How to Approach Algorithmic Thinking in Problem Solving
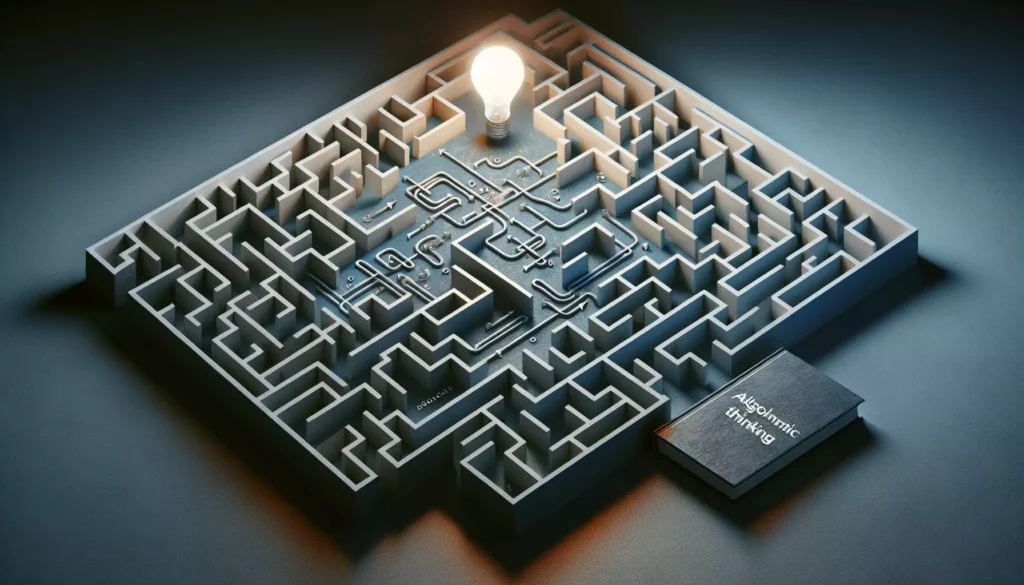
In the world of programming and software development, algorithmic thinking is a fundamental skill that separates good programmers from great ones. It’s the ability to break down complex problems into smaller, manageable steps and devise efficient solutions. Whether you’re a beginner just starting your coding journey or an experienced developer preparing for technical interviews at top tech companies, mastering algorithmic thinking is crucial for your success. In this comprehensive guide, we’ll explore the concept of algorithmic thinking, its importance in problem-solving, and provide you with practical strategies to improve your skills.
What is Algorithmic Thinking?
Algorithmic thinking is a problem-solving approach that involves breaking down complex problems into smaller, more manageable steps. It’s the process of creating a set of clear, precise instructions (an algorithm) that can be followed to solve a problem or perform a task. This approach is not limited to computer programming; it’s a valuable skill in many areas of life and work.
In the context of coding and software development, algorithmic thinking is essential for:
- Designing efficient and scalable solutions
- Optimizing existing code and processes
- Solving complex programming challenges
- Preparing for technical interviews at top tech companies
- Developing a deeper understanding of data structures and algorithms
The Importance of Algorithmic Thinking in Problem Solving
Algorithmic thinking is not just about writing code; it’s about approaching problems in a structured and logical manner. Here’s why it’s crucial for problem-solving:
- Efficiency: By breaking down problems into smaller steps, you can identify the most efficient way to solve them, saving time and resources.
- Scalability: Solutions developed with algorithmic thinking are often more scalable, able to handle larger inputs or more complex scenarios.
- Clarity: The step-by-step nature of algorithms makes it easier to communicate your solution to others and debug issues.
- Reusability: Well-designed algorithms can often be adapted and reused for similar problems in the future.
- Problem-solving skills: Practicing algorithmic thinking enhances your overall problem-solving abilities, which is valuable in many aspects of life and work.
Key Components of Algorithmic Thinking
To effectively apply algorithmic thinking to problem-solving, it’s important to understand its key components:
1. Problem Analysis
Before diving into a solution, take the time to thoroughly understand the problem. This involves:
- Identifying the inputs and expected outputs
- Recognizing any constraints or limitations
- Breaking down the problem into smaller subproblems
- Identifying patterns or similarities to known problems
2. Algorithm Design
Once you understand the problem, you can start designing your algorithm. This step includes:
- Choosing appropriate data structures
- Outlining the steps needed to solve the problem
- Considering different approaches and their trade-offs
- Ensuring the solution is efficient and scalable
3. Implementation
Translating your algorithm into code is the next step. This involves:
- Writing clean, readable code
- Following best practices and coding standards
- Implementing error handling and edge cases
- Optimizing the code for performance where necessary
4. Testing and Debugging
Verifying that your solution works correctly is crucial. This includes:
- Creating test cases to cover various scenarios
- Debugging any issues that arise
- Analyzing the time and space complexity of your solution
- Refining the algorithm based on test results
5. Optimization and Refinement
After implementing a working solution, consider ways to improve it:
- Identifying bottlenecks or inefficiencies
- Exploring alternative algorithms or data structures
- Balancing trade-offs between time and space complexity
- Considering edge cases and potential improvements
Strategies for Developing Algorithmic Thinking Skills
Now that we understand the components of algorithmic thinking, let’s explore some strategies to help you develop and improve these skills:
1. Practice Regularly
Like any skill, algorithmic thinking improves with practice. Set aside time regularly to solve coding challenges and algorithmic problems. Platforms like AlgoCademy, LeetCode, HackerRank, and CodeSignal offer a wide range of problems to practice on.
2. Start with Simple Problems
Begin with easy problems and gradually increase the difficulty. This helps build confidence and allows you to learn fundamental concepts before tackling more complex challenges.
3. Analyze Multiple Solutions
For each problem you solve, explore different approaches. Compare their time and space complexities, and understand the trade-offs between different solutions. This broadens your problem-solving toolkit.
4. Learn and Apply Data Structures
A solid understanding of data structures is crucial for algorithmic thinking. Learn about arrays, linked lists, stacks, queues, trees, graphs, and hash tables. Practice implementing these structures and using them to solve problems efficiently.
5. Study Algorithm Design Techniques
Familiarize yourself with common algorithm design techniques such as:
- Divide and Conquer
- Dynamic Programming
- Greedy Algorithms
- Backtracking
- Recursion
Understanding these techniques will help you recognize patterns in problems and apply appropriate strategies.
6. Analyze Time and Space Complexity
Learn to analyze the efficiency of your algorithms using Big O notation. This helps you understand the scalability of your solutions and make informed decisions about trade-offs between time and space complexity.
7. Pseudocode Before Coding
Before implementing your solution in code, write it out in pseudocode. This helps you focus on the logic and structure of your algorithm without getting bogged down in syntax details.
8. Collaborate and Discuss
Engage in discussions with other programmers, participate in coding forums, or join a study group. Explaining your thought process to others and learning from their approaches can greatly enhance your algorithmic thinking skills.
9. Review and Reflect
After solving a problem, take time to reflect on your approach. Consider what worked well and what could be improved. Review solutions from others to learn alternative approaches.
10. Apply Algorithmic Thinking to Real-World Problems
Look for opportunities to apply algorithmic thinking in your daily life or work. This could involve optimizing a process, solving a logistical problem, or breaking down a complex task into manageable steps.
Common Pitfalls to Avoid
As you develop your algorithmic thinking skills, be aware of these common pitfalls:
1. Rushing to Code
Avoid jumping into coding without fully understanding the problem or planning your approach. Take the time to analyze the problem and design your algorithm before implementation.
2. Overlooking Edge Cases
Always consider edge cases and boundary conditions in your solutions. Test your algorithm with extreme or unusual inputs to ensure it handles all scenarios correctly.
3. Ignoring Time and Space Complexity
Don’t focus solely on getting a working solution. Consider the efficiency of your algorithm in terms of both time and space complexity, especially for larger inputs.
4. Reinventing the Wheel
While it’s important to understand how algorithms work, don’t hesitate to use existing libraries or built-in functions when appropriate. Know when to implement something from scratch and when to leverage existing tools.
5. Neglecting Code Readability
Write clean, well-documented code. A highly optimized but unreadable solution is often less valuable than a slightly less efficient but clear and maintainable one.
Applying Algorithmic Thinking in Technical Interviews
Algorithmic thinking is particularly crucial when preparing for technical interviews, especially at top tech companies often referred to as FAANG (Facebook/Meta, Amazon, Apple, Netflix, Google) or MAANG (Meta, Amazon, Apple, Netflix, Google). Here are some tips for applying algorithmic thinking during interviews:
1. Think Aloud
Verbalize your thought process as you work through the problem. This gives the interviewer insight into your algorithmic thinking and problem-solving approach.
2. Ask Clarifying Questions
Before diving into a solution, ask questions to ensure you fully understand the problem, constraints, and expected outputs.
3. Discuss Multiple Approaches
If you can think of multiple ways to solve the problem, briefly discuss them with the interviewer. Explain the trade-offs between different approaches.
4. Start with a Brute Force Solution
If you’re stuck, start with a simple, brute force solution. Then, work on optimizing it. This shows you can at least solve the problem, even if not optimally at first.
5. Analyze Time and Space Complexity
Be prepared to discuss the time and space complexity of your solution. This demonstrates your understanding of algorithmic efficiency.
6. Test Your Solution
After implementing your solution, walk through a few test cases to verify its correctness. This shows attention to detail and thoroughness.
Tools and Resources for Improving Algorithmic Thinking
To support your journey in developing strong algorithmic thinking skills, consider using these tools and resources:
1. Online Platforms
- AlgoCademy: Offers interactive coding tutorials and AI-powered assistance for learning algorithms and data structures.
- LeetCode: Provides a vast collection of coding challenges with a focus on interview preparation.
- HackerRank: Offers coding challenges and competitions across various domains.
- CodeSignal: Features a mix of practice problems and real-world coding challenges.
2. Books
- “Introduction to Algorithms” by Thomas H. Cormen, Charles E. Leiserson, Ronald L. Rivest, and Clifford Stein
- “Grokking Algorithms” by Aditya Bhargava
- “Cracking the Coding Interview” by Gayle Laakmann McDowell
3. Online Courses
- Coursera’s “Algorithms Specialization” by Stanford University
- edX’s “Algorithm Design and Analysis” by PennX
- Udacity’s “Data Structures and Algorithms Nanodegree”
4. Visualization Tools
- VisuAlgo: Visualizes various data structures and algorithms
- Algorithm Visualizer: Interactive platform for visualizing algorithms
Conclusion
Algorithmic thinking is a powerful skill that can significantly enhance your problem-solving abilities and make you a more effective programmer. By breaking down complex problems, designing efficient solutions, and continuously refining your approach, you can tackle even the most challenging coding tasks with confidence.
Remember that developing strong algorithmic thinking skills takes time and practice. Be patient with yourself, embrace the learning process, and don’t be afraid to tackle difficult problems. With persistence and the right approach, you can master algorithmic thinking and open up new opportunities in your coding career.
Whether you’re preparing for technical interviews at top tech companies or simply looking to improve your coding skills, focusing on algorithmic thinking will serve you well. It’s not just about solving specific problems; it’s about developing a mindset that allows you to approach any challenge methodically and creatively.
As you continue your journey in coding and software development, make algorithmic thinking a core part of your skill set. Embrace the challenge, enjoy the process of problem-solving, and watch as your abilities grow. With strong algorithmic thinking skills, you’ll be well-equipped to tackle whatever coding challenges come your way and thrive in the ever-evolving world of technology.