How to Approach a New Coding Challenge: A Comprehensive Guide
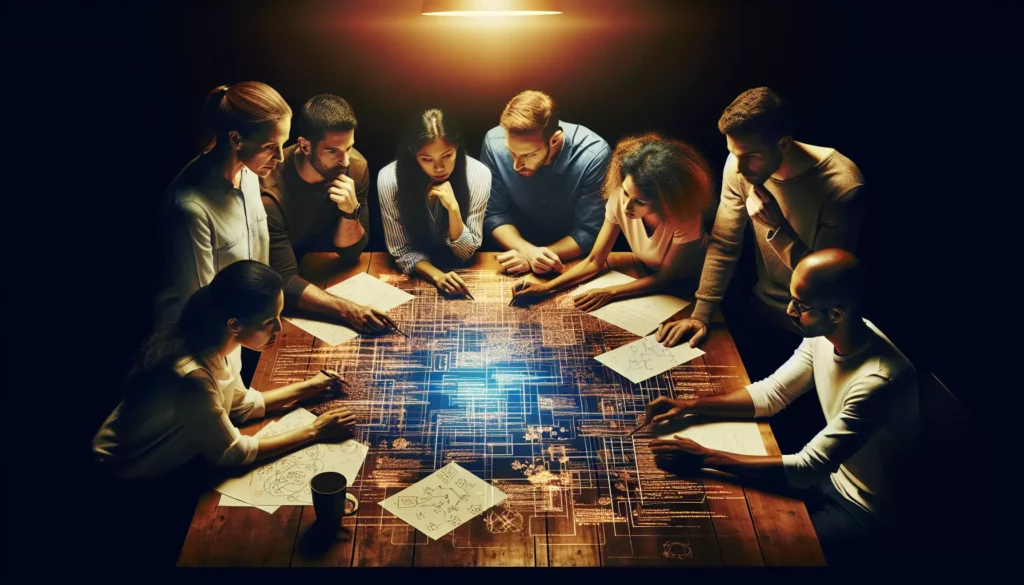
In the world of programming and software development, facing new coding challenges is a regular occurrence. Whether you’re a beginner just starting your journey or an experienced developer preparing for technical interviews at top tech companies, knowing how to approach a new coding challenge effectively is crucial. This comprehensive guide will walk you through the steps to tackle any coding problem with confidence and efficiency.
1. Understand the Problem
The first and most crucial step in approaching any coding challenge is to thoroughly understand the problem at hand. This involves several sub-steps:
1.1. Read the Problem Statement Carefully
Take your time to read the problem statement multiple times. Pay attention to every detail, as even small nuances can significantly impact your solution. Look for key information such as:
- Input format and constraints
- Expected output format
- Any specific requirements or limitations
- Edge cases or special conditions
1.2. Identify the Goal
Clearly define what the problem is asking you to achieve. Are you supposed to find a specific value, transform data, or optimize a process? Having a clear goal in mind will guide your problem-solving approach.
1.3. Analyze the Given Examples
Most coding challenges come with example inputs and outputs. Analyze these examples carefully to understand how the input relates to the output. This can provide valuable insights into the problem’s logic and help you identify patterns.
1.4. Ask Clarifying Questions
If you’re in an interview setting or have the opportunity to ask questions, don’t hesitate to seek clarification. Some potential questions could be:
- Are there any constraints on time or space complexity?
- Can I assume the input will always be valid?
- How should the program handle edge cases or errors?
2. Plan Your Approach
Once you have a solid understanding of the problem, it’s time to plan your approach. This step is crucial for developing an efficient and effective solution.
2.1. Break Down the Problem
Complex problems can often be broken down into smaller, more manageable sub-problems. Identify these sub-problems and consider how they relate to each other. This technique, known as “divide and conquer,” can make seemingly insurmountable challenges more approachable.
2.2. Consider Multiple Approaches
Before diving into coding, brainstorm different ways to solve the problem. Consider various algorithms and data structures that might be applicable. Some common approaches include:
- Brute force
- Greedy algorithms
- Dynamic programming
- Divide and conquer
- Two-pointer technique
- Sliding window
- Depth-First Search (DFS) or Breadth-First Search (BFS) for graph problems
2.3. Analyze Time and Space Complexity
For each potential approach, consider its time and space complexity. This is especially important when preparing for technical interviews at major tech companies. Aim for the most efficient solution that meets the problem’s requirements.
2.4. Choose the Best Approach
After considering multiple approaches, select the one that best balances efficiency, simplicity, and adherence to any given constraints. Remember, the “best” approach may vary depending on the specific requirements of the problem or interview.
2.5. Outline Your Algorithm
Before writing any code, outline your chosen algorithm in plain English or pseudocode. This step helps organize your thoughts and can reveal potential issues or optimizations before you start coding.
3. Implement Your Solution
With a solid plan in place, it’s time to translate your algorithm into actual code. Here are some best practices to follow during the implementation phase:
3.1. Start with a Simple Implementation
Begin by implementing a basic version of your solution. Don’t worry about optimizations or edge cases at this stage. The goal is to get a working solution that handles the main logic of the problem.
3.2. Use Clear Variable Names and Comments
Write clean, readable code with descriptive variable names. Add comments to explain complex parts of your logic. This not only helps others understand your code but also aids you in keeping track of your thought process.
3.3. Follow Good Coding Practices
Adhere to the coding standards and best practices of your chosen programming language. This includes proper indentation, consistent naming conventions, and efficient use of language-specific features.
3.4. Implement Step by Step
If your solution involves multiple steps or components, implement them one at a time. Test each component individually before moving on to the next. This incremental approach makes it easier to identify and fix issues.
3.5. Use Version Control
If you’re working on a larger project or want to keep track of different versions of your solution, consider using a version control system like Git. This allows you to experiment with different approaches while maintaining a history of your work.
4. Test Your Solution
Testing is a critical part of the problem-solving process. It helps ensure your solution works correctly and handles various scenarios.
4.1. Start with the Given Examples
Begin by testing your solution against the examples provided in the problem statement. Your code should produce the expected output for these cases.
4.2. Test Edge Cases
Identify and test edge cases that might cause your solution to fail. These could include:
- Empty input
- Very large or very small inputs
- Inputs with special characters or unexpected formats
- Boundary conditions (e.g., the maximum or minimum possible input values)
4.3. Create Additional Test Cases
Generate your own test cases to cover scenarios not explicitly mentioned in the problem statement. Try to think of inputs that might reveal weaknesses in your algorithm.
4.4. Use Debug Statements
If your solution isn’t producing the expected results, use debug statements or breakpoints to trace the execution of your code. This can help you identify where the logic is failing.
4.5. Consider Time and Space Efficiency
If the problem has specific time or space complexity requirements, test your solution with large inputs to ensure it meets these constraints.
5. Optimize and Refine
Once you have a working solution, look for ways to improve it. This step is particularly important for technical interviews and competitive programming.
5.1. Analyze for Bottlenecks
Identify parts of your code that are computationally expensive or memory-intensive. These are prime candidates for optimization.
5.2. Consider Alternative Data Structures
Sometimes, changing the data structure you use can significantly improve performance. For example, using a hash table instead of an array for lookups can reduce time complexity from O(n) to O(1) in many cases.
5.3. Look for Redundant Computations
Check if your code is performing the same calculations multiple times. If so, consider caching results or restructuring your algorithm to avoid redundancy.
5.4. Simplify Your Code
Look for opportunities to simplify your code without sacrificing functionality or efficiency. Simpler code is often easier to understand, maintain, and debug.
5.5. Consider Space-Time Tradeoffs
Sometimes, you can improve time complexity by using more memory, or vice versa. Evaluate whether such tradeoffs are appropriate for your specific problem and constraints.
6. Reflect and Learn
After solving a coding challenge, take some time to reflect on the process and what you’ve learned.
6.1. Review Your Approach
Consider the effectiveness of your problem-solving approach. What worked well? What could be improved? This reflection can help you refine your process for future challenges.
6.2. Study Alternative Solutions
If possible, look at other solutions to the same problem. You might discover more efficient algorithms or clever techniques that you can add to your problem-solving toolkit.
6.3. Identify Knowledge Gaps
Did you encounter any concepts or algorithms during the challenge that you weren’t familiar with? Make a note of these and plan to study them further.
6.4. Practice Similar Problems
If you found the challenge particularly difficult or interesting, look for similar problems to reinforce your learning and expand your skills.
7. Practical Example: Two Sum Problem
Let’s apply the approach we’ve discussed to a common coding challenge: the Two Sum problem. This problem is often encountered in coding interviews and serves as a good example of how to approach a coding challenge.
Problem Statement:
Given an array of integers nums
and an integer target
, return indices of the two numbers in the array such that they add up to the target
. You may assume that each input would have exactly one solution, and you may not use the same element twice.
Step 1: Understand the Problem
Input: An array of integers and a target sum
Output: Indices of two numbers that add up to the target
Constraints: Each input has exactly one solution, and an element can’t be used twice
Step 2: Plan Your Approach
We can consider two approaches:
- Brute Force: Check every pair of numbers (O(n^2) time complexity)
- Hash Table: Use a hash table to store complements (O(n) time complexity)
Let’s choose the hash table approach for better efficiency.
Step 3: Implement Your Solution
Here’s a Python implementation of the Two Sum problem using a hash table:
def two_sum(nums, target):
complement_map = {}
for i, num in enumerate(nums):
complement = target - num
if complement in complement_map:
return [complement_map[complement], i]
complement_map[num] = i
return [] # No solution found
Step 4: Test Your Solution
Let’s test our solution with some examples:
print(two_sum([2, 7, 11, 15], 9)) # Expected: [0, 1]
print(two_sum([3, 2, 4], 6)) # Expected: [1, 2]
print(two_sum([3, 3], 6)) # Expected: [0, 1]
print(two_sum([1, 2, 3, 4], 10)) # Expected: [] (no solution)
Step 5: Optimize and Refine
Our solution already has O(n) time complexity and O(n) space complexity, which is optimal for this problem. However, we can make a small optimization by checking if the complement exists before adding the current number to the hash table:
def two_sum_optimized(nums, target):
complement_map = {}
for i, num in enumerate(nums):
if num in complement_map:
return [complement_map[num], i]
complement_map[target - num] = i
return [] # No solution found
Step 6: Reflect and Learn
This problem introduces the concept of using a hash table for O(1) lookups, which is a common technique in many coding challenges. It also demonstrates how to optimize space-time tradeoffs, as we use extra space (the hash table) to achieve linear time complexity.
Conclusion
Approaching a new coding challenge can be daunting, but with a systematic method, you can tackle even the most complex problems with confidence. Remember to thoroughly understand the problem, plan your approach, implement your solution carefully, test rigorously, and always look for ways to optimize and learn from the experience.
As you practice more coding challenges, you’ll develop a intuition for common patterns and techniques. Platforms like AlgoCademy offer a wealth of resources, including interactive tutorials, AI-powered assistance, and a wide range of coding challenges to help you hone your skills and prepare for technical interviews at top tech companies.
Keep in mind that becoming proficient at solving coding challenges is a journey that requires consistent practice and a willingness to learn from both successes and failures. Each challenge you face is an opportunity to grow as a programmer and problem-solver. So, embrace the process, stay curious, and happy coding!