How to Approach a New Coding Problem: Step-by-Step Guide
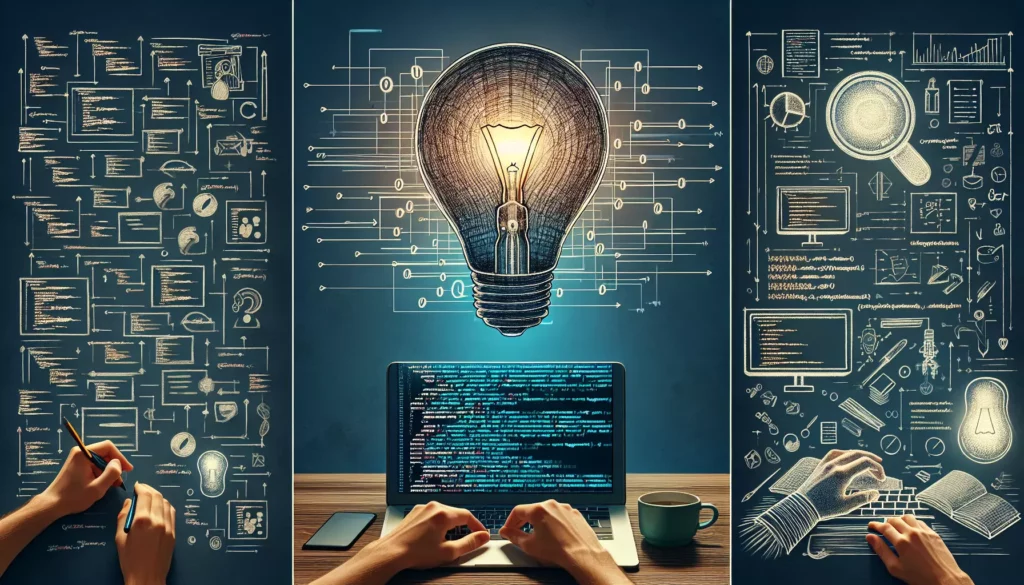
In the world of programming, facing new coding problems is a regular occurrence. Whether you’re a beginner just starting your journey or an experienced developer preparing for technical interviews at major tech companies, having a systematic approach to tackle coding challenges is crucial. This comprehensive guide will walk you through a step-by-step process on how to approach a new coding problem effectively, helping you build confidence and improve your problem-solving skills.
1. Understand the Problem
The first and most crucial step in approaching any coding problem is to thoroughly understand what is being asked. This involves:
- Reading the problem statement carefully, multiple times if necessary
- Identifying the input and output requirements
- Noting any constraints or special conditions mentioned
- Clarifying any ambiguities or uncertainties
For instance, if you’re given a problem to find the maximum element in an array, make sure you understand:
- What type of elements are in the array? (integers, floating-point numbers, strings)
- Is the array sorted or unsorted?
- How should you handle an empty array?
- Are there any time or space complexity requirements?
Taking the time to fully grasp the problem will save you from potential pitfalls and wasted effort later in the process.
2. Analyze the Given Examples
Most coding problems come with example inputs and expected outputs. These examples are invaluable for understanding the problem better and validating your approach. Here’s what you should do:
- Work through each example manually
- Understand how the input transforms into the output
- Look for patterns or special cases in the examples
- Think about edge cases that might not be covered in the given examples
For example, if you’re given a problem to reverse a string, and one of the examples is:
Input: "hello"
Output: "olleh"
You should mentally walk through how each character moves to its new position. This process can often reveal insights about the algorithm you’ll need to implement.
3. Visualize the Problem
Many coding problems can be better understood through visualization. This step involves:
- Drawing diagrams or flowcharts
- Using physical objects or props to represent data structures
- Creating visual representations of the problem state and its transitions
For instance, if you’re working on a linked list problem, draw out the nodes and arrows to represent the list. If it’s a tree problem, sketch the tree structure. These visual aids can help you see patterns and relationships that might not be immediately apparent from the problem description alone.
4. Break Down the Problem
Complex problems can often be intimidating at first glance. The key is to break them down into smaller, more manageable sub-problems. This approach, known as “divide and conquer,” involves:
- Identifying the main components or steps of the solution
- Breaking each component into smaller, solvable tasks
- Focusing on solving one sub-problem at a time
For example, if you’re tasked with implementing a sorting algorithm, you might break it down into these steps:
- Choose a pivot element
- Partition the array around the pivot
- Recursively sort the sub-arrays
- Combine the sorted sub-arrays
By tackling each sub-problem individually, you make the overall task less daunting and more approachable.
5. Plan Your Approach
Before diving into coding, it’s crucial to plan your approach. This involves:
- Choosing an appropriate algorithm or data structure
- Outlining the steps of your solution in pseudocode
- Considering alternative approaches and their trade-offs
- Estimating the time and space complexity of your solution
For instance, if you’re working on a problem to find the kth largest element in an unsorted array, you might consider these approaches:
- Sort the array and return the kth element from the end (Time: O(n log n), Space: O(1) or O(n) depending on the sorting algorithm)
- Use a min-heap of size k (Time: O(n log k), Space: O(k))
- Use the QuickSelect algorithm (Average Time: O(n), Worst Time: O(n^2), Space: O(1))
By planning your approach beforehand, you can make informed decisions about the best solution for the given constraints.
6. Implement the Solution
Once you have a solid plan, it’s time to write the actual code. During this step:
- Choose an appropriate programming language
- Follow good coding practices (clear variable names, proper indentation, etc.)
- Implement one function or module at a time
- Use comments to explain complex logic or important steps
Here’s an example of how you might start implementing a function to find the maximum element in an array:
def find_max(arr):
# Check if the array is empty
if not arr:
return None
# Initialize max_element with the first element
max_element = arr[0]
# Iterate through the array, updating max_element if necessary
for element in arr[1:]:
if element > max_element:
max_element = element
return max_element
Remember, your first implementation doesn’t need to be perfect. Focus on getting a working solution first, then you can optimize it later.
7. Test Your Solution
Testing is a crucial part of the problem-solving process. It helps you catch errors and ensure your solution works as expected. Here’s how to approach testing:
- Start with the given example cases
- Test edge cases (empty input, single element, very large input, etc.)
- Create additional test cases to cover various scenarios
- Use print statements or a debugger to track the flow of your program
For the find_max function, you might test it like this:
# Test cases
print(find_max([1, 5, 3, 9, 2])) # Expected output: 9
print(find_max([-1, -5, -3])) # Expected output: -1
print(find_max([])) # Expected output: None
print(find_max([42])) # Expected output: 42
If you encounter any errors or unexpected results, go back to your implementation and make the necessary corrections.
8. Optimize Your Solution
Once you have a working solution, consider if there’s room for optimization. This step involves:
- Analyzing the time and space complexity of your current solution
- Identifying bottlenecks or inefficiencies
- Researching more efficient algorithms or data structures
- Implementing and testing the optimized solution
For example, if you initially solved a problem using a nested loop with O(n^2) time complexity, you might look for a way to solve it in O(n log n) or O(n) time.
Here’s an example of optimizing a function that finds the two numbers in an array that add up to a target sum:
# Initial brute force solution (O(n^2) time complexity)
def find_pair_sum_brute_force(arr, target):
for i in range(len(arr)):
for j in range(i+1, len(arr)):
if arr[i] + arr[j] == target:
return [arr[i], arr[j]]
return None
# Optimized solution using a hash table (O(n) time complexity)
def find_pair_sum_optimized(arr, target):
complement_dict = {}
for num in arr:
complement = target - num
if complement in complement_dict:
return [complement, num]
complement_dict[num] = True
return None
The optimized solution reduces the time complexity from O(n^2) to O(n) by using a hash table to store complements, trading some space for improved time efficiency.
9. Reflect on Your Solution
After solving the problem, take some time to reflect on your solution and the problem-solving process. This step is often overlooked but is crucial for long-term improvement. Consider the following:
- What worked well in your approach?
- What challenges did you face, and how did you overcome them?
- Are there any patterns or techniques you can apply to similar problems in the future?
- How does your solution compare to other possible approaches?
For instance, if you solved a dynamic programming problem, you might reflect on how you identified the optimal substructure and overlapping subproblems. This reflection can help you recognize similar patterns in future problems and apply the same techniques more quickly.
10. Document Your Solution
The final step in approaching a coding problem is to document your solution. This is particularly important if you’re working on a project or preparing for technical interviews. Good documentation includes:
- A brief description of the problem
- Your approach and reasoning
- The time and space complexity of your solution
- Any assumptions or constraints you considered
- Examples of how to use your function or code
Here’s an example of how you might document the find_max function:
"""
Function: find_max
Description:
This function finds the maximum element in an array of numbers.
Parameters:
arr (list): An array of numbers (can be empty)
Returns:
The maximum element in the array, or None if the array is empty.
Time Complexity: O(n), where n is the length of the array
Space Complexity: O(1)
Example Usage:
>>> find_max([1, 5, 3, 9, 2])
9
>>> find_max([])
None
"""
def find_max(arr):
# Implementation here...
Good documentation not only helps others understand your code but also serves as a valuable reference for yourself in the future.
Conclusion
Approaching a new coding problem can be challenging, but following these steps can help you tackle even the most complex challenges with confidence. Remember, the key to becoming proficient at problem-solving is practice. The more problems you solve, the more patterns you’ll recognize, and the more efficient you’ll become at navigating the problem-solving process.
As you continue your coding journey, whether you’re using platforms like AlgoCademy to prepare for technical interviews or working on personal projects, keep these steps in mind. They’ll serve as a reliable framework for approaching any coding problem you encounter.
Don’t be discouraged if you struggle with a problem at first. Problem-solving is a skill that improves with time and practice. Each problem you tackle, regardless of whether you solve it immediately or need to research and learn new concepts, contributes to your growth as a programmer.
Finally, remember that there’s often more than one way to solve a problem. As you gain experience, you’ll develop your own style and preferences. The most important thing is to find an approach that works for you and allows you to solve problems efficiently and effectively.
Happy coding, and may your problem-solving skills continue to grow and evolve!