How to Approach a Coding Problem as a Consultant: Focus on Understanding First
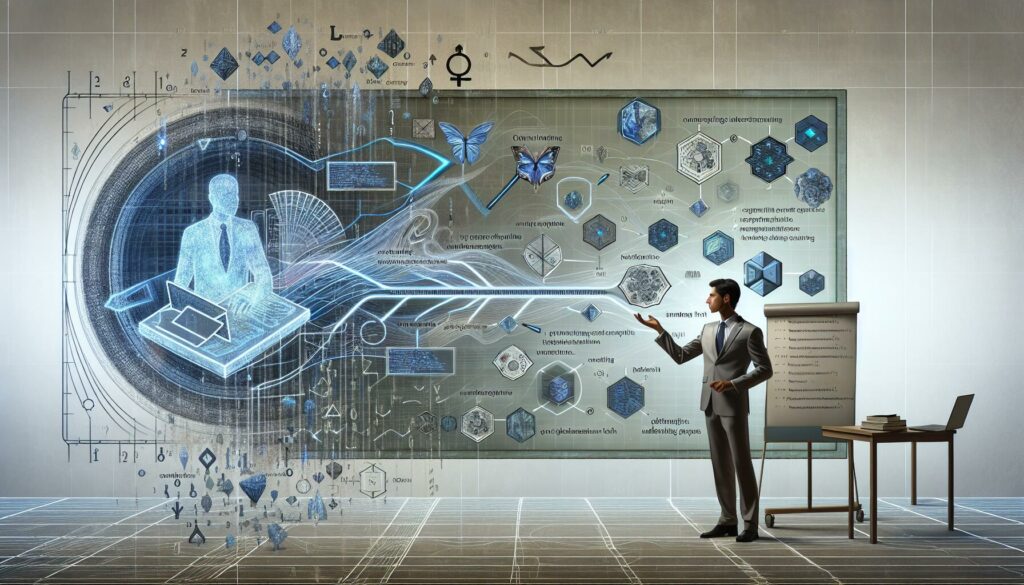
In the fast-paced world of software development, consultants are often called upon to solve complex coding problems for various clients. Whether you’re preparing for a technical interview at a major tech company or working on a challenging project, the ability to approach coding problems methodically is crucial. This article will guide you through the process of tackling coding challenges with a consultant’s mindset, emphasizing the importance of understanding the problem before diving into the solution.
The Importance of Understanding First
As a consultant, your primary goal is to provide value to your clients. This means not just writing code that works, but creating solutions that are efficient, maintainable, and aligned with the client’s needs. The key to achieving this lies in developing a deep understanding of the problem at hand before you even begin to code.
Here’s why focusing on understanding is crucial:
- It helps you identify the root cause of the problem, not just its symptoms
- It allows you to consider multiple approaches and choose the most appropriate one
- It prevents wasted time and effort on solutions that don’t address the real issue
- It enables you to communicate more effectively with stakeholders
- It sets the foundation for writing cleaner, more efficient code
The 5-Step Approach to Coding Problems
Let’s break down the process of approaching a coding problem into five key steps:
1. Clarify the Problem
Before you start coding, make sure you have a clear understanding of what the problem is asking. This step involves:
- Reading the problem statement carefully
- Identifying the inputs and expected outputs
- Asking clarifying questions
- Confirming any assumptions you’re making
For example, if you’re asked to implement a function that finds the longest substring without repeating characters, you might ask:
- What should the function return if the input string is empty?
- Are we considering only ASCII characters, or should it work for Unicode as well?
- Should the function be case-sensitive?
2. Analyze the Requirements
Once you’ve clarified the problem, dig deeper into the requirements:
- Identify any constraints (e.g., time complexity, space complexity)
- Consider edge cases and special scenarios
- Think about scalability requirements
For instance, if you’re working on a sorting algorithm, you might consider:
- What’s the expected size of the input?
- Does it need to be stable?
- Is the data likely to be partially sorted already?
- Are there memory constraints to consider?
3. Design the Solution
With a clear understanding of the problem and its requirements, it’s time to design your solution:
- Brainstorm multiple approaches
- Consider trade-offs between different solutions
- Choose the most appropriate data structures and algorithms
- Sketch out the high-level structure of your code
For example, if you’re designing a solution for finding the k-th largest element in an unsorted array, you might consider:
- Sorting the entire array (O(n log n) time complexity)
- Using a min-heap of size k (O(n log k) time complexity)
- Using QuickSelect algorithm (O(n) average time complexity)
4. Implement the Solution
Now that you have a solid plan, it’s time to write the code:
- Start with a basic implementation
- Use meaningful variable and function names
- Write clean, modular code
- Add comments to explain complex logic
Here’s an example of how you might start implementing a solution for finding the longest substring without repeating characters:
def longest_substring_without_repeats(s):
char_index = {}
start = 0
max_length = 0
for i, char in enumerate(s):
if char in char_index and char_index[char] >= start:
start = char_index[char] + 1
else:
max_length = max(max_length, i - start + 1)
char_index[char] = i
return max_length
5. Test and Refine
The final step is to thoroughly test your solution and refine it as needed:
- Test with various inputs, including edge cases
- Analyze the time and space complexity
- Optimize the code if necessary
- Consider writing unit tests
- “abcabcbb” (expected output: 3)
- “bbbbb” (expected output: 1)
- “pwwkew” (expected output: 3)
- “” (expected output: 0)
- Explain your thought process
- Discuss trade-offs between different approaches
- Provide regular updates on your progress
- Be open to feedback and suggestions
- Consider how your solution fits into the overall architecture
- Think about maintainability and scalability
- Consider potential future requirements
- Align your solution with the client’s long-term goals
- Write clear, concise comments in your code
- Provide a high-level overview of your solution
- Document any assumptions or decisions you made
- Include instructions for running and testing the code
- Keep learning new programming languages and frameworks
- Stay informed about emerging technologies
- Participate in coding challenges and hackathons
- Engage with the developer community through forums and conferences
- Wasted time implementing the wrong solution
- Overlooking important edge cases
- Difficulty explaining your approach to others
- Avoid adding unnecessary complexity
- Don’t optimize prematurely
- Focus on meeting the current requirements first
- Write readable and maintainable code
- Follow naming conventions
- Use appropriate error handling
- Write unit tests
- Don’t make assumptions without verifying
- Keep stakeholders informed of your progress
- Be prepared to explain your solution in both technical and non-technical terms
- LeetCode: Practice coding problems and prepare for technical interviews
- HackerRank: Improve your coding skills and participate in coding challenges
- CodeSignal: Take coding assessments and showcase your skills to potential clients
- Git: Essential for tracking changes and collaborating with others
- GitHub: Host your projects and contribute to open-source
- GitLab: Manage your entire DevOps lifecycle
- Visual Studio Code: A versatile, lightweight code editor
- JetBrains IDEs: Powerful, language-specific IDEs (e.g., IntelliJ IDEA, PyCharm)
- Sublime Text: A fast, customizable text editor
- Confluence: Collaborate on documentation and project planning
- Notion: Create and organize documentation, notes, and project plans
- Swagger: Design, build, and document APIs
- Set aside time each day or week for coding practice
- Work on a variety of problem types to broaden your skills
- Participate in coding competitions or hackathons
- Study solution discussions on coding platforms
- Participate in code reviews
- Collaborate on open-source projects
- Ask colleagues or mentors to review your solutions
- Participate in coding workshops or meetups
- Be open to constructive criticism
- Write blog posts or tutorials about coding concepts
- Mentor junior developers
- Give presentations or workshops on technical topics
For the longest substring problem, you might test with inputs like:
Best Practices for Consultants
As a consultant, there are additional best practices to keep in mind when approaching coding problems:
Communicate Clearly
Throughout the problem-solving process, maintain clear communication with your client or team:
Consider the Bigger Picture
Remember that the coding problem you’re solving is likely part of a larger system or project:
Document Your Work
Proper documentation is crucial for consultants:
Stay Updated with Industry Trends
The tech industry is constantly evolving, so it’s important to stay current:
Common Pitfalls to Avoid
As you approach coding problems, be aware of these common pitfalls:
Rushing to Code
One of the biggest mistakes is starting to code before fully understanding the problem. This can lead to:
Overengineering
While it’s important to create robust solutions, be careful not to overengineer:
Ignoring Best Practices
In the rush to solve a problem, don’t forget about coding best practices:
Failing to Communicate
As a consultant, clear communication is crucial:
Tools and Resources for Consultants
To enhance your problem-solving skills and stay competitive as a consultant, consider using these tools and resources:
Online Coding Platforms
Version Control Systems
IDEs and Code Editors
Documentation Tools
Continuous Improvement
As a consultant, your journey of learning and improvement never ends. Here are some strategies to continually enhance your problem-solving skills:
Practice Regularly
Consistent practice is key to improving your coding skills:
Analyze and Learn from Others
Don’t just solve problems; learn from how others approach them:
Seek Feedback
Actively seek feedback on your problem-solving approach and code:
Teach Others
Teaching is an excellent way to solidify your own understanding:
Conclusion
Approaching coding problems as a consultant requires a blend of technical skills, problem-solving abilities, and effective communication. By focusing on understanding the problem first and following a structured approach, you can create solutions that not only solve the immediate issue but also provide long-term value to your clients.
Remember, the key steps are:
- Clarify the problem
- Analyze the requirements
- Design the solution
- Implement the solution
- Test and refine
By consistently applying these principles and continually improving your skills, you’ll be well-equipped to tackle any coding challenge that comes your way as a consultant. Whether you’re preparing for technical interviews at top tech companies or working on complex projects for clients, this approach will serve you well in your journey as a software development consultant.
Remember, the goal is not just to write code that works, but to create solutions that are efficient, maintainable, and aligned with your client’s needs. By focusing on understanding first and approaching problems methodically, you’ll be able to deliver high-quality results and build a strong reputation as a skilled and reliable consultant in the competitive world of software development.