How to Approach Optimization Problems in Coding Interviews
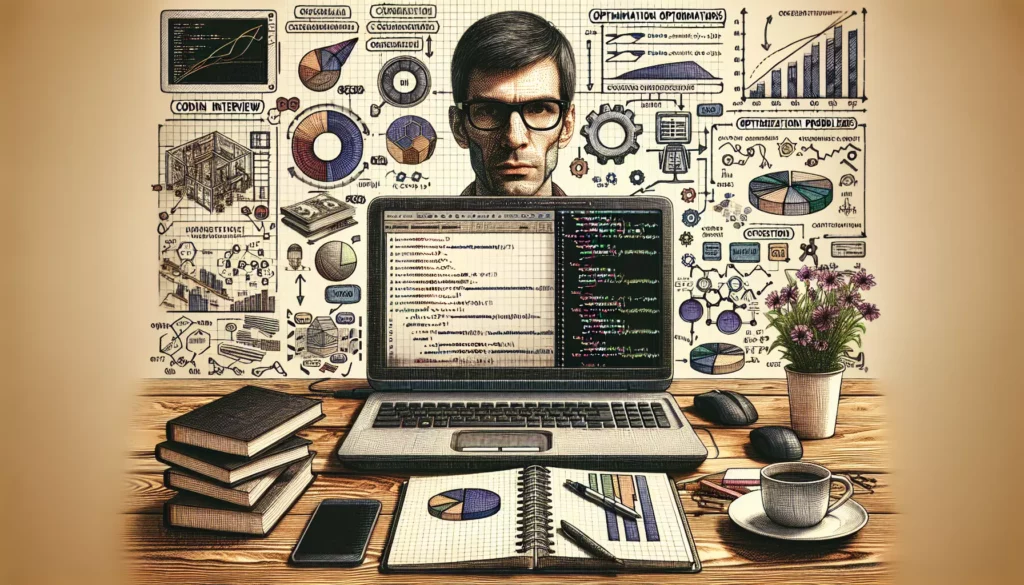
When it comes to coding interviews, particularly those at major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), optimization problems are a common and crucial component. These problems test a candidate’s ability to not only solve a given problem but to do so in the most efficient manner possible. In this comprehensive guide, we’ll explore how to approach optimization problems in coding interviews, providing you with strategies, techniques, and practical examples to help you excel.
Understanding Optimization Problems
Before diving into the strategies for tackling optimization problems, it’s essential to understand what they are and why they’re important in coding interviews.
What are Optimization Problems?
Optimization problems in coding interviews are tasks that require you to find the most efficient solution to a given problem. This efficiency can be measured in terms of:
- Time complexity: How quickly the algorithm runs
- Space complexity: How much memory the algorithm uses
- Code readability: How clear and maintainable the code is
The goal is to balance these factors to create a solution that is not only correct but also performs well under various conditions and constraints.
Why are Optimization Problems Important?
Optimization problems are crucial in coding interviews for several reasons:
- They test your problem-solving skills and ability to think critically.
- They demonstrate your understanding of data structures and algorithms.
- They showcase your ability to write efficient and scalable code.
- They reflect real-world scenarios where optimization is often necessary for large-scale applications.
Strategies for Approaching Optimization Problems
Now that we understand the importance of optimization problems, let’s explore some strategies for tackling them effectively in coding interviews.
1. Understand the Problem Thoroughly
Before jumping into coding, make sure you have a clear understanding of the problem. This involves:
- Carefully reading the problem statement
- Identifying the input and expected output
- Clarifying any ambiguities with the interviewer
- Considering edge cases and constraints
Taking the time to fully grasp the problem will help you avoid misunderstandings and save time in the long run.
2. Start with a Brute Force Solution
Begin by developing a simple, straightforward solution to the problem. This approach, often called the brute force method, may not be the most efficient, but it serves several purposes:
- It demonstrates that you can solve the problem
- It provides a baseline for optimization
- It helps you understand the problem better
Once you have a working brute force solution, you can analyze its time and space complexity and look for areas to optimize.
3. Analyze the Current Solution
After implementing the brute force solution, take a step back and analyze it. Consider the following questions:
- What is the time complexity of the current solution?
- What is the space complexity?
- Are there any redundant operations or unnecessary data structures?
- Can any parts of the algorithm be improved or simplified?
This analysis will help you identify areas for optimization and guide your approach to improving the solution.
4. Consider Alternative Data Structures and Algorithms
One of the most effective ways to optimize a solution is to consider using different data structures or algorithms. Ask yourself:
- Can a more efficient data structure improve time or space complexity?
- Is there a well-known algorithm that solves this type of problem more efficiently?
- Can preprocessing or caching help reduce repeated computations?
For example, using a hash table instead of an array can often reduce time complexity from O(n) to O(1) for lookup operations.
5. Apply Common Optimization Techniques
There are several common optimization techniques that you can apply to many problems:
- Two-pointer technique: Useful for array-based problems
- Sliding window: Efficient for substring or subarray problems
- Dynamic programming: Helps solve problems with overlapping subproblems
- Memoization: Caches results of expensive function calls
- Binary search: Reduces search space in sorted arrays
Familiarize yourself with these techniques and practice applying them to various problems.
6. Implement and Test the Optimized Solution
Once you’ve identified potential optimizations, implement the improved solution. As you code, keep the following in mind:
- Maintain code readability and clarity
- Use meaningful variable and function names
- Add comments to explain complex logic
- Test your solution with various inputs, including edge cases
Remember, a highly optimized but unreadable or buggy solution is not ideal. Strive for a balance between efficiency and maintainability.
7. Analyze and Explain the Optimization
After implementing the optimized solution, be prepared to explain your thought process and the improvements you’ve made. This involves:
- Comparing the time and space complexity of the optimized solution to the original
- Explaining the trade-offs you’ve made (if any)
- Discussing any potential further optimizations
Clear communication about your optimization process is crucial in coding interviews.
Practical Example: Two Sum Problem
Let’s walk through a practical example of optimizing a common coding interview problem: the Two Sum problem.
Problem Statement:
Given an array of integers nums
and an integer target
, return indices of the two numbers in the array such that they add up to the target
. You may assume that each input would have exactly one solution, and you may not use the same element twice.
Brute Force Solution:
Let’s start with a simple brute force approach:
def two_sum_brute_force(nums, target):
for i in range(len(nums)):
for j in range(i + 1, len(nums)):
if nums[i] + nums[j] == target:
return [i, j]
return [] # No solution found
This solution has a time complexity of O(n^2) and a space complexity of O(1).
Optimized Solution:
Now, let’s optimize this solution using a hash table:
def two_sum_optimized(nums, target):
num_dict = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_dict:
return [num_dict[complement], i]
num_dict[num] = i
return [] # No solution found
This optimized solution has a time complexity of O(n) and a space complexity of O(n).
Explanation of Optimization:
In the optimized solution, we use a hash table (dictionary in Python) to store each number and its index as we iterate through the array. For each number, we calculate its complement (target – num) and check if this complement exists in our hash table. If it does, we’ve found our pair and can return their indices.
This approach reduces the time complexity from O(n^2) to O(n) because we only need to iterate through the array once. The trade-off is that we use additional space (O(n)) to store the hash table, but this is generally considered a worthwhile trade-off for the significant improvement in time complexity.
Common Optimization Techniques in Detail
Let’s delve deeper into some common optimization techniques that can be applied to various coding interview problems.
1. Two-Pointer Technique
The two-pointer technique involves using two pointers to iterate through a data structure, often moving in opposite directions or at different speeds. This technique is particularly useful for array-based problems and can often reduce time complexity from O(n^2) to O(n).
Example: Reverse a String
def reverse_string(s):
left, right = 0, len(s) - 1
while left < right:
s[left], s[right] = s[right], s[left]
left += 1
right -= 1
return s
This solution has a time complexity of O(n) and a space complexity of O(1).
2. Sliding Window
The sliding window technique is used to perform operations on a specific window of elements in an array or string. It’s particularly useful for problems involving subarrays or substrings.
Example: Find the maximum sum subarray of size k
def max_sum_subarray(arr, k):
n = len(arr)
if n < k:
return None
window_sum = sum(arr[:k])
max_sum = window_sum
for i in range(k, n):
window_sum = window_sum - arr[i-k] + arr[i]
max_sum = max(max_sum, window_sum)
return max_sum
This solution has a time complexity of O(n) and a space complexity of O(1).
3. Dynamic Programming
Dynamic programming is a method for solving complex problems by breaking them down into simpler subproblems. It’s especially useful for optimization problems with overlapping subproblems.
Example: Fibonacci Sequence
def fibonacci(n):
if n <= 1:
return n
dp = [0] * (n + 1)
dp[1] = 1
for i in range(2, n + 1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
This solution has a time complexity of O(n) and a space complexity of O(n).
4. Memoization
Memoization is a technique used in dynamic programming where we store the results of expensive function calls and return the cached result when the same inputs occur again.
Example: Fibonacci Sequence with Memoization
def fibonacci_memo(n, memo={}):
if n in memo:
return memo[n]
if n <= 1:
return n
memo[n] = fibonacci_memo(n-1, memo) + fibonacci_memo(n-2, memo)
return memo[n]
This solution has a time complexity of O(n) and a space complexity of O(n).
5. Binary Search
Binary search is an efficient algorithm for searching a sorted array by repeatedly dividing the search interval in half. It reduces the search space by half in each step, resulting in a logarithmic time complexity.
Example: Search in Sorted Array
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1 # Target not found
This solution has a time complexity of O(log n) and a space complexity of O(1).
Tips for Mastering Optimization in Coding Interviews
To excel at optimization problems in coding interviews, consider the following tips:
- Practice regularly: Solve a variety of problems and focus on optimizing your solutions.
- Study time and space complexity: Develop a strong understanding of Big O notation and how to analyze algorithms.
- Learn common patterns: Familiarize yourself with problem-solving patterns and when to apply them.
- Analyze multiple solutions: For each problem, try to come up with multiple solutions and compare their efficiency.
- Understand trade-offs: Recognize that optimization often involves trade-offs between time and space complexity.
- Communicate clearly: Practice explaining your thought process and optimization strategies out loud.
- Stay updated: Keep up with new algorithms and data structures in computer science.
- Review real-world scenarios: Study how optimization is applied in real software systems.
Conclusion
Mastering optimization problems is a crucial skill for succeeding in coding interviews, especially for positions at top tech companies. By understanding the importance of optimization, learning key strategies and techniques, and practicing regularly, you can significantly improve your ability to tackle these challenging problems.
Remember that optimization is not just about finding the most efficient solution, but also about balancing efficiency with readability and maintainability. As you prepare for coding interviews, focus on developing a systematic approach to problem-solving and optimization. With time and practice, you’ll be well-equipped to handle even the most complex optimization challenges in your coding interviews.
Keep in mind that the journey to mastering optimization is ongoing. Continue to challenge yourself with new problems, explore different approaches, and stay curious about advancements in algorithms and data structures. With dedication and perseverance, you’ll be well on your way to excelling in coding interviews and building a successful career in software development.