Dynamic Programming: O(mn)
- Optimized DP: O(mn)
- Space Complexity:
- Recursive: O(min(m,n)) due to the call stack
- Dynamic Programming: O(mn)
- Optimized DP: O(min(m,n))
- Readability:
- Recursive: Most intuitive and easy to understand
- Dynamic Programming: Requires understanding of DP concept
- Optimized DP: Most complex to understand
In this case study, we see how different approaches to the same problem can have varying trade-offs in terms of time complexity, space complexity, and readability. The choice of which solution to use would depend on the specific requirements of the project, such as input size, memory constraints, and the need for code maintainability.
Conclusion
Approaching problems with multiple solutions is a skill that improves with practice and experience. By following a structured approach of analysis, brainstorming, evaluation, implementation, and reflection, you can develop the ability to choose the most appropriate solution for any given problem.
Remember that there’s rarely a one-size-fits-all solution in programming. The “best” solution often depends on the specific context, constraints, and requirements of your project. As you continue to solve diverse problems and explore different approaches, you’ll build a toolkit of strategies and techniques that will make you a more effective problem-solver.
Keep challenging yourself with new problems, study different algorithms and data structures, and don’t be afraid to explore multiple solutions to the same problem. With time and practice, you’ll develop the intuition to quickly identify and implement optimal solutions, making you a more versatile and valuable programmer.