How to Approach Open-Ended Design Questions: A Comprehensive Guide
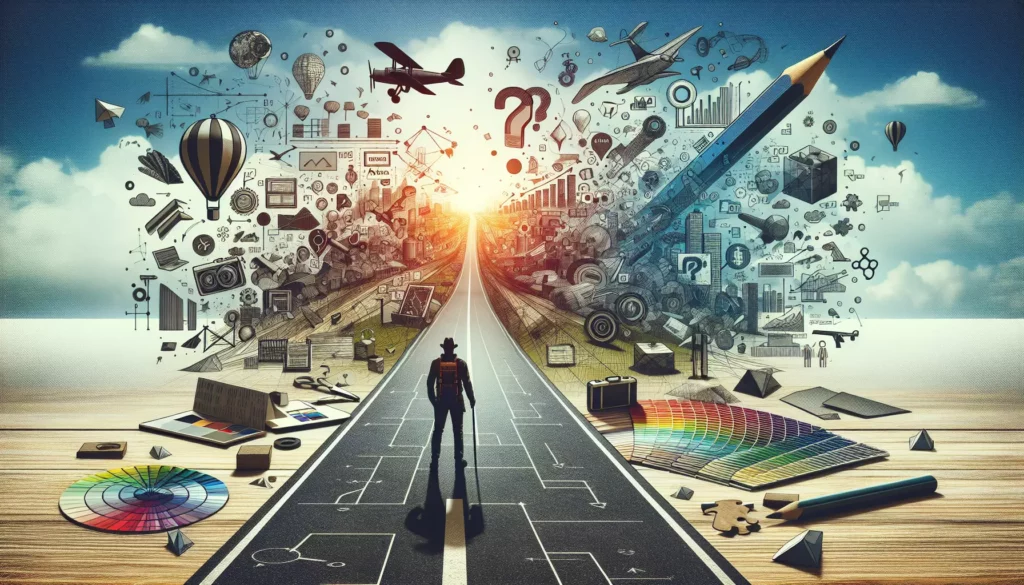
In the world of software engineering and technical interviews, particularly for positions at major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), open-ended design questions are a crucial component of the assessment process. These questions are designed to evaluate a candidate’s ability to think critically, solve complex problems, and communicate effectively. In this comprehensive guide, we’ll explore strategies and techniques to help you approach open-ended design questions with confidence and skill.
Understanding Open-Ended Design Questions
Open-ended design questions are intentionally broad and ambiguous. They often ask candidates to design a system, application, or feature without providing specific requirements or constraints. The goal is to assess how well you can:
- Gather and clarify requirements
- Break down complex problems into manageable components
- Consider various trade-offs and make informed decisions
- Communicate your thought process and rationale
- Adapt to new information or constraints introduced during the discussion
Examples of open-ended design questions might include:
- Design a URL shortening service like bit.ly
- Create a system for a parking lot
- Design a social media platform’s news feed
- Develop an elevator system for a skyscraper
The STAR Approach to Open-Ended Design Questions
To tackle open-ended design questions effectively, we recommend using the STAR approach:
- Scope the problem
- Think about the components
- Analyze the trade-offs
- Review and refine
Let’s dive into each of these steps in detail.
1. Scope the Problem
The first step in approaching an open-ended design question is to clearly define the scope of the problem. This involves asking clarifying questions to understand the requirements, constraints, and expectations of the system you’re designing.
Key questions to ask during this phase include:
- What are the primary use cases for this system?
- Who are the target users?
- What is the expected scale of the system (e.g., number of users, data volume)?
- Are there any specific performance requirements or SLAs (Service Level Agreements)?
- What are the key features that must be included in the initial design?
- Are there any regulatory or compliance requirements to consider?
By asking these questions, you demonstrate your ability to gather requirements and show that you’re not making assumptions about the problem. This also helps you focus your design efforts on the most critical aspects of the system.
Example: Scoping a URL Shortening Service
Let’s say you’re asked to design a URL shortening service. Here’s how you might scope the problem:
Interviewer: "Design a URL shortening service like bit.ly."
You: "Certainly! Before we dive into the design, I'd like to ask a few questions to clarify the requirements:
1. What's the expected scale of the service? How many URLs do we expect to shorten per day?
2. Do we need to support custom short URLs, or will they all be randomly generated?
3. Is there a requirement for analytics, such as tracking the number of clicks on each shortened URL?
4. Do we need to consider any security features, like preventing malicious URLs?
5. Are there any specific latency requirements for generating and resolving shortened URLs?
6. Do we need to support link expiration or the ability to delete shortened URLs?"
Interviewer: "Great questions! Let's assume:
- We expect to shorten about 1 million URLs per day.
- We'll support both random and custom short URLs.
- Basic analytics (click counting) should be included.
- We should have a simple mechanism to prevent obviously malicious URLs.
- The service should generate and resolve URLs quickly, ideally under 100ms.
- We don't need link expiration for now, but the ability to delete URLs would be useful."
You: "Thank you for clarifying. Based on these requirements, I'll design a system that can handle high volume, support custom URLs, include basic analytics, incorporate simple security measures, and prioritize low latency for URL generation and resolution."
By scoping the problem in this way, you’ve set a clear foundation for your design and demonstrated your ability to gather and clarify requirements.
2. Think About the Components
Once you’ve scoped the problem, the next step is to break down the system into its core components. This involves identifying the main building blocks of your design and how they interact with each other.
Key aspects to consider during this phase include:
- High-level architecture
- Data models and storage
- APIs and interfaces
- Key algorithms or processes
- Scalability considerations
It’s often helpful to start with a simple diagram or flowchart to visualize the system components and their interactions.
Example: Components of a URL Shortening Service
Continuing with our URL shortening service example, here’s how you might break down the components:
You: "Based on our requirements, I propose the following high-level components for our URL shortening service:
1. Web Server: Handles incoming requests for URL shortening and redirection.
2. Application Server: Contains the core logic for generating short URLs and managing redirects.
3. Database: Stores the mapping between short URLs and original URLs, along with analytics data.
4. Cache: Improves performance by storing frequently accessed URL mappings.
5. Analytics Service: Tracks and updates click counts for shortened URLs.
6. URL Validation Service: Checks submitted URLs for potential security issues.
Let me sketch out a basic diagram to show how these components interact."
[At this point, you would draw a simple diagram showing the flow of requests through the system]
"Now, let's dive into each component:
1. Web Server:
- Nginx or Apache to handle HTTP requests
- Load balancer to distribute traffic across multiple application servers
2. Application Server:
- RESTful API endpoints for URL shortening, redirection, and management
- URL shortening algorithm (we'll discuss this in more detail)
- Custom URL validation and conflict resolution
3. Database:
- We'll use a relational database like PostgreSQL for its ACID properties
- Main table structure:
- id: bigint (primary key)
- short_url: varchar(10) (indexed)
- long_url: text
- user_id: bigint (if we implement user accounts)
- created_at: timestamp
- click_count: bigint
4. Cache:
- Redis for in-memory caching of frequently accessed URL mappings
- Improves read performance for popular links
5. Analytics Service:
- Separate service to handle click tracking asynchronously
- Uses a message queue (e.g., RabbitMQ) to process analytics updates
6. URL Validation Service:
- Checks submitted URLs against known malicious site lists
- Performs basic syntax validation
For the URL shortening algorithm, we'll use a base62 encoding of an auto-incrementing ID. This allows for short, unique URLs while maintaining a simple, scalable approach."
Interviewer: "That's a good start. Can you elaborate on how you'd handle custom URLs and ensure uniqueness?"
You: "Certainly! For custom URLs, we'll add an additional field to our database table:
- is_custom: boolean
When a user requests a custom URL, we'll first check if it's available. If it is, we'll set is_custom to true and use the provided short URL. If not, we'll return an error and ask the user to choose a different custom URL.
To ensure uniqueness for both custom and generated URLs, we'll use a combination of database constraints and application-level checks:
1. Add a unique constraint on the short_url column in the database.
2. Before inserting a new URL (custom or generated), we'll attempt to insert it with a unique constraint.
3. If the insertion fails due to a constraint violation, we'll either:
a) For custom URLs: Return an error to the user.
b) For generated URLs: Generate a new short URL and try again.
This approach allows us to handle race conditions and ensure uniqueness even under high concurrency."
By thinking through the components in this way, you demonstrate your ability to design a complex system and consider various technical aspects of the implementation.
3. Analyze the Trade-offs
After outlining the components of your system, it’s crucial to analyze the trade-offs inherent in your design decisions. This step shows your ability to think critically about the implications of different choices and make informed decisions based on the specific requirements of the problem.
Key areas to consider when analyzing trade-offs include:
- Performance vs. cost
- Consistency vs. availability
- Simplicity vs. flexibility
- Security vs. usability
- Scalability considerations
Be prepared to discuss alternative approaches and explain why you chose one solution over another.
Example: Analyzing Trade-offs in the URL Shortening Service
Continuing with our URL shortening service example, here’s how you might analyze some of the trade-offs in your design:
You: "Let's analyze some of the key trade-offs in our URL shortening service design:
1. Database Choice: PostgreSQL vs. NoSQL
- We chose PostgreSQL for its ACID properties and strong consistency, which are important for ensuring unique short URLs and accurate analytics.
- Trade-off: While NoSQL databases like Cassandra might offer better write scalability, we prioritized data integrity and chose to handle scalability through other means (e.g., sharding if necessary).
2. Caching Strategy: Redis vs. Application-level caching
- We opted for Redis as an external cache to improve read performance for popular URLs.
- Trade-off: This adds complexity to our infrastructure but provides better scalability and faster access times compared to application-level caching.
3. URL Shortening Algorithm: Base62 encoding vs. Random string generation
- We chose base62 encoding of an auto-incrementing ID for its simplicity and guaranteed uniqueness.
- Trade-off: This approach may make it easier to guess sequential URLs, but it's more efficient and scalable than generating and checking random strings for collisions.
4. Analytics Processing: Real-time vs. Batch processing
- We decided to use a message queue for asynchronous analytics updates to reduce the impact on the main application's performance.
- Trade-off: This introduces a slight delay in analytics data but significantly improves the system's ability to handle high traffic volumes.
5. Custom URL Support: Allow vs. Disallow
- We chose to support custom URLs to enhance user experience and branding opportunities.
- Trade-off: This increases complexity in URL generation and storage, and may require additional measures to prevent abuse or squatting on popular terms.
6. Security Measures: Basic checks vs. Advanced threat detection
- We implemented basic URL validation and checks against known malicious sites.
- Trade-off: While more advanced threat detection could provide better security, it would increase complexity and potentially impact performance. Our approach balances security with simplicity and performance.
7. Scalability Approach: Vertical vs. Horizontal scaling
- Our design allows for horizontal scaling of web and application servers to handle increased load.
- Trade-off: This approach is more complex to manage but provides better scalability and fault tolerance compared to vertical scaling.
These trade-offs were made considering our requirements for high performance, scalability, and basic security while maintaining a relatively simple system design. Depending on future needs, we can adjust these decisions to prioritize different aspects of the system."
Interviewer: "That's a good analysis. How would you handle the potential issue of hot keys in your cache for extremely popular URLs?"
You: "Excellent point. Hot keys in the cache for very popular URLs could indeed become a bottleneck. Here's how we could address this issue:
1. Cache Sharding: We can implement cache sharding based on the short URL. This distributes popular URLs across multiple Redis instances, reducing the load on any single cache server.
2. Multi-level Caching: Implement a two-level caching strategy:
- L1 Cache: An application-level cache (e.g., using Caffeine in Java) for the most frequently accessed URLs.
- L2 Cache: Our existing Redis cache.
This approach reduces the load on Redis for extremely popular URLs.
3. Cache-aside with TTL: Implement a cache-aside strategy with a relatively short Time-To-Live (TTL) for cached entries. This ensures that even if a URL becomes suddenly popular, the load is distributed over time as the cache entries expire and are refreshed.
4. Read-through Cache with Write-behind: For extremely popular URLs, we could implement a read-through cache with write-behind updates for click counts. This allows us to update analytics in batches, reducing database write pressure.
5. Intelligent Caching: Implement an algorithm that dynamically adjusts caching strategies based on URL popularity. For instance, extremely popular URLs could have longer cache durations or be pinned in memory.
6. CDN Integration: For the most popular URLs, we could integrate a Content Delivery Network (CDN) to handle redirects directly, bypassing our application servers entirely for these specific cases.
The choice between these strategies would depend on the specific patterns of URL popularity we observe in production. We'd likely start with cache sharding and the two-level caching approach, then monitor performance and adjust as needed."
By analyzing trade-offs in this manner, you demonstrate your ability to think critically about design decisions and consider their implications on various aspects of the system.
4. Review and Refine
The final step in approaching open-ended design questions is to review your design and refine it based on any new information or insights gained during the discussion. This phase demonstrates your ability to iterate on your ideas and incorporate feedback.
Key aspects of the review and refine phase include:
- Summarizing your design
- Identifying potential bottlenecks or weak points
- Discussing how the system could evolve or scale in the future
- Addressing any concerns or questions raised by the interviewer
- Proposing improvements or alternative approaches
Example: Reviewing and Refining the URL Shortening Service Design
Let’s conclude our URL shortening service example with a review and refinement phase:
You: "Now that we've discussed the components and trade-offs of our URL shortening service, let's review the design and consider some refinements:
Summary of the current design:
1. Web and application servers handle URL shortening and redirection requests.
2. PostgreSQL database stores URL mappings and analytics data.
3. Redis cache improves read performance for popular URLs.
4. Asynchronous analytics processing via a message queue.
5. Basic URL validation for security.
6. Support for both generated and custom short URLs.
Potential improvements and considerations for scaling:
1. Database Sharding: As our data grows, we may need to implement database sharding to distribute the load. We could shard based on the first few characters of the short URL.
2. Read Replicas: Implement read replicas of our PostgreSQL database to handle increased read traffic, especially for analytics queries.
3. Geo-distribution: To reduce latency for users in different regions, we could deploy our service across multiple geographical locations, using DNS-based load balancing to route users to the nearest datacenter.
4. Enhanced Analytics: Expand our analytics capabilities to include features like geographic distribution of clicks, referrer tracking, and time-based click patterns.
5. Rate Limiting: Implement rate limiting on our API to prevent abuse and ensure fair usage of the service.
6. URL Expiration: Add support for URL expiration, allowing users to set an expiration date for their shortened URLs.
7. User Accounts: Introduce user accounts to provide personalized dashboards, URL management, and premium features.
8. Monitoring and Alerting: Implement comprehensive monitoring and alerting systems to track system health, performance metrics, and unusual patterns that might indicate security issues.
9. A/B Testing Framework: Build an A/B testing framework to easily test and roll out new features or optimizations.
10. Improved Security: Enhance our security measures with more advanced malicious URL detection, potentially integrating with third-party security services.
These refinements would allow our system to scale effectively, improve performance and reliability, and add valuable features for our users. The specific priorities for these improvements would depend on user feedback, observed usage patterns, and business goals.
Is there any particular aspect of the design you'd like me to elaborate on further?"
Interviewer: "This is a comprehensive review. One last question: how would you handle data consistency if you implemented database sharding?"
You: "Excellent question. Maintaining data consistency across shards is indeed a challenge. Here's how we could approach it:
1. Consistent Hashing: Use consistent hashing to determine which shard a particular URL belongs to. This ensures that even as we add or remove shards, most URLs will still map to the same shard.
2. Distributed Transactions: For operations that might span multiple shards (e.g., checking if a custom URL is available across all shards), we could use a two-phase commit protocol. However, this can be slow, so we'd use it sparingly.
3. Eventual Consistency Model: For less critical operations, like updating click counts, we could adopt an eventual consistency model. Updates would be applied to the primary shard first, then propagated to other shards asynchronously.
4. Centralized Metadata Service: Maintain a separate metadata service that keeps track of shard locations and handles the routing of requests to the appropriate shard.
5. Read-Your-Writes Consistency: Ensure that after a write operation, subsequent reads reflect that write, even if it hasn't been propagated to all shards yet. This can be achieved by routing reads to the primary shard for a short period after a write.
6. Quorum-based Approach: For critical read operations, we could implement a quorum-based approach where we read from multiple shards and return the most up-to-date result.
7. Conflict Resolution: Implement conflict resolution mechanisms for cases where inconsistencies are detected. This could involve choosing the most recent update based on timestamps or using more complex merge algorithms.
8. Regular Consistency Checks: Run background jobs to detect and resolve any inconsistencies across shards.
9. Transactional Outbox Pattern: For operations that need to update both the database and send messages (e.g., updating click counts and sending to the analytics service), use the transactional outbox pattern to ensure consistency between database updates and message publishing.
The specific approach would depend on our exact consistency requirements and the types of operations we're performing. We'd likely use a combination of these techniques to balance consistency, performance, and complexity."
By reviewing and refining your design in this way, you demonstrate your ability to think holistically about the system, consider future improvements, and address complex technical challenges.
Additional Tips for Tackling Open-Ended Design Questions
In addition to following the STAR approach, here are some additional tips to help you excel at open-ended design questions:
- Practice, practice, practice: Regularly work on system design problems to build your skills and confidence.
- Stay up-to-date with technology: Keep abreast of current trends and best practices in system design and architecture.
- Learn from existing systems: Study the architectures of popular services and platforms to understand how they solve complex problems.
- Communicate clearly: Explain your thought process clearly and concisely. Use diagrams to illustrate your ideas when appropriate.
- Be open to feedback: Listen carefully to the interviewer’s questions and be willing to adapt your design based on new information or constraints.
- Consider non-functional requirements: Don’t forget about aspects like security, maintainability, and monitoring when designing your system.
- Start simple and iterate: Begin with a basic design that solves the core problem, then iterate and add complexity as needed.
- Justify your decisions: Be prepared to explain the reasoning behind your design choices and discuss alternatives.
- Think about edge cases: Consider how your system will handle unusual situations or extreme scenarios.
- Be honest about trade-offs: Acknowledge the limitations of your design and be prepared to discuss how you might address them.
Conclusion
Open-ended design questions are a crucial component of technical interviews, especially for positions at major tech companies. By following the STAR approach (Scope, Think, Analyze, Review) and incorporating the additional tips provided, you can approach these questions with confidence and demonstrate your ability to design complex systems.
Remember that the goal of these questions is not necessarily to arrive at a perfect solution, but rather to showcase your problem-solving skills, technical knowledge, and ability to communicate complex ideas effectively. With practice and a structured approach, you can excel at open-ended design questions and improve your chances of success in technical interviews.
Keep honing your skills, stay curious about system design and architecture, and don’t hesitate to dive deep into the intricacies of building scalable, efficient, and robust systems. Your journey in mastering open-ended design questions is an ongoing process that will serve you well throughout your career in software engineering.