How to Approach Coding Problems With Fresh Eyes (Even If You’ve Seen Them Before)
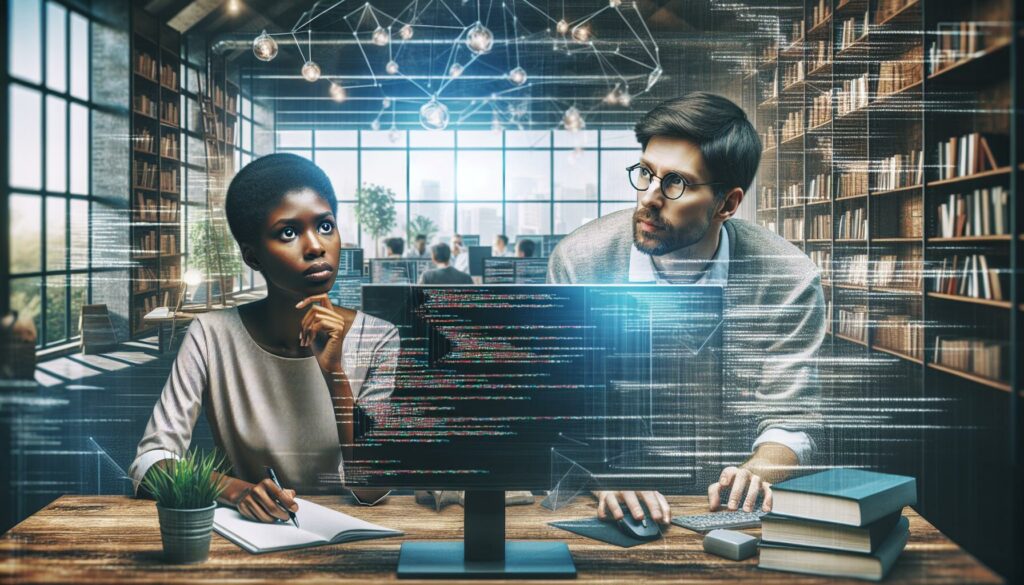
In the world of programming and software development, encountering coding problems is an everyday occurrence. Whether you’re a seasoned developer or just starting your journey, the ability to approach these challenges with a fresh perspective is crucial. This skill becomes even more important when you’re faced with problems you’ve seen before, as it’s easy to fall into the trap of relying on past solutions without considering new, potentially more efficient approaches.
In this comprehensive guide, we’ll explore various strategies and techniques to help you tackle coding problems with renewed vigor and creativity, even if you’ve encountered them previously. We’ll delve into the importance of maintaining a growth mindset, utilizing different problem-solving frameworks, and leveraging tools and resources to enhance your problem-solving abilities.
The Importance of a Fresh Perspective
Before we dive into specific strategies, it’s essential to understand why approaching coding problems with fresh eyes is so crucial:
- Improved Problem-Solving Skills: Constantly challenging yourself to find new solutions enhances your overall problem-solving abilities.
- Adaptability: Technology evolves rapidly, and being able to adapt your thinking helps you stay current with new methodologies and best practices.
- Creativity and Innovation: A fresh approach can lead to innovative solutions that you might not have considered before.
- Continuous Learning: Treating each problem as new encourages continuous learning and personal growth.
- Better Code Quality: Reconsidering problems often leads to more efficient, cleaner, and more maintainable code.
Strategies for Approaching Coding Problems with Fresh Eyes
1. Embrace the Beginner’s Mindset
The beginner’s mindset is a concept from Zen Buddhism that encourages approaching situations with openness, eagerness, and lack of preconceptions. In coding, this means:
- Questioning your assumptions about the problem
- Being open to learning new approaches
- Viewing the problem as if you’re seeing it for the first time
To practice this:
- Ask yourself, “If I knew nothing about this problem, how would I approach it?”
- Challenge your initial instincts and consider alternative perspectives
- Embrace curiosity and ask questions, even if you think you know the answers
2. Reframe the Problem
Sometimes, looking at a problem from a different angle can lead to new insights. Try these techniques:
- Reverse the problem: Instead of solving for the given output, start with the desired result and work backwards.
- Break it down: Divide the problem into smaller, more manageable sub-problems.
- Analogize: Compare the problem to a similar situation in a different context.
For example, if you’re working on a sorting algorithm, instead of thinking about how to order elements, consider how to identify disorder and correct it.
3. Use Different Problem-Solving Frameworks
Applying various problem-solving frameworks can help you approach coding challenges from different angles:
a) IDEAL Problem-Solving Framework
- Identify the problem
- Define the context
- Explore possible strategies
- Act on the best strategy
- Look back and learn
b) Design Thinking
- Empathize with the user
- Define the core problem
- Ideate potential solutions
- Prototype a solution
- Test and refine
c) The Five Whys
Ask “Why?” five times to dig deeper into the root cause of the problem. This can help you understand the underlying issues and potentially find a more fundamental solution.
4. Leverage Different Coding Paradigms
If you’re used to solving problems using a particular programming paradigm, try approaching the problem using a different one. For example:
- If you typically use an object-oriented approach, try functional programming
- If you usually think iteratively, consider a recursive solution
- If you often use imperative programming, explore declarative programming
Here’s a simple example of solving the same problem (calculating the sum of an array) using different paradigms:
Imperative Approach:
function sumArray(arr) {
let sum = 0;
for (let i = 0; i < arr.length; i++) {
sum += arr[i];
}
return sum;
}
Functional Approach:
const sumArray = arr => arr.reduce((sum, num) => sum + num, 0);
Recursive Approach:
function sumArray(arr) {
if (arr.length === 0) return 0;
return arr[0] + sumArray(arr.slice(1));
}
5. Time-Boxing and Rapid Prototyping
When faced with a familiar problem, it’s easy to jump straight into coding a solution you’ve used before. Instead, try these techniques:
- Time-boxing: Set a short time limit (e.g., 15 minutes) to come up with as many different approaches as possible without implementing them fully.
- Rapid prototyping: Quickly implement partial solutions or key components of different approaches to compare their viability.
These techniques encourage creative thinking and help you explore multiple solutions before committing to one.
6. Collaborate and Seek Different Perspectives
Discussing the problem with others can provide fresh insights:
- Pair program with a colleague
- Explain the problem to someone with a different level of expertise (rubber duck debugging)
- Participate in coding forums or communities to see how others approach similar problems
7. Use Visualization Techniques
Visual representations can help you see the problem from a new angle:
- Draw diagrams or flowcharts
- Use mind mapping to explore different aspects of the problem
- Create visual models of data structures or algorithms
8. Explore New Technologies or Libraries
Sometimes, a fresh approach comes from using new tools:
- Research if there are new libraries or frameworks that could simplify the problem
- Explore how the problem might be solved using a different programming language
- Consider how emerging technologies (e.g., machine learning, blockchain) might offer novel solutions
Practical Exercises to Cultivate a Fresh Perspective
To develop your ability to approach problems with fresh eyes, try these exercises:
1. The Alternate Solution Challenge
Take a coding problem you’ve solved before and challenge yourself to solve it in three different ways. For each solution, impose a constraint, such as:
- Use a different data structure
- Optimize for a different aspect (e.g., time complexity vs. space complexity)
- Use only pure functions
2. The Explanation Rotation
Form a group with other developers. Each person brings a coding problem they’ve solved. Rotate through the group, with each person explaining their problem and solution. The rest of the group then brainstorms alternative approaches.
3. The Time Travel Technique
Imagine you’re explaining the problem to yourself from five years ago. What fundamental concepts would you need to cover? This exercise can help you identify core principles you might be taking for granted.
4. The Analogy Game
Practice describing coding problems using analogies from everyday life. For example, describe a sorting algorithm as a method for organizing a bookshelf. This exercise helps you think about problems in more abstract, generalizable terms.
Leveraging Tools and Resources
While developing your ability to think creatively is crucial, it’s also important to leverage available tools and resources:
1. Online Coding Platforms
Platforms like LeetCode, HackerRank, and CodeSignal offer a wide variety of coding problems. Even if you’ve solved these problems before, try approaching them again with the strategies we’ve discussed.
2. Version Control Systems
Use Git to create separate branches for different solution approaches. This allows you to easily compare and contrast different implementations.
3. Code Analysis Tools
Use static analysis tools to evaluate your different solutions. They can provide insights into code quality, complexity, and potential optimizations.
4. Benchmarking Tools
For problems where performance is crucial, use benchmarking tools to compare the efficiency of different solutions empirically.
5. AI-Powered Coding Assistants
Tools like GitHub Copilot or GPT-based coding assistants can sometimes suggest alternative approaches you might not have considered. Use these as inspiration, but always understand and critically evaluate the suggested code.
Overcoming Challenges and Pitfalls
While striving to approach problems with fresh eyes, you may encounter some challenges:
1. Overcoming Cognitive Biases
Be aware of cognitive biases that can hinder fresh thinking:
- Confirmation Bias: The tendency to search for information that confirms your preexisting beliefs.
- Functional Fixedness: The tendency to see objects or ideas only in terms of their usual functions.
- Anchoring: The tendency to rely too heavily on the first piece of information encountered.
To combat these, consciously challenge your initial assumptions and actively seek out contradictory information.
2. Balancing Efficiency and Exploration
While it’s valuable to explore new approaches, it’s also important to be pragmatic, especially in professional settings with deadlines. Strike a balance by:
- Allocating specific time for exploration and innovation
- Using your judgment to decide when a familiar solution is truly the best option
- Documenting alternative approaches you’ve considered, even if not implemented
3. Dealing with Frustration
Approaching familiar problems in new ways can sometimes be frustrating. Remember:
- It’s okay to not immediately find a better solution
- The process of exploration is valuable in itself
- Take breaks to refresh your mind when you feel stuck
Conclusion
Approaching coding problems with fresh eyes, even when they’re familiar, is a valuable skill that can significantly enhance your problem-solving abilities and make you a more versatile developer. By embracing a beginner’s mindset, reframing problems, using various problem-solving frameworks, and leveraging different coding paradigms, you can unlock new perspectives and innovative solutions.
Remember, the goal isn’t always to find a completely new solution, but to cultivate a mindset of curiosity and continuous learning. This approach not only helps you solve individual coding problems more effectively but also contributes to your overall growth as a developer.
As you practice these techniques, you’ll likely find that your ability to think creatively and approach problems from multiple angles becomes second nature. This skill will serve you well throughout your career, helping you adapt to new technologies, tackle complex challenges, and continually improve your coding abilities.
So the next time you encounter a coding problem, whether it’s new or familiar, take a moment to step back, clear your mind, and approach it with fresh eyes. You might be surprised at the innovative solutions you discover.