How to Approach Matrix Manipulation Problems: A Comprehensive Guide
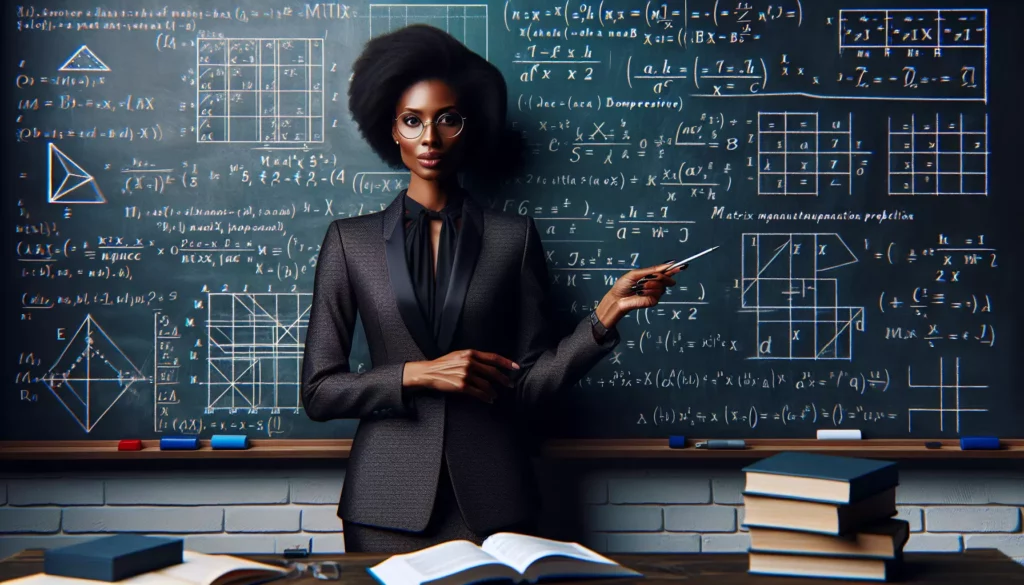
Matrix manipulation problems are a common sight in coding interviews, especially for positions at major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google). These problems test a candidate’s ability to work with 2D arrays, understand spatial relationships, and implement efficient algorithms. In this comprehensive guide, we’ll explore various techniques and strategies to tackle matrix manipulation problems effectively.
Table of Contents
- Understanding Matrices in Programming
- Common Matrix Operations
- Matrix Traversal Techniques
- In-place Matrix Manipulation
- Search Algorithms for Matrices
- Dynamic Programming on Matrices
- Graph Problems on Matrices
- Optimization Techniques
- Practice Problems and Solutions
- Interview Tips for Matrix Problems
1. Understanding Matrices in Programming
Before diving into matrix manipulation problems, it’s crucial to have a solid understanding of how matrices are represented in programming languages. In most languages, matrices are implemented as 2D arrays or lists of lists.
Representation in Different Languages
Java:
int[][] matrix = new int[rows][columns];
Python:
matrix = [[0 for j in range(columns)] for i in range(rows)]
C++:
vector<vector<int>> matrix(rows, vector<int>(columns));
Accessing and Modifying Elements
To access or modify an element in a matrix, you typically use two indices:
// Accessing an element
int element = matrix[row][column];
// Modifying an element
matrix[row][column] = newValue;
Understanding this basic structure is crucial for solving matrix manipulation problems efficiently.
2. Common Matrix Operations
Familiarizing yourself with common matrix operations will give you a solid foundation for tackling more complex problems. Here are some operations you should be comfortable with:
Matrix Transposition
Transposing a matrix means switching its rows and columns. Here’s a simple implementation:
def transpose(matrix):
rows, cols = len(matrix), len(matrix[0])
transposed = [[0 for _ in range(rows)] for _ in range(cols)]
for i in range(rows):
for j in range(cols):
transposed[j][i] = matrix[i][j]
return transposed
Matrix Rotation
Rotating a matrix is a common interview question. Here’s how to rotate a matrix 90 degrees clockwise:
def rotate90Clockwise(matrix):
n = len(matrix)
# Transpose the matrix
for i in range(n):
for j in range(i, n):
matrix[i][j], matrix[j][i] = matrix[j][i], matrix[i][j]
# Reverse each row
for i in range(n):
matrix[i].reverse()
return matrix
Matrix Addition and Multiplication
While less common in interviews, understanding these operations can be helpful:
def matrix_add(A, B):
return [[A[i][j] + B[i][j] for j in range(len(A[0]))] for i in range(len(A))]
def matrix_multiply(A, B):
return [[sum(a*b for a,b in zip(row,col)) for col in zip(*B)] for row in A]
3. Matrix Traversal Techniques
Efficient traversal of matrices is crucial for many problems. Here are some common traversal patterns:
Row-wise and Column-wise Traversal
def row_wise_traversal(matrix):
for row in matrix:
for element in row:
print(element, end=' ')
print()
def column_wise_traversal(matrix):
for j in range(len(matrix[0])):
for i in range(len(matrix)):
print(matrix[i][j], end=' ')
print()
Diagonal Traversal
def diagonal_traversal(matrix):
rows, cols = len(matrix), len(matrix[0])
for d in range(rows + cols - 1):
for i in range(max(0, d-cols+1), min(d+1, rows)):
j = d - i
print(matrix[i][j], end=' ')
print()
Spiral Traversal
Spiral traversal is a popular interview question. Here’s an implementation:
def spiral_traversal(matrix):
result = []
if not matrix: return result
top, bottom = 0, len(matrix) - 1
left, right = 0, len(matrix[0]) - 1
while top <= bottom and left <= right:
# Traverse right
for j in range(left, right + 1):
result.append(matrix[top][j])
top += 1
# Traverse down
for i in range(top, bottom + 1):
result.append(matrix[i][right])
right -= 1
if top <= bottom:
# Traverse left
for j in range(right, left - 1, -1):
result.append(matrix[bottom][j])
bottom -= 1
if left <= right:
# Traverse up
for i in range(bottom, top - 1, -1):
result.append(matrix[i][left])
left += 1
return result
4. In-place Matrix Manipulation
In-place manipulation of matrices is an important skill, especially when dealing with space complexity constraints. Here are some techniques:
Using Extra Variables
Sometimes, you can use a few extra variables to perform in-place operations. For example, rotating a matrix in-place:
def rotate_in_place(matrix):
n = len(matrix)
for i in range(n // 2):
for j in range(i, n - i - 1):
temp = matrix[i][j]
matrix[i][j] = matrix[n-1-j][i]
matrix[n-1-j][i] = matrix[n-1-i][n-1-j]
matrix[n-1-i][n-1-j] = matrix[j][n-1-i]
matrix[j][n-1-i] = temp
return matrix
Using Bitwise XOR
For problems involving swapping elements, you can use XOR to swap without using an extra variable:
def swap_without_temp(a, b):
a = a ^ b
b = a ^ b
a = a ^ b
return a, b
Reversing Rows or Columns
Many matrix problems can be solved by reversing rows or columns in-place:
def reverse_rows(matrix):
for row in matrix:
row.reverse()
return matrix
def reverse_columns(matrix):
for j in range(len(matrix[0])):
i, k = 0, len(matrix) - 1
while i < k:
matrix[i][j], matrix[k][j] = matrix[k][j], matrix[i][j]
i += 1
k -= 1
return matrix
5. Search Algorithms for Matrices
Efficient search algorithms are crucial for many matrix problems. Here are some common search techniques:
Binary Search on Sorted Matrix
If a matrix is sorted (either row-wise or column-wise), you can use binary search:
def search_sorted_matrix(matrix, target):
if not matrix or not matrix[0]:
return False
rows, cols = len(matrix), len(matrix[0])
left, right = 0, rows * cols - 1
while left <= right:
mid = (left + right) // 2
mid_value = matrix[mid // cols][mid % cols]
if mid_value == target:
return True
elif mid_value < target:
left = mid + 1
else:
right = mid - 1
return False
Search in Row-wise and Column-wise Sorted Matrix
For matrices sorted both row-wise and column-wise, you can use this efficient search method:
def search_row_col_sorted(matrix, target):
if not matrix or not matrix[0]:
return False
rows, cols = len(matrix), len(matrix[0])
row, col = 0, cols - 1
while row < rows and col >= 0:
if matrix[row][col] == target:
return True
elif matrix[row][col] > target:
col -= 1
else:
row += 1
return False
6. Dynamic Programming on Matrices
Dynamic Programming (DP) is a powerful technique for solving optimization problems on matrices. Here are some common DP patterns for matrix problems:
2D DP Table
Many matrix DP problems involve creating a 2D DP table. Here’s an example of finding the maximum square submatrix of 1s:
def max_square_submatrix(matrix):
if not matrix or not matrix[0]:
return 0
rows, cols = len(matrix), len(matrix[0])
dp = [[0] * (cols + 1) for _ in range(rows + 1)]
max_side = 0
for i in range(1, rows + 1):
for j in range(1, cols + 1):
if matrix[i-1][j-1] == 1:
dp[i][j] = min(dp[i-1][j], dp[i][j-1], dp[i-1][j-1]) + 1
max_side = max(max_side, dp[i][j])
return max_side * max_side
Prefix Sum Matrix
Prefix sum matrices are useful for quickly calculating the sum of any rectangular submatrix:
def create_prefix_sum_matrix(matrix):
rows, cols = len(matrix), len(matrix[0])
prefix_sum = [[0] * (cols + 1) for _ in range(rows + 1)]
for i in range(1, rows + 1):
for j in range(1, cols + 1):
prefix_sum[i][j] = (prefix_sum[i-1][j] + prefix_sum[i][j-1]
- prefix_sum[i-1][j-1] + matrix[i-1][j-1])
return prefix_sum
def sum_region(prefix_sum, row1, col1, row2, col2):
return (prefix_sum[row2+1][col2+1] - prefix_sum[row1][col2+1]
- prefix_sum[row2+1][col1] + prefix_sum[row1][col1])
7. Graph Problems on Matrices
Many graph problems can be represented and solved using matrices. Here are some common patterns:
DFS on Matrix
Depth-First Search (DFS) can be used to solve problems like finding connected components or paths in a matrix:
def dfs_matrix(matrix, i, j, visited):
if (i < 0 or i >= len(matrix) or
j < 0 or j >= len(matrix[0]) or
visited[i][j] or matrix[i][j] == 0):
return
visited[i][j] = True
# Visit all 4 adjacent cells
directions = [(0, 1), (1, 0), (0, -1), (-1, 0)]
for di, dj in directions:
dfs_matrix(matrix, i + di, j + dj, visited)
def find_connected_components(matrix):
if not matrix or not matrix[0]:
return 0
rows, cols = len(matrix), len(matrix[0])
visited = [[False] * cols for _ in range(rows)]
count = 0
for i in range(rows):
for j in range(cols):
if matrix[i][j] == 1 and not visited[i][j]:
dfs_matrix(matrix, i, j, visited)
count += 1
return count
BFS on Matrix
Breadth-First Search (BFS) is useful for problems involving shortest paths or level-order traversals:
from collections import deque
def bfs_matrix(matrix, start_i, start_j):
rows, cols = len(matrix), len(matrix[0])
visited = [[False] * cols for _ in range(rows)]
queue = deque([(start_i, start_j)])
visited[start_i][start_j] = True
directions = [(0, 1), (1, 0), (0, -1), (-1, 0)]
while queue:
i, j = queue.popleft()
print(f"Visited: ({i}, {j})")
for di, dj in directions:
ni, nj = i + di, j + dj
if (0 <= ni < rows and 0 <= nj < cols and
not visited[ni][nj] and matrix[ni][nj] == 1):
queue.append((ni, nj))
visited[ni][nj] = True
# Example usage
matrix = [
[1, 0, 1, 1],
[1, 1, 1, 0],
[0, 1, 0, 1],
[1, 1, 1, 1]
]
bfs_matrix(matrix, 0, 0)
8. Optimization Techniques
When working with large matrices, optimization becomes crucial. Here are some techniques to improve the efficiency of your matrix algorithms:
Space Optimization
Sometimes, you can reduce the space complexity by using only a single row or column instead of the entire matrix. For example, in the problem of finding the maximum sum submatrix, you can optimize space like this:
def max_sum_submatrix(matrix):
if not matrix or not matrix[0]:
return 0
rows, cols = len(matrix), len(matrix[0])
max_sum = float('-inf')
for left in range(cols):
temp = [0] * rows
for right in range(left, cols):
for i in range(rows):
temp[i] += matrix[i][right]
current_sum = kadane(temp)
max_sum = max(max_sum, current_sum)
return max_sum
def kadane(arr):
max_sum = current_sum = arr[0]
for num in arr[1:]:
current_sum = max(num, current_sum + num)
max_sum = max(max_sum, current_sum)
return max_sum
Bit Manipulation
For certain problems, especially those involving binary matrices, bit manipulation can lead to significant optimizations:
def count_submatrices_all_ones(matrix):
if not matrix or not matrix[0]:
return 0
rows, cols = len(matrix), len(matrix[0])
result = 0
for i in range(rows):
row_mask = (1 << cols) - 1
for j in range(i, rows):
row_mask &= int(''.join(map(str, matrix[j])), 2)
result += bin(row_mask).count('1')
return result
Matrix Compression
For sparse matrices, you can use compression techniques to save space and improve performance:
class SparseMatrix:
def __init__(self, matrix):
self.sparse = {}
for i in range(len(matrix)):
for j in range(len(matrix[0])):
if matrix[i][j] != 0:
self.sparse[(i, j)] = matrix[i][j]
def get(self, i, j):
return self.sparse.get((i, j), 0)
def set(self, i, j, value):
if value != 0:
self.sparse[(i, j)] = value
elif (i, j) in self.sparse:
del self.sparse[(i, j)]
# Example usage
matrix = [
[0, 0, 3, 0],
[0, 2, 0, 0],
[1, 0, 0, 4]
]
sparse_matrix = SparseMatrix(matrix)
print(sparse_matrix.get(0, 2)) # Output: 3
print(sparse_matrix.get(1, 1)) # Output: 2
sparse_matrix.set(1, 1, 0)
print(sparse_matrix.get(1, 1)) # Output: 0
9. Practice Problems and Solutions
To solidify your understanding of matrix manipulation, here are some practice problems with brief solutions:
Problem 1: Island Perimeter
Given a 2D grid map of 1s (land) and 0s (water), count the perimeter of the island.
def island_perimeter(grid):
perimeter = 0
rows, cols = len(grid), len(grid[0])
for i in range(rows):
for j in range(cols):
if grid[i][j] == 1:
perimeter += 4
if i > 0 and grid[i-1][j] == 1:
perimeter -= 2
if j > 0 and grid[i][j-1] == 1:
perimeter -= 2
return perimeter
Problem 2: Set Matrix Zeroes
Given a matrix, if an element is 0, set its entire row and column to 0. Do it in-place.
def set_zeroes(matrix):
rows, cols = len(matrix), len(matrix[0])
first_row_zero = False
first_col_zero = False
# Check if first row contains zero
for j in range(cols):
if matrix[0][j] == 0:
first_row_zero = True
break
# Check if first column contains zero
for i in range(rows):
if matrix[i][0] == 0:
first_col_zero = True
break
# Use first row and column as markers
for i in range(1, rows):
for j in range(1, cols):
if matrix[i][j] == 0:
matrix[i][0] = 0
matrix[0][j] = 0
# Set zeros based on markers
for i in range(1, rows):
for j in range(1, cols):
if matrix[i][0] == 0 or matrix[0][j] == 0:
matrix[i][j] = 0
# Set first row to zero if needed
if first_row_zero:
for j in range(cols):
matrix[0][j] = 0
# Set first column to zero if needed
if first_col_zero:
for i in range(rows):
matrix[i][0] = 0
Problem 3: Longest Increasing Path in a Matrix
Given an integer matrix, find the length of the longest increasing path.
def longest_increasing_path(matrix):
if not matrix or not matrix[0]:
return 0
rows, cols = len(matrix), len(matrix[0])
dp = [[0] * cols for _ in range(rows)]
def dfs(i, j):
if dp[i][j] != 0:
return dp[i][j]
directions = [(0, 1), (1, 0), (0, -1), (-1, 0)]
max_length = 1
for di, dj in directions:
ni, nj = i + di, j + dj
if (0 <= ni < rows and 0 <= nj < cols and
matrix[ni][nj] > matrix[i][j]):
max_length = max(max_length, 1 + dfs(ni, nj))
dp[i][j] = max_length
return max_length
return max(dfs(i, j) for i in range(rows) for j in range(cols))
10. Interview Tips for Matrix Problems
When tackling matrix problems in interviews, keep these tips in mind:
- Clarify the Input: Always ask about the dimensions of the matrix and any constraints on the values.
- Consider Edge Cases: Think about empty matrices, single-row or single-column matrices, and matrices with all identical elements.
- Visualize the Problem: Draw out small examples to help understand the problem and explain your approach.
- Start Simple: Begin with a brute-force solution, then optimize. It’s better to have a working solution than no solution at all.
- Think About Space Complexity: If asked to solve in-place, consider using the input matrix itself for storage.
- Use Standard Techniques: Many matrix problems can be solved using DFS, BFS, DP, or two-pointer techniques.
- Practice Common Patterns: Familiarize yourself with spiral traversal, diagonal traversal, and matrix rotation.
- Optimize Incrementally: If your initial solution is not optimal, explain how you would improve it step by step.
- Test Your Solution: Before declaring you’re done, mentally walk through your code with a small example.
- Communicate Clearly: Explain your thought process, including why you chose certain approaches over others.
By mastering these techniques and practicing regularly, you’ll be well-prepared to tackle matrix manipulation problems in coding interviews. Remember, the key is not just to solve the problem, but to demonstrate your problem-solving process and ability to optimize solutions.