How to Approach Bit Manipulation Questions: A Comprehensive Guide
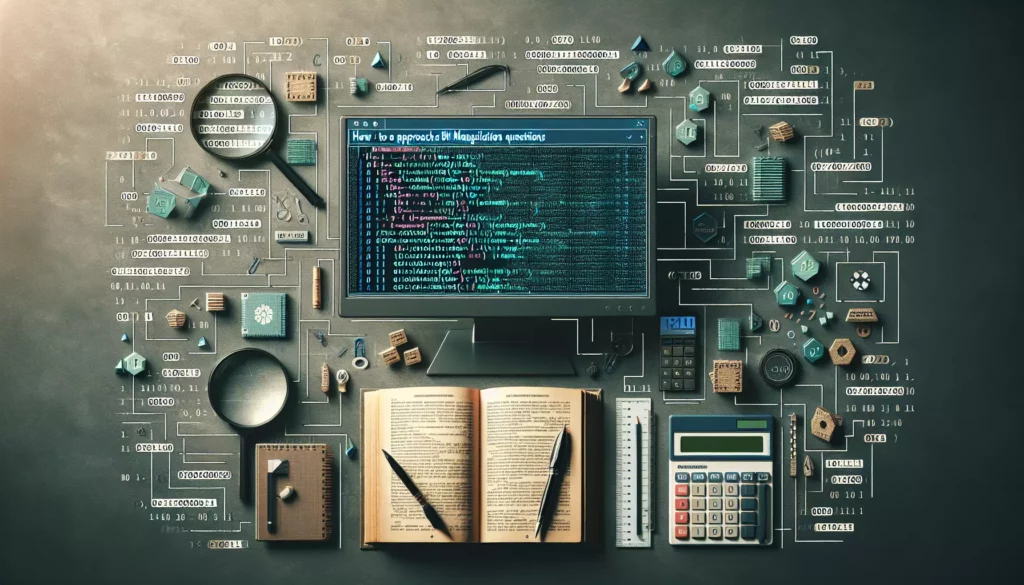
Bit manipulation is a fundamental concept in computer science and programming that often appears in technical interviews, especially for positions at top tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google). Understanding and mastering bit manipulation can give you a significant edge in these interviews and improve your overall programming skills. In this comprehensive guide, we’ll explore how to approach bit manipulation questions, common techniques, and practical examples to help you excel in your coding journey.
Table of Contents
- Understanding Bit Manipulation
- Common Bit Manipulation Operations
- Approaching Bit Manipulation Questions
- Common Bit Manipulation Problems
- Tips and Tricks for Bit Manipulation
- Practice Problems
- Conclusion
1. Understanding Bit Manipulation
Bit manipulation involves performing operations on individual bits of a number. In computer systems, all data is ultimately represented as a series of binary digits (bits), each of which can be either 0 or 1. By manipulating these bits directly, we can perform various operations more efficiently than traditional arithmetic operations.
To work effectively with bit manipulation, it’s crucial to understand the binary representation of numbers and how bitwise operations work. Let’s start with a quick refresher on binary numbers:
- In binary, each digit represents a power of 2, starting from the rightmost digit (2^0, 2^1, 2^2, and so on).
- For example, the binary number 1010 represents (1 * 2^3) + (0 * 2^2) + (1 * 2^1) + (0 * 2^0) = 8 + 0 + 2 + 0 = 10 in decimal.
Understanding this representation is crucial for performing bit manipulation operations effectively.
2. Common Bit Manipulation Operations
Before diving into specific questions, let’s review some common bit manipulation operations that you’ll frequently encounter:
2.1 Bitwise AND (&)
The bitwise AND operation compares corresponding bits of two numbers and returns 1 if both bits are 1, otherwise 0.
1010 (10 in decimal)
& 1100 (12 in decimal)
----
1000 (8 in decimal)
2.2 Bitwise OR (|)
The bitwise OR operation compares corresponding bits of two numbers and returns 1 if at least one of the bits is 1, otherwise 0.
1010 (10 in decimal)
| 1100 (12 in decimal)
----
1110 (14 in decimal)
2.3 Bitwise XOR (^)
The bitwise XOR (exclusive OR) operation compares corresponding bits of two numbers and returns 1 if the bits are different, otherwise 0.
1010 (10 in decimal)
^ 1100 (12 in decimal)
----
0110 (6 in decimal)
2.4 Bitwise NOT (~)
The bitwise NOT operation inverts all the bits of a number, changing 0 to 1 and 1 to 0.
~ 1010 (10 in decimal)
----
0101 (5 in decimal, assuming 4-bit representation)
2.5 Left Shift (<<)
The left shift operation moves all bits to the left by a specified number of positions, filling the rightmost bits with 0.
1010 << 1 = 10100 (20 in decimal)
1010 << 2 = 101000 (40 in decimal)
2.6 Right Shift (>>)
The right shift operation moves all bits to the right by a specified number of positions. For unsigned numbers, the leftmost bits are filled with 0. For signed numbers, the behavior depends on the language and implementation.
1010 >> 1 = 0101 (5 in decimal)
1010 >> 2 = 0010 (2 in decimal)
3. Approaching Bit Manipulation Questions
When faced with a bit manipulation question in an interview or coding challenge, follow these steps to approach the problem systematically:
3.1 Understand the Problem
Carefully read the problem statement and make sure you understand what’s being asked. Identify the input, expected output, and any constraints or special conditions.
3.2 Visualize the Binary Representation
Try to visualize how the numbers or data would look in binary. This can often provide insights into which bit manipulation operations might be useful.
3.3 Identify Relevant Bit Patterns
Look for patterns in the binary representation that could be exploited using bitwise operations. For example, if you need to check if a number is even or odd, you can use the fact that the least significant bit is 0 for even numbers and 1 for odd numbers.
3.4 Choose Appropriate Bit Operations
Based on the patterns you’ve identified, select the most appropriate bit manipulation operations to solve the problem efficiently.
3.5 Implement the Solution
Write the code to implement your solution using the chosen bit operations. Be careful with operator precedence and use parentheses when necessary to ensure correct execution order.
3.6 Test and Verify
Test your solution with various input cases, including edge cases, to ensure it works correctly. Verify the output matches the expected results.
3.7 Optimize if Necessary
If time permits, consider if there are any optimizations you can make to improve the efficiency or readability of your solution.
4. Common Bit Manipulation Problems
Let’s explore some common bit manipulation problems you might encounter in interviews, along with their solutions:
4.1 Check if a Number is Even or Odd
To determine if a number is even or odd, we can use the fact that the least significant bit of an even number is always 0, while for an odd number, it’s 1.
bool isEven(int num) {
return (num & 1) == 0;
}
bool isOdd(int num) {
return (num & 1) == 1;
}
4.2 Count the Number of Set Bits (1’s) in an Integer
This problem, also known as the “population count” or “Hamming weight,” can be solved efficiently using the Brian Kernighan’s algorithm:
int countSetBits(int num) {
int count = 0;
while (num) {
num &= (num - 1);
count++;
}
return count;
}
4.3 Find the Single Number in an Array where Every Element Appears Twice Except for One
This problem can be solved efficiently using the XOR operation, as XORing a number with itself results in 0:
int findSingleNumber(vector<int>& nums) {
int result = 0;
for (int num : nums) {
result ^= num;
}
return result;
}
4.4 Swap Two Numbers Without Using a Temporary Variable
We can use XOR to swap two numbers without using an additional variable:
void swapNumbers(int& a, int& b) {
a = a ^ b;
b = a ^ b;
a = a ^ b;
}
4.5 Check if a Number is a Power of Two
A number that is a power of two has only one bit set in its binary representation. We can use this property to check if a number is a power of two:
bool isPowerOfTwo(int n) {
return n > 0 && (n & (n - 1)) == 0;
}
5. Tips and Tricks for Bit Manipulation
Here are some additional tips and tricks to keep in mind when working with bit manipulation:
5.1 Use Bit Masks
Bit masks are predefined binary patterns used to manipulate specific bits. For example, to set the nth bit of a number, you can use the mask (1 << n) with the OR operation:
int setNthBit(int num, int n) {
return num | (1 << n);
}
5.2 Remember Common Bit Patterns
Familiarize yourself with common bit patterns and their meanings:
- 0xFFFFFFFF: All bits set to 1 (for a 32-bit integer)
- 0x55555555: Alternating 0 and 1 (01010101…)
- 0x33333333: Two 1’s followed by two 0’s (00110011…)
- 0x0F0F0F0F: Four 1’s followed by four 0’s (00001111…)
5.3 Use De Morgan’s Laws
De Morgan’s laws can be applied to bitwise operations:
- ~(a & b) = ~a | ~b
- ~(a | b) = ~a & ~b
5.4 Leverage XOR Properties
XOR has several useful properties:
- a ^ a = 0
- a ^ 0 = a
- a ^ b ^ a = b
5.5 Use Shifting for Multiplication and Division
Left shifting by n is equivalent to multiplying by 2^n, while right shifting by n is equivalent to dividing by 2^n (for positive numbers):
int multiplyByPowerOfTwo(int num, int n) {
return num << n;
}
int divideByPowerOfTwo(int num, int n) {
return num >> n;
}
6. Practice Problems
To improve your bit manipulation skills, practice solving these problems:
6.1 Reverse Bits
Reverse the bits of a given 32-bit unsigned integer.
uint32_t reverseBits(uint32_t n) {
uint32_t result = 0;
for (int i = 0; i < 32; i++) {
result = (result << 1) | (n & 1);
n >>= 1;
}
return result;
}
6.2 Find the Missing Number
Given an array containing n distinct numbers taken from 0, 1, 2, …, n, find the one that is missing from the array.
int missingNumber(vector<int>& nums) {
int result = nums.size();
for (int i = 0; i < nums.size(); i++) {
result ^= i ^ nums[i];
}
return result;
}
6.3 Count Number of Flips to Make A OR B Equal to C
Given 3 positives numbers a, b and c. Return the minimum flips required in some bits of a and b to make (a OR b == c). (bitwise OR operation).
int minFlips(int a, int b, int c) {
int flips = 0;
for (int i = 0; i < 32; i++) {
bool bitA = (a >> i) & 1;
bool bitB = (b >> i) & 1;
bool bitC = (c >> i) & 1;
if ((bitA | bitB) != bitC) {
if (bitC == 0) {
flips += (bitA == 1) + (bitB == 1);
} else {
flips += 1;
}
}
}
return flips;
}
6.4 Find the Difference
Given two strings s and t which consist of only lowercase letters. String t is generated by random shuffling string s and then add one more letter at a random position. Find the letter that was added in t.
char findTheDifference(string s, string t) {
char result = 0;
for (char c : s) result ^= c;
for (char c : t) result ^= c;
return result;
}
6.5 Decode XORed Array
There is a hidden integer array arr that consists of n non-negative integers. It was encoded into another integer array encoded of length n – 1, such that encoded[i] = arr[i] XOR arr[i + 1]. For example, if arr = [1,0,2,1], then encoded = [1,2,3]. You are given the encoded array. You are also given an integer first, that is the first element of arr, i.e. arr[0]. Return the original array arr. It is guaranteed that the answer exists and is unique.
vector<int> decode(vector<int>& encoded, int first) {
vector<int> result(encoded.size() + 1);
result[0] = first;
for (int i = 0; i < encoded.size(); i++) {
result[i + 1] = result[i] ^ encoded[i];
}
return result;
}
7. Conclusion
Mastering bit manipulation is an essential skill for any programmer, especially those aiming for positions at top tech companies. By understanding the fundamentals of binary representation and bitwise operations, and practicing with various problems, you can significantly improve your problem-solving abilities and coding efficiency.
Remember that bit manipulation often leads to more efficient solutions for certain types of problems, but it’s important to balance efficiency with code readability. Always consider the trade-offs between using bit manipulation techniques and more straightforward arithmetic operations, depending on the specific requirements of your project or interview question.
As you continue your coding journey, make sure to practice bit manipulation problems regularly. Platforms like AlgoCademy offer a wealth of resources, including interactive tutorials and practice problems, to help you hone your skills and prepare for technical interviews. With consistent practice and a solid understanding of the concepts covered in this guide, you’ll be well-equipped to tackle even the most challenging bit manipulation questions in your coding interviews and real-world programming tasks.